Common XML Interview Pitfalls for Java Programmers
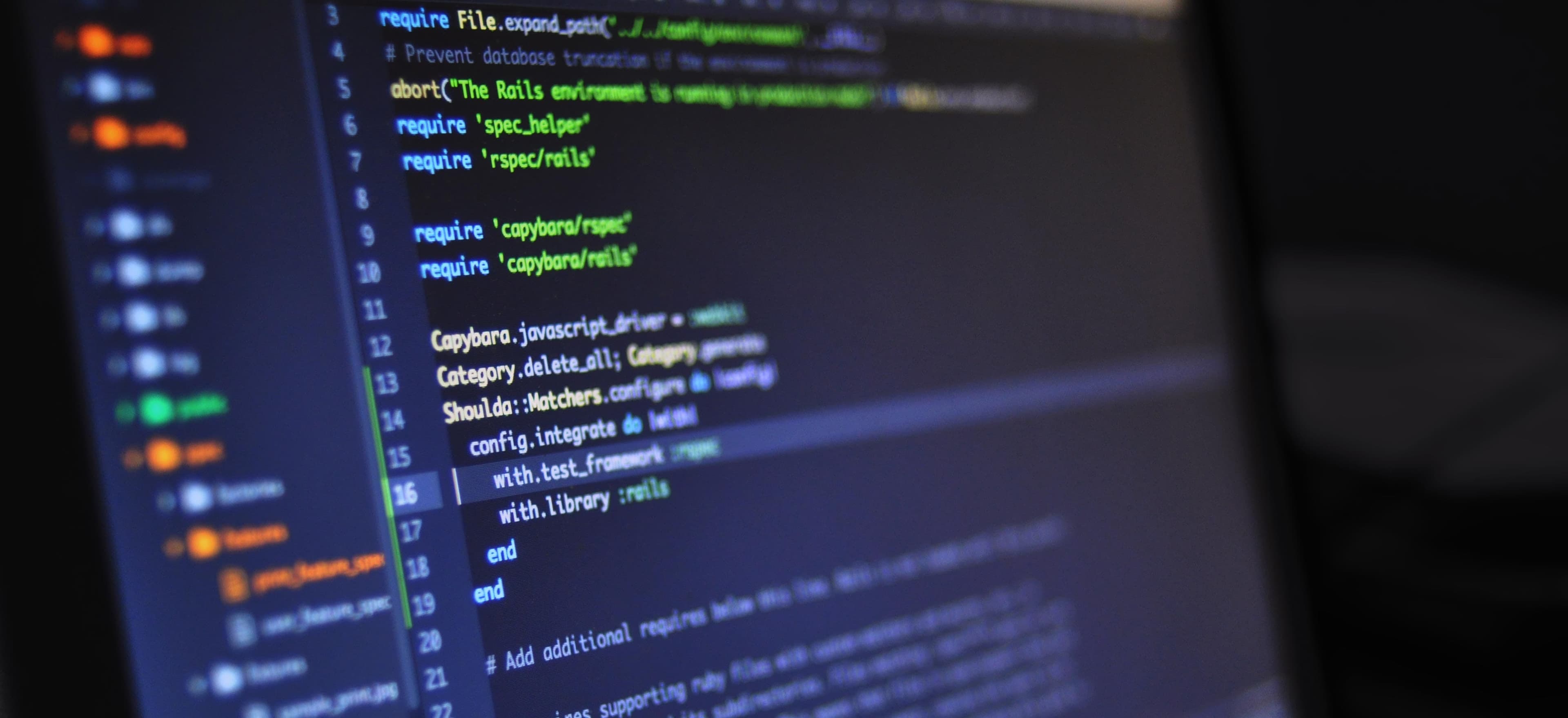
- Published on
Common XML Interview Pitfalls for Java Programmers
With the rise of web services, APIs, and data interchange formats, knowledge of XML has become indispensable for Java programmers. However, navigating through XML-related questions can lead to unintentional missteps in interviews. In this blog post, we will explore some common pitfalls encountered during XML interviews, provide insightful examples, and offer tips on how to avoid these traps.
Understanding XML Basics
Before diving into the common pitfalls, it’s essential to have a solid understanding of XML fundamentals. XML (Extensible Markup Language) is a markup language that defines a set of rules for encoding documents in a format that is both human-readable and machine-readable.
Key Features of XML:
- Self-descriptive: XML files are structured in a way that facilitates understanding of the data's meaning.
- Hierarchical structure: Data is organized in a tree-like format enabling a clear representation.
