Overcoming Bottlenecks in High-Performance App Development
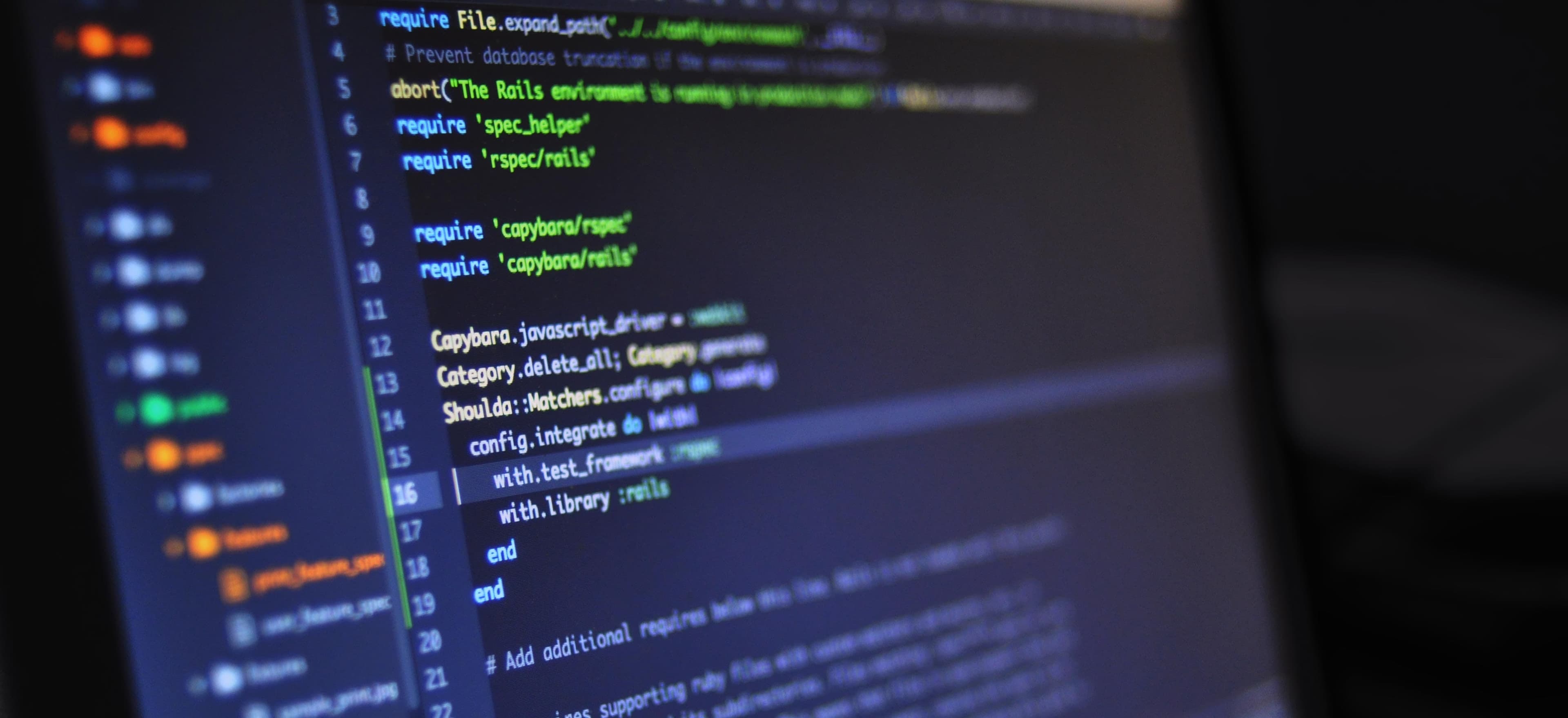
- Published on
Overcoming Bottlenecks in High-Performance App Development
In today’s fast-paced tech landscape, developing high-performance applications is not just an asset; it’s a necessity. Users demand efficiency and speed, and any bottleneck in the application’s performance can lead to dissatisfaction and abandonment. In this blog post, we will dive into common bottlenecks encountered in app development and discuss effective strategies for overcoming these hurdles.
Understanding Bottlenecks
Bottlenecks in software development are points in the system where performance is significantly hampered, causing delays or failures. These can arise from multiple sources including:
- Inefficient Algorithms: Poorly optimized code can slow down response times.
- Database Concurrency Issues: High traffic can lead to database lock contention.
- Network Latency: Delays in server communication can slow down data retrieval.
