Securing Your JAX-RS API: Custom Header Authentication Steps
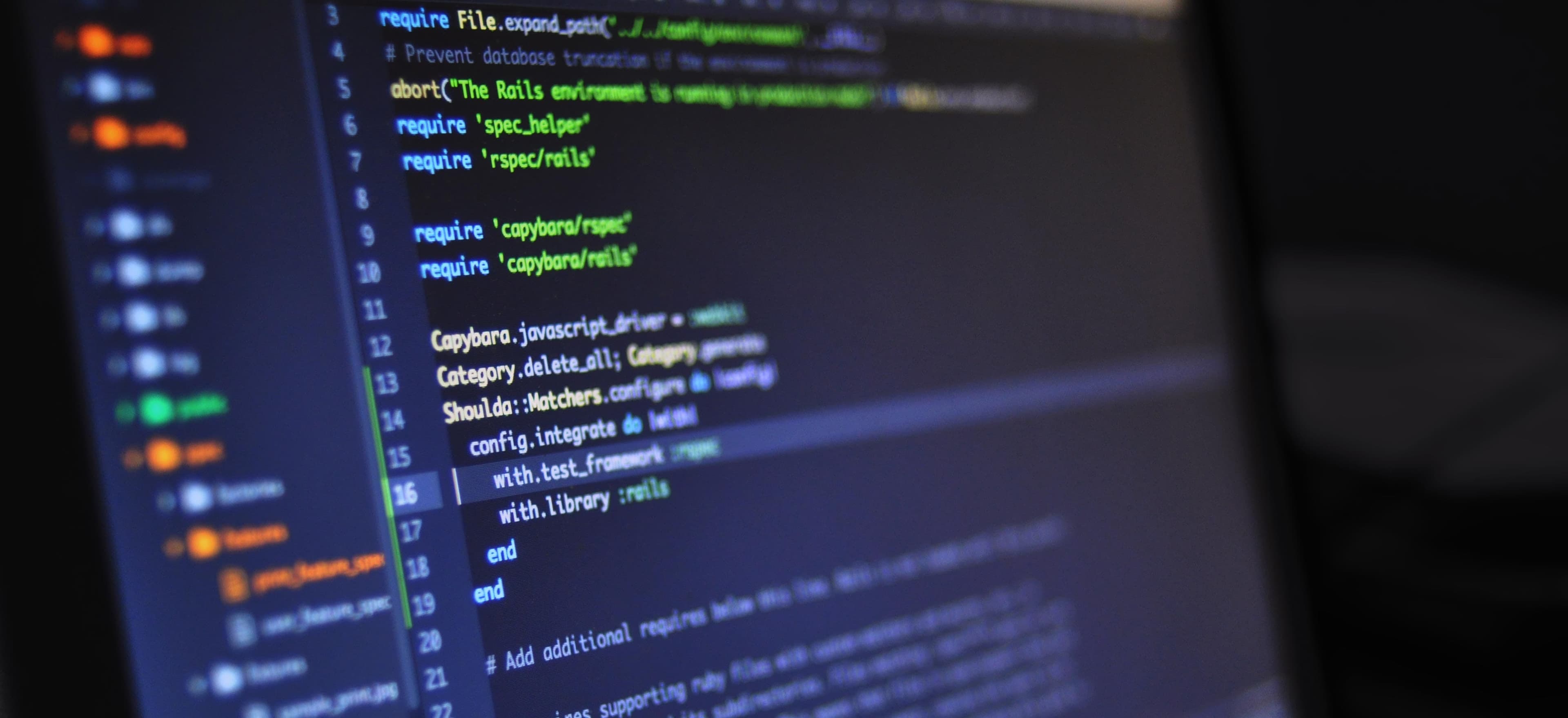
- Published on
Securing Your JAX-RS API: Custom Header Authentication Steps
In today's digital landscape, API security has become a paramount concern. As developers, our applications need to protect sensitive data while ensuring only authorized users can access specific resources. One effective way to bolster security measures for your JAX-RS API is through custom header authentication. This article will guide you step by step on implementing custom header authentication in your JAX-RS application while adhering to best practices.
What is JAX-RS?
JAX-RS (Java API for RESTful Web Services) is a powerful API developed for building RESTful services in Java. It simplifies the process of developing web services by providing easy-to-use annotations, thus allowing developers to focus more on the business logic than technicalities.
