Common JAXB Issues with Java Util Map: How to Fix Them
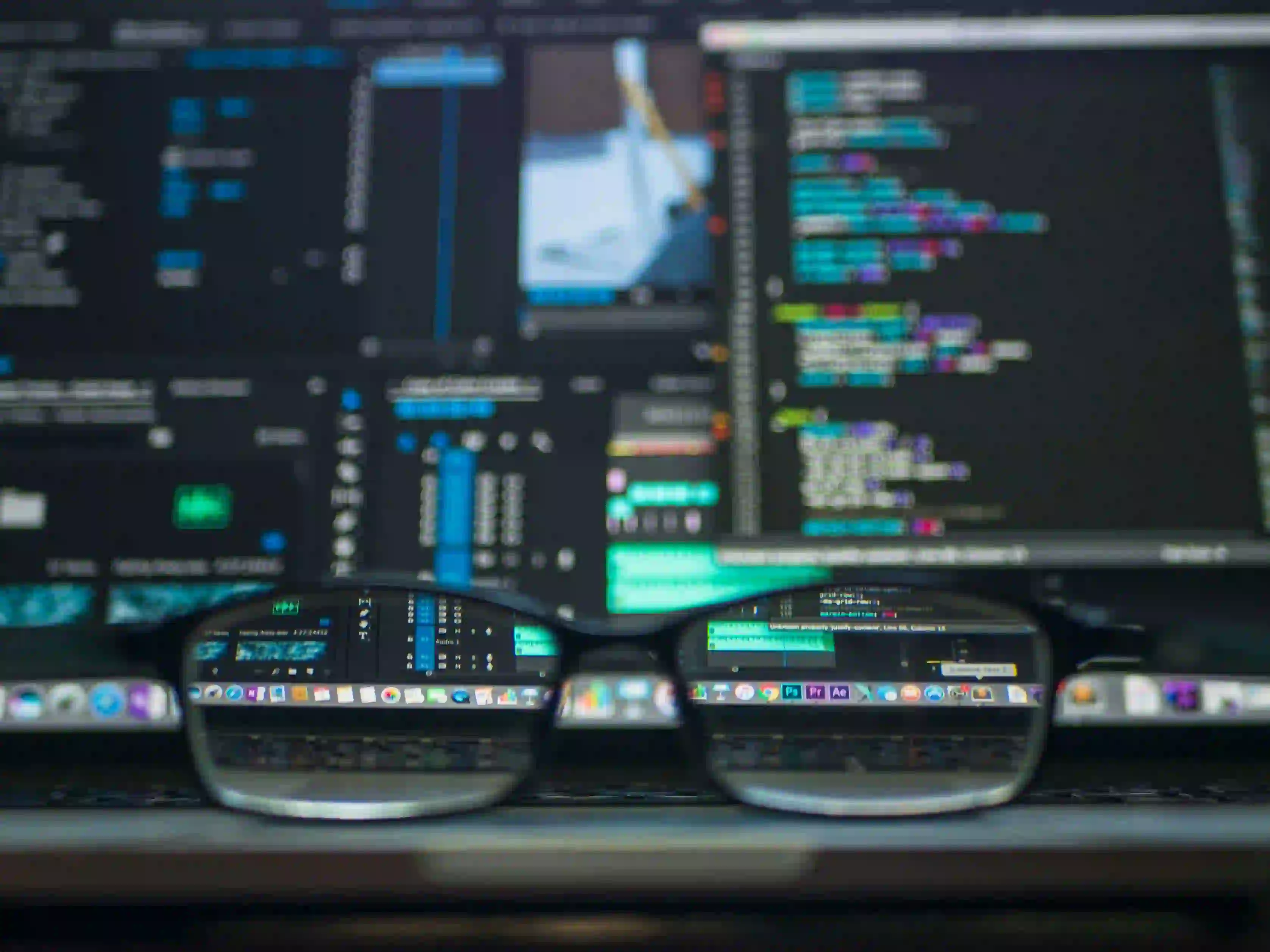
Common JAXB Issues with Java Util Map: How to Fix Them
Java Architecture for XML Binding (JAXB) is a powerful tool that converts Java objects into XML and vice versa, facilitating the interaction between XML and Java applications. However, when using the java.util.Map
interface within JAXB, developers often encounter several common issues. In this blog post, we will delve into these issues, explore their causes, and provide solutions to fix them.
Understanding JAXB and Its Context
JAXB is designed to make it easy to transform Java objects into XML in a straightforward manner. It is especially useful for developers working with web services and data exchange formats. It allows them to focus on business logic rather than on the details of XML serialization and deserialization.