Recognizing and diagnosing these bottlenecks is the first step to enhancing application performance.
Common Bottlenecks and Their Solutions
1. Algorithm Inefficiency
Inefficient algorithms are often the root of slow application performance. For example, a naive sorting algorithm can exponentially increase execution time as the dataset grows.
Example Code Snippet:
public void naiveSort(int[] array) {
for (int i = 0; i < array.length; i++) {
for (int j = i + 1; j < array.length; j++) {
if (array[i] > array[j]) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
}
}
Commentary:
The above code uses a simple nested loop for sorting, leading to O(n^2) complexity. For larger datasets, this will severely slow down your application. Instead, utilize more efficient algorithms, such as QuickSort or MergeSort, which offer O(n log n) complexity.
Optimized Code Snippet:
import java.util.Arrays;
public void optimizedSort(int[] array) {
Arrays.sort(array); // Uses Dual-Pivot Quicksort
}
Why This Help:
By using Java's built-in sorting function, you leverage optimized algorithms that outperform naive approaches. This transition can drastically reduce execution time in sorting tasks.
2. Database Performance
High-concurrent access to databases can lead to contention, causing a bottleneck in application response time.
Example Scenario:
Imagine an e-commerce platform with many simultaneous users trying to check out simultaneously. If your database locks the entire table, users must wait for their turn.
Solution:
- Optimistic Concurrency Control: Use versioning rather than locks to handle updates.
- Indexing: Implement proper indexing to speed up query performance.
Optimized Query Code:
CREATE INDEX idx_user_email ON users(email);
Why This Help:
Indexes drastically reduce the amount of data scanned during query execution. For frequently searched fields, such as user emails, this can lead to substantial performance gains.
3. Caching Strategies
Achieving a seamless user experience often hinges on how well your app manages data retrieval. Repeatedly accessing the database can add latency.
Solution:
Use caching mechanisms to store frequently accessed data temporarily.
Example Code Snippet:
import java.util.HashMap;
public class SimpleCache {
private HashMap<String, String> cache = new HashMap<>();
public void addToCache(String key, String value) {
cache.put(key, value);
}
public String getFromCache(String key) {
return cache.getOrDefault(key, null);
}
}
Commentary:
Here, a simple in-memory cache is implemented to quickly retrieve values. Although rudimentary, this showcases the basic concept. More robust solutions like Ehcache or Redis can be adopted based on specific requirements.
Why This Helps:
Caching reduces the frequency of database access, leading to quicker response times and an overall smoother experience.
4. Network Latency
In web applications, slow network calls can hinder performance significantly.
Solution:
- Minimize Requests: Reduce the total number of HTTP requests.
- Asynchronous Communication: Use asynchronous calls to prevent blocking.
Example Code Snippet:
import java.net.HttpURLConnection;
import java.net.URL;
public void fetchDataAsync(String apiUrl) {
new Thread(() -> {
try {
URL url = new URL(apiUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.connect();
// Process the response...
} catch (Exception e) {
e.printStackTrace();
}
}).start();
}
Why This Helps:
By using multi-threading, we can handle network calls without blocking the main thread, allowing the application to remain responsive while waiting for data.
Profiling Your Application
Bottlenecks vary by application, making it essential to perform profiling. Profiling tools can help reveal areas needing improvement.
Recommended Tools:
- JProfiler: A powerful profiling tool for Java applications.
- VisualVM: A visual tool to monitor and troubleshoot Java applications.
By using these tools, developers can obtain performance insights and diagnose bottlenecks effectively.
Testing and Optimization
Once you have identified potential bottlenecks, rigorous testing is critical.
- Load Testing: Simulate high-traffic scenarios to observe how the application handles it.
- Benchmarking: Measure performance metrics before and after optimizations to ensure improvements are genuine.
By conducting these tests, developers can create a responsive application, enhancing overall user satisfaction.
The Bottom Line
Overcoming bottlenecks in high-performance app development requires diligence and strategy. By understanding the root causes, employing optimized techniques, leveraging effective caching mechanisms, and utilizing profiling tools, developers can significantly enhance their applications' performance.
For developers interested in deepening their understanding of application performance, I recommend checking out these resources:
- Effective Java by Joshua Bloch - A must-read for Java developers.
- Designing Data-Intensive Applications by Martin Kleppmann - Provides insights into data handling and optimizations.
By adapting the techniques discussed in this post, you can ensure your application meets user demands in today’s competitive market. Happy coding!