Maximize Java Performance: Tackle JVM Profiling Challenges
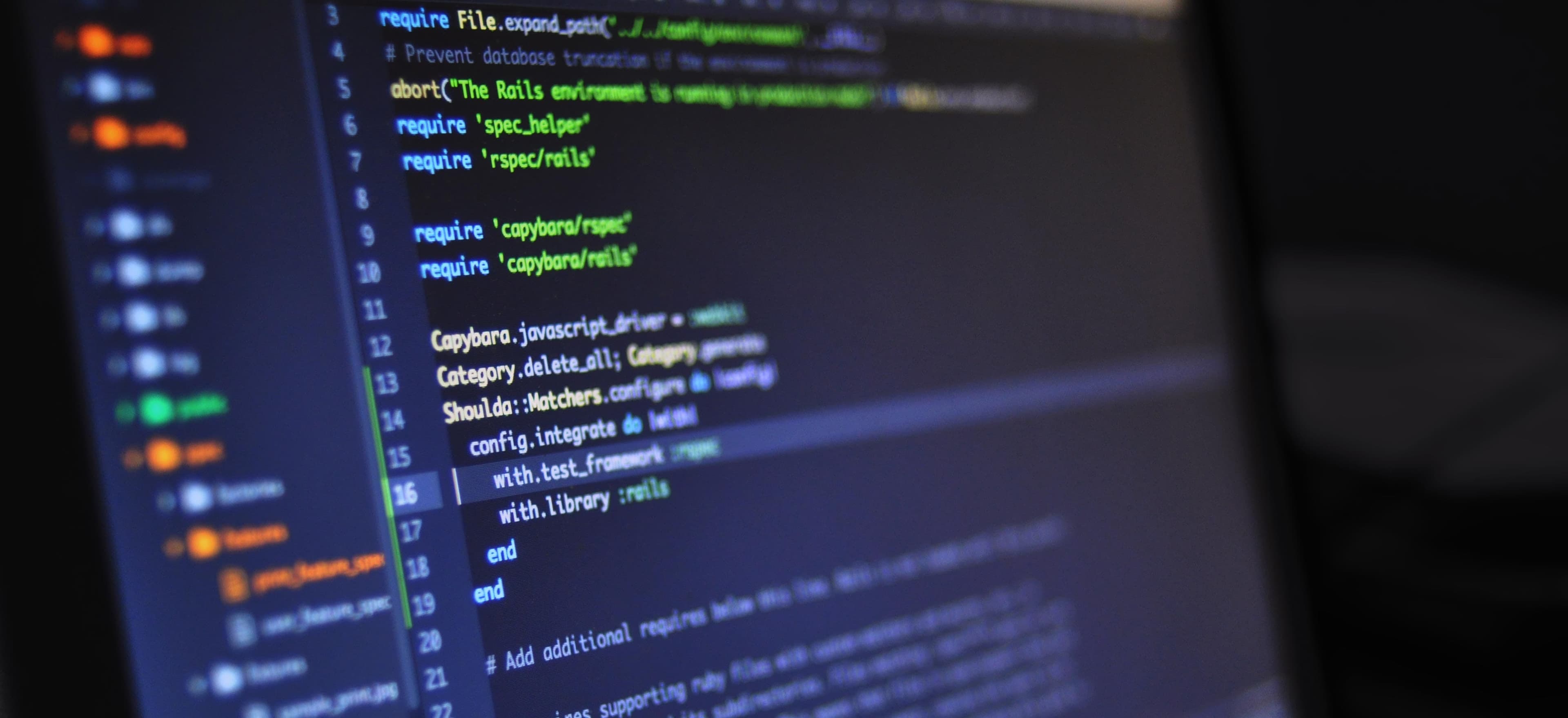
- Published on
Maximize Java Performance: Tackle JVM Profiling Challenges
Java has long reigned as one of the most robust and widely used programming languages in the world. With its platform independence, rich library support, and strong community, many developers turn to Java for high-performance applications. However, performance bottlenecks can arise, leaving developers puzzled. This is where JVM profiling comes into play.
In this blog post, we will delve into the challenges developers face during JVM profiling and how to overcome them. We will also provide practical examples and insightful tips to maximize Java performance.
Understanding JVM Profiling
Before diving into the challenges, let's clarify what JVM profiling is. JVM profiling involves analyzing a Java application's performance to identify inefficiencies. This process utilizes profiling tools that gather data regarding memory usage, CPU usage, and thread activity to optimize the performance of Java applications.
