Preventing Common Memory Leak Pitfalls in Java
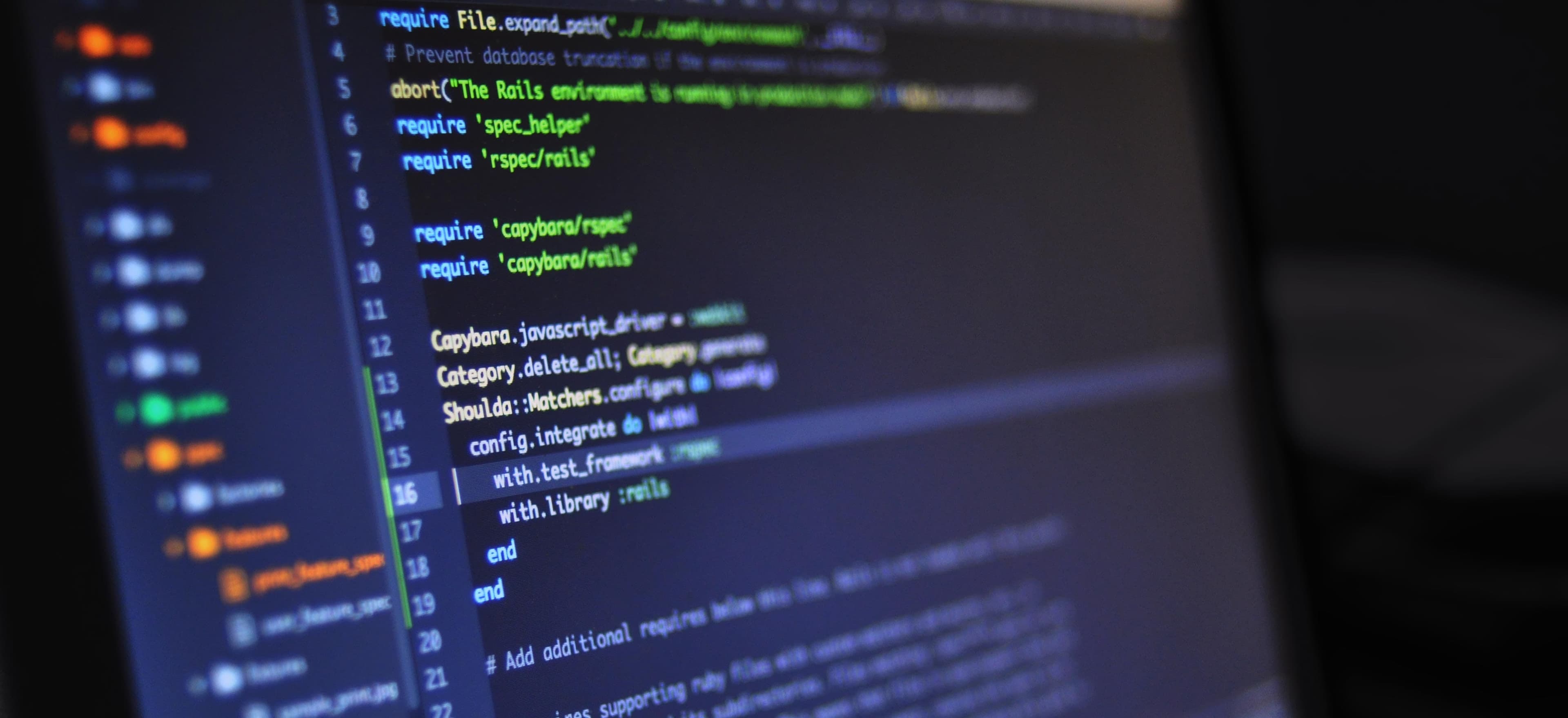
- Published on
Preventing Common Memory Leak Pitfalls in Java
Java, known for its ease of use and robust security features, offers automatic garbage collection to help manage memory. However, Java developers can still fall into the trap of memory leaks. These leaks can significantly impact application performance and lead to wasted system resources. In this blog post, we'll explore common memory leak pitfalls in Java and provide code snippets to demonstrate the best practices to prevent them.
What is a Memory Leak?
A memory leak in Java occurs when an application retains references to objects that are no longer needed, preventing the garbage collector from reclaiming that memory. Over time, this can lead to increased memory consumption, slower application performance, and potential application crashes.
Why Should You Care?
