Handling JPA Transactions in Asynchronous Processing
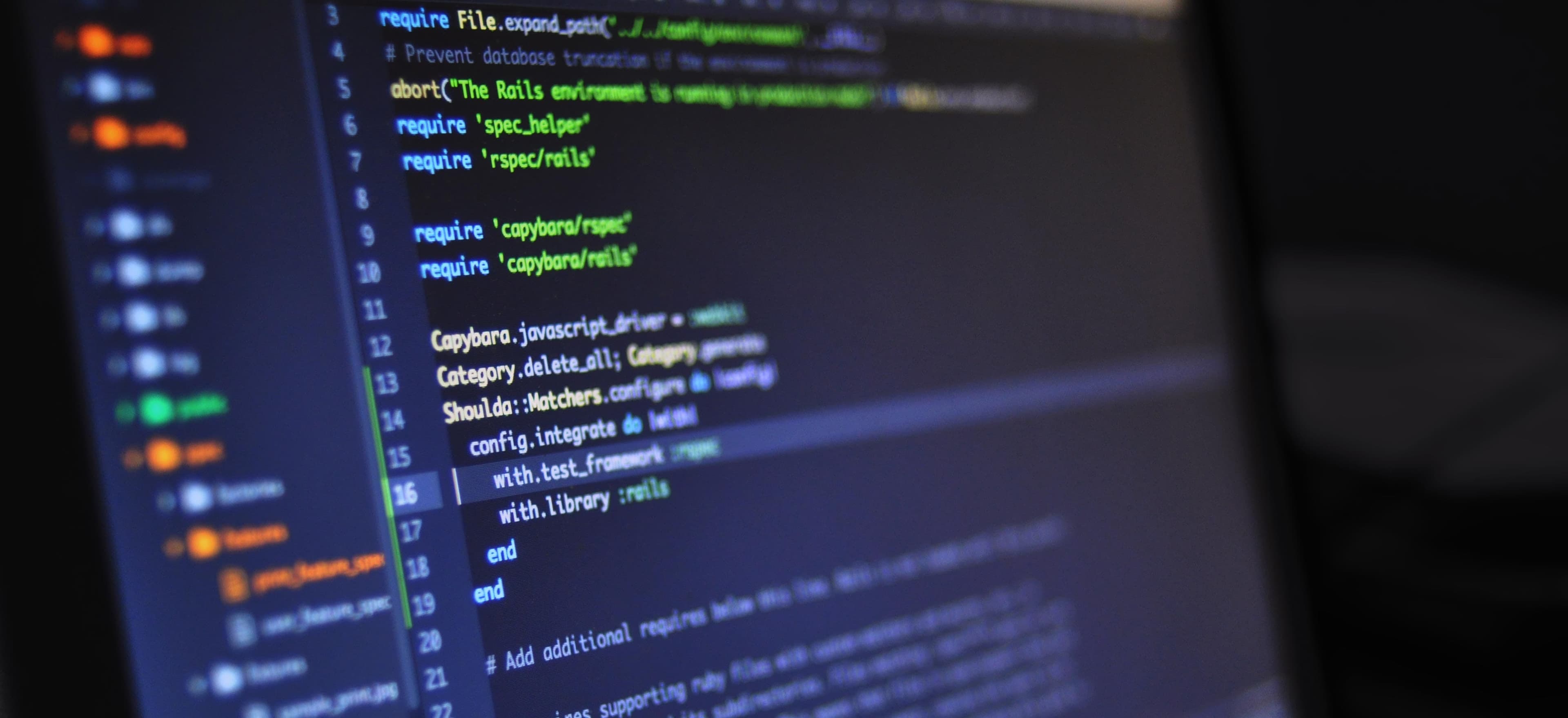
- Published on
Handling JPA Transactions in Asynchronous Processing
In today's application development landscape, the need for handling asynchronous processing and database transactions is more common than ever. Developers are constantly looking for efficient ways to manage data persistence while maintaining performance. With Java Persistence API (JPA) and the introduction of Java's CompletableFuture
for asynchronous programming, developers can simplify the interactions with the database.
This blog post will guide you through the essentials of handling JPA transactions in asynchronous processing while highlighting best practices, pitfalls, and exemplary code snippets.
Understanding the Basics of JPA and Transactions
JPA is a specification that provides a systematic way to manage relational data in Java applications. It abstracts the information stored in relational databases and allows developers to map Java objects to database tables. Transactions ensure data integrity and consistency, particularly vital in multi-user environments.
Transactions in JPA typically revolve around two key components:
- EntityManager: This is the primary JPA interface used to interact with the persistence context.
- Transaction Control: This includes managing the lifecycle of transactions such as beginning, committing, and rolling back.
Example Code Snippet: Basic JPA Transaction
import javax.persistence.EntityManager;
import javax.persistence.EntityTransaction;
public void saveEntity(MyEntity entity, EntityManager entityManager) {
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
entityManager.persist(entity);
transaction.commit();
} catch (RuntimeException e) {
transaction.rollback();
throw e; // Consider logging the error
}
}
This code snippet demonstrates the basic structure of managing a transaction using JPA. A transaction is initiated, an entity is persisted, and then the transaction is committed. If an exception occurs, a rollback is performed to prevent any inconsistent state.
