Troubleshooting Stream Data Issues in Corda with Webflux
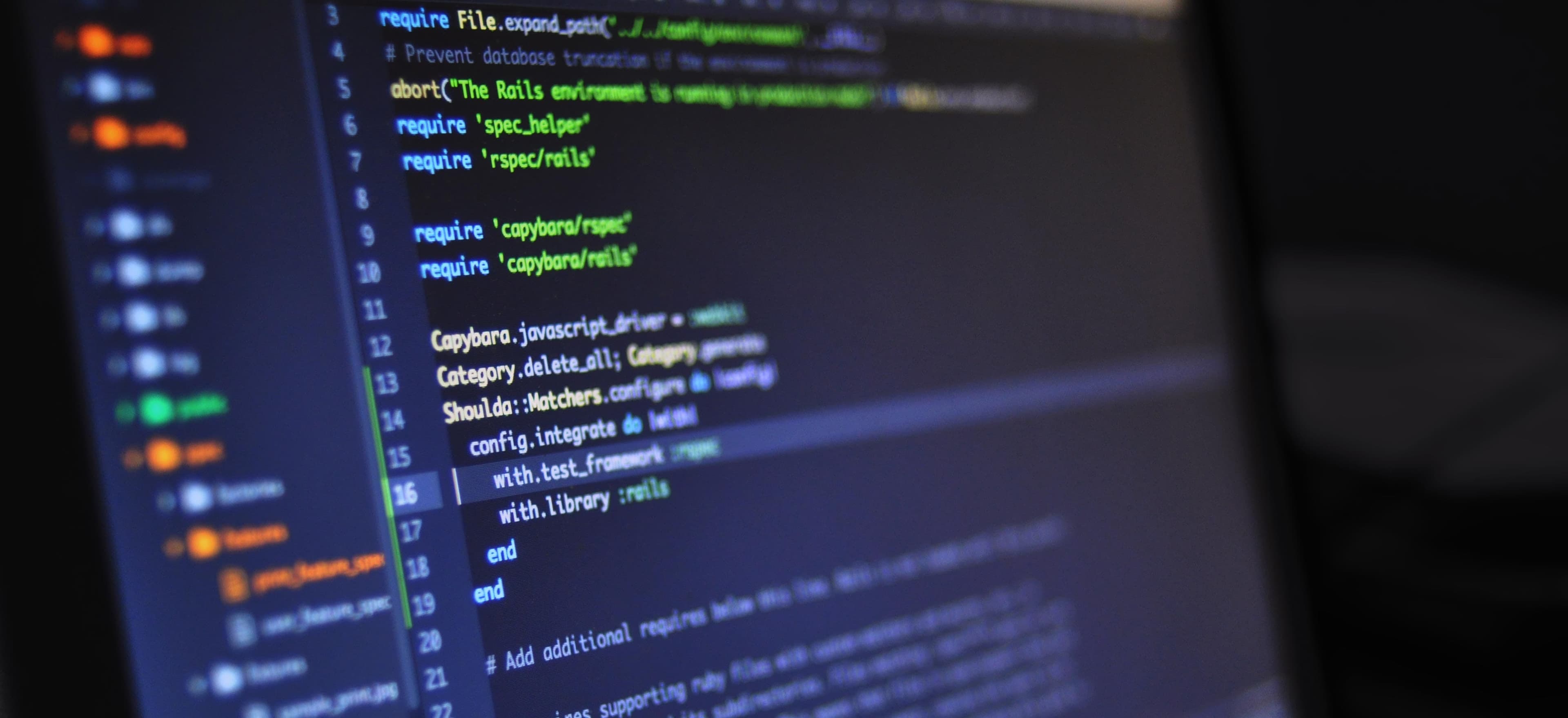
- Published on
Troubleshooting Stream Data Issues in Corda with Webflux
In the world of distributed ledger technologies, Corda is an eminent player. It brings together the concepts of blockchain and smart contracts, allowing diverse organizations to transact securely and efficiently. However, handling data streams in Corda can be challenging, especially when integrating with reactive programming libraries like Webflux. This blog post will delve into the common issues associated with stream data in Corda when using Webflux, how to troubleshoot these issues, and provide effective solutions.
Understanding Corda and Webflux
Before diving into troubleshooting, let’s clarify the technologies involved. Corda is a distributed ledger platform that excels in privacy and scalability. It allows transactions to be shared only among involved parties, unlike traditional blockchain networks. On the other hand, Webflux is a reactive programming framework built around the Reactor project, enabling asynchronous and non-blocking applications.
Reactive programming allows applications to handle a stream of data efficiently, enhancing performance in applications that rely on reactive data streams. However, integrating Corda's data persistence and transaction mechanisms with Webflux's reactive model can introduce complications.
Common Issues When Using Corda with Webflux
-
Concurrency Problems: In a reactive environment, multiple transactions might be attempting to access or modify the same data concurrently. This can lead to unexpected outcomes.
