Achieving Low Latency in Java: Common Pitfalls to Avoid
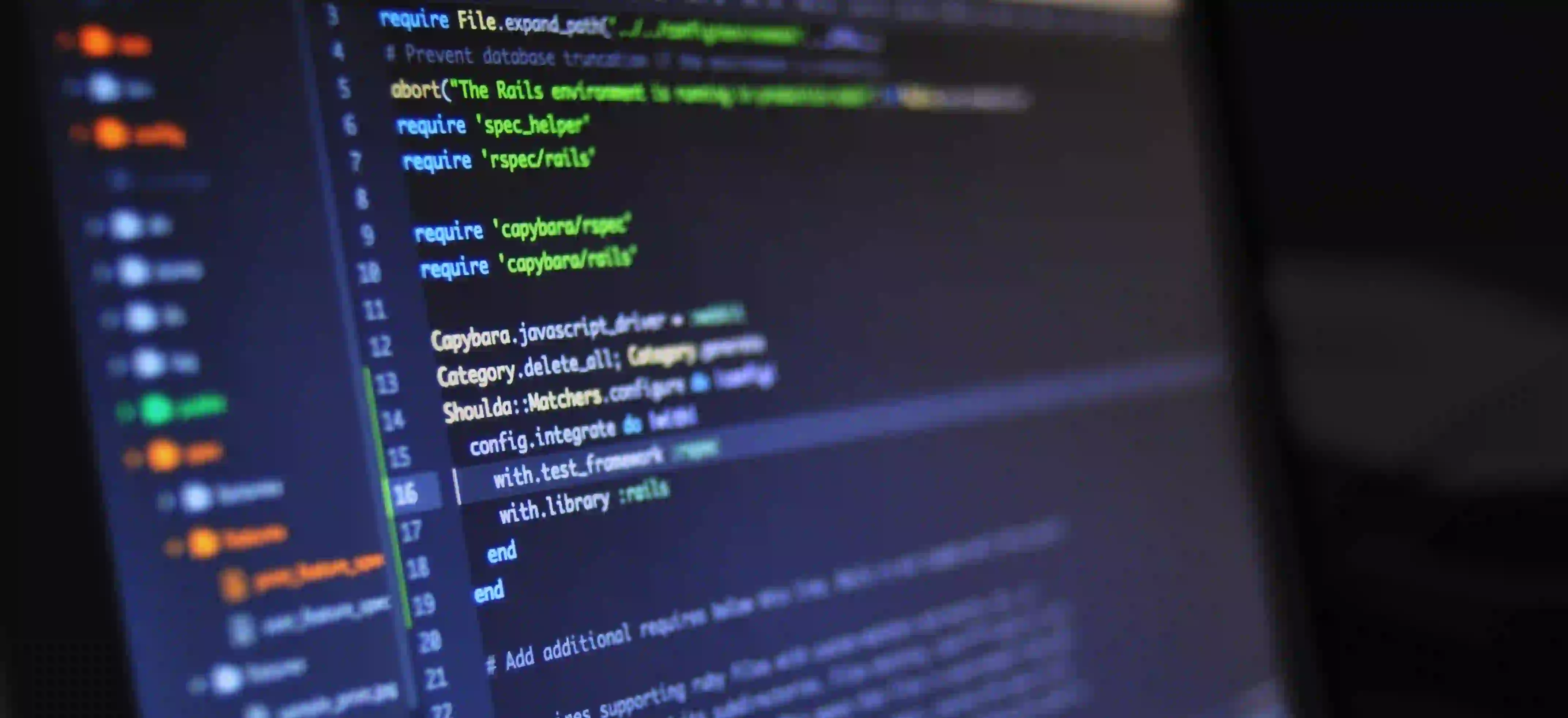
Achieving Low Latency in Java: Common Pitfalls to Avoid
In the world of software development, low latency is not merely a target; it is a necessity. In applications where user experience and real-time processing are critical—like online gaming, trading platforms, and high-frequency analytical tools—latency can make or break success.
As a seasoned Java developer, you may find that achieving low latency isn't just about writing high-performance code. It involves understanding the intricacies of the Java Virtual Machine (JVM), garbage collection (GC) considerations, threading, and many other factors. In this blog post, we will discuss common pitfalls to avoid when striving for low latency in Java applications.
Understanding Latency
What is Latency?
Latency refers to the time taken to process a request. It encompasses several phases, including network latency, server processing time, and response back to the client.
Why Low Latency is Crucial
Low latency improves user experience. Imagine a web application where every click takes seconds to respond—frustration sets in quickly. Moreover, in applications like trading platforms, every millisecond counts. Achieving low latency can increase efficiency and maximize your competitive edge.
