Mastering Neo4j: Taming Multiple Value Collections
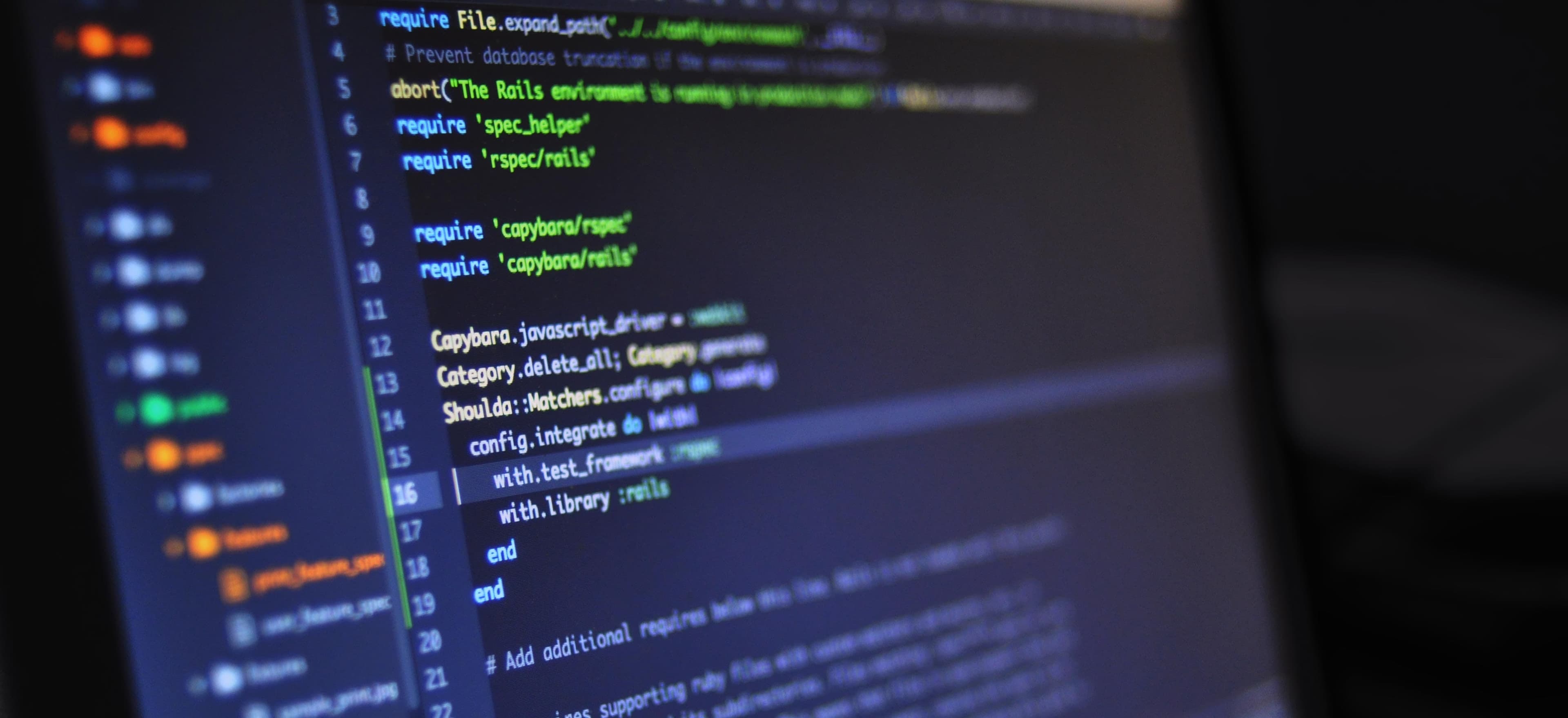
- Published on
Mastering Neo4j: Taming Multiple Value Collections
In the world of graph databases, Neo4j stands as a beacon of power and flexibility. Unlike traditional relational databases, Neo4j thrives on relationships, making it an ideal choice for complex data structures. However, as we delve deeper into Neo4j, we often encounter the challenge of managing collections of multiple values. In this post, we'll explore how to effectively handle multiple value collections using Neo4j, ensuring that you leverage the full power of this remarkable database.
