Struggling with MongoDB Performance in Web Apps?
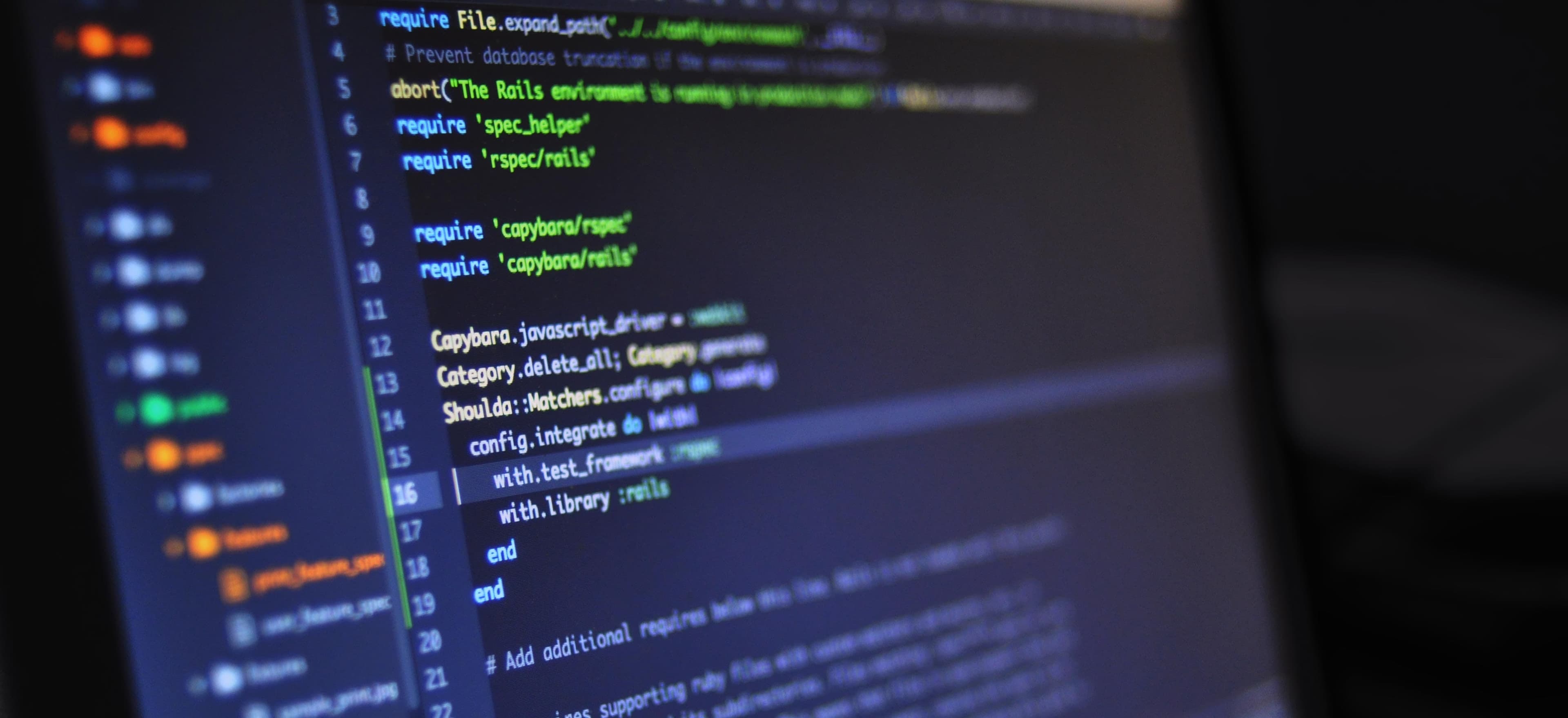
- Published on
Struggling with MongoDB Performance in Web Apps?
As the popularity of NoSQL databases has soared, MongoDB has emerged as a go-to solution for many web applications. Its flexibility, scalability, and ease of use have made it a favorite among developers. However, as applications grow, issues relating to performance can arise. If you’re experiencing slow queries or lag in data retrieval in your web apps, you’re in the right place.
