Cloning Challenges: Mastering Serializable vs. Non-Serializable
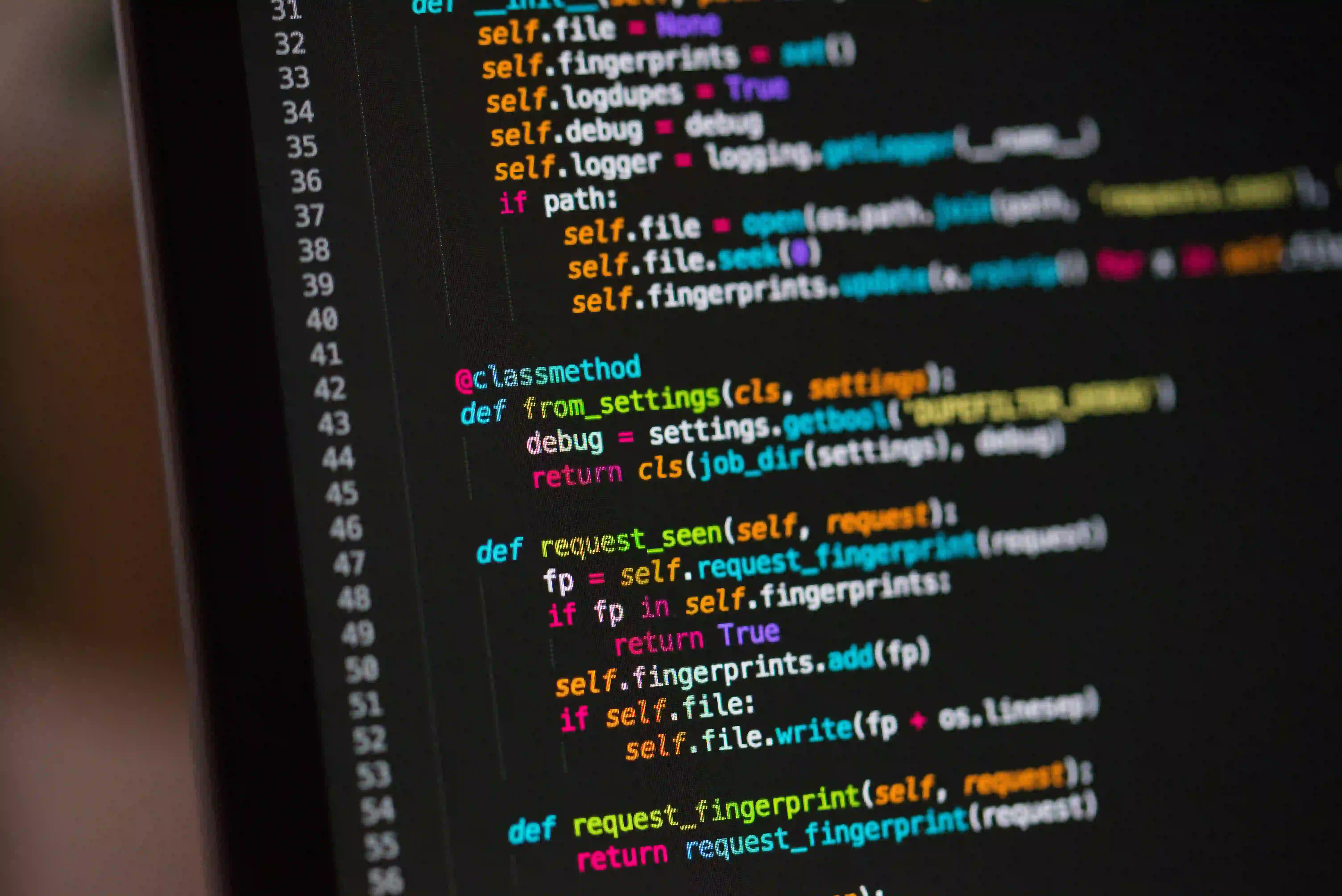
Cloning Challenges: Mastering Serializable vs. Non-Serializable in Java
Cloning in Java can be a bit like walking on a tightrope. One misstep, and you can find yourself in a world of confusion, especially when differentiating between Serializable and Non-Serializable classes. Understanding these concepts is crucial, not just for cloning, but for creating robust applications. This blog post dives deep into the challenges faced during cloning, focusing on the significance of serialization and deserialization.
What is Cloning?
