Mastering Java Strings: Answers to 10 Common Questions
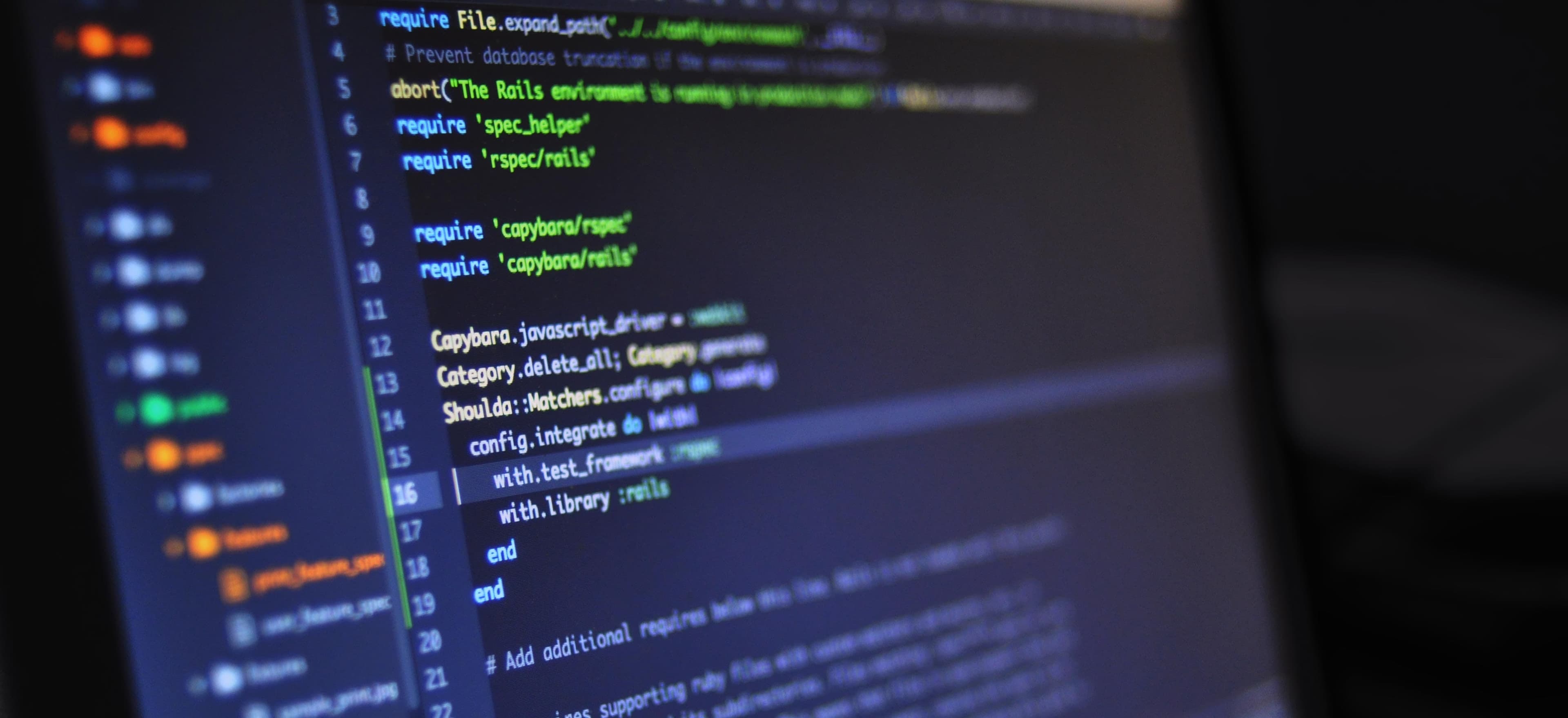
- Published on
Mastering Java Strings: Answers to 10 Common Questions
Java is a powerful programming language renowned for its broad applicability and efficiency. Among its many features, the String class stands out as a fundamental part of working with text. Despite its significance, many developers—especially those new to Java—often find themselves puzzled by strings. In this blog post, we will address 10 common questions about Java strings, enhancing your understanding and mastery of this essential topic.
Table of Contents
- What Are Strings in Java?
- How to Create Strings?
- What is the Difference Between String, StringBuilder, and StringBuffer?
- How to Concatenate Strings?
- How to Compare Strings?
- How to Extract Substrings?
- How to Convert Strings to Other Types?
- How to Manipulate String Case?
- How to Trim Whitespace from Strings?
