Boost Your Java App's Speed with Parallel Processing Tricks
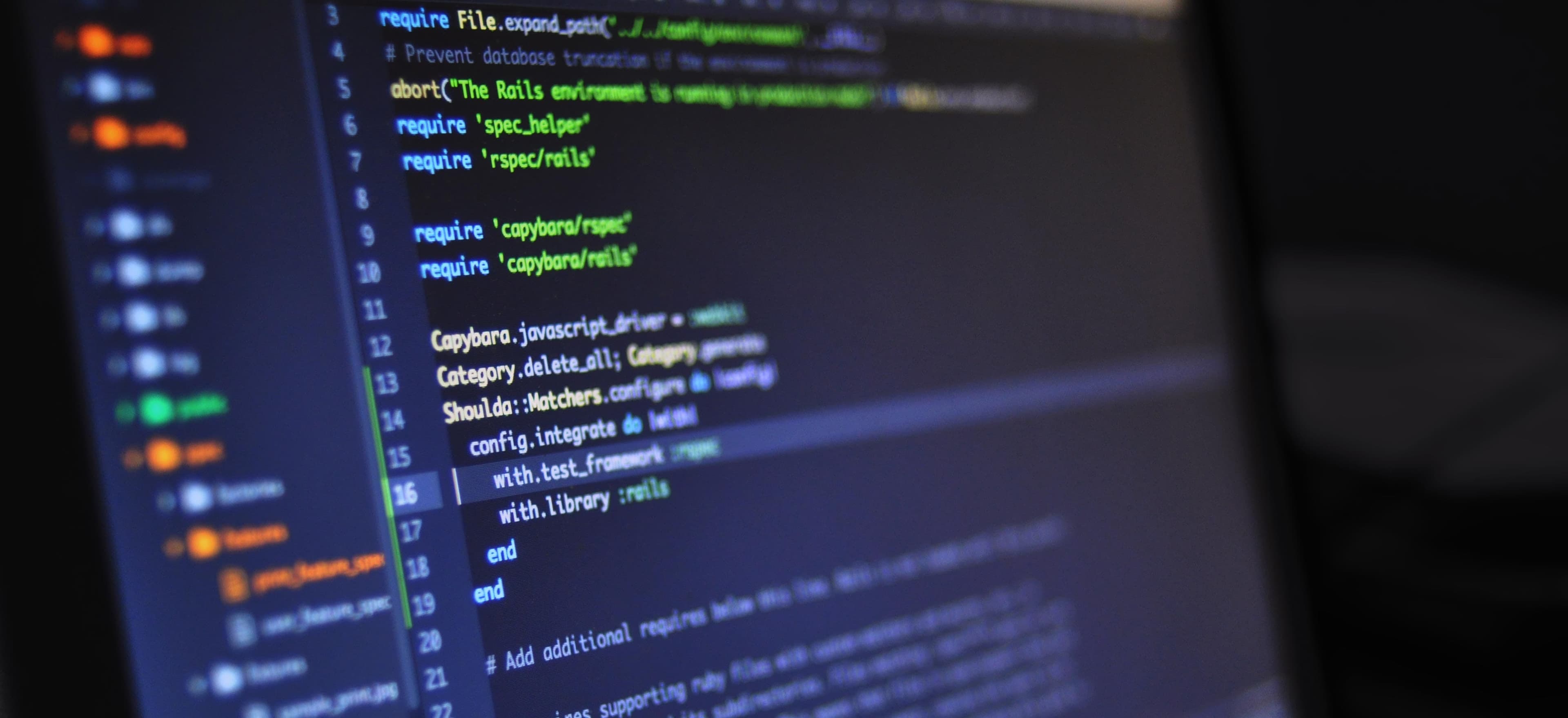
- Published on
Boost Your Java App's Speed with Parallel Processing Tricks
In the ever-evolving landscape of software development, speed and efficiency hold immense value. Java applications, whether enterprise-level or small-scale projects, can significantly benefit from improving their processing capabilities. One highly effective way to achieve this is by employing parallel processing techniques. In this blog post, we will explore various methods to harness the power of parallel processing in Java, enhancing your app's performance and user experience.
Understanding Parallel Processing
Parallel processing refers to the execution of multiple tasks simultaneously. In Java, this can be accomplished through various means, such as multi-threading, the Fork/Join framework, and libraries like Java Streams. By breaking down tasks into smaller parts that can be processed at the same time, you can utilize the full potential of modern multicore processors.
