Mastering Android Copy-Paste: Intent Failures Explained
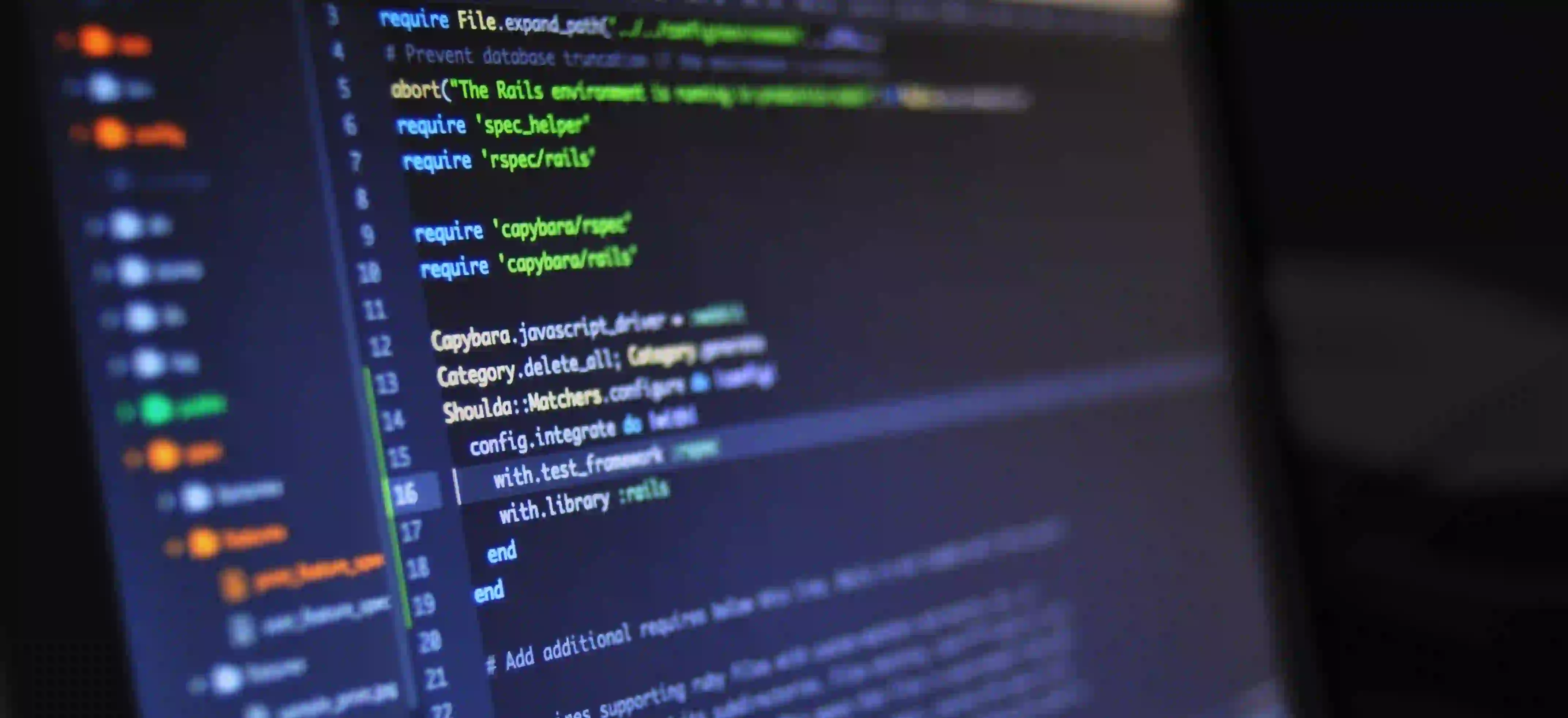
Mastering Android Copy-Paste: Intent Failures Explained
Android's Copy-Paste functionality is one of the most widely used features that enhances user experience across applications. While it seems straightforward, several subtle nuances can lead to unexpected behaviors, particularly related to Intents. In this post, we'll dive into how copying and pasting works in Android, examine potential failures with Intents, and offer solutions to help you master this essential functionality.
Understanding the Copy-Paste Mechanism in Android
The copy-paste feature in Android relies heavily on the ClipData and ClipboardManager classes. When you copy something, it doesn't just disappear—it gets placed on the system clipboard, making it accessible to any app that supports the paste functionality.
Here's a quick look at the key components:
- ClipboardManager: This is the class that manages the clipboard. You can use it to access and modify the contents of the clipboard.
