Streamlining Gradle Releases: GitLab, Jenkins, Artifactory
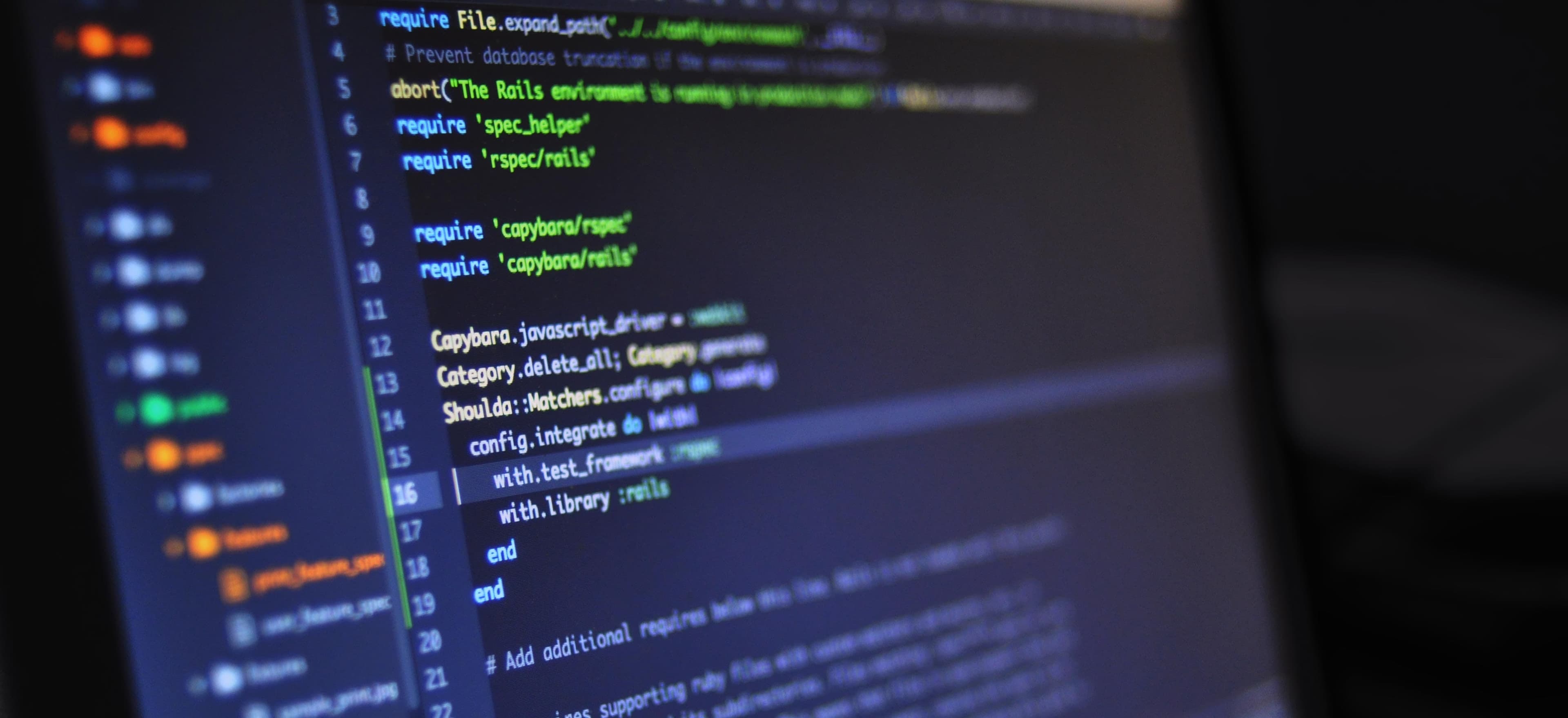
- Published on
Streamlining Gradle Releases: GitLab, Jenkins, Artifactory
In today’s fast-paced development landscape, efficiently managing releases is paramount. For Java applications, Gradle is among the most popular build systems, while GitLab, Jenkins, and Artifactory stand out as robust tools to aid in the Continuous Integration and Continuous Deployment (CI/CD) process. This article will walk you through streamlining your Gradle release process utilizing these tools, ensuring your workflow remains fluid and effective.
