Struggling to Write Clean Tests? Here’s How to Simplify!
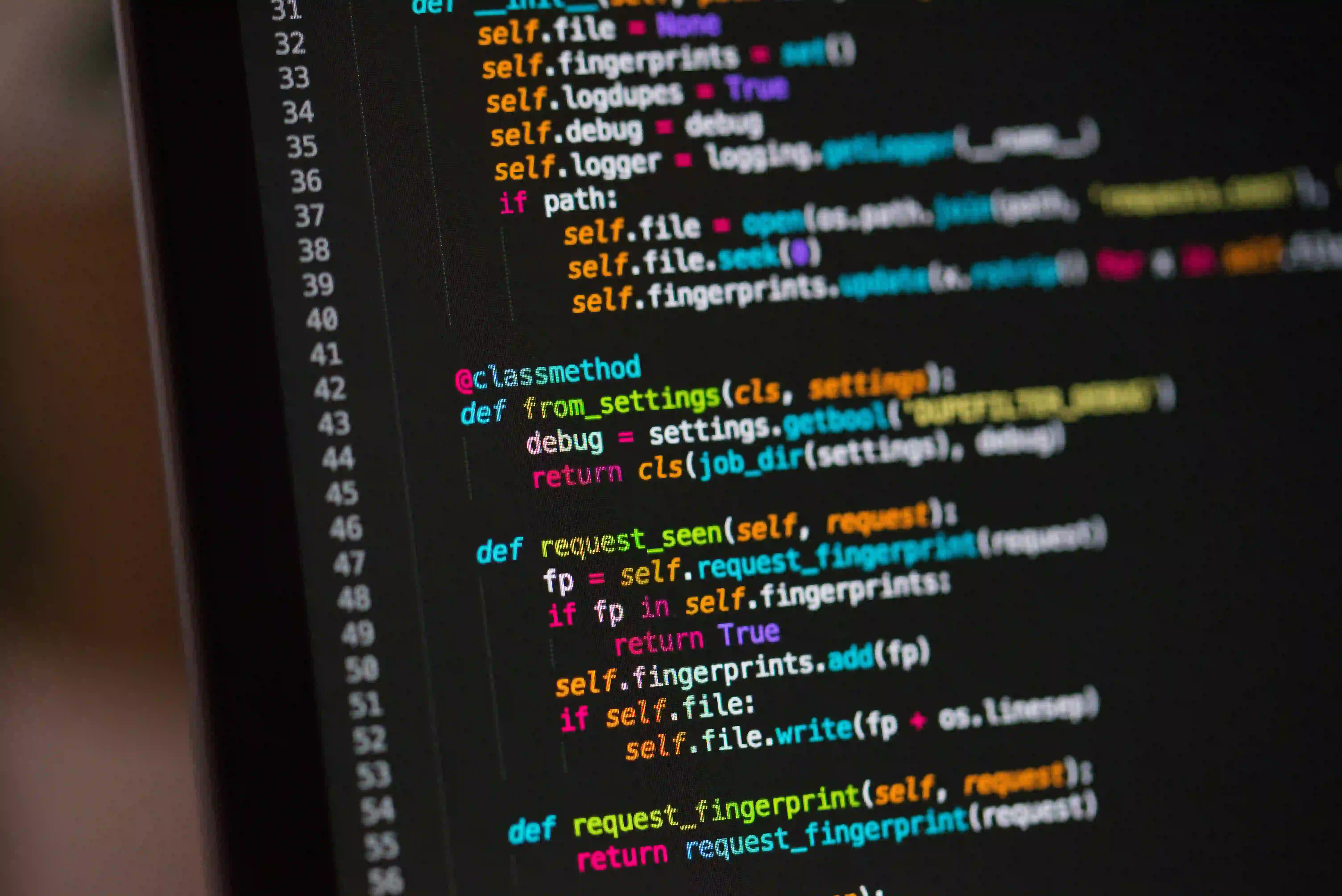
Struggling to Write Clean Tests? Here’s How to Simplify!
Testing is an essential part of software development. Yet, many developers find it challenging to write clean tests. It often feels like you’re stuck in a conductor’s role in a chaotic orchestra, with coverage and maintainability playing dissonant notes. Fortunately, simplifying your testing strategy can harmonize your efforts. In this post, we will discuss proven strategies to help you write clean tests in Java, enhancing readability and effectiveness.
Why Clean Tests Matter
Before diving into strategies, let's clarify why clean tests are crucial:
- Maintainability: Clear tests are easier to update when requirements change.
- Readability: They allow developers to understand the intent quickly.
- Debugging: Clean tests pinpoint issues faster, reducing development time.
- Collaboration: Team members can contribute and understand tests without extensive onboarding.
Principles of Writing Clean Tests
Let's explore some key principles that help you simplify your Java tests.