Why Parallel Processing?
- Improved Performance: When tasks are executed in parallel, completion times can decrease significantly.
- Efficient Resource Utilization: Parallel processing leverages multiple CPU cores, maximizing computational efficiency.
- Scalability: Applications can handle larger datasets more effectively, providing room for growth without major overhauls.
Java Multi-threading Basics
Java's built-in support for multi-threading allows developers to create applications that can perform multiple operations concurrently. Let’s dive into a simple example to illustrate how this works.
class Task extends Thread {
public void run() {
System.out.println(Thread.currentThread().getName() + " is running.");
// Simulating a time-consuming task
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class MultiThreadExample {
public static void main(String[] args) {
Task task1 = new Task();
Task task2 = new Task();
task1.start();
task2.start();
// Wait for tasks to finish
try {
task1.join();
task2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Tasks completed.");
}
}
Why This Code?
- Extending Thread: By extending the
Thread
class, we define a task that can run independently. - Running Tasks: Each task runs in a separate thread, allowing both tasks to execute concurrently.
- Join Method: This ensures the main thread waits for the completion of both tasks before proceeding.
This is a foundational approach, but as applications grow in complexity, managing multiple threads manually can become cumbersome. Thus, we turn our attention to higher-level abstractions.
Executor Framework
Java’s Executor
framework simplifies multi-threading and provides a higher level of control over the thread lifecycle. Here's how you can use it:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
class Task implements Runnable {
public void run() {
System.out.println(Thread.currentThread().getName() + " is executing task.");
// Simulating work with sleep
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class ExecutorExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(2);
for (int i = 0; i < 4; i++) {
executor.execute(new Task());
}
executor.shutdown();
while (!executor.isTerminated()) {
// wait for all tasks to complete
}
System.out.println("All tasks completed.");
}
}
Benefits of the Executor Framework:
- Thread Pool Management: You don’t need to manually create and manage threads. The
ExecutorService
handles it for you. - Scalability: With a fixed thread pool, you can easily control how many threads are running at a time, reducing resource contention.
Fork/Join Framework
For tasks that can be broken into smaller sub-tasks, the Fork/Join framework is an excellent option. It's built to take advantage of multicore processors and dynamically adjust the number of threads needed to complete tasks. Here’s an illustrative example:
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
class SumTask extends RecursiveTask<Integer> {
private final int start;
private final int end;
public SumTask(int start, int end) {
this.start = start;
this.end = end;
}
@Override
protected Integer compute() {
if (end - start <= 10) {
// Small enough to compute directly
int sum = 0;
for (int i = start; i < end; i++) {
sum += i;
}
return sum;
} else {
// Split the task
int mid = (start + end) / 2;
SumTask leftTask = new SumTask(start, mid);
SumTask rightTask = new SumTask(mid, end);
leftTask.fork(); // initiate the left task
return rightTask.compute() + leftTask.join(); // compute right task
}
}
}
public class ForkJoinExample {
public static void main(String[] args) {
ForkJoinPool pool = new ForkJoinPool();
SumTask task = new SumTask(0, 100);
int result = pool.invoke(task);
System.out.println("Sum is: " + result);
}
}
Explanation:
- RecursiveTask: This is a specialized task type that can return a result.
- Splitting Tasks: The
compute
method splits the task when it is too large, allowing for efficient parallel execution. - Fork and Join: The
fork()
method starts the left task, whilejoin()
waits for its completion.
Using Java Streams for Parallel Processing
Java 8 introduced the Streams API, which allows you to implement parallel processing with minimal code. Let’s take a look at an example:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
// Using parallel streams to sum numbers
int sum = numbers.parallelStream()
.mapToInt(Integer::intValue)
.sum();
System.out.println("Sum is: " + sum);
}
}
Why Use Streams?
- Conciseness: The syntax is straightforward; you can process data without dealing with threading explicitly.
- Built-in Parallelism: Simply changing
stream()
toparallelStream()
enables parallel processing. - Lazy Execution: Streams are lazily evaluated, which can lead to further performance improvements.
Final Considerations
Parallel processing can drastically improve the performance of your Java applications by leveraging multithreading and multicore processors effectively. By using various tools like the Executor framework, Fork/Join framework, and the Streams API, you can create robust, efficient applications that handle tasks swiftly.
If you're interested in diving deeper into parallel processing techniques in Java, consider exploring the following resources:
Implement these tricks, and watch your Java applications soar in speed and efficiency. Happy coding!
Checkout our other articles