Mastering the Single Responsibility Principle in Software Design
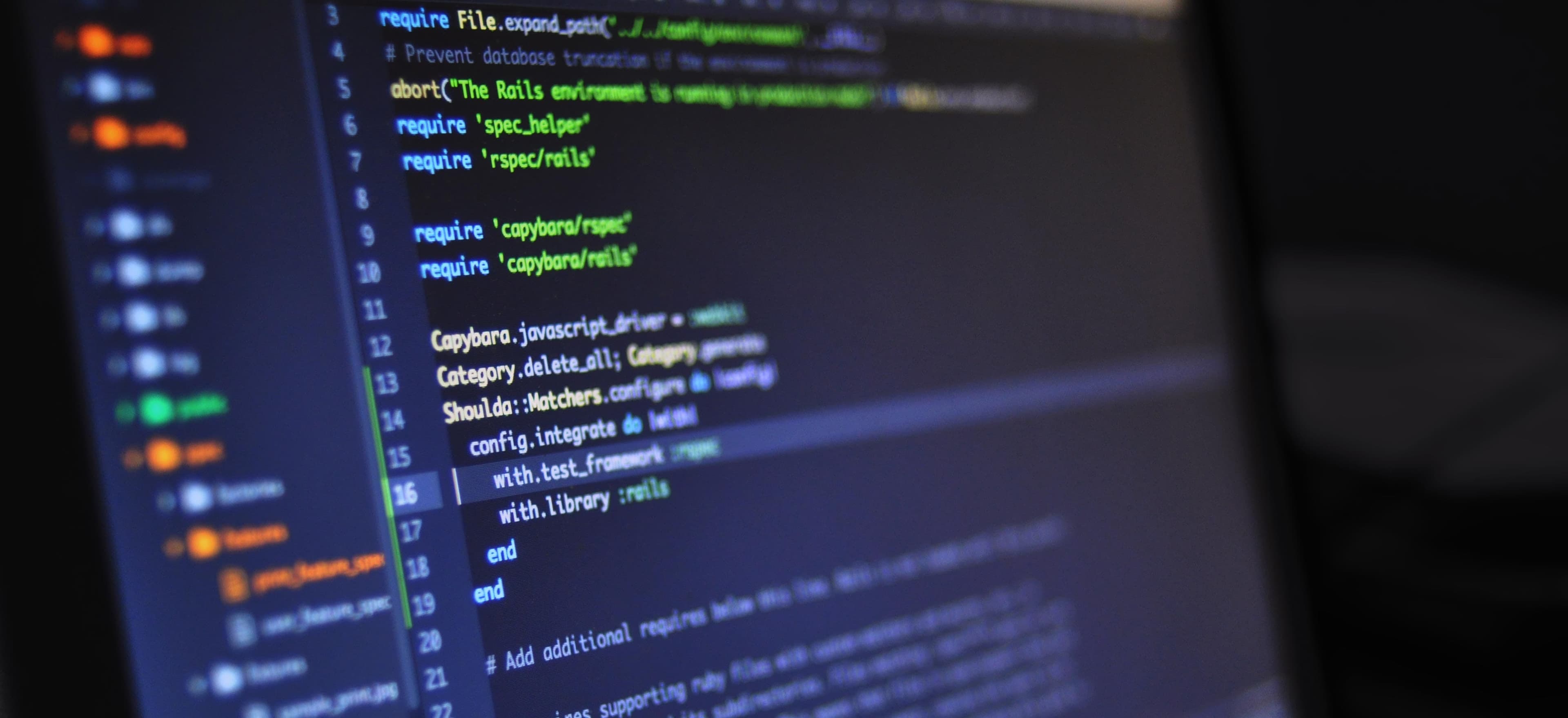
- Published on
Mastering the Single Responsibility Principle in Software Design
When writing software, maintaining clean, maintainable code should be a primary objective. One essential concept in achieving this is the Single Responsibility Principle (SRP), one of the five SOLID principles that guide object-oriented design. In this blog post, we will explore the SRP in detail, understand its importance, and see how to apply it in your Java projects.
What is the Single Responsibility Principle?
The Single Responsibility Principle states that a class should have only one reason to change, meaning it should only have one reason to exist. In simpler terms, every class should focus on a single task or functionality. This principle enhances code readability, maintainability, and testability.
Let’s contemplate an example. Imagine a class that handles both user input and database operations. If you need to change the database structure, you might inadvertently also modify how user input is processed. This can lead to bugs and complications that are hard to untangle.
