Choosing the Right Primary Key: Common Pitfalls to Avoid
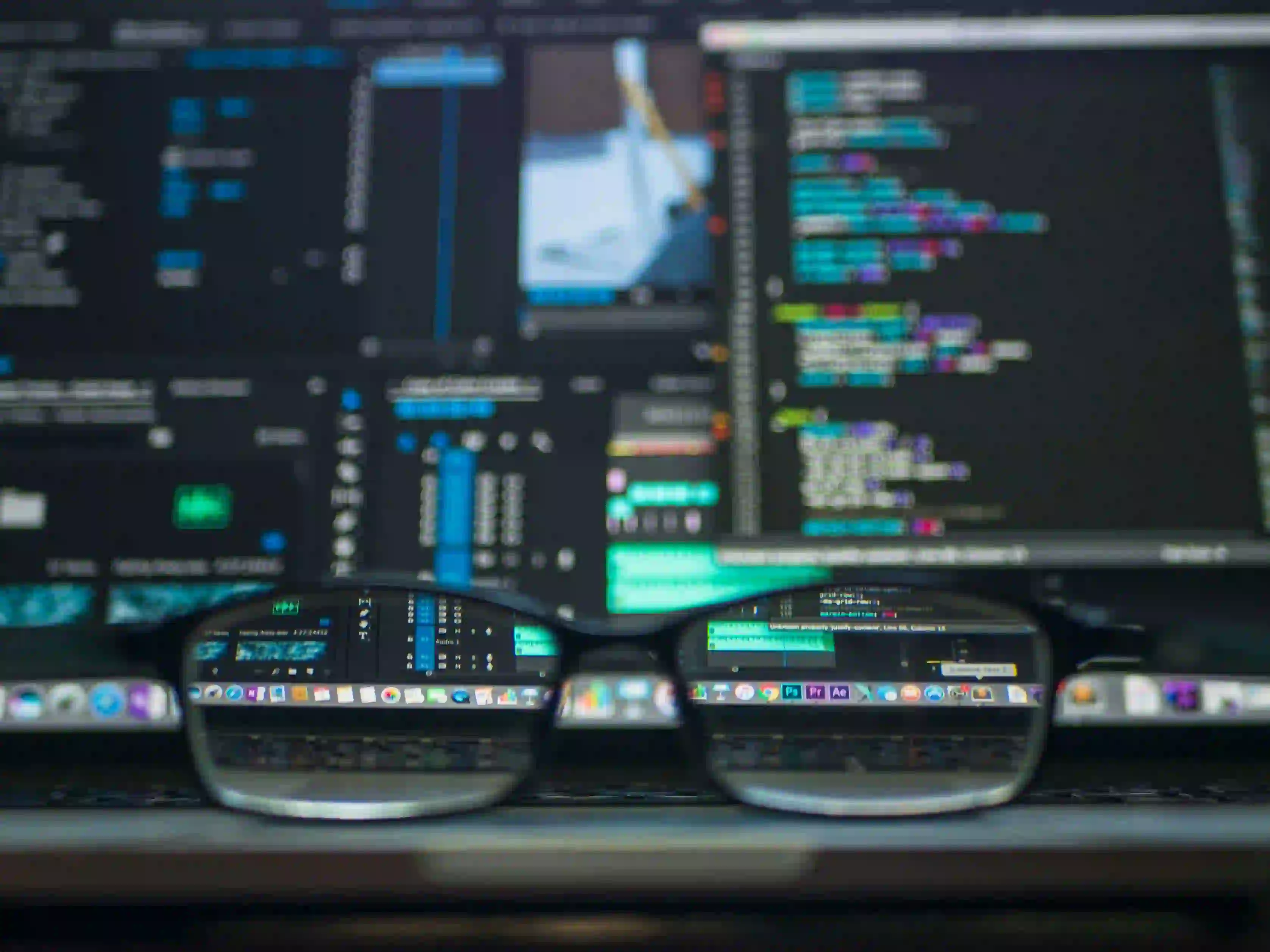
Choosing the Right Primary Key: Common Pitfalls to Avoid
In the realm of database design, the primary key serves as a crucial element that uniquely identifies each record in a table. A well-chosen primary key not only improves data integrity but also plays a vital role in query performance and relational database structure. However, the decision-making process regarding the right primary key can pose several challenges. In this blog post, we will explore common pitfalls to avoid when selecting a primary key and provide strategies for making the best choice.
Understanding the Role of Primary Keys
Before we delve into the common pitfalls, it's important to understand the fundamental purpose of a primary key. According to Wikipedia, a primary key must be unique across all rows in the table and cannot contain null values. It supports the integrity of the data, allowing for the identification and distinction of records.
Characteristics of a Good Primary Key
A good primary key should possess several characteristics:
- Uniqueness: Each value must be distinct.
- Non-nullability: It cannot accept null values.
- Immutability: Ideally, it should not change over time.
- Simplicity: It should be as short as possible.
By adhering to these characteristics, you can maximize the effectiveness of your primary key.
Common Pitfalls When Choosing a Primary Key
While choosing a primary key might seem straightforward, several common pitfalls can lead to poor database design. Let's explore these pitfalls in detail.