In simple terms, cloning is the process of creating an identical copy of an object. Java provides two mechanisms for cloning objects:
- Shallow Copy: A copy of the original object is created with reference type fields pointing to the same objects.
- Deep Copy: Copies of all fields are created recursively.
The Cloneable Interface
To allow cloning, a class must implement the Cloneable
interface. By default, the clone()
method in the Object
class performs a shallow copy. However, deep copying needs manual implementation.
To create a class that is cloneable, consider the following implementation:
class Person implements Cloneable {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
@Override
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
Why this code? Implementing the Cloneable
interface is crucial to inform the Java runtime that this class can be cloned. The clone()
method overrides the default functionality to create a copy of the object.
Understanding Serialization
Serialization is the process of converting an object into a byte stream, enabling it to be easily saved or transferred. In Java, for a class to be serializable, it must implement the Serializable
interface.
Example of a Serializable Class
import java.io.Serializable;
class Address implements Serializable {
private String street;
private String city;
public Address(String street, String city) {
this.street = street;
this.city = city;
}
// getters and setters
}
Why this code? The Address
class implements Serializable
, indicating that its objects can be serialized. This is the basic requirement for leveraging serialization in Java.
Cloning with Serialization
Cloning can be elegantly achieved using serialization and deserialization techniques. By serializing an object, you effectively create a deep copy without needing to manually duplicate each field.
import java.io.*;
public class Serializer {
public static <T> T clone(T object) {
try {
// Serialize the object to a byte array
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
ObjectOutputStream out = new ObjectOutputStream(byteArrayOutputStream);
out.writeObject(object);
out.flush();
out.close();
// Now deserialize the byte array back to an object
ObjectInputStream in = new ObjectInputStream(new ByteArrayInputStream(byteArrayOutputStream.toByteArray()));
return (T) in.readObject();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
return null;
}
}
}
Why this code? This generic method clone
leverages serialization to create a deep copy of any serializable object by first turning it into a byte array and then back into an object. It's versatile and reusable!
Non-Serializable Classes and Cloning Issues
Not every class in Java is serializable. If you try to serialize a non-serializable class, a NotSerializableException
will be thrown. Consider what happens if you attempt to clone a non-serializable class:
Example of a Non-Serializable Class
class Company {
private String name;
private Address headquarters;
public Company(String name, Address headquarters) {
this.name = name;
this.headquarters = headquarters;
}
// getters
}
If you try to clone a Company
instance that contains a non-serializable field, you'll encounter issues during the serialization process.
The Solution: Marking as Transient
To handle these problems, you can mark non-serializable fields as transient
. This keyword ensures that a field is ignored during serialization.
class Company implements Serializable {
private String name;
private transient Address headquarters;
public Company(String name, Address headquarters) {
this.name = name;
this.headquarters = headquarters;
}
// Implement getters, setters, and clone method
}
Why this code? By using the transient
keyword, any attempt to serialize the headquarters
field will be ignored. This allows serialization to proceed without the risk of a NotSerializableException
.
The Challenges of Cloning
When dealing with cloning in Java, several challenges surface. Here are some you may encounter:
- Shallow vs. Deep Copy: Understand when to use each copy type based on your application's needs.
- Handling Non-Serializable Fields: Utilizing
transient
makes sense when certain fields should be ignored, but remember this may cause loss of data. - Performance Concerns: Serialization can be slower compared to direct cloning. A careful analysis is required to consider trade-offs.
Best Practices for Cloning
To successfully implement cloning in your applications:
- Choose Between Shallow and Deep Copy: Assess your needs carefully.
- Favor Serialization when Possible: Using a serialization approach can simplify deep copying.
- Implement Cloneable: Always implement the
Cloneable
interface if you plan to leverage cloning. - Error Handling: Always ensure proper exception handling around cloning operations.
- Documentation: Maintain clear documentation on which classes are cloneable and how they should be used.
To Wrap Things Up
Cloning in Java presents unique challenges, particularly when it comes to Serializable
and Non-Serializable
classes. But, by understanding the fundamentals and applying best practices, you can navigate these challenges with ease.
This knowledge not only enhances your programming skills but also equips you to build robust applications. So remember—whether deep or shallow—mind how you clone!
For additional insight on Java Cloning, check out the following resources:
Feel free to implement the code snippets provided and leverage the discussed strategies in your projects. Happy Coding!