Why is SRP Important?
- Enhanced Maintainability: Changes in one responsibility won’t affect others. Class modifications become predictable and controlled.
- Improved Testability: Each class can be tested in isolation. The chances of side effects are diminished as responsibilities are clear-cut.
- Better Collaboration: In teams, different developers can work on discrete classes simultaneously, reducing merge conflicts.
- Increased Reusability: Smaller classes or components that focus on a single task can be reused in different contexts or applications.
How to Implement SRP in Java
To illustrate SRP in action, let's consider a basic application that manages a library's operations. We’ll start with a poor design that violates the SRP.
Example of SRP Violation
public class Library {
public void addBook(String title) {
// Logic to add book
System.out.println("Book added: " + title);
}
public void sendEmail(String email) {
// Logic to send email
System.out.println("Email sent to: " + email);
}
public void printBookDetails(String title) {
// Logic to print book details
System.out.println("Book details of: " + title);
}
}
In this example, the Library
class is responsible for adding books, sending emails, and printing details. This is a violation of the SRP because changes to email functionality or how books are printed can affect the core library operations.
Refactoring to Follow SRP
Let us refactor this code to adhere to the SRP. We will create distinct classes for managing each responsibility.
public class BookManager {
public void addBook(String title) {
// Logic to add book
System.out.println("Book added: " + title);
}
}
public class EmailNotification {
public void sendEmail(String email) {
// Logic to send email
System.out.println("Email sent to: " + email);
}
}
public class BookPrinter {
public void printBookDetails(String title) {
// Logic to print book details
System.out.println("Book details of: " + title);
}
}
In this refactored design, we have three classes, each handling one specific responsibility. The separation enables each class to evolve independently. For instance, should we want to change how book details are printed, we only need to modify the BookPrinter
class without affecting the other functionalities.
Benefits of the SRP Approach
- Clear Class Responsibilities: Each class communicates its intent clearly.
- Isolated Changes: Modifications are limited to specific classes, minimizing the risk of errors.
- Ease of Testing: Each class can be thoroughly tested without invoking unrelated components.
Real-World Application of SRP
In modern software development, adhering to the SRP can be particularly beneficial in large-scale applications. Consider frameworks like Spring, where components often become intricate. Utilizing SRP ensures that your business logic, data access, and notification features are decoupled.
Here’s a practical case:
Suppose you're developing an e-commerce platform. You have several functionalities such as user authentication, payment processing, and order management. All of these functionalities can be organized by applying SRP.
Example Code Implementation
public class UserAuthentication {
public void login(String username, String password) {
// Logic for logging in a user
System.out.println("User logged in: " + username);
}
}
public class PaymentProcessor {
public void processPayment(String paymentDetails) {
// Logic for processing payment
System.out.println("Payment processed: " + paymentDetails);
}
}
public class OrderManager {
public void placeOrder(String orderDetails) {
// Logic for placing an order
System.out.println("Order placed: " + orderDetails);
}
}
Each class here encapsulates a single responsibility concerning user actions. This structure allows for easy modifications and scaling as the project evolves.
Recognizing When SRP is Being Violated
Sometimes, identifying a violation of the Single Responsibility Principle isn't straightforward. Here are some red flags to watch for:
- Large Classes: If a class contains 200+ lines of code, it’s likely handling too many responsibilities.
- Tight Coupling: When one class relies heavily on another for functionality, it may signify non-compliance with SRP.
- Frequent Changes: If a class requires constant changes for several reasons, it’s a clear sign that it is doing more than it should.
Best Practices for Maintaining SRP
- Keep Classes Small: If a class feels too large or complex, it probably indicates that it has multiple responsibilities.
- Group Related Behaviors: Ensure that methods in a class are related to the single responsibility of that class.
- Use Interfaces: Leverage interfaces to separate functionalities, ensuring that implementing classes only focus on what they must handle.
- Review Regularly: Schedule refactoring sessions to review classes. Make it a habit to ask which responsibilities a class has.
The Closing Argument
Mastering the Single Responsibility Principle is invaluable for any software developer. It aids in creating clean, maintainable, and testable code. By refactoring our code into focused, responsibility-driven classes, we not only streamline our development process but also set ourselves up for success in the future.
For more insights into object-oriented design principles, check out SOLID Principles Explained. Engage with your code, observe patterns, and don't hesitate to refactor. Only then can you achieve clean and efficient designs.
If you wish to explore more fundamental design principles, consider reading about Design Patterns and their applications in software development. Happy coding!