Well-known JVM profilers include:
- Java Mission Control: Provides detailed analysis of application performance and resource consumption.
- VisualVM: A comprehensive tool for monitoring and troubleshooting applications.
- YourKit: A commercial profiler that offers powerful capabilities for analyzing CPU and memory.
Why Profiling Matters
Profiling is essential for several reasons:
- Identifying Bottlenecks: It helps pinpoint slow sections of code.
- Memory Management: Identifies memory leaks and monitors garbage collection (GC).
- Thread Activity: Observes thread contention and synchronization issues.
Each of these aspects is critical for ensuring your application runs efficiently and scales effectively.
Common JVM Profiling Challenges
Despite its significance, developers frequently encounter challenges during JVM profiling. Here, we outline some of these hurdles along with strategies to conquer them.
Challenge 1: High Overhead and Inaccurate Data
Profiling tools often introduce overhead to the application, distorting the performance metrics. This can lead to inaccuracies in understanding how the application performs under normal circumstances.
Solution: Use lightweight profiling during development and revert to comprehensive profiling during stress testing.
Example Code Snippet:
import java.util.stream.IntStream;
public class Calculation {
public static void main(String[] args) {
long startTime = System.nanoTime();
int[] numbers = IntStream.range(1, 1000000).toArray();
int sum = IntStream.of(numbers).sum();
long endTime = System.nanoTime();
System.out.println("Sum: " + sum);
System.out.println("Execution Time: " + (endTime - startTime) + " ns");
}
}
In the above code, using System.nanoTime() allows you to measure execution time without impacting the application significantly. This method gives you a basic understanding of performance without the heavy overhead of a profiler.
Challenge 2: Too Much Data to Analyze
Many profilers generate large amounts of data, making it overwhelming to sift through the information. Key insights can often get lost in this sea of metrics.
Solution: Focus on specific areas of concern first, such as CPU usage or memory consumption, before diving deeper into other metrics.
Example Code Snippet:
public class MemoryTest {
public static void main(String[] args) {
Runtime runtime = Runtime.getRuntime();
runtime.gc(); // Suggest garbage collection
long startMemory = runtime.totalMemory() - runtime.freeMemory();
List<String> list = new ArrayList<>();
for (int i = 0; i < 100000; i++) {
list.add(String.valueOf(i));
}
long endMemory = runtime.totalMemory() - runtime.freeMemory();
System.out.println("Memory Used: " + (endMemory - startMemory) + " bytes");
}
}
This example illustrates how to focus data analysis by measuring memory usage before and after a specific operation. This targeted approach helps in narrowing down your analysis.
Challenge 3: Inconsistent Test Environments
Profiling results can differ significantly between development, staging, and production environments. These inconsistencies can frustrate the profiling process.
Solution: Maintain uniformity in your environments as much as possible. Use containerization through tools like Docker to ensure your application behaves consistently across all environments.
Advanced JVM Profiling Tips
Tip 1: Use the Right Tool for the Right Job
Choosing an appropriate tool for specific profiling tasks can be a game-changer.
- For CPU profiling, Java Mission Control is powerful and provides low overhead.
- For memory leaks, VisualVM can be effective in displaying heap dumps.
Tip 2: Continuously Monitor Performance
Besides periodic profiling, continuous monitoring of performance metrics can help identify issues early. A well-established monitoring system will provide alerts for metrics breaching certain thresholds.
Tip 3: Analyze Garbage Collection
Garbage collection can significantly affect Java applications' performance. Utilize profiling tools to monitor GC pauses and optimize collection methods. Here’s how you can visualize GC metrics:
-XX:+PrintGCDetails -Xloggc:<path-to-gc-log>
Use JVM flags during runtime to generate insights about garbage collection, making it easier to spot issues.
Bringing It All Together
JVM profiling is crucial for maximizing the performance of Java applications. By addressing common challenges, such as overhead, excessive data, and inconsistent environments, developers can gain actionable insights to enhance their applications.
Focus on targeted analysis, choose the right tools, and continuously monitor performance metrics. With these strategies, you'll be well-equipped to tackle JVM profiling challenges and uplift your Java development experience.
For further insights into Java performance tuning, you may check the resources provided by Oracle's official documentation.
Remember, profiling is not a one-time task; it's a continual journey toward optimization. Happy profiling!
Checkout our other articles