Effortlessly Generating Infinite Primes in Java
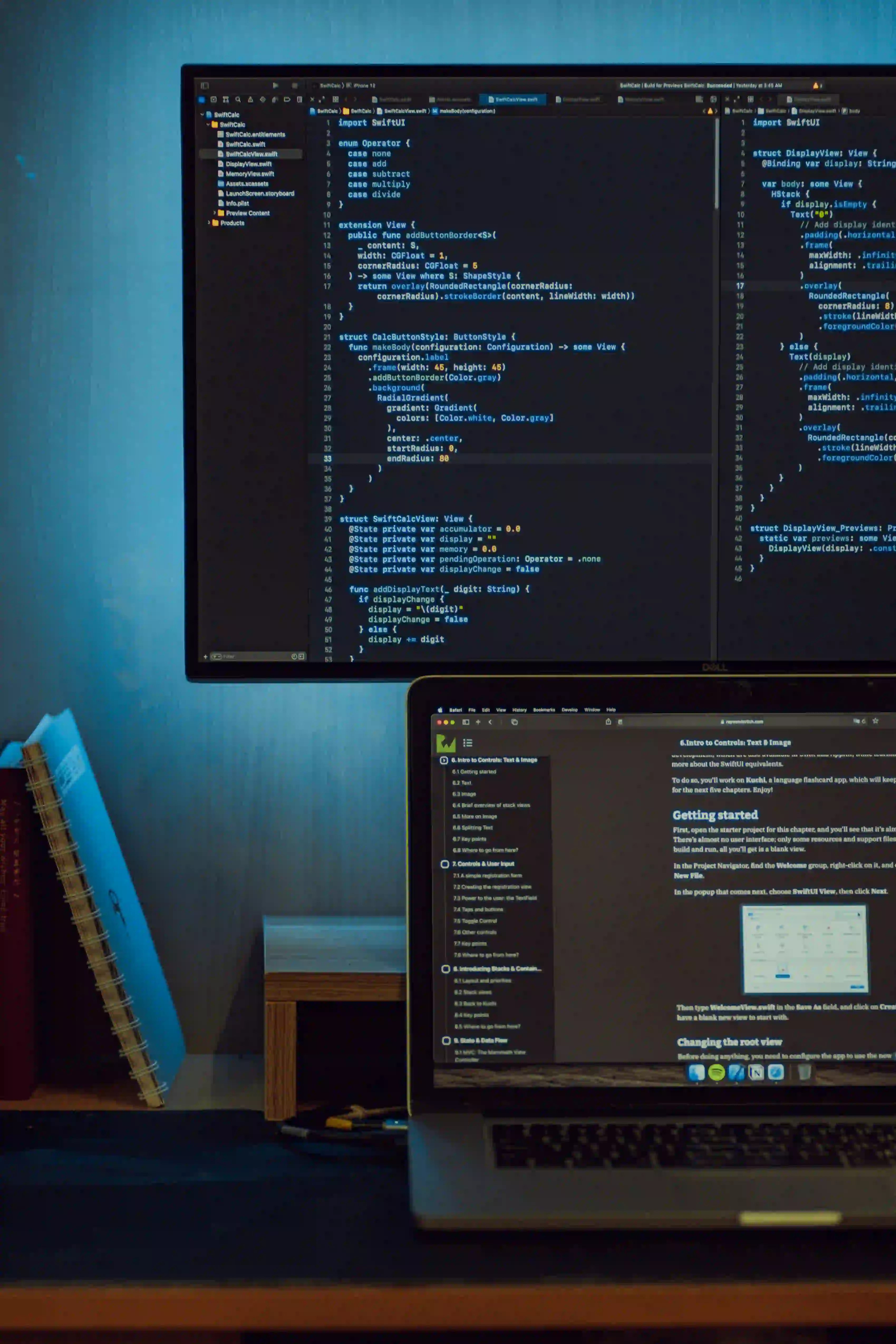
Effortlessly Generating Infinite Primes in Java
Generating prime numbers is a fundamental task in mathematics and computer science. In this blog post, we will explore how to generate an infinite number of prime numbers using Java. This not only showcases the power of programming but also demonstrates the beauty of prime numbers.
Prime numbers are defined as natural numbers greater than 1 that have no positive divisors other than 1 and itself. Examples include 2, 3, 5, 7, 11, and 13. The beauty of primes lies in their deceptively simple definition while their distribution is quite complex.
