Streamlining Cloud Foundry App Manifests with Kotlin DSL
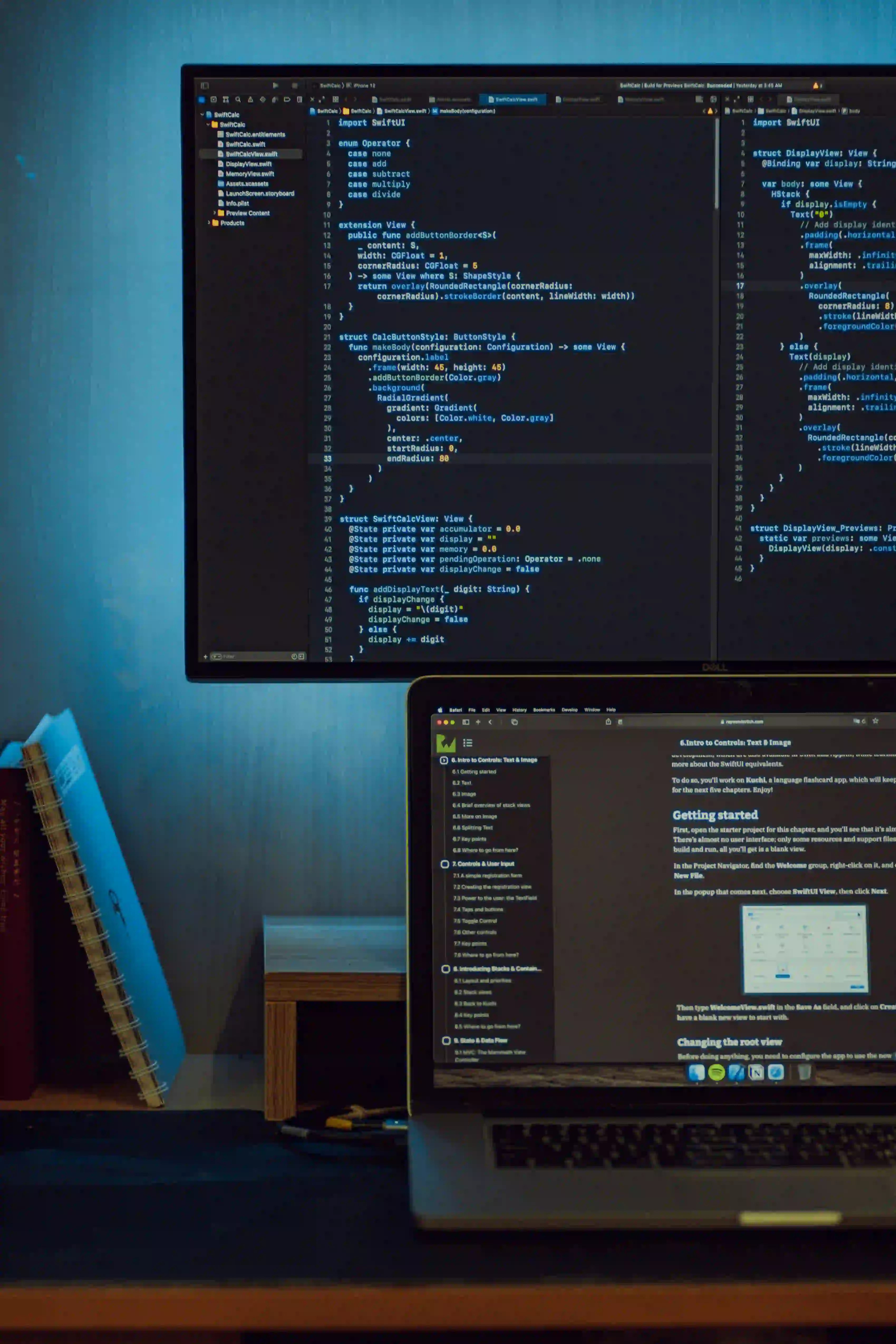
Streamlining Cloud Foundry App Manifests with Kotlin DSL
In today’s era of cloud computing, cloud applications must be efficient and easy to manage. Cloud Foundry, an open-source platform as a service (PaaS), offers developers the opportunity to deploy and run applications with minimal overhead. However, managing application configurations, particularly app manifests, can sometimes be cumbersome. In this blog post, we're going to explore how you can streamline your Cloud Foundry app manifests using Kotlin DSL (Domain-Specific Language).
