Understanding Database Deadlocks in Multi-Tier Applications
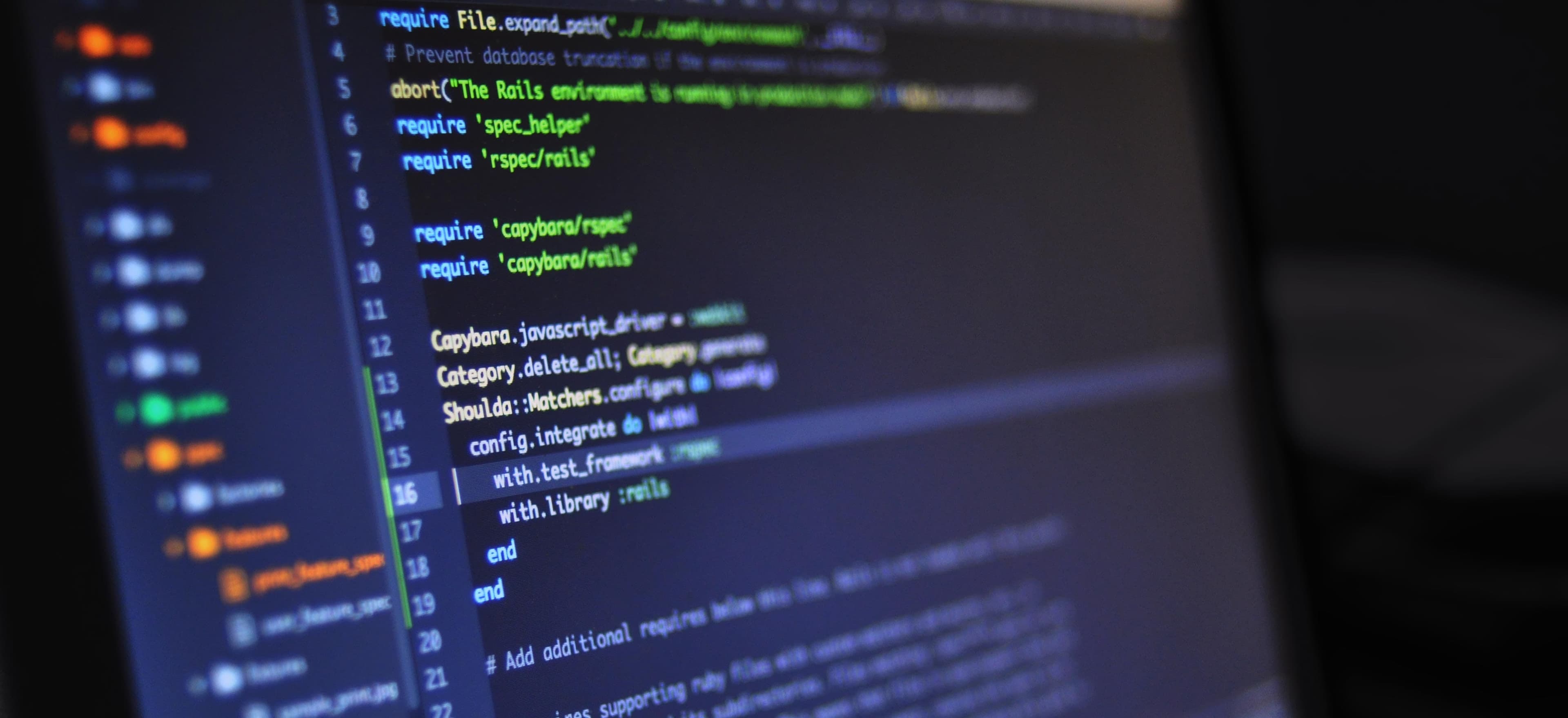
- Published on
Understanding Database Deadlocks in Multi-Tier Applications
In today's world of complex software architectures, the intricacies of multi-tier applications are often paired with the challenges of managing database operations. One of the most critical issues that developers encounter is database deadlocks. This blog post aims to elucidate the concept of deadlocks, their causes, and various strategies to handle them effectively.
What is a Database Deadlock?
A deadlock occurs when two or more transactions or processes are blocked forever, each waiting for the other to release resources. Imagine a scenario where two drivers are encountered at a narrow bridge, each waiting for the other to back up. In simpler terms, in the realm of databases, a deadlock leads to a halt in operation, affecting your application's performance and user experience.