Effective memory management is critical in building scalable and responsive Java applications. Ignoring memory leaks can lead to resource depletion, cause OutOfMemoryError exceptions, and negatively affect user experience. Understanding how to prevent memory leaks is essential for any Java developer.
Common Memory Leak Pitfalls
1. Unmanaged Event Listeners
Event listeners are a common source of memory leaks in Java applications. When an object registers for event notifications and does not deregister when it is no longer needed, it can remain in memory, preventing garbage collection.
Example
import java.awt.*;
import java.awt.event.*;
public class MemoryLeakExample {
private Frame frame;
public void createFrame() {
frame = new Frame("Memory Leak Example");
Button button = new Button("Click Me");
// Registering an event listener
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked");
}
});
frame.add(button);
frame.setSize(300, 200);
frame.setVisible(true);
}
public void cleanUp() {
// Problematic: Not removing the listener
// The Frame might still reference the ActionListener
frame.dispose();
}
}
Solution
To prevent memory leaks in event listeners, ensure that you deregister them when the object is no longer needed.
public void cleanUp() {
for (ActionListener listener : button.getActionListeners()) {
button.removeActionListener(listener);
}
frame.dispose();
}
2. Static Collection References
Using static collections (like lists or maps) to hold references to objects can lead to memory leaks. If you store objects in a static collection, they persist for the lifetime of the application.
Example
import java.util.*;
public class StaticCollectionExample {
private static List<Object> objects = new ArrayList<>();
public void addObject() {
objects.add(new Object()); // Object reference retained
}
}
Solution
Use weak references or ensure that you clear the static collection when it is no longer needed.
import java.util.*;
public class StaticCollectionFixed {
private static List<Object> objects = new ArrayList<>();
public void addObject() {
objects.add(new Object());
// Consider clearing this list when done
}
public void clearObjects() {
objects.clear(); // Prevent memory leak
}
}
3. Long-lived Threads Holding onto Resources
Background threads can inadvertently hold onto resources if they reference objects that have outlived their usefulness.
Example
public class MemoryLeakInThread {
private static Thread longRunningThread;
public void startThread() {
longRunningThread = new Thread(() -> {
while (true) {
// Some long-running operation
}
});
longRunningThread.start();
}
}
Solution
Always ensure that long-running threads clean up resources they hold references to and terminate gracefully.
public void stopThread() {
if (longRunningThread != null && longRunningThread.isAlive()) {
longRunningThread.interrupt(); // Signal the thread to stop
}
}
4. Overusing Inner Classes
Anonymous inner classes hold a reference to their enclosing class. If an inner class is registered as a listener within a long-lived object, it will prevent the enclosing class from being garbage-collected.
Example
public class OuterClass {
private String instanceVariable = "Keep me alive";
public void createInnerClass() {
new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println(instanceVariable);
}
};
}
}
Solution
Use static inner classes to avoid unintended references to enclosing instances.
public static class StaticListener implements ActionListener {
private String data;
public StaticListener(String data) {
this.data = data; // Maintain a reference as needed
}
public void actionPerformed(ActionEvent e) {
System.out.println(data);
}
}
5. Inefficient Data Caching
Many implementations use memory for caching to improve performance, but if not managed correctly, this can lead to memory leaks.
Example
import java.util.*;
public class CacheExample {
private Map<String, Object> cache = new HashMap<>();
public void cacheData(String key, Object value) {
cache.put(key, value); // Cached data never removed
}
}
Solution
Regularly purge the cache based on application requirements or use a library that manages cache size and eviction policies (like Caffeine or Guava).
import com.github.benmanes.caffeine.cache.CacheBuilder;
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.TimeUnit;
public class CaffeineCacheExample {
private com.github.benmanes.caffeine.cache.Cache<String, Object> cache;
public CaffeineCacheExample() {
cache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(100)
.build();
}
public void cacheData(String key, Object value) {
cache.put(key, value);
}
}
Final Thoughts
While Java provides features such as automatic garbage collection, developers must still be vigilant about memory management practices. Awareness of common memory leak pitfalls and following best practices can help ensure that applications perform efficiently, maximizing both resources and user experience.
For more in-depth learning about Java memory management, check out the official Oracle documentation. Additionally, exploring memory management frameworks like Apache Commons can offer further tools to help optimize your Java applications.
By adhering to these guidelines and learning from potential pitfalls, Java developers can create applications that are not only effective but also robustly resource-efficient.
Checkout our other articles