Mastering One-to-Many Relationships in REST APIs
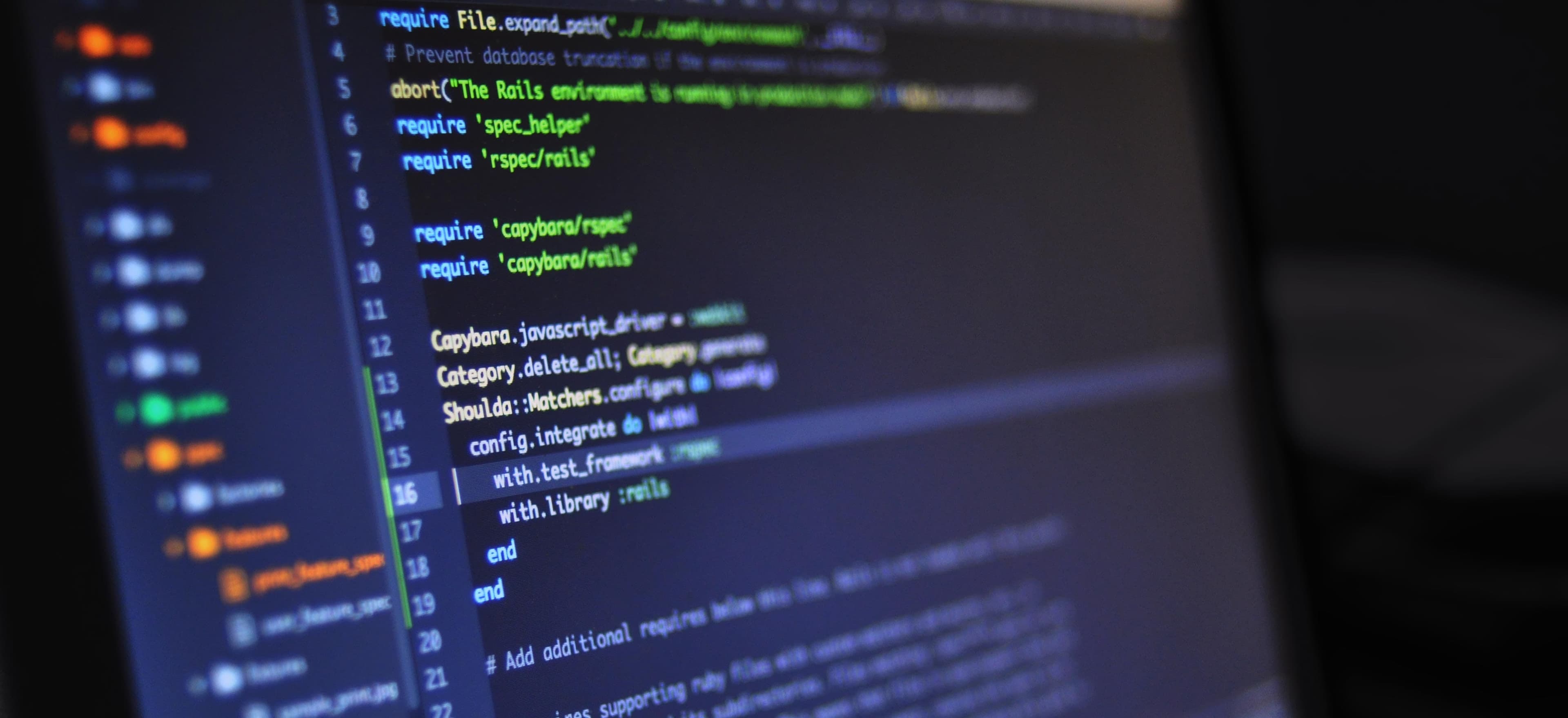
- Published on
Mastering One-to-Many Relationships in REST APIs
In today's digital world, APIs (Application Programming Interfaces) are the backbone of communication between different software components. Among the various design patterns in APIs, one-to-many relationships are particularly crucial, especially in relational databases. Understanding how to orchestrate these relationships effectively can significantly enhance the functionality and performance of your application. In this blog post, we will explore how to implement one-to-many relationships in REST APIs using Java, along with useful examples and best practices.
What Are One-to-Many Relationships?
