Common JMX Configuration Issues in Spring Applications
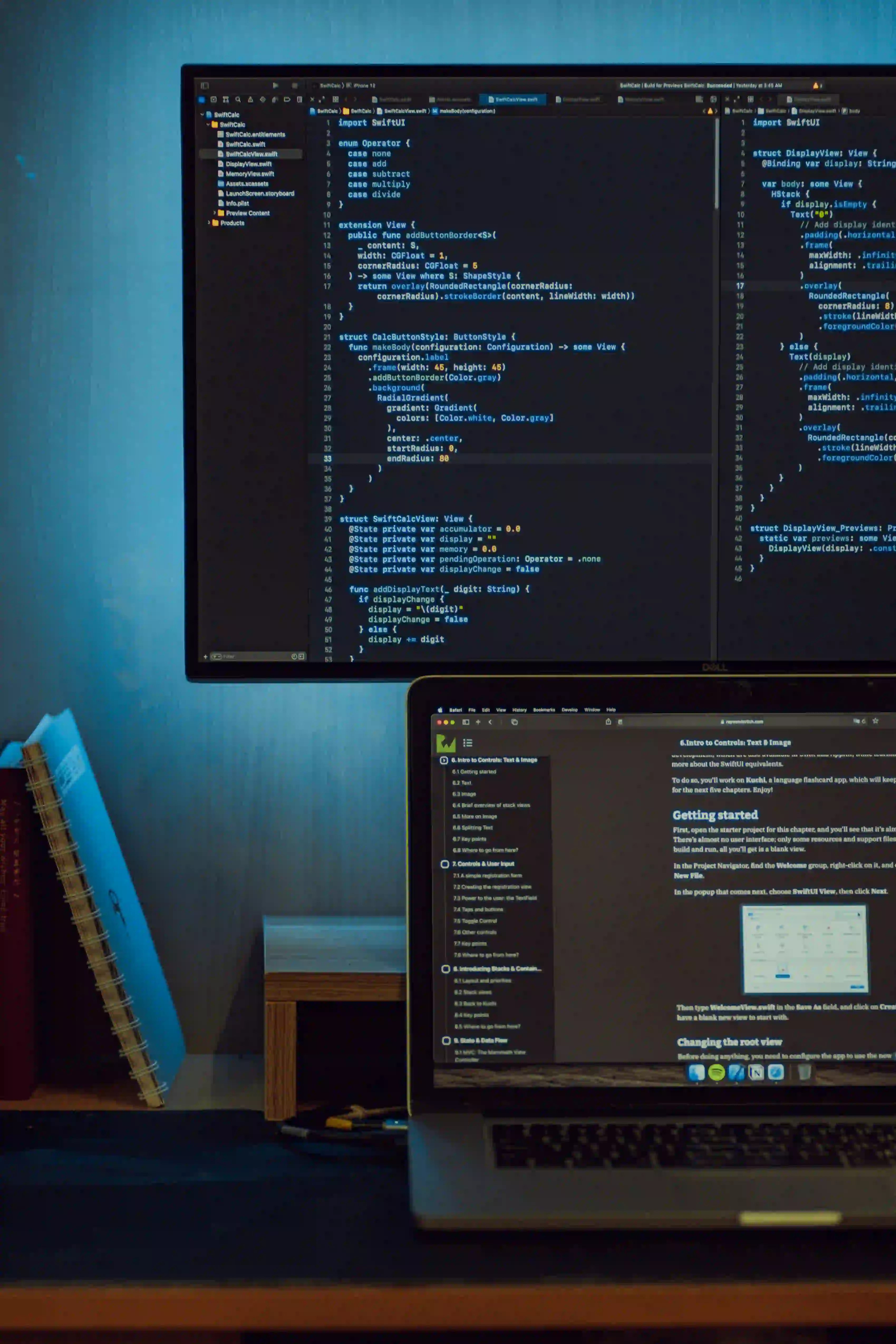
Common JMX Configuration Issues in Spring Applications
Java Management Extensions (JMX) provide a powerful way to manage and monitor Java applications. When utilizing JMX in Spring applications, numerous configuration issues can arise that may lead to application performance degradation or other unexpected behaviors. In this blog post, we will explore common JMX configuration problems and how to resolve them effectively.
Understanding JMX
JMX is a technology that allows for the management and monitoring of resources in a Java environment. This can include applications, system resources, and even devices. With JMX, you can create managed bean classes (MBeans) that expose attributes and operations for various components within your application.