The Shift to Asynchronous Processing
Asynchronous processing allows an application to perform multiple tasks concurrently, improving performance and user experience. In Java, the CompletableFuture
class provides a powerful way to manage asynchronous operations. However, integrating JPA transactions in this model requires a careful approach.
When working with JPA and CompletableFuture
, there are important considerations to ensure that transactions are managed correctly.
Key Considerations When Combining JPA with Asynchronous Tasks
-
Thread Safety: The
EntityManager
is not thread-safe. It should only be accessed within the same thread where it was created. This means that if you are performing database operations asynchronously, you cannot reuse the sameEntityManager
across threads. -
Transaction Scope: JPA EntityManager's transaction scope must be clear. You should manage transactions explicitly within the asynchronous task. This means starting and committing/rolling back transactions within the same thread.
-
Transaction Propagation: If you are using frameworks like Spring, understanding transaction propagation behaviors (such as
REQUIRES_NEW
) can be crucial in controlling transaction boundaries within asynchronous processing.
Example Code Snippet: Asynchronous Entity Save
Below is an example that illustrates how to handle JPA transactions effectively in an asynchronous environment.
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import java.util.concurrent.CompletableFuture;
public class AsyncService {
private final EntityManagerFactory entityManagerFactory;
public AsyncService(EntityManagerFactory entityManagerFactory) {
this.entityManagerFactory = entityManagerFactory;
}
public CompletableFuture<Void> saveEntityAsync(MyEntity entity) {
return CompletableFuture.runAsync(() -> {
EntityManager entityManager = entityManagerFactory.createEntityManager();
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
entityManager.persist(entity);
transaction.commit();
} catch (Exception e) {
if (transaction.isActive()) {
transaction.rollback();
}
throw e; // Log the exception as necessary
} finally {
entityManager.close();
}
});
}
}
Explanation of the Code
- EntityManagerFactory: This is injected into the service to create new
EntityManager
instances for each request. - CompletableFuture.runAsync: The async operation is encapsulated in a
CompletableFuture
, allowing it to run in a separate thread. - Transaction Management: The transaction is wrapped within the asynchronous method, ensuring that it is unique to the thread of execution.
- Resource Management: The
EntityManager
is closed whether the transaction is successful or an exception occurs.
Error Handling in Asynchronous Transactions
When working asynchronously, error handling must ensure that all resources are released properly. In the example above, the finally
block ensures that the EntityManager
is closed, preventing memory leaks.
Using Custom Exception Handling
You can create custom exceptions to be thrown in the event of errors during the asynchronous operations. This practice helps in managing different failure scenarios systematically.
public class EntitySaveException extends RuntimeException {
public EntitySaveException(String message, Throwable cause) {
super(message, cause);
}
}
// In the AsyncService class
throw new EntitySaveException("Error saving entity", e);
Increasing Scalability with Connection Pooling
When handling multiple asynchronous requests, a well-designed connection pooling strategy plays a crucial role. By using a connection pool, you can manage multiple database connections efficiently, which is particularly important for high-load applications.
Frameworks like HikariCP are popular for effective connection pooling in Java applications.
Example Configuration for HikariCP:
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.0.0</version>
</dependency>
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("user");
config.setPassword("password");
config.setMaximumPoolSize(10);
HikariDataSource ds = new HikariDataSource(config);
By integrating HikariCP with JPA, you can manage connections efficiently while ensuring that your application remains responsive under load.
Testing Asynchronous JPA Transactions
To ensure that async operations work correctly, comprehensive testing is essential. You can use JUnit and Mockito to write unit tests for your async service.
Example Test Case:
import static org.mockito.Mockito.*;
@Test
public void testSaveEntityAsync() {
// Create mocks for EntityManager and EntityManagerFactory
EntityManagerFactory entityManagerFactory = mock(EntityManagerFactory.class);
EntityManager entityManager = mock(EntityManager.class);
when(entityManagerFactory.createEntityManager()).thenReturn(entityManager);
AsyncService service = new AsyncService(entityManagerFactory);
MyEntity entity = new MyEntity("Sample");
CompletableFuture<Void> future = service.saveEntityAsync(entity);
future.join(); // Join to wait for completion
verify(entityManager).persist(entity); // Check if entity was persisted
verify(entityManager).close(); // Ensure EntityManager is closed
}
Final Thoughts
Handling JPA transactions in asynchronous processing is a critical skill for modern Java developers. By adhering to the principles highlighted in this blog post, you can effectively manage persistence in an asynchronous environment while ensuring data integrity and performance.
In summary:
- Be mindful of the thread-safety of
EntityManager
. - Manage transactions within their own scope per asynchronous task.
- Implement comprehensive error handling and resource management.
- Consider scalability with appropriate connection pooling.
- Don’t neglect testing to ensure your implementation is robust.
By mastering these concepts, developers can maximize the potential of both JPA and asynchronous processing, leading to efficient and scalable Java applications.
For more insights into best practices for JPA and asynchronous programming, check out the official JPA documentation and related resources.
Happy coding!
Checkout our other articles