-
Data Manipulation Delays: Reactive systems are designed for responsiveness. Delays in Corda’s data access can lead to increased latency in data streams.
-
Error Handling: Reactive programming changes the paradigm of error handling compared to traditional synchronous programming.
-
Backpressure Handling: When the producer speed is faster than the consumer, it can lead to data loss or system overload.
-
Integration Overhead: Bridging two fundamentally different paradigms can add complexity to application design.
Setting Up Corda with Webflux: A Primer
Before we deep dive into troubleshooting, let's set up a basic Corda and Webflux integration to understand their interaction better.
Setup Basic Gradle Configuration
In your build.gradle
, include the necessary dependencies:
dependencies {
implementation "net.corda:corda-core:$corda_version"
implementation "org.springframework.boot:spring-boot-starter-webflux"
implementation "io.projectreactor.kotlin:reactor-kotlin-extensions"
// Other dependencies…
}
Corda Flow Example
A simple Corda flow that emits events might look like this:
class ExampleFlow : FlowLogic<SignedTransaction>() {
@Suspendable
override fun call(): SignedTransaction {
val transaction = TransactionBuilder()
// Build transaction logic here
// Finalize the transaction
return serviceHub.signInitialTransaction(transaction)
}
}
This flow emits a signed transaction, fundamental to performing actions in Corda.
Troubleshooting Data Flow Issues
Now that we've set the groundwork, let’s explore common issues and their solutions.
1. Concurrency Issues
Problem: When multiple flows run concurrently, they may attempt to read/write shared states simultaneously.
Solution: Utilize Corda's locking mechanism to ensure that only one flow can access a shared state at a time. Consider using the @StartableByRPC
annotation for flows that are meant to be initiated externally.
@StartableByRPC
class UpdateFlow(private val stateToUpdate: StateRef) : FlowLogic<SignedTransaction>() {
@Suspendable
override fun call(): SignedTransaction {
// Add logic to lock the state, in line with other flow conventions
//...
}
}
Commentary: By explicitly managing flow initiation, you impose control over which flows can concurrently execute, thus reducing the risk of unexpected errors.
2. Data Manipulation Delays
Problem: Latency in data access can be exacerbated in reactive systems, impacting performance.
Solution: Make use of caching strategies to reduce the number of read/write operations involving the shared ledger. This can greatly minimize delays.
Example of caching:
val cachedStates = ConcurrentHashMap<StateRef, StateType>()
fun getState(stateRef: StateRef): StateType? {
return cachedStates.computeIfAbsent(stateRef) {
// Fetch state from Corda serviceHub
}
}
Commentary: By caching the states that are frequently accessed, you reduce the need for repetitive ledger calls, thereby improving performance.
3. Error Handling
Problem: In a reactive context, errors propagated through streams may not always surface where you expect them to.
Solution: Utilize doOnError
and onErrorResume
in Webflux to gracefully handle exceptions.
Example:
fun getTransactionFlow(): Mono<SignedTransaction> {
return Mono.fromCallable {
// Call Corda service logic
}
.doOnError { error ->
// Log error or handle accordingly
}
.onErrorResume { error ->
// Fallback logic
Mono.empty()
}
}
Commentary: By specifying error-handling logic, you can control the response to failures in a more predictable way.
4. Backpressure Handling
Problem: When the input event stream overwhelms the processing stream, you may run into performance degradation.
Solution: Reactive frameworks, including Webflux, provide inherent mechanisms for backpressure. Configuring your sink can mitigate this.
Example implementation:
Flux.range(1, 10)
.onBackpressureBuffer(5) // Buffer with a limit
.subscribe {
// Processing logic
}
Commentary: By establishing backpressure strategies, you can manage your resource consumption more effectively.
5. Integration Overhead
Problem: Bridging Corda with Webflux introduces complication and additional latency.
Solution: Clearly define your boundaries and responsibilities. Avoid crossing the streams unnecessarily; instead, leverage reactive streams whenever feasible.
Consider having dedicated services interacting with Corda that expose APIs for other components, minimizing direct integration points.
The Closing Argument
While integrating Corda's robust framework with the reactive programming model of Webflux can introduce complexities, understanding potential pitfalls combined with effective strategies for troubleshooting can pave the path for success.
To summarize:
- Monitor concurrency with Corda locks.
- Implement caching to reduce data manipulation delays.
- Adopt reactive error-handling strategies.
- Manage backpressure to maintain system integrity.
- Limit integration points to balance complexity.
For a deeper understanding of Corda’s mechanisms, explore the Corda Documentation and for more on reactive programming, refer to the Project Reactor Documentation.
By using these troubleshooting methods and best practices, developers can efficiently handle stream data issues in Corda while harnessing the power of Webflux. Keep experimenting, and you will be on your way to mastering reactive programming within the Corda ecosystem!