Mastering Logging of Auth Attempts in Spring Boot Apps
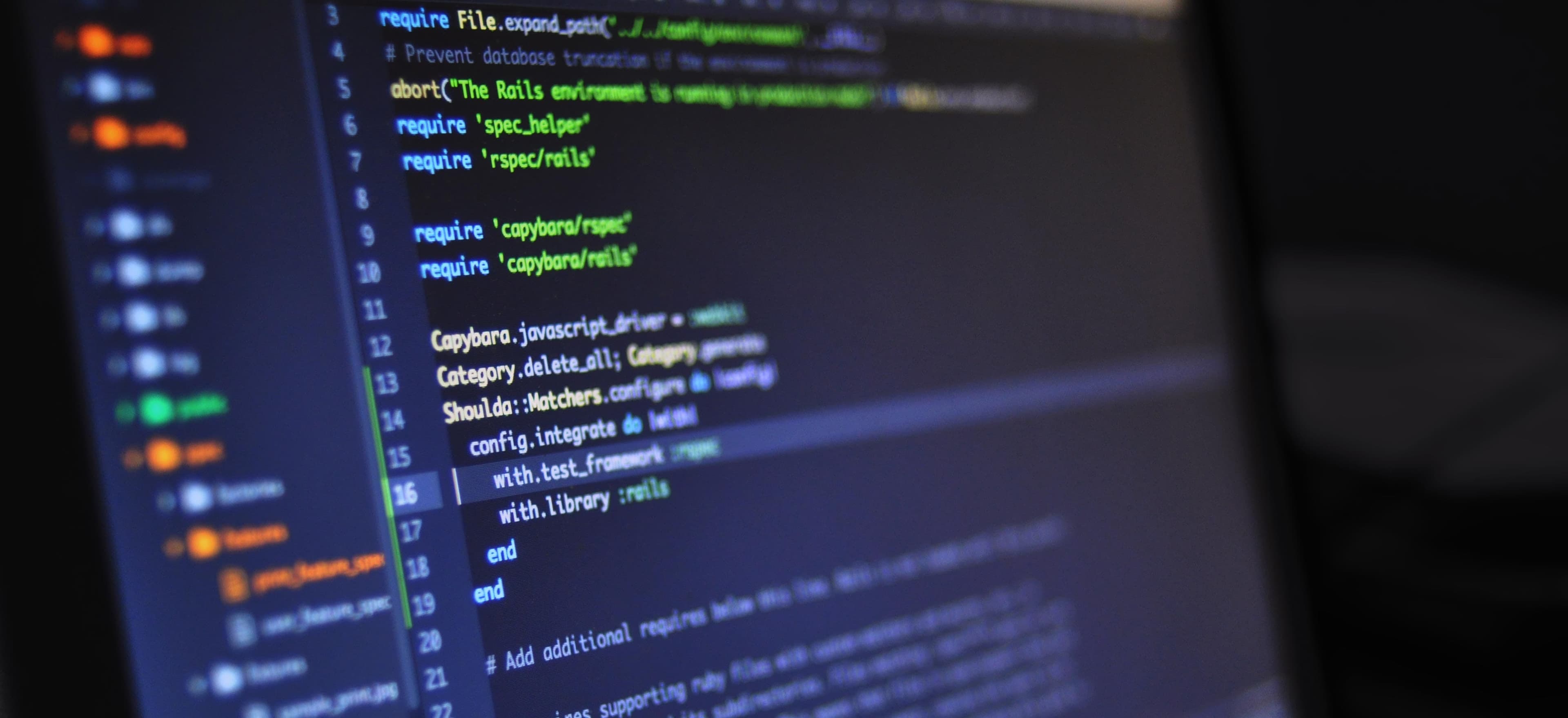
- Published on
Mastering Logging of Auth Attempts in Spring Boot Apps
In modern application development, particularly with Spring Boot, security and logging play a crucial role in maintaining a robust system. As more applications shift to online platforms, the relevance of secure authentication practices grows. Logging authentication attempts is not just beneficial for security audits; it also serves to help identify potential misuse or malicious activity.
In this blog post, we will delve into how to master logging of authentication attempts in your Spring Boot applications. We will cover:
- The importance of logging authentication attempts
- Setting up a Spring Boot application
- Implementing a logging mechanism for auth attempts
- Best practices for logging
Why Logging Authentication Attempts is Important?
Understanding why we need to log authentication attempts can help frame our discussion:
- Security Audits: Regular audits require a clear log of authentication requests.
- Intrusion Detection: By logging failed attempts, you can quickly identify patterns of malicious behavior.
