Common Pitfalls When Implementing Protocol Buffers in Java
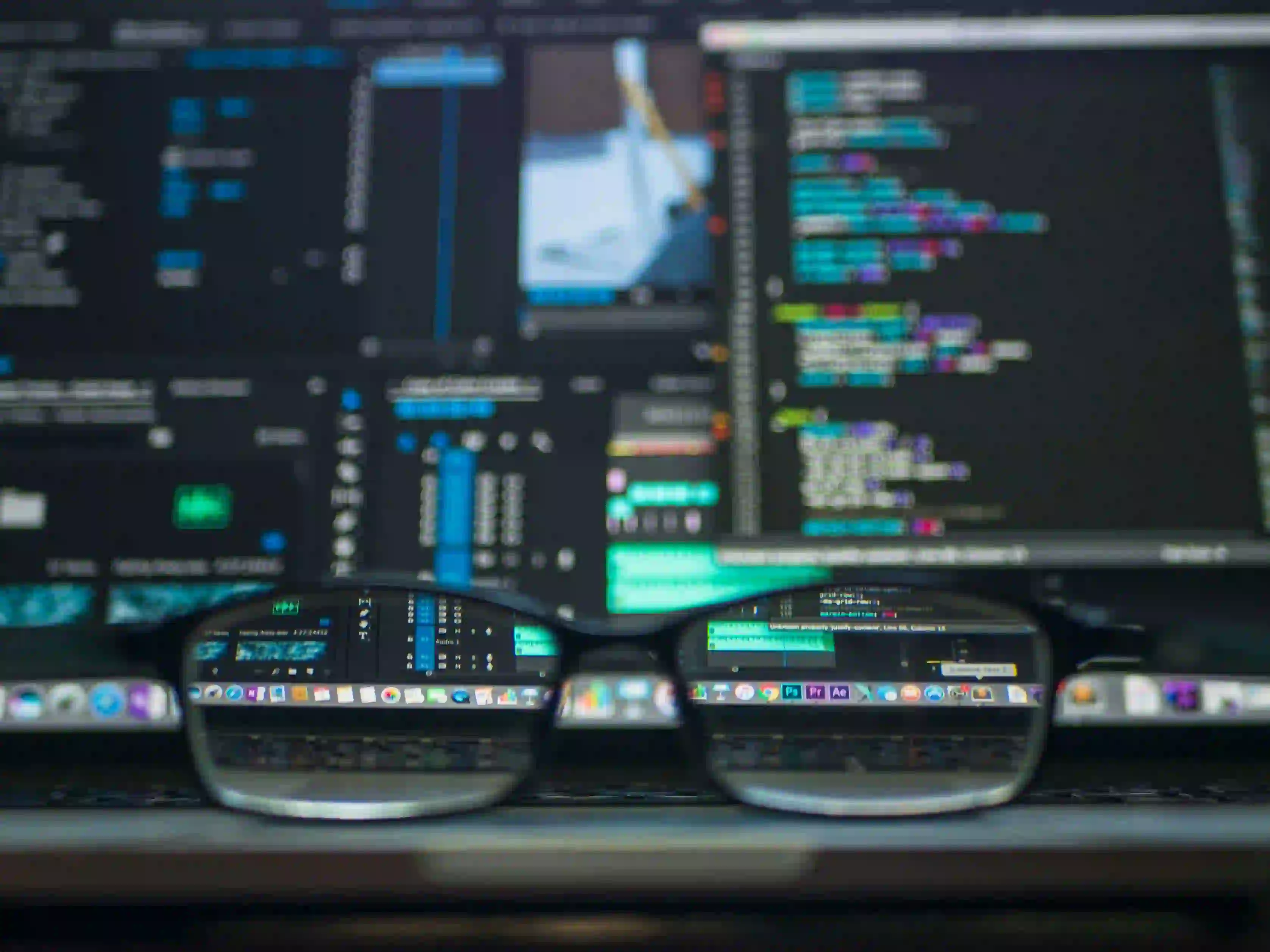
Common Pitfalls When Implementing Protocol Buffers in Java
Protocol Buffers (Protobuf) is a powerful serialization format developed by Google. It's widely used for effective communication between services, especially in microservices architecture. Despite its advantages, developers often encounter challenges while implementing Protocol Buffers in Java. This blog post will dive into these common pitfalls, accompanied by code snippets and practical insights to help you avoid these traps.