For example, let's say two concurrent transactions are trying to update two different rows in the same table:
- Transaction A locks Row 1 and wants to lock Row 2.
- Transaction B locks Row 2 and wants to lock Row 1.
As both transactions wait for each other to release locks, a deadlock is formed.
The Technical Perspective of a Deadlock
Here's a simplified visual representation of a potential deadlock:
Transaction A: Locks Row 1 -> Wants Row 2
Transaction B: Locks Row 2 -> Wants Row 1
Causes of Deadlocks
- Concurrency: When multiple transactions are trying to execute simultaneously, the chances of deadlocks increase.
- Order of Operations: If the order in which locks are acquired varies across transactions, it can cause deadlocks.
- Long Transactions: Transactions that take longer to complete tend to hold locks for extended periods, leading to higher deadlock chances.
Detecting Deadlocks
Most modern database management systems (DBMS) such as MySQL, PostgreSQL, and SQL Server come equipped with mechanisms to detect deadlocks. Here’s how you can visualize this in SQL:
SET LOCK_TIMEOUT 1000; -- Set timeout for locking
BEGIN TRANSACTION;
-- Acquire a lock on Row A
UPDATE Table1 SET column1 = 'Value' WHERE id = 'A';
-- Attempt to acquire a lock on Row B
UPDATE Table1 SET column1 = 'Value' WHERE id = 'B';
COMMIT TRANSACTION;
If Transaction A tries to lock Row B while holding Row A, and Transaction B is trying to lock Row A, the DBMS typically throws a deadlock error.
Recovery from Deadlocks
- Timeouts: In most systems, if a transaction waits too long to acquire a lock, it is rolled back, allowing other transactions to proceed.
- Deadlock Detection Algorithms: Databases like SQL Server employ algorithms to periodically check for deadlocks. If detected, the DBMS may automatically terminate one transaction to break the deadlock.
Best Practices to Avoid Deadlocks
1. Ordering
Ensure that all transactions acquire locks in a consistent order. For instance, if one transaction always locks Row A before Row B, all other transactions should follow the same order.
// Java Pseudo-code for acquiring locks in a consistent order
synchronized(lockA) {
synchronized(lockB) {
// Perform operations
}
}
This simple ordering dramatically decreases the likelihood of deadlocks, as there’s a clear and consistent path for how locks are obtained.
2. Keep Transactions Short
Limit the duration of transactions, ensuring they hold locks for minimal time. The longer they run, the higher the probability they'll interfere with other transactions.
-- Sample SQL transaction
BEGIN TRANSACTION;
-- Execute only necessary operations
UPDATE table SET column = value WHERE condition;
COMMIT TRANSACTION;
By reducing the number of operations within a transaction, you'll minimize lock contention.
3. Use Appropriate Isolation Levels
Understanding the isolation levels can also help in avoiding deadlocks. The 'READ COMMITTED' level provides a good balance between consistency and concurrency. By using it, even if two transactions try to read and write simultaneously, the chances of a deadlock are significantly reduced.
Additional Techniques
4. Implementing Retry Logic
When a transaction fails due to a deadlock, implementing retry logic allows the application to attempt the transaction again. This is often done in a loop with a defined maximum number of retries:
public void safeExecute(Runnable task) {
int attempts = 0;
while (attempts < MAX_RETRIES) {
try {
task.run();
break; // Exit if successful
} catch (DeadlockException e) {
attempts++;
// Optionally log the retry attempt
}
}
}
This method promotes resilience within your application, allowing it to recover from transient failures.
5. Analyzing Execution Plans
Leverage your Database Management System to analyze the execution plans for your queries. With tools like SQL Server Management Studio, you can dynamically assess how your queries run and optimize them accordingly.
You may delve deeper into how deadlock detection works by checking out Microsoft’s documentation on deadlocks and blocking.
The Bottom Line
While database deadlocks may seem daunting, understanding their causes, detection, and avoidance strategies can greatly enhance the reliability of multi-tier applications. By implementing tight locking protocols, managing transaction lengths, and employing retry logic, developers can not only prevent deadlocks but also create robust applications that perform effectively even under heavy loads.
Understanding deadlocks is an essential part of designing scalable, high-performance applications. As you delve deeper into your database management practices, always keep deadlocks in mind. They may seem elusive, but with the right strategies, you can manage them effectively.
By focusing on these essential aspects of database management within multi-tier applications, you’ll not only optimize performance but also ensure a smoother user experience.
Checkout our other articles