- Best Practices for Working with Strings
1. What Are Strings in Java?
In Java, a String is a sequence of characters. They are objects that encapsulate a fixed string of text and offer various methods for string manipulation. Strings are immutable, meaning once created, they cannot be altered; any changes result in a new string object.
Here's an example:
String greeting = "Hello, World!";
In this code, we declare a string variable greeting
and assign it the text "Hello, World!". Understanding the immutability of strings is crucial to avoid common pitfalls in Java programming.
2. How to Create Strings?
You can create strings in two primary ways:
- String Literal: This is straightforward, as shown earlier.
new
Keyword: Using the new keyword allows you to create a string explicitly.
Examples:
String literalString = "Hello, World!";
String newString = new String("Hello, World!");
While both approaches result in a string with the same content, the first method is preferred for its simplicity and efficiency.
3. What is the Difference Between String, StringBuilder, and StringBuffer?
String
As discussed, strings are immutable. If you concatenate two strings, a new string object is created.
StringBuilder and StringBuffer
-
StringBuilder: This is used for mutable strings and is faster than StringBuffer because it is not synchronized.
-
StringBuffer: Like StringBuilder, it allows modifications but is synchronized, making it thread-safe.
Example of using StringBuilder:
StringBuilder sb = new StringBuilder("Hello");
sb.append(", World!");
System.out.println(sb.toString()); // Outputs: Hello, World!
When to use which? If you are working in a single-threaded context, prefer StringBuilder for efficiency. Use StringBuffer in a multi-threaded environment.
4. How to Concatenate Strings?
Java provides several ways to concatenate strings:
-
Using the
+
Operator:String first = "Hello"; String second = "World"; String combined = first + ", " + second + "!"; System.out.println(combined);
-
Using
concat()
Method:String combined = first.concat(", ").concat(second).concat("!");
-
Using StringBuilder:
StringBuilder sb = new StringBuilder(); sb.append(first).append(", ").append(second).append("!"); String combinedBuilder = sb.toString();
Each method has its own use case, but for performance considerations in large-scale string operations, StringBuilder is preferred.
5. How to Compare Strings?
You can compare strings in Java using the equals()
method for content equality and ==
for reference equality.
Example:
String s1 = new String("test");
String s2 = new String("test");
String s3 = s1;
System.out.println(s1.equals(s2)); // true (content-wise)
System.out.println(s1 == s2); // false (reference-wise)
System.out.println(s1 == s3); // true (same reference)
Using equals()
is the safe way to check string contents since it compares the actual text rather than the memory addresses.
6. How to Extract Substrings?
To extract parts of a string, you can use the substring()
method, which takes two indices.
Example:
String str = "Hello, World!";
String subStr = str.substring(7, 12); // Extracts "World"
System.out.println(subStr);
The first parameter is inclusive, while the second is exclusive. If you only want to extract from a starting index to the end, you can use:
String subStrToEnd = str.substring(7); // "World!"
Understanding how to extract substrings can help you process text more flexibly.
7. How to Convert Strings to Other Types?
Converting strings to other types is relatively straightforward in Java.
To Integer:
String numberString = "12345";
int number = Integer.parseInt(numberString);
To Double:
String floatString = "12.34";
double value = Double.parseDouble(floatString);
Always handle exceptions with a try-catch
block, as NumberFormatException
will be thrown if the string cannot be parsed.
8. How to Manipulate String Case?
Java provides methods to change the case of strings easily:
toUpperCase()
toLowerCase()
Example:
String mixedCase = "Hello, World!";
String upper = mixedCase.toUpperCase(); // "HELLO, WORLD!"
String lower = mixedCase.toLowerCase(); // "hello, world!"
Changing string case is important for uniformity when comparing strings.
9. How to Trim Whitespace from Strings?
To remove leading and trailing whitespace from a string, use the trim()
method:
String paddedString = " Hello, World! ";
String trimmed = paddedString.trim(); // "Hello, World!"
Trimming is essential for cleaning user input or data that may come from external sources.
10. Best Practices for Working with Strings
- Prefer StringBuilder for Concatenation: When combining strings in loops or complex operations, use StringBuilder.
- Immutable Objects: Remember that strings are immutable. Concatenations will create new string objects.
- Use String Constants: Use string constants when applicable to conserve memory and enhance performance.
In conclusion, mastering Java strings paves the way for writing efficient, readable, and maintainable code. As you continue your Java journey, remember that practice makes perfect. For more advanced string manipulation techniques and best practices, explore the Oracle Java Documentation.
With this post, we hope you feel more confident tackling the often-misunderstood realm of Java strings. Happy coding!
Checkout our other articles