What Are Protocol Buffers?
At its core, Protocol Buffers is a clean and efficient method of serializing structured data. Unlike XML or JSON, Protobuf reduces the size of the data while improving performance, making it ideal for APIs and data storage.
1. Misunderstanding the Syntax of .proto Files
The first pitfall many developers face is misunderstanding the syntax of .proto
files. A .proto
file serves as the contract between the sender and the receiver.
Example of a Simple .proto File
syntax = "proto3";
message Person {
string name = 1;
int32 id = 2;
repeated string email = 3;
}
Why This Matters
Each line in a .proto
file represents a field and its properties—such as data type and field number. Misdefining these can lead to data inconsistencies or, worse, runtime errors. Always validate your .proto
file in the Protobuf compiler to ensure accuracy.
2. Ignoring Field Numbers
Field numbers in Protocol Buffers are essential. Once assigned, you should not change them. Field numbers are used to match serialized data fields with their respective definitions in code.
Example of Correct Usage
message AddressBook {
repeated Person people = 1; // Field number must be unique
}
Why This Matters
If you change field numbers after having serialized data using an older version of your schema, Protobuf may not deserializing the data correctly, leading to data loss or corruption.
3. Overusing repeated
Fields
Fields in a message can be repeated
, which means zero or more of that field can be present. While multiple entries might seem beneficial, overusing this can cause performance issues and complicate data management.
Example of Misuse
message Organization {
string name = 1;
repeated string departments = 2; // Potential performance hit
}
Why This Matters
Using repeated
fields unnecessarily bloats your data, impacting processing time and performance. If an entity logically should not have multiple instances (like a unique ID), consider using a single occurrence field.
4. Not Handling Missing Fields
In Protobuf, fields can be optional. If a field is omitted during serialization, it won't be part of the byte stream. Java clients must recognize this.
Example of Handling Missing Fields
Person p = Person.newBuilder().setName("John").build();
// No ID is set, it defaults to 0, so check for that
if (p.getId() == 0) {
System.out.println("ID is missing!");
}
Why This Matters
Failing to account for missing fields can lead to NullPointerExceptions. Always validate your parsed data before using it!
5. Not Registering Your Data Types
When working with Protobuf in Java, you must ensure that your generated classes are registered correctly with your serialization framework. If you don't do this, you might run into ClassNotFoundException errors.
Example of Registration
import com.google.protobuf.Descriptors;
// Registering the Person class
Descriptors.Descriptor descriptor = Person.getDescriptor();
Why This Matters
Unregistered classes can't be serialized/deserialized correctly. Pay close attention to ensure everything registers at the start of your application.
6. Forgetting Backward Compatibility
Backward compatibility is crucial in any production API. Protocol Buffers inherently support this, but nonchalantly making changes, like adding or removing fields, can break existing clients.
Example of Best Practices
message Car {
string make = 1;
string model = 2;
int32 year = 3; // Adding a new field (3) is safe.
}
Why This Matters
Ensure your .proto
files are designed with extensibility in mind. This means using optional fields and avoiding field number rearrangements.
7. Not Understanding Default Values
Fields in Protobuf have implicit default values. For instance, an int32
defaults to 0, a bool
defaults to false, and a string
defaults to an empty string. Misunderstanding default values can lead to bugs.
Example of Default Values
message User {
string username = 1;
bool active = 2; // Defaults to false
}
Why This Matters
Developers might assume fields are populated, which can introduce bugs. Always check fields explicitly if they contain meaningful data.
8. Skipping Code Generation Steps
Generating Java classes from .proto
files is a crucial step. Failing to do this will leave you with your data schema only, but no usable classes.
Command for Code Generation
protoc --java_out=src/main/java src/main/proto/*.proto
Why This Matters
Without the generated classes, your code won't compile. Automate this step in your build process to avoid headaches in the future.
My Closing Thoughts on the Matter
Implementing Protocol Buffers in Java can be a rewarding endeavor, but it comes with its challenges. By avoiding these common pitfalls—such as misunderstanding .proto
file syntax, mismanaging field numbers, and neglecting backward compatibility—you can leverage the full potential of the Protobuf serialization format.
For further reading, consider exploring the official Protocol Buffers documentation or the Java implementation guidelines to deepen your understanding.
By staying informed and vigilant, you'll be well on your way to mastering Protocol Buffers in Java. Happy coding!