1. Using Natural Keys Instead of Surrogate Keys
Natural Keys are keys with a logical relationship to the data, like email addresses or Social Security Numbers, whereas Surrogate Keys are artificial keys, typically auto-incremented integers, that have no business meaning.
Pitfall Explanation
Natural keys can lead to problems when the business rules change. For instance, if a user changes their email address, using it as a primary key would require updating all related records.
Code Example
-- Example of a natural key
CREATE TABLE Users (
Email VARCHAR(255) PRIMARY KEY,
Name VARCHAR(100),
CreatedAt TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
In this example, using Email as a primary key can complicate updates. Instead, consider using a surrogate key approach.
Suggested Approach
-- Using a surrogate key
CREATE TABLE Users (
UserID INT AUTO_INCREMENT PRIMARY KEY,
Email VARCHAR(255) UNIQUE,
Name VARCHAR(100),
CreatedAt TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
This adjustment maintains uniqueness without sacrificing flexibility.
2. Choosing Composite Keys for Simple Entities
Composite Keys are formed by combining two or more columns. While they can be useful, they also add complexity.
Pitfall Explanation
Overusing composite keys can complicate SQL queries and foreign key references. This can lead to performance issues and make the schema more challenging to maintain.
Code Example
-- Example of a composite key
CREATE TABLE Orders (
ProductID INT,
UserID INT,
OrderDate TIMESTAMP,
PRIMARY KEY (ProductID, UserID)
);
While this example uses a composite key effectively, it can be problematic when referencing from other tables.
Suggested Approach
-- Using a surrogate key instead
CREATE TABLE Orders (
OrderID INT AUTO_INCREMENT PRIMARY KEY,
ProductID INT,
UserID INT,
OrderDate TIMESTAMP
);
Now, referencing orders becomes simpler and improves query performance.
3. Not Considering Future Growth
When designing a schema, it's vital to consider future scalability and potential changes to data volume.
Pitfall Explanation
Choosing a primary key type that may not support the expected growth can lead to significant redesign efforts later.
Code Example
-- Poor choice for a growing dataset
CREATE TABLE Products (
ProductCode VARCHAR(10) PRIMARY KEY, -- Limited to 10 characters
ProductName VARCHAR(255)
);
This design might seem efficient for a small inventory, but what happens if the number of products exceeds existing codes?
Suggested Approach
-- A more scalable option
CREATE TABLE Products (
ProductID INT AUTO_INCREMENT PRIMARY KEY,
ProductCode VARCHAR(255) UNIQUE,
ProductName VARCHAR(255)
);
Using a surrogate key allows for an expansive range of product codes without impacting the primary key system.
4. Ignoring Indexing Considerations
Indexing is essential for performance optimization. The choice of primary key can significantly affect how indexing works in a database.
Pitfall Explanation
Failure to consider how a primary key is indexed can lead to slow query performance and inefficient data retrieval.
Code Example
-- Inefficient for indexing
CREATE TABLE Customers (
PhoneNumber VARCHAR(15) PRIMARY KEY,
Address VARCHAR(255)
);
Using PhoneNumber as the primary key may slow down queries since string comparisons are generally slower than integer comparisons.
Suggested Approach
-- More efficient for indexing
CREATE TABLE Customers (
CustomerID INT AUTO_INCREMENT PRIMARY KEY,
PhoneNumber VARCHAR(15) UNIQUE,
Address VARCHAR(255)
);
Using an integer as the primary key allows the database to utilize indexing more effectively, speeding up query performance.
5. Overlooking Referential Integrity
Referential integrity ensures that relationships between tables remain consistent. When selecting a primary key, consider how it will be referenced in foreign keys.
Pitfall Explanation
Neglecting referential integrity can lead to orphaned records and data inconsistencies.
Code Example
-- Potential issue with referential integrity
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
UserEmail VARCHAR(255), -- Not enforcing FK constraint
);
Without a foreign key relationship, you can have orders associated with non-existent users.
Suggested Approach
CREATE TABLE Users (
UserID INT AUTO_INCREMENT PRIMARY KEY,
Email VARCHAR(255) UNIQUE NOT NULL
);
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
UserID INT,
FOREIGN KEY (UserID) REFERENCES Users(UserID)
);
This structure preserves referential integrity, ensuring that every order relates to a valid user.
The Closing Argument
Selecting the right primary key is a critical aspect of relational database design. By avoiding common pitfalls, such as relying too heavily on natural keys, opting for composite keys in simple scenarios, and overlooking indexing considerations, you can improve your database's performance and maintainability.
Best Practices Recap
- Prefer Surrogate Keys: When business rules are likely to change.
- Limit Composite Keys: Keep it simple where possible.
- Plan for Growth: Anticipate future data volume.
- Consider Indexing: Choose keys that offer efficient lookups.
- Enforce Referential Integrity: Maintain relationships between tables effectively.
By keeping these considerations in mind, you will set your database up for success. Always remember, thoughtful design lays the groundwork for robust data management.
For further reading on relational database design, check the Microsoft documentation for design best practices that go deeper into these topics.
If you have any questions or need further clarification on primary keys and database design, please feel free to leave a comment below!