Table of Contents
- Understanding Gradle
- Integrating GitLab for Version Control
- Automating Builds with Jenkins
- Managing Artifacts with Artifactory
- Putting It All Together
- Conclusion
Understanding Gradle
Gradle is a powerful build automation system often used in Java projects. It provides flexibility and performance that other build tools might lack. Here’s a simple example of a Gradle build.gradle
file:
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework:spring-core:5.2.9.RELEASE'
testImplementation 'junit:junit:4.12'
}
Why Gradle?
Gradle stands out due to its ability to manage multi-project builds with ease and its rich ecosystem of plugins. By leveraging Gradle's task
feature, you can define custom build logic. Clear, concise structure in a build.gradle
file also makes it easy to understand dependencies and configurations.
For further reading on Gradle, check out the official Gradle documentation.
Integrating GitLab for Version Control
GitLab provides a comprehensive platform for version control. One of the main advantages of using GitLab is its built-in CI/CD capabilities. This allows you to define your build, test, and deployment processes all in one place.
Here’s how to set up a simple GitLab CI/CD pipeline for your Gradle project by including a .gitlab-ci.yml
file.
stages:
- build
- test
- deploy
build:
stage: build
script:
- ./gradlew build
artifacts:
paths:
- build/libs/*.jar
test:
stage: test
script:
- ./gradlew test
deploy:
stage: deploy
script:
- echo "Deploying to Artifactory"
Why GitLab?
By using GitLab, you can automate your CI/CD pipelines easily. The .gitlab-ci.yml
file defines all stages and scripts to run at each stage. This ensures every commit to your GitLab repository kicks off your release process automatically.
Automating Builds with Jenkins
While GitLab provides integrated CI/CD tooling, Jenkins is a standalone tool that specializes in building and deploying Java applications. You can connect Jenkins to GitLab to automate the build process.
Setting Up Jenkins
When setting up Jenkins for a Gradle project, you’ll often need to define a Jenkins pipeline. Below is a simple example of a Jenkinsfile:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sh './gradlew build'
}
}
}
stage('Test') {
steps {
script {
sh './gradlew test'
}
}
}
stage('Deploy') {
steps {
script {
echo "Deploying to Artifactory"
}
}
}
}
}
Why Jenkins?
Jenkins provides flexibility and an extensive plugin ecosystem to support various use cases. By connecting it to GitLab, you create a powerful combination for continuous delivery.
To dive deeper into Jenkins pipelines, visit the Jenkins Pipeline documentation.
Managing Artifacts with Artifactory
Artifactory is the solution for artifact management in your release process. After building your application with Gradle and automating that process in GitLab or Jenkins, you'll want a reliable place to store your binaries.
Publishing to Artifactory
Here’s a snippet of how you can publish your Gradle artifacts to Artifactory using the Gradle Artifactory Plugin:
plugins {
id "com.jfrog.artifactory" version "4.24.4"
}
apply from: "$rootDir/artifactory.gradle"
artifactory {
contextUrl = 'https://yourartifactory.com/artifactory'
publish {
repository {
repoKey = 'libs-release-local'
username = 'your-username'
password = 'your-password'
}
defaults {
publications('mavenJava') // Assuming you define a publication called 'mavenJava'
publishArtifacts = true
}
}
}
Why Artifactory?
Using Artifactory, you centralize all your binaries in one repository. It allows for better versioning management, dependency resolution, and security control. It can significantly simplify your release workflow by ensuring that the right artifacts are available during deployment.
Putting It All Together
When you combine GitLab, Jenkins, Gradle, and Artifactory, your release process becomes more streamlined. You can start with a version-controlled Gradle project hosted on GitLab. Every commit triggers a Jenkins job that builds and tests your application. Finally, successful builds publish artifacts to Artifactory.
Process Overview:
- Code changes: Developers push changes to GitLab.
- Build Trigger: GitLab CI/CD or Jenkins triggers build and test automatically.
- Artifact Storage: Upon successful builds, Jenkins publishes artifacts to Artifactory.
- Deployment Ready: Artifacts are now stored reliably for any subsequent deployments.
My Closing Thoughts on the Matter
As you can see, integrating Gradle with GitLab, Jenkins, and Artifactory can significantly streamline your release process for Java applications. Understanding how each tool works and how they complement each other is essential for building efficient CI/CD pipelines.
Whether you're a solo developer or part of a larger team, adopting these technologies can lead to faster development cycles, more reliable builds, and easier artifact management. Start streamlining your process today and take your Java application releases to the next level!
By consistently improving your CI/CD practices using these tools, the journey from code commit to production can be smoother and more predictable. Happy coding!