The Role of Spring in JMX
Spring Framework offers comprehensive support to integrate JMX. You can effortlessly expose beans as MBeans using the Spring JMX support, which enables you to manage your Spring beans dynamically at runtime. However, whenever there’s an integration of two powerful toolsets like Spring and JMX, configuration issues can arise.
Common JMX Configuration Issues
1. Improper Syntax in Configuration
One of the most common mistakes is a simple syntax error in the JMX configuration. This can happen within XML configuration files or annotation-based configurations in Spring.
XML Example:
Here’s an example of how to declare a JMX bean in an XML configuration:
<bean id="myMBean" class="com.example.MyMBean" >
<property name="service" ref="myService"/>
</bean>
<jmx:mbean name="com.example:type=MyMBean" ref="myMBean" />
Why: In the configuration above, ensure that the package names, class names, and properties are correctly set. A minor typo can prevent the application from loading the MBean.
2. MBean Registration Issues
MBeans must be registered correctly with the MBean server, or they won’t be accessible for monitoring and management.
Java Example:
If you are using Java to register MBeans:
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
MyMBean myMBean = new MyMBean();
ObjectName objectName = new ObjectName("com.example:type=MyMBean");
mbs.registerMBean(myMBean, objectName);
Why: Make sure that the ObjectName
is defined correctly as it uniquely identifies the MBean. Errors in this can lead to registration failure and subsequently make MBeans inaccessible.
3. Security Configuration
JMX allows remote management, which can pose security risks if not properly configured. By default, JMX does not have any security, leading to open access.
To Secure Your JMX:
You can configure SSL and authentication:
System.setProperty("com.sun.management.jmxremote", "true");
System.setProperty("com.sun.management.jmxremote.port", "9999");
System.setProperty("com.sun.management.jmxremote.authenticate", "true");
System.setProperty("com.sun.management.jmxremote.password.file", "jmxremote.password");
System.setProperty("com.sun.management.jmxremote.access.file", "jmxremote.access");
Why: Security risks can be deadly. Always implement authentication and preferably use SSL to protect data in transit between the JMX client and server.
4. Spring Boot JMX Properties
In Spring Boot applications, you can directly configure JMX properties in application.properties
or application.yml
. However, misconfiguration can lead to issues.
Example in application.properties:
spring.jmx.enabled=true
spring.jmx.default-domain=my.application
spring.jmx.locator.enabled=true
Why: Ensure that JMX is enabled, and check your default domain and locator settings. If these are incorrect, you may not access the MBeans as intended.
5. Exposing Non-Spring Beans
Sometimes, you may want to expose non-Spring managed beans as MBeans. This can lead to misunderstanding, as JMX will only work seamlessly with Spring-managed beans.
Example:
If you have a custom service:
public class NonSpringService {
public void performAction() {
// Action logic here
}
}
You must wrap it in a Spring-managed bean to expose it as an MBean:
@Bean
public NonSpringService nonSpringService() {
return new NonSpringService();
}
Why: Only Spring beans will be managed by the Spring context. Therefore, you must ensure that all MBeans are Spring beans for proper lifecycle management.
Best Practices for JMX Configuration
-
Consistent Naming Conventions: Use consistent naming for your MBeans to avoid confusion and facilitate easier access.
-
Define Clear Attributes and Operations: Clearly define which attributes and operations should be exposed in your MBeans. This keeps the MBean interface clean and prevents overly complex management interfaces.
-
Monitor Performance Impacts: Once JMX is configured, monitor the overhead introduced by additional management interfaces. Use performance testing especially in high-load applications.
-
Documentation: Document your JMX configuration carefully. Include details about how to access the MBeans, what attributes to monitor, and any security configurations.
-
Testing: Test your JMX configurations frequently. It’s essential that they work as expected, especially in critical production deployment scenarios.
In Conclusion, Here is What Matters
While JMX is an excellent tool for managing and monitoring Java applications, configuring it properly is crucial to avoid pitfalls. Common issues such as improper syntax, registration problems, and security concerns can hinder effective monitoring.
By understanding and addressing these potential problems, you can ensure that your Spring applications are not only configurable but also manageable. For detailed configuration and best practices with JMX in Spring, check out the Spring Framework Reference Documentation.
If you’re interested in further reading, explore JMX Basics, which provides a foundational understanding of JMX.
Implementing proper JMX configurations is a reliable way to monitor your Spring applications in real-time while ensuring high performance and security standards.