- Debugging Issues: Knowing how authentication requests are handled can help debug issues in your application.
- Compliance: Many industries have strict regulations regarding user data and access.
Setting Up a Spring Boot Application
For this guide, we will create a simple Spring Boot application that includes basic user authentication. You can set up a new Spring Boot project using Spring Initializr. Select Spring Web and Spring Security as dependencies.
Example Project Structure
src
└── main
├── java
│ └── com
│ └── example
│ └── loggingdemo
│ ├── LoggingDemoApplication.java
│ ├── SecurityConfig.java
│ └── AuthController.java
└── resources
└── application.properties
Creating the Main Application Class
In LoggingDemoApplication.java
, create your Spring Boot application.
package com.example.loggingdemo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class LoggingDemoApplication {
public static void main(String[] args) {
SpringApplication.run(LoggingDemoApplication.class, args);
}
}
Utilizing the @SpringBootApplication
annotation encapsulates the functionality required to configure the application context.
Configuring Security
Now let's create the SecurityConfig.java
file to configure Spring Security.
package com.example.loggingdemo;
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin")
.password("{noop}password")
.roles("USER");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin();
}
}
The @EnableWebSecurity
Annotation
This annotation allows Spring Security to set up their default configurations, while WebSecurityConfigurerAdapter
enables the customization of security features.
Implementing Logging for Authentication Attempts
Now that we have our security configured, the next step is to implement logging for authentication attempts. We will leverage Spring Security's authentication events to capture login success and failure.
Adding an Event Listener
We'll create an AuthEventListener
class that listens for authentication events.
package com.example.loggingdemo;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.context.ApplicationListener;
import org.springframework.security.authentication.event.AuthenticationFailureBadCredentialsEvent;
import org.springframework.security.authentication.event.AuthenticationSuccessEvent;
import org.springframework.stereotype.Component;
@Component
public class AuthEventListener implements ApplicationListener<AuthenticationSuccessEvent> {
private static final Logger logger = LoggerFactory.getLogger(AuthEventListener.class);
@Override
public void onApplicationEvent(AuthenticationSuccessEvent event) {
logger.info("Authentication successful for user: " + event.getAuthentication().getName());
}
@Component
public class AuthFailureListener implements ApplicationListener<AuthenticationFailureBadCredentialsEvent> {
@Override
public void onApplicationEvent(AuthenticationFailureBadCredentialsEvent event) {
logger.warn("Authentication failed for user: " + event.getAuthentication().getName());
}
}
}
The Importance of Event Listeners
By implementing the ApplicationListener
interface, we can respond to authentication events, be they successes or failures. The logging framework provides a way to log both successful and failed attempts.
Configuring the Logging Level
Make sure to configure the logging level in application.properties
to capture these logs.
logging.level.com.example.loggingdemo=INFO
This configuration ensures that our login attempts are correctly logged at the desired level.
Sample Authentication Request
With our code in place, run your Spring Boot application, and navigate to the login form located at http://localhost:8080/login
. Here you can enter credentials.
Successful Authentication
When a user logs in successfully, you should see an entry in your application's log similar to:
INFO c.e.loggingdemo.AuthEventListener - Authentication successful for user: admin
Failed Authentication
If a user attempts to log in with incorrect credentials, the log entry would resemble:
WARN c.e.loggingdemo.AuthEventListener$AuthFailureListener - Authentication failed for user: admin
Best Practices for Logging
Now that we are logging authentication attempts, here are some best practices to consider:
-
Log Sensitive Information Judiciously: Never log passwords or sensitive information. Always ensure user information is logged securely.
-
Log Levels: Use appropriate log levels (INFO, WARN, ERROR). Successful attempts should be lower in severity than failed attempts to help prioritize incidents.
-
Retention Policy: Implement a retention policy for logs to manage disk space. Retain logs based on your organization's compliance requirements.
-
Centralized Logging: Consider centralizing your logs using platforms like ELK Stack or Splunk. This can greatly enhance your ability to monitor and analyze logs.
-
Alerting Mechanisms: Set alerts for anomalous behavior patterns such as multiple failed attempts to notify administrators proactively.
Bringing It All Together
Logging authentication attempts in a Spring Boot application is not only a best practice; it is essential for security and compliance. We explored a simple setup and implementation of logging using Spring Security's event framework, ensuring that both successful and failed authentication events were logged effectively.
With the information provided, you are well-equipped to implement robust authentication logging in your Spring Boot applications. This will greatly enhance your application's security posture and provide insights for future improvements.
For a deeper dive into Spring Security practices, explore the Spring Security documentation. Happy coding!