- ClipData: This class is used to create the data you want to copy. It can hold multiple items in various formats.
Here’s a simple code snippet demonstrating how to copy text to the clipboard:
ClipboardManager clipboard = (ClipboardManager) getSystemService(Context.CLIPBOARD_SERVICE);
ClipData clip = ClipData.newPlainText("label", "Text to copy");
clipboard.setPrimaryClip(clip);
Code Explanation
- ClipboardManager: This service allows you to interact with the clipboard.
- ClipData.newPlainText(): This method allows you to create a simple text clip. The first argument is a label that describes the clip content, and the second argument is the actual data (text) you want to copy.
This code snippet will enable users to copy "Text to copy" to the clipboard, which can then be pasted anywhere.
Why Intent Failures Happen with Copy-Paste
Despite the relatively simple mechanism of the clipboard, problems can arise, especially when using Intents to copy or share data between applications. These failures can stem from multiple factors:
- Data Format Mismatch: The data may not be in a compatible format, leading to failures during the paste operation.
- Permissions Issues: Some applications may not have the proper permissions to read from the clipboard.
- Improperly Handled Intents: If an Intent is not handled properly, the target application may fail to retrieve data.
- Context and State Management: Certain application states may not allow clipboard access.
Handling Intent Failures
To mitigate these potential issues, you should adhere to best practices in handling Intents and clipboard data. Here’s how:
1. Ensuring Data Formats Align
When sharing data via Intents, it’s crucial to ensure that the receiving application can handle the format you are sending. Most applications expect data in specific formats, such as plain text, images, or URIs. Here’s an example of sharing plain text:
Intent sendIntent = new Intent(Intent.ACTION_SEND);
sendIntent.setType("text/plain");
sendIntent.putExtra(Intent.EXTRA_TEXT, "Text to share");
startActivity(Intent.createChooser(sendIntent, null));
Code Explanation
- Intent.ACTION_SEND: This action is used when you want to send something to another app.
- setType("text/plain"): This specifies the MIME type of the data you're sharing.
By ensuring the correct MIME type and data format, you can avoid mismatches and facilitate smoother communication between applications.
2. Requesting Necessary Permissions
Make sure your application has the correct permissions to access clipboard data. Typically, clipboard access does not require any special permissions, but if you're accessing it within another app or working with secure data, permissions may come into play.
3. Implementing Robust Error Handling
Always anticipate that Intents might fail. Implementing robust error handling can provide a better user experience. This approach not only helps in managing failures gracefully but also aids in debugging.
Here's an example of basic error handling for an Intent:
try {
startActivity(Intent.createChooser(sendIntent, null));
} catch (ActivityNotFoundException e) {
Toast.makeText(this, "No application available to share text", Toast.LENGTH_SHORT).show();
}
Code Explanation
- try-catch: Surround your Intent operation with a try-catch block to catch exceptions that may arise when no suitable activity can handle the Intent.
4. Context Management
In Android, the context in which an operation is being executed is crucial. Ensure you're using an appropriate context (like an Activity or Service context) when performing clipboard operations and sharing data.
Resolving Intent Failures
If you're facing persistent Intent failures, consider the following steps:
- Testing with Multiple Apps: Ensure that the data you're trying to copy and paste is compatible with various applications you aim to interact with.
- Debugging Logs: Use logging to monitor what data is being copied to ensure it’s what you expect.
- Review Android Documentation: The official Android documentation can help clarify specifications and requirements for Intents and copyrights. You can check these related documents:
Best Practices for Copy-Paste Functionality in Android
-
User Feedback: Always provide feedback upon copying or pasting. Use Toast messages or Snackbar to inform users about successful actions.
-
Accessibility: Consider how visually impaired users interact with your app. Ensure that copy-paste actions are easily accessible and executable via screen readers.
-
Limit Clipboard Contents: For security reasons, consider limiting the types of data placed on the clipboard to avoid potential misuse.
-
Regular Testing: Since Android devices run various OS versions and custom ROMs, regularly test your app across different devices to identify any unique issues.
Final Thoughts
Mastering the copy-paste functionality in Android can greatly enhance your application’s usability. By understanding how to leverage Intents, properly managing data formats, adhering to permissions, and implementing effective error handling, you can create a seamless experience for your users.
Navigating through Intent failures may seem daunting at first, but with proper guidance and best practices, you can set your application up for success. Always remember to test thoroughly and keep up-to-date with Android's evolving architecture.
For further insights on Android development, check out Android Developers Documentation for comprehensive guides and more advanced techniques.
Happy coding!