Before We Begin to Prime Numbers
The list of prime numbers goes on infinitely, as proven by the ancient Greek mathematician Euclid around 300 BC. Despite their infinite nature, generating prime numbers efficiently is paramount, especially in cryptographic applications.
In this post, we will cover several methods to generate primes in Java, including:
- The Sieve of Eratosthenes
- A simple trial division method
- An infinite prime generator using Java Streams
Let's dive in!
The Sieve of Eratosthenes
The Sieve of Eratosthenes is a classic algorithm for finding all prime numbers up to a certain limit. Here’s the Java implementation:
import java.util.Arrays;
public class SieveOfEratosthenes {
public static void main(String[] args) {
int limit = 100;
boolean[] prime = new boolean[limit + 1];
Arrays.fill(prime, true);
prime[0] = prime[1] = false; // 0 and 1 are not prime numbers
// Sieve algorithm
for (int p = 2; p * p <= limit; p++) {
if (prime[p]) {
for (int i = p * p; i <= limit; i += p) {
prime[i] = false;
}
}
}
// Printing the prime numbers
System.out.println("Prime numbers up to " + limit + ":");
for (int p = 2; p <= limit; p++) {
if (prime[p]) {
System.out.print(p + " ");
}
}
}
}
Why Use the Sieve of Eratosthenes?
The Sieve of Eratosthenes is efficient for finding all primes up to a specific limit. The algorithm runs in O(n log log n) time complexity and uses O(n) space, making it considerably faster than checking each number for primality individually. This is due to the fact that it eliminates multiples of each prime starting from 2.
Trial Division Method
The simplest method to check if a number is prime is trial division. Here is a straightforward implementation:
public class PrimeChecker {
public static boolean isPrime(int n) {
if (n <= 1) return false; // 0 and 1 are not prime numbers
if (n <= 3) return true; // 2 and 3 are prime numbers
if (n % 2 == 0 || n % 3 == 0) return false; // eliminate multiples of 2 and 3
for (int i = 5; i * i <= n; i += 6) {
if (n % i == 0 || n % (i + 2) == 0) {
return false; // skip even numbers
}
}
return true;
}
public static void main(String[] args) {
int num = 29;
if (isPrime(num)) {
System.out.println(num + " is a prime number.");
} else {
System.out.println(num + " is not a prime number.");
}
}
}
Why Use Trial Division?
Trial division is simple and serves well for smaller numbers but becomes inefficient for larger ones. The time complexity is O(√n), making it not the best option for large prime generation tasks compared to the Sieve of Eratosthenes.
Infinite Prime Generator using Java Streams
One of the most elegant ways to generate primes in Java is by using Java Streams. This method allows us to generate an infinite sequence of prime numbers, demonstrating the versatility of streams in modern Java.
import java.util.stream.IntStream;
import java.util.stream.Stream;
public class InfinitePrimes {
public static void main(String[] args) {
Stream<Integer> primes = Stream.iterate(2, n -> n + 1)
.filter(InfinitePrimes::isPrime);
// Print the first 10 primes
primes.limit(10).forEach(System.out::print);
}
public static boolean isPrime(int n) {
// Same isPrime method as before
if (n <= 1) return false;
if (n <= 3) return true;
if (n % 2 == 0 || n % 3 == 0) return false;
for (int i = 5; i * i <= n; i += 6) {
if (n % i == 0 || n % (i + 2) == 0) {
return false;
}
}
return true;
}
}
Why Use Java Streams?
Java Streams allow for a more functional programming approach, enabling more concise and readable code. The use of iterate
generates an unbounded stream starting from 2, while the filter method keeps only prime numbers. This method is highly flexible and can be adapted to generate primes as needed.
My Closing Thoughts on the Matter
In this post, we explored various ways to generate prime numbers in Java. We started with the Sieve of Eratosthenes for efficient prime generation up to a limit, moved on to the straightforward trial division method, and finally discussed an elegant infinite prime generator using Java Streams.
Each method has its advantages and disadvantages. The Sieve of Eratosthenes is best for a predetermined range, the trial division method is simple but limiting for larger primes, while Java Streams provide a modern, elegant solution for generating an infinite series of primes.
If you're interested in deeper exploration, check out Prime Numbers in Cryptography and Understanding Time Complexity for further insights.
Happy coding, and may your journey in the world of prime numbers be both enjoyable and enlightening!