In this blog post, we’ll explore common performance challenges in MongoDB, best practices to enhance performance, and how you can implement these strategies in your web applications.
Understanding MongoDB Performance Issues
Before diving into the solutions, it’s essential to understand why performance issues occur in MongoDB. Common pitfalls include:
- Suboptimal Indexing: Without proper indexing, MongoDB will have to scan every document in a collection, leading to slow queries.
- Inadequate Hardware Resources: Performance issues often arise from insufficient RAM or CPU resources.
- Large Document Sizes: Storing excessively large documents can affect performance, especially when updating and querying.
- Poorly Designed Schema: Using a suboptimal schema can lead to inefficient data retrieval.
Best Practices for Improving MongoDB Performance
1. Proper Indexing
Indexes are critical for query performance. They help MongoDB locate data quickly rather than scanning every document. Here’s how to leverage indexing effectively:
Create Compound Indexes
When queries filter by multiple fields, consider creating compound indexes. For example:
// Java Code to create a compound index on "username" and "age"
MongoCollection<Document> collection = database.getCollection("users");
IndexOptions indexOptions = new IndexOptions().background(true);
collection.createIndex(Indexes.compoundIndex(Indexes.asc("username"), Indexes.desc("age")), indexOptions);
This code snippet creates an index on the username
field in ascending order and the age
in descending order. Compound indexes can significantly speed up queries that filter or sort by these properties.
Analyze Index Usage
Use the explain()
method to evaluate how your queries utilize indexes:
Document query = new Document("username", "john_doe");
Document explain = collection.find(query).explain();
System.out.println("Query Plan: " + explain.toJson());
This will provide insights into query plans to help determine if the right indexes are being used.
2. Optimize Query Structure
Designing efficient queries is just as crucial as indexing. Here are some tips to consider:
- Limit Returned Fields: Use projection to return only the fields you need. It reduces data transfer size.
Document query = new Document("age", new Document("$gt", 25));
FindIterable<Document> results = collection.find(query).projection(Projections.fields(Projections.include("username", "email")));
This query only retrieves the username
and email
fields for users over the age of 25.
- Avoid
$where
Queries: These can be slow as they use JavaScript execution. Instead, try to leverage built-in operators.
3. Data Modelling
MongoDB is schema-less but that doesn't mean you can ignore schema design best practices.
Embedding vs. Referencing
Deciding whether to embed documents or reference them can impact performance.
-
Embedding: Use when the data is often retrieved together. For example, storing user profiles with their addresses embedded is efficient.
-
Referencing: Use when documents are large or frequently updated independently, like orders linked to users.
4. Use Caching
Sometimes, the quickest way to improve performance is to cache frequent queries or results. Consider using a Redis cache for this purpose. It reduces the load on your database by keeping frequently accessed data in memory.
5. Sharding
If your application handles large datasets, MongoDB's sharding feature can help distribute the load across multiple servers. Each shard is a separate database that contains a subset of your data. It allows horizontal scaling, thus improving performance.
Implementing Sharding
You can enable sharding with the following commands:
// Enable sharding for your database
use admin
sh.enableSharding("myDatabase")
// Shard a collection
sh.shardCollection("myDatabase.users", {"username": 1});
Ensure you choose a shard key that allows evenly distributed load across shards, as a poor shard key can lead to performance degradation.
6. Monitor Performance
Monitoring is vital to maintain a healthy database. Use MongoDB's built-in tools or APM solutions to track performance metrics.
Useful metrics to monitor include:
- Operation times: Monitor how long queries and writes take.
- Disk I/O: Identify bottlenecks based on read and write operations.
- Connection Counts: Manage active connections to prevent overload.
Bringing It All Together
Improving MongoDB performance in web apps requires a multi-faceted approach. By applying effective indexing strategies, optimizing query structures, considering the document design carefully, utilizing caching solutions, and monitoring performance metrics, you can significantly enhance your application's response times and efficiency.
For further reading, check out MongoDB Documentation on Indexing and Sharding Overview to gain deeper insights.
Implement these practices in your web applications, and watch as your MongoDB performance transforms from sluggish to spectacular. Happy coding!