Why Use Custom Header Authentication?
While there are standard approaches to API authentication like OAuth2 and Basic Authentication, there are scenarios wherein a custom approach suits better:
- Simplicity: When you need to provide a straightforward solution for a smaller application.
- Flexibility: Custom headers allow you to tailor the authentication scheme for your specific needs.
- Costs: Reduces reliance on third-party identity providers which might incur costs.
Setting Up Your JAX-RS API
First, ensure you have your JAX-RS environment set up. Below is a basic configuration using Maven. For more details on setting up JAX-RS, you can refer to this guide.
Maven Dependencies
To kick-off, add the following Maven dependencies in your pom.xml
file:
<dependency>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
<version>2.1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.34</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.inject</groupId>
<artifactId>jersey-hk2</artifactId>
<version>2.34</version>
</dependency>
Now let's create a simple REST service where we will implement our custom header authentication.
Create a Simple REST Resource
Here's an example of a simple JAX-RS resource:
@Path("/api")
public class MyResource {
@GET
@Path("/data")
@Produces(MediaType.APPLICATION_JSON)
public Response getData() {
// Simple data retrieval logic
return Response.ok("{\"message\":\"Hello World!\"}").build();
}
}
This code snippet creates a basic endpoint that returns a JSON message.
Adding Custom Authentication
To make this API secure, we can use custom headers for authentication. The process generally involves intercepting requests and verifying authentication tokens.
Step 1: Create a Filter
You can create a filter to inspect the incoming requests for the required authentication header.
import javax.ws.rs.container.ContainerRequestContext;
import javax.ws.rs.container.ContainerRequestFilter;
import javax.ws.rs.ext.Provider;
import java.io.IOException;
@Provider
public class AuthFilter implements ContainerRequestFilter {
@Override
public void filter(ContainerRequestContext requestContext) throws IOException {
// Check for the presence of the "X-Auth-Token" header
String authToken = requestContext.getHeaderString("X-Auth-Token");
if (authToken == null || !isValidToken(authToken)) {
// If the token is not present or invalid, respond with 401 Unauthorized
requestContext.abortWith(Response.status(Response.Status.UNAUTHORIZED).build());
}
}
private boolean isValidToken(String token) {
// Here you would add your logic to verify the token
// For instance, checking it against a database or a hardcoded value
return "my-secure-token".equals(token);
}
}
Explanation of the Auth Filter
- @Provider: This annotation allows the filter to be registered automatically.
- filter method: This is where you implement the logic for validating the token from the request's header.
- isValidToken: This helper method does the actual work of validating the token. You can replace the hardcoded token checks with dynamic validation logic as necessary.
Step 2: Register the Filter
To register the AuthFilter
, add it to your Application class:
import org.glassfish.jersey.server.ResourceConfig;
public class MyApplication extends ResourceConfig {
public MyApplication() {
packages("your.package.path"); // Replace with your package
register(AuthFilter.class);
}
}
Testing Custom Header Authentication
Once you have implemented the authentication filter, it's time to test it. We will use a simple HTTP client like Postman or curl.
Effective Testing with cURL
You can use the following command to test your API without the custom header:
curl -X GET http://localhost:8080/api/data
This will result in a 401 Unauthorized response since we haven't provided the required X-Auth-Token
header.
To provide the token:
curl -X GET http://localhost:8080/api/data -H "X-Auth-Token: my-secure-token"
Now you should see a successful response:
{"message": "Hello World!"}
Best Practices for Securing your API
- Password Management: While using tokens, consider one-time tokens or hashed passwords for an added layer of security.
- HTTPS: Always use HTTPS to encrypt the data in transit.
- Limit Token Scope: Tokens should have a specific scope and lifetime to minimize potential for abuse.
- Logging: Implement logging for authentication attempts for audits and tracking suspicious activity.
A Final Look
Implementing custom header authentication for your JAX-RS API is a straightforward and effective method to secure your resources. By filtering incoming requests and validating the tokens, you ensure that only authenticated users can access your endpoints while excitingly maintaining simplicity and control.
Feel free to expand upon this foundation by adopting libraries like JWT for token generation or integrating more complex authentication schemes. For more insights on securing APIs, check out OWASP API Security Best Practices.
Happy coding, and stay secure!
Checkout our other articles