Less Verbosity: Streamlining Java Code for Developers
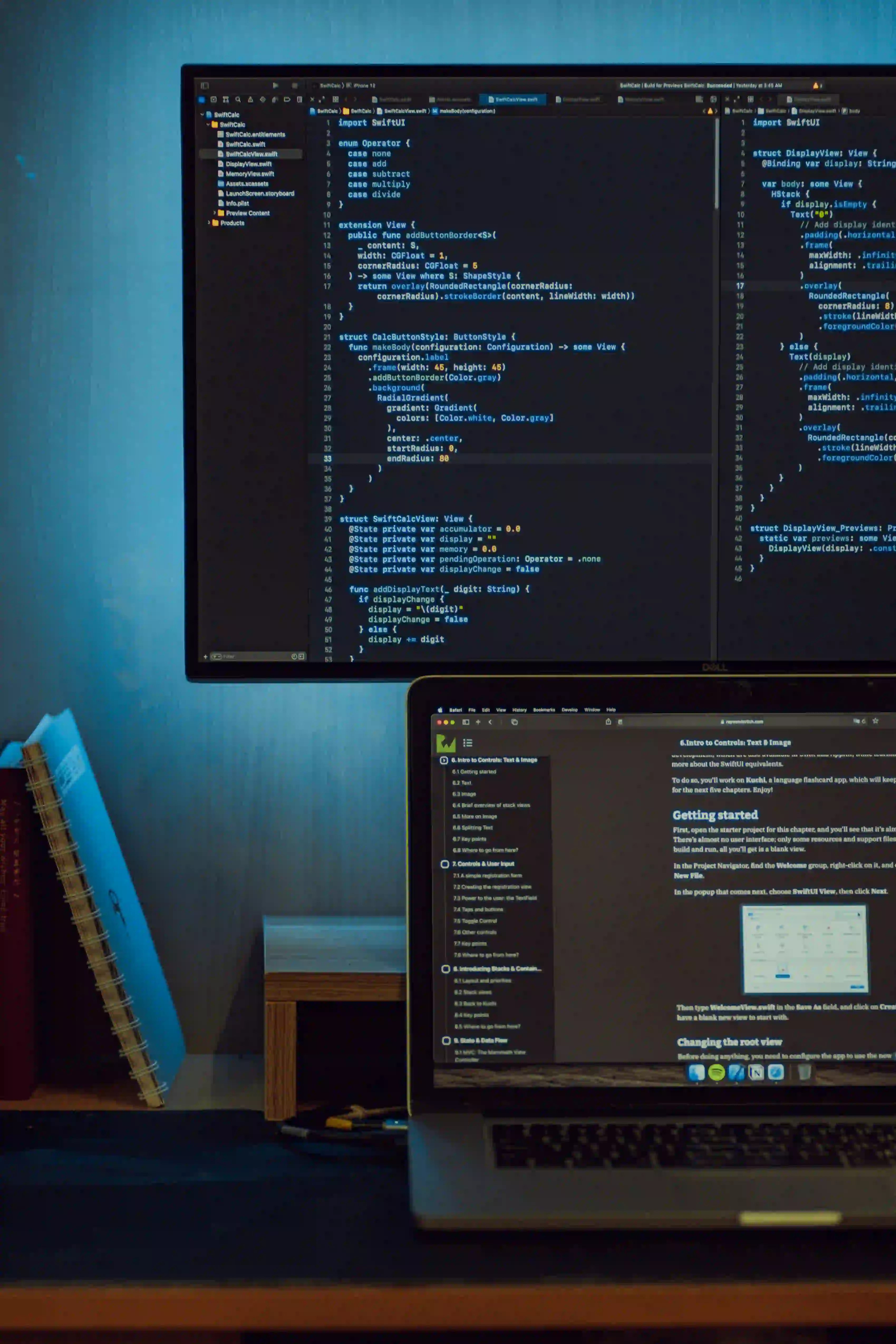
Less Verbosity: Streamlining Java Code for Developers
In the world of Java programming, verbosity can sometimes hinder the development process. Excessively long classes, methods, and even variable declarations can make code cumbersome and difficult to read. Fortunately, there are effective strategies for streamlining Java code. In this blog post, we'll explore key techniques to simplify your Java code, improve readability, and enhance maintainability.
Understanding the Problem with Verbosity
Java is a strongly typed, object-oriented programming language. As a result, it can often lead to code that is more verbose than necessary. This verbosity may seem beneficial at first—providing clarity and structure—but it can also mask the logic, making it more challenging to maintain or extend.