Understanding Neo4j and Graph Databases
Before we jump into handling multiple value collections, it's essential to understand what Neo4j is and how it relates to graph databases. Neo4j is a native graph database that stores data in graph structures. Nodes represent entities, and relationships connect these nodes, allowing for efficient querying of connected data. For more foundational insights, you can visit the official Neo4j documentation.
Setting the Stage: Creating Your First Graph
To better illustrate how to manage multiple value collections, let’s start by setting up a simple graph. Imagine we are building a movie database. We’ll represent actors and their movies as nodes and create relationships that define who acted in which film.
Step 1: Creating a Sample Graph
CREATE (a:Actor {name: 'Keanu Reeves'}),
(a)-[:ACTED_IN]->(m:Movie {title: 'The Matrix'}),
(a)-[:ACTED_IN]->(m2:Movie {title: 'John Wick'}),
(b:Actor {name: 'Carrie-Anne Moss'}),
(b)-[:ACTED_IN]->(m),
(b)-[:ACTED_IN]->(m2);
In the code above, we create two actors, Keanu Reeves and Carrie-Anne Moss, and relate them to the movies they’ve acted in. Each actor is linked to multiple movies through the ACTED_IN
relationship.
Why is this important? Handling relationships in Neo4j allows you to efficiently traverse and query interconnected data.
Taming Multiple Value Collections
What Are Multiple Value Collections?
In Neo4j, a multiple value collection can be represented as properties that hold lists of values. For instance, an actor might have a list of genres they typically act in or awards they have won. Maintaining these lists as properties of a node helps streamline queries and ensures data integrity.
Example: Storing Awards as a Collection
Let's see how to store multiple awards for each actor in our graph. This will require the use of an array.
MATCH (a:Actor {name: 'Keanu Reeves'})
SET a.awards = ['MTV Movie Awards', 'Teen Choice Awards', 'Saturn Award'];
Here, we used the SET
command to assign an array of awards to Keanu Reeves. This approach is not only concise but allows easy retrieval of multiple related values.
Retrieving Data from Collections
Now, let's say we want to retrieve all actors and their awards. The following query makes this straightforward:
MATCH (a:Actor)
RETURN a.name AS Actor, a.awards AS Awards;
This will output a table listing each actor alongside their respective awards. The direct nature of the query exemplifies the power of Neo4j’s data retrieval capabilities, especially for complex, interconnected datasets.
Creating Indexes for Performance
As your dataset grows, querying on multiple value collections can become slow. This is where indexing comes into play. Indexes in Neo4j can drastically improve query performance, especially for properties that are queried frequently.
Example: Creating an Index on Awards
While Neo4j does not support indexing specifically for array properties, you can create indexes on individual properties to improve retrieval times. Let’s assume we want to create an index on the name
property of actors:
CREATE INDEX ON :Actor(name);
Now, when querying for an actor by name, Neo4j can utilize the index for faster lookups. Remember, indexes are a key aspect of any effective database strategy, telling Neo4j where to look first when processing queries.
Useful Querying Techniques
As we're dealing with collections, Neo4j provides functions that can help manage and retrieve these values effectively. For example:
Filtering Based on Collections
Suppose we want to find actors who have won a specific award, like 'MTV Movie Awards'. Here’s how to do that:
MATCH (a:Actor)
WHERE 'MTV Movie Awards' IN a.awards
RETURN a.name AS Actor;
This IN
clause filters actors effectively, allowing us to focus on those who meet our conditional requirements. Such filtering capabilities are essential when dealing with multiple value collections.
Updating Multiple Value Collections
If an actor wins a new award, we need a way to update their awards collection. Using the +
operator allows us to efficiently append new values to array properties.
MATCH (a:Actor {name: 'Keanu Reeves'})
SET a.awards = a.awards + ['Oscar']
RETURN a.awards AS UpdatedAwards;
Here, we dynamically add 'Oscar' to Keanu Reeves's list of awards while retaining the previous entries. This preserves his achievements without the need for cumbersome updates.
Handling Null Values in Collections
When