Before delving into the implementation, let's clarify what a one-to-many relationship entails. This relationship indicates that a single record in one table can be associated with multiple records in another table. For instance, think of a blogging platform where a single author can write multiple blog posts. Here, each author represents one record in the authors table, while the posts correspond to multiple records in the posts table.
Key Concepts:
- Primary Key: A unique identifier for each record in a table.
- Foreign Key: A field in the child table (posts) that links back to the parent table (authors) using the parent’s primary key.
Building the Model in Java
To illustrate how we can represent this relationship in Java, let's create two classes: Author
and Post
.
Author Class
import javax.persistence.*;
import java.util.Set;
@Entity
@Table(name = "authors")
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private Set<Post> posts;
// Constructors, Getters, and Setters
public Author() {}
public Author(String name) {
this.name = name;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Set<Post> getPosts() {
return posts;
}
public void setPosts(Set<Post> posts) {
this.posts = posts;
}
}
Why We Use Annotations
- @Entity: Marks the class as a database entity.
- @Table: Specifies the table name in the database.
- @Id: Defines the primary key for the entity.
- @GeneratedValue: Automatically generates the primary key value.
- @OneToMany: Indicates a one-to-many relationship between
Author
andPost
. - CascadeType.ALL: Specifies that operations (like persist, merge, remove) will be applied to both Author and its associated Posts.
- FetchType.LAZY: Ensures that related posts are not loaded until accessed.
Post Class
import javax.persistence.*;
@Entity
@Table(name = "posts")
public class Post {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
private String content;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "author_id", nullable = false)
private Author author;
// Constructors, Getters, and Setters
public Post() {}
public Post(String title, String content, Author author) {
this.title = title;
this.content = content;
this.author = author;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public Author getAuthor() {
return author;
}
public void setAuthor(Author author) {
this.author = author;
}
}
Explanation of the Post Class
- @ManyToOne: Indicates that many posts can be linked to one author, a crucial part of understanding one-to-many relationships.
- @JoinColumn: Specifies the foreign key in the
posts
table that references theauthors
table.
Creating REST APIs
With our data model set up, it's time to expose this relationship through a REST API. We will create two endpoints: one for authors and another for their posts.
AuthorController Class
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/authors")
public class AuthorController {
@Autowired
private AuthorRepository authorRepository;
@GetMapping
public List<Author> getAllAuthors() {
return authorRepository.findAll();
}
@PostMapping
public Author createAuthor(@RequestBody Author author) {
return authorRepository.save(author);
}
@GetMapping("/{id}/posts")
public ResponseEntity<List<Post>> getPostsByAuthorId(@PathVariable(value = "id") Long authorId) {
Author author = authorRepository.findById(authorId)
.orElseThrow(() -> new ResourceNotFoundException("Author not found"));
return ResponseEntity.ok().body(new ArrayList<>(author.getPosts()));
}
}
Code Breakdown
- @RestController: This annotation is from Spring and signifies that the class will handle HTTP requests.
- @RequestMapping: Defines the base URI for accessing the author endpoints.
- @GetMapping and @PostMapping: Used for handling GET and POST requests, respectively.
- ResponseEntity: A Spring class that helps build a response object, which can include headers, status codes, and body.
Development and Testing
When developing REST APIs, it's extremely useful to test endpoints thoroughly using tools like Postman or cURL. They allow you to rapidly prototype requests and verify that your endpoints return expected results.
Handling Exceptions
To ensure a robust API, we need to handle exceptions. Here’s an example of how to handle resource-not-found scenarios gracefully.
Custom Exception Handler
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<String> handleResourceNotFound(ResourceNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
}
My Closing Thoughts on the Matter
Mastering one-to-many relationships in REST APIs can be a cornerstone in building scalable and efficient web applications. The ability to represent these relationships correctly ensures that your system can handle data interactions without unnecessary complexity.
Key Takeaways
- Use JPA annotations to model one-to-many relationships effectively.
- Develop clear REST endpoints to expose your data structure.
- Utilize proper exception handling for a better user experience.
For more detailed tutorials on REST APIs and Java, consider checking resources like Spring Documentation and Java Persistence API (JPA) Guide.
By understanding and implementing these concepts, you'll be well on your way to creating APIs that can efficiently manage complex data relationships. Happy coding!