While JAXB works well with Java bean conventions, handling Map
objects can lead to unexpected results if not approached with care. JAXB may have difficulty interpreting Map
due to its generic and dynamic nature.
Common JAXB Issues with java.util.Map
Serialization Problems
One of the primary issues developers encounter is with JAXB's serialization process when working with generic collections such as Map
. By default, JAXB expects to work with objects that have an explicit XML representation. Here's a brief illustration of this problem.
Example Code Snippet: Problematic Serialization
import javax.xml.bind.annotation.XmlRootElement;
import java.util.HashMap;
import java.util.Map;
@XmlRootElement
public class User {
private String name;
private Map<String, String> attributes;
// Getters and Setters
}
In this example, the attributes
Map might not be serialized into XML as expected, resulting in an improper XML structure or no representation at all.
Deserialization Issues
Deserialization is another aspect where Map
types can cause trouble. JAXB prefers a specific known type for each property. Therefore, when it attempts to deserialize XML back into a Map
, it may not know how to handle the diverse value types often stored in Map
.
Example Code Snippet: Deserialization Issues
Suppose an XML structure is provided as follows:
<user>
<name>John Doe</name>
<attributes>
<entry>
<key>age</key>
<value>30</value>
</entry>
<entry>
<key>gender</key>
<value>male</value>
</entry>
</attributes>
</user>
This XML representation assumes the existence of an entry
structure within the attributes mapping, which JAXB does not interpret correctly without proper annotations.
Solutions to Fix JAXB Issues with Map
Using an Adaptor
A common solution to the above challenges is to use an adapter to convert between the Map
and a structure JAXB can understand. The adapter takes care of the serialization and deserialization process.
Example Code Snippet: Using an Adapter
import javax.xml.bind.annotation.adapters.XmlAdapter;
import java.util.HashMap;
import java.util.Map;
public class MapAdapter extends XmlAdapter<Entry[], Map<String, String>> {
@Override
public Map<String, String> unmarshal(Entry[] entries) {
Map<String, String> map = new HashMap<>();
for (Entry entry : entries) {
map.put(entry.key, entry.value);
}
return map;
}
@Override
public Entry[] marshal(Map<String, String> map) {
Entry[] entries = new Entry[map.size()];
int i = 0;
for (Map.Entry<String, String> entry : map.entrySet()) {
entries[i++] = new Entry(entry.getKey(), entry.getValue());
}
return entries;
}
}
class Entry {
public String key;
public String value;
public Entry() {
}
public Entry(String key, String value) {
this.key = key;
this.value = value;
}
}
In this example, MapAdapter
converts between a Map<String, String>
and an array of Entry
objects for JAXB processing. Each entry contains a key-value pair representing the original map.
Incorporating the Adapter in Your User Class
With the adapter implemented, the next step is to incorporate it into your JAXB-annotated class.
Example Code Snippet: Annotating with the Adapter
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import java.util.HashMap;
import java.util.Map;
@XmlRootElement
public class User {
private String name;
@XmlJavaTypeAdapter(MapAdapter.class)
private Map<String, String> attributes = new HashMap<>();
// Getters and Setters
}
Why This Works
Using an adapter allows JAXB to understand how to handle the Map
structure while keeping the original data types intact. It offers a clean separation of concerns. The adapter transforms the Map
into an array of key-value pairs during serialization and provides the mechanism to reconstruct the Map
during deserialization.
Additional Tips for Working with JAXB and Map
-
Use Concrete Implementations: Itβs often best to use concrete implementations like
HashMap
instead of the interface typeMap
when defining fields since JAXB handles concrete classes better. -
Handle Null Values: JAXB does not serialize null values by default. Make sure to either handle or filter any null values within your adapter to prevent unexpected behavior.
-
Testing Your Adapters: Always test your adapters independently to ensure they convert correctly between
Map
and the XML representation. This can prevent bugs that are hard to trace in larger projects.
Lessons Learned
Using JAXB with java.util.Map
can seem daunting at first due to its complex nature, but with the right approach, it becomes manageable. By employing an adapter, developers can ensure a smooth serialization and deserialization process.
For further reading on JAXB and its various aspects, consider checking out the official JAXB documentation and Java Tutorials for comprehensive insights.
By addressing common issues, implementing best practices, and utilizing adapters effectively, you can seamlessly integrate maps into your JAXB-based applications, saving you time and enhancing your application's efficiency.
Happy coding!