1. Follow the AAA Pattern
The Arrange, Act, Assert (AAA) pattern is a well-known structure for writing clean tests. It divides tests into three distinct steps.
Example Code
@Test
public void testAdd() {
// Arrange
Calculator calculator = new Calculator();
int a = 5;
int b = 3;
// Act
int result = calculator.add(a, b);
// Assert
assertEquals(8, result);
}
Commentary
In this example, we first arranged the necessary variables. We then invoked the add
method and finally asserted the expected outcome. Following this pattern not only clarifies purpose but also makes test code consistent.
2. Keep Tests Isolated
Tests should be independent. This means changes in one area of code or test should not affect others. This principle promotes robust testing, showcasing weaknesses clearly.
Example Code
@Test
public void testSubtract() {
Calculator calculator = new Calculator();
int result = calculator.subtract(10, 5);
assertEquals(5, result); // Isolated test
}
@Test
public void testMultiply() {
Calculator calculator = new Calculator();
int result = calculator.multiply(4, 5);
assertEquals(20, result); // Another isolated test
}
Commentary
In this code snippet, we have separate tests for subtraction and multiplication. Each test stands alone, ensuring that a failure in multiplication doesn’t obscure results from subtraction.
3. Use Descriptive Names
Test method names should be descriptive to clearly state their intent. Good names provide insights into what’s being tested, reducing the need to dig into test bodies.
Example Code
@Test
public void shouldReturnPositiveWhenAddingPositiveNumbers() {
Calculator calculator = new Calculator();
assertEquals(10, calculator.add(4, 6));
}
@Test
public void shouldReturnZeroWhenAddingZeroToAnyNumber() {
Calculator calculator = new Calculator();
assertEquals(4, calculator.add(0, 4));
}
Commentary
The names of these tests clearly communicate what is expected. Such clarity allows team members to quickly understand the purpose of the tests, fostering better collaboration.
4. Leverage Helper Methods and Test Fixtures
When multiple tests share setup logic, using helper methods or test fixtures reduces duplication and enhances maintainability.
Example Code
public class CalculatorTest {
private Calculator calculator;
@Before
public void setUp() {
calculator = new Calculator();
}
@Test
public void testAdd() {
assertEquals(7, calculator.add(3, 4));
}
@Test
public void testDivide() {
assertEquals(2, calculator.divide(10, 5));
}
}
Commentary
By instantiating Calculator
in a single setUp
method, we eliminate code repetition across tests. This pattern makes the code DRY (Don't Repeat Yourself) and easier to manage.
5. Use Parameterized Tests
Parameterized tests help run the same test logic multiple times with different inputs. This is particularly useful when dealing with a variety of inputs or edge cases.
Example Code
@RunWith(Parameterized.class)
public class ParameterizedCalculatorTest {
@Parameterized.Parameter(0)
public int input1;
@Parameterized.Parameter(1)
public int input2;
@Parameterized.Parameter(2)
public int expectedResult;
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 1, 2, 3 },
{ 2, 3, 5 },
{ -1, 1, 0 }
});
}
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(expectedResult, calculator.add(input1, input2));
}
}
Commentary
With parameterized tests, you define multiple input sets, executing the same testing logic. This reduces redundancy and simplifies your test suite.
6. Keep Tests Focused
Each test should ideally validate a single concept or behavior. When tests are focused, they're easier to understand and maintain.
Example Code
@Test
public void testCalculatingAreaOfCircle() {
Circle circle = new Circle(5);
double expectedArea = Math.PI * Math.pow(5, 2);
assertEquals(expectedArea, circle.calculateArea(), 0.01);
}
@Test
public void testCalculatingPerimeterOfCircle() {
Circle circle = new Circle(5);
double expectedPerimeter = 2 * Math.PI * 5;
assertEquals(expectedPerimeter, circle.calculatePerimeter(), 0.01);
}
Commentary
In this example, the tests evaluate different aspects of the Circle
class. Each test remains focused on a specific behavior, making it clear what is being tested.
7. Review Tests Regularly
In dynamic environments, code changes often demand updates in tests. Regular reviews ensure your tests remain relevant and effective. Automated tools, such as SonarQube, can assist in evaluating test coverage and quality.
The Bottom Line
Writing clean tests in Java may seem challenging at first, but by applying these principles and practices, you'll create a robust testing framework that enhances both development speed and code quality.
- Follow the AAA pattern.
- Keep tests isolated and focused.
- Use descriptive naming.
- Leverage helper methods and parameterized tests.
- Regularly review and maintain your tests.
Embrace these strategies to simplify your testing process. Remember, clean tests not only save you time but also foster a collaborative and efficient development environment.
By making code testing easier, you’ll find your software development experience becoming significantly more productive. Happy testing!