What is a Cloud Foundry App Manifest?
An app manifest is essentially a YAML file that defines how your application should behave when deployed on Cloud Foundry. It describes all the necessary configuration details, including the application name, memory allocation, instances, buildpack, environment variables, and more. A well-structured manifest ensures a smooth deployment and operational efficiency.
Here's a basic example of an app manifest:
---
applications:
- name: my-app
memory: 512M
instances: 1
buildpack: java_buildpack
env:
SPRING_PROFILES_ACTIVE: production
This YAML configuration might be okay for small projects, but as applications grow in complexity, managing these files in raw YAML can become tedious and error-prone.
Enter Kotlin DSL
Kotlin DSL offers a way to write these configurations in a more concise and idiomatic Kotlin style. With Kotlin DSL, you can take advantage of type safety, autocompletion, and more structured syntax.
Setting Up Kotlin DSL for Cloud Foundry
To streamline your Cloud Foundry app manifests, follow these simple steps to set up Kotlin DSL.
Step 1: Include Kotlin Compiler Dependency
First, you need to add the required dependencies in your build tool. If you are using Gradle, update your build.gradle
file accordingly:
plugins {
id 'org.jetbrains.kotlin.jvm' version '1.7.20'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.jetbrains.kotlin:kotlin-stdlib-jdk8'
}
Step 2: Create Your DSL
You can then create a Kotlin DSL for your manifests. Here's how to define your app manifests in a more structured way.
import org.jetbrains.kotlin.gradle.dsl.KotlinManifest
fun kotlinManifest(block: KotlinManifest.() -> Unit): KotlinManifest {
return KotlinManifest().apply(block)
}
val appManifest = kotlinManifest {
application {
name = "my-app"
memory = "512M"
instances = 1
buildpack = "java_buildpack"
env {
put("SPRING_PROFILES_ACTIVE", "production")
}
}
}
Why Use Kotlin DSL for Cloud Foundry Manifests?
1. Improved Readability: Kotlin’s syntax is concise, making the manifest much easier to read and maintain. By using Kotlin DSL, you gain clarity over the structure of your configurations.
2. Type Safety: When you define your app manifest in Kotlin, you eliminate the risk of typos commonly found in YAML files. For example, you can catch issues related to type mismatches at compile-time rather than runtime.
3. IDE Support: Modern IDEs provide excellent support for Kotlin development, including code completion and refactoring tools. This greatly enhances developer productivity and reduces errors.
4. Reusability: You can create reusable components to define common settings. For instance, if you deploy multiple microservices that share similar configurations, you can create a base manifest and extend it.
Finalizing the App Manifest
With the DSL setup defined, you might need the ability to convert it into a YAML format for Cloud Foundry deployment. For this, you can use libraries like Klaxon
for converting your data structures into JSON and YAML formats.
Sample Code to Convert to YAML:
import com.fasterxml.jackson.dataformat.yaml.YAMLMapper
fun toYaml(appManifest: KotlinManifest): String {
val yamlMapper = YAMLMapper()
return yamlMapper.writeValueAsString(appManifest)
}
Deploying the App Manifest
After generating your YAML output, you can deploy your app on Cloud Foundry either through the CLI or using a CI/CD pipeline. A simple command to deploy it through the CLI looks like this:
cf push
This command reads the manifest.yml
and orchestrates the deployment process.
My Closing Thoughts on the Matter
In conclusion, shifting from traditional YAML manifests to Kotlin DSL for handling Cloud Foundry app manifests can significantly simplify your development workflow. With enhanced readability, type safety, IDE support, and reusability, Kotlin DSL serves as an excellent choice for managing your configurations effectively.
For further reading on YAML vs. Kotlin DSL and cloud application development, you may want to check out the following resources:
- Cloud Foundry Documentation
- Kotlin DSL for Gradle
By adopting Kotlin DSL, you’ll not only improve the manageability of your app manifests but also elevate your overall cloud application development experience. Keep coding, keep improving!