To illustrate, consider the following overly verbose Java class:
public class UserDetail {
private String firstName;
private String lastName;
private int age;
public UserDetail(String fn, String ln, int a) {
this.firstName = fn;
this.lastName = ln;
this.age = a;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public int getAge() {
return age;
}
}
While this class works perfectly fine, it is quite lengthy. The goal is to retain the structure while reducing verbosity.
Todo: Use Constructors Wisely
One straightforward approach to streamline your code is to utilize constructors effectively. In the example above, we can take advantage of Java 14's Record feature to reduce verbosity significantly. A record in Java is a special kind of class meant for immutable data.
Here's how you can apply it:
public record UserDetail(String firstName, String lastName, int age) {}
Why Use Records?
- Less Boilerplate: There’s no need for getters or constructors.
- Immutability: Records are immutable by design, making your objects inherently thread-safe.
Leveraging Lambda Expressions
Another way to trim down your code in Java is through the use of lambda expressions. Introduced in Java 8, lambda expressions enable you to implement functional interfaces in a much less verbose yet readable manner.
Consider the traditional way of creating a thread:
public class Example {
public static void main(String[] args) {
Runnable runnable = new Runnable() {
public void run() {
System.out.println("Thread is running");
}
};
Thread thread = new Thread(runnable);
thread.start();
}
}
This can be simplified to:
public class Example {
public static void main(String[] args) {
Thread thread = new Thread(() -> System.out.println("Thread is running"));
thread.start();
}
}
Why Use Lambda Expressions?
- Conciseness: They reduce the amount of boilerplate code.
- Improved Readability: Similar functionalities are easier to follow at a glance.
Utilizing Method References
In addition to lambda expressions, Java also supports method references, which provide a concise way to refer to methods. Instead of writing out the full lambda, you can typically replace it with a method reference.
Let’s modify our previous example using method references:
import java.util.Arrays;
public class Example {
public static void main(String[] args) {
String[] names = {"Alice", "Bob", "Charlie"};
Arrays.sort(names, String::compareToIgnoreCase);
System.out.println(Arrays.toString(names)); // Output: [Alice, Bob, Charlie]
}
}
Why Use Method References?
- Simplicity: They remove the explicit lambda syntax.
- Clarity: It leads to cleaner code since the intent of the method is made clearer.
Use of Streams for Collections
Before Java 8, working with collections could result in verbose and complex logic. By utilizing the Stream API, we can effectively reduce the verbosity of operations like filtering, mapping, and collecting.
Here's how you might traditionally filter a list of numbers in Java:
import java.util.ArrayList;
import java.util.List;
public class Example {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
numbers.add(4);
List<Integer> evenNumbers = new ArrayList<>();
for (Integer number : numbers) {
if (number % 2 == 0) {
evenNumbers.add(number);
}
}
System.out.println(evenNumbers);
}
}
This can be streamlined using streams:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Output: [2, 4]
}
}
Why Use Streams?
- Expressiveness: Code becomes more declarative, focusing on what to achieve.
- Performance: Streams can leverage multi-core architectures to improve performance with parallel processing.
Avoiding Unnecessary Code
Refactoring is an essential aspect of clean code. A crucial part of this is eliminating unnecessary code. Duplicate methods, excessive comments, or complex logic that can be simplified should always be scrutinized.
For example, let’s say you have a method that calculates the sum of two numbers. If that method is duplicated, consider consolidating it:
public int add(int a, int b) {
return a + b;
}
// Later in your code...
int result1 = add(5, 10);
int result2 = add(15, 20);
By reducing the number of times the same logic appears, you improve clarity and ease of maintenance.
Why Eliminate Unnecessary Code?
- Maintainability: Less code means fewer places to keep track of changes.
- Readability: Clear and concise code is easier to understand at first glance.
My Closing Thoughts on the Matter
Streamlining Java code is not just about making it shorter; it’s about making it more understandable and maintainable. By adopting techniques such as using records, lambda expressions, method references, streams, and diligent refactoring, Java developers can enhance their code without sacrificing quality.
The less verbose your code is, the easier it becomes for others to understand, modify, and build upon it. It also lowers the chances of bugs and increases productivity. So, next time you write your Java code, keep these principles in mind, and make your code less verbose, while retaining its clarity and functionality.
If you're just starting with Java or want to dive deeper, don't forget to check resources like Oracle's Java Tutorials and Effective Java by Joshua Bloch for further insights into best practices.
Happy coding!