- Extensible: XML allows you to define your own tags to suit the data it's meant to hold.
Basic XML Example
Here is a simple XML structure:
<book>
<title>Effective Java</title>
<author>Joshua Bloch</author>
<year>2018</year>
</book>
This representation of a book includes a title, author, and year, demonstrating the self-descriptive nature of XML.
Common Pitfalls to Avoid
1. Ignoring XML Standards
One of the most frequent missteps by developers is not adhering to XML standards. XML has specific guidelines, such as case sensitivity, well-formedness, and valid syntax.
Example of Well-formedness:
<book>
<title>Effective Java</title>
<author>Joshua Bloch</author>
<year>2018</year>
</book>
Contrast this with the following incorrect XML:
<Book>
<Title>Effective Java</Title>
<author>Joshua Bloch</author>
<year>2018</year>
<book>
The capitalization of tags in the first example must be consistent and should always be closed. A well-formed XML document is critical as many parsers will fail if it does not meet these elementary rules.
2. Overlooking XML Parsing Techniques
XML parsing isn’t just about reading data but involves handling that data effectively. There are multiple libraries in Java for parsing XML documents, such as:
- DOM (Document Object Model)
- SAX (Simple API for XML)
- StAX (Streaming API for XML)
Each of these has strengths and weaknesses.
Example of DOM Parsing:
import org.w3c.dom.*;
import javax.xml.parsers.*;
public class XMLDOMParser {
public static void main(String[] args) throws Exception {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse("books.xml");
NodeList nodeList = doc.getElementsByTagName("book");
for (int i = 0; i < nodeList.getLength(); i++) {
Element element = (Element) nodeList.item(i);
System.out.println("Title: " + element.getElementsByTagName("title").item(0).getTextContent());
}
}
}
Why Choose DOM?
- It loads the entire XML document into memory.
- It allows manipulation of elements, attributes, and text content through the Java interface.
However, one should be cautious of memory consumption, especially with large XML files.
3. Confusing XML with JSON
Developers often get mixed up by equating XML with JSON (JavaScript Object Notation). Though they serve similar purposes, their use-cases, structures, and advantages differ.
Key Differences:
- Syntax: XML is verbose and includes tags, while JSON uses key-value pairs.
- Data Types: JSON supports primitive data types, whereas XML does not impose any data types.
- Readability: JSON tends to be easier for humans to read and write.
A well-rounded developer must understand scenarios where XML might be more beneficial, such as when needing extensive metadata support or document validation.
4. Neglecting XML Namespaces
XML namespaces are crucial when dealing with XML documents that contain elements from multiple vocabularies. Without them, developers can face issues with element name conflicts.
Example of Using XML Namespaces:
<book xmlns:auth="http://example.com/authors">
<auth:title>Effective Java</auth:title>
<auth:author>Joshua Bloch</auth:author>
</book>
Using namespaces prevents collisions between tags that might have the same name but different meanings.
5. Underestimating Performance Considerations
XML's ability to represent data in a flexible manner comes with trade-offs, often in terms of performance.
- Memory Consumption: Libraries like DOM can consume substantial memory for large XML files.
- Parsing Speed: SAX and StAX parsers are more memory-efficient but require a different programming paradigm, often leading to callbacks that can complicate code.
Utilize benchmarks to determine your parsing strategy based on XML size and context.
Practical Tips to Prepare
1. Familiarize with Common Libraries
Learn several substantial XML libraries available in Java, such as:
- JAXP (Java API for XML Processing)
- Jakarta XML Binding (JAXB)
- XStream for serialization.
2. Keep Up with XML Standards
Regularly visit the W3C (World Wide Web Consortium) to stay updated on XML standards and practices. Understanding these changes can provide an edge during discussions.
3. Practice XML Manipulation
Create sample XML files and practice parsing and manipulating them using different libraries. The more you practice, the more comfortable you'll become.
4. Mock Interviews
Conduct mock technical interviews focusing on XML-related questions to gain confidence. Platforms like LeetCode and Interviewing.io can provide valuable insights and practice.
Final Thoughts
In summary, mastering XML and its nuances can significantly impact your performance in Java-related interviews. By understanding the foundational principles of XML, avoiding common pitfalls, and equipping yourself with practical experience and knowledge, you'll bolster your confidence and proficiency.
Engaging with tried-and-true practices will not only prepare you for your next interview but also enhance your capability to work with XML in real-world applications. Embrace the power of XML and show potential employers that you are well-versed in this critical domain.
Happy coding!
Checkout our other articles