The Java Ecosystem
Before addressing common pitfalls, it's essential to grasp our tools—the Java ecosystem itself:
- Java Virtual Machine (JVM): The JVM plays a pivotal role in how Java applications run and how memory is managed.
- Garbage Collection (GC): Automatic memory management comes with its own set of challenges, particularly with latencies during GC pauses.
Understanding and optimizing your use of the JVM and GC can lead to lower latency—a crucial step for finely-tuned Java applications.
Common Pitfalls and How to Avoid Them
1. Ignoring Garbage Collection
A common mistake developers make is underestimating the impact of garbage collection on application latency. The GC is essential for memory management, but certain algorithms can introduce unpredictable pauses.
Solution: Choose the Right Garbage Collector
The JVM offers several GC options:
- G1 GC: Good for low-latency applications with overshoot settings.
- ZGC: For applications that require lower latency while managing large heaps.
// Example of setting up G1 GC in your Java application.
java -XX:+UseG1GC -Xmx2g -Xms1g -jar YourApplication.jar
Using the right collector can minimize latency during memory cleanup cycles.
2. Inefficient Thread Management
Java provides robust threading capabilities. However, creating too many threads can lead to contention and increased latency.
Solution: Use Thread Pools
Using a thread pool can significantly enhance performance by controlling the number of active threads.
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
public class ThreadPoolExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(4);
for (int i = 0; i < 10; i++) {
final int taskId = i;
executor.submit(() -> {
System.out.println("Executing task: " + taskId);
});
}
executor.shutdown();
}
}
By using a fixed thread pool, you reduce the overhead associated with creating and destroying threads, thereby minimizing latency.
3. Excessive Object Creation
Frequent object creation leads to more frequent garbage collection cycles. This can introduce unpredictable latencies into your application.
Solution: Reuse Objects
Reusing immutable objects can reduce garbage collection overhead.
public class ConnectionPool {
private static final int POOL_SIZE = 10;
private Connection[] connections = new Connection[POOL_SIZE];
private boolean[] available = new boolean[POOL_SIZE];
public ConnectionPool() {
for (int i = 0; i < POOL_SIZE; i++) {
connections[i] = new Connection();
available[i] = true;
}
}
public synchronized Connection getConnection() {
for (int i = 0; i < POOL_SIZE; i++) {
if (available[i]) {
available[i] = false;
return connections[i];
}
}
return null; // Or wait
}
public synchronized void releaseConnection(Connection conn) {
// Logic to release connection back to pool
}
}
Reusing objects minimizes the garbage collection cycle and keeps latency low.
4. Ignoring Network Latency
Network calls can introduce significant latency, especially if using synchronous blocking calls.
Solution: Utilize Asynchronous Calls
By using asynchronous communication, you can continue processing other tasks without waiting on network responses.
import java.util.concurrent.CompletableFuture;
public class AsyncNetworkCall {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
return makeNetworkCall();
});
// Continue doing other work
System.out.println("Doing other processing...");
// Wait for result
future.thenAccept(result -> System.out.println("Received result: " + result));
}
private static String makeNetworkCall() {
// Simulate network call
return "Network Response";
}
}
This allows you to reduce the impact of network latency on your application's performance.
5. Overhead from Reflection
Reflection is a powerful but slow feature in Java that could introduce additional latency.
Solution: Minimize Reflection
Use reflection only where absolutely necessary. If you find yourself using it frequently, consider alternative approaches.
// Instead of reflection, use this:
public class User {
private String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Minimize the use of reflection to enhance performance.
6. High-Level Libraries
While high-level libraries can speed up development, they sometimes introduce latency due to abstraction layers.
Solution: Optimize Libraries and Frameworks
Evaluate the libraries you use. Opt for those known for performance. Familiarize yourself with lightweight alternatives like RxJava or Spring WebFlux for reactive programming—a great way to enhance responsiveness.
Bringing It All Together
Low latency is a paramount objective for many Java applications, but reaching it requires vigilance. You must be aware of and avoid common pitfalls like poor garbage collection practices, inefficient threading, excessive object creation, network latency, reflection overhead, and bloated libraries.
Keep in mind the robust features that Java offers—the proper use of garbage collection strategies, smart threading, object reuse, and asynchronous processing can lead to significant improvements in latency. Optimizing your Java application isn't merely about code quality; it's also about leveraging the right tools and techniques.
As you progress in your journey toward building low-latency applications, continual profiling and tuning are essential. Tools like VisualVM or Java Mission Control can provide deeper insights into your application's performance. Armed with this knowledge, you are well on your way to creating high-performance, low-latency applications in Java.
Further Reading
- Java Garbage Collection Basics
- Java Concurrency in Practice
- Optimizing Java: Performance Tuning Tips
By taking these steps, you can ensure your Java applications meet the low latency goals necessary to thrive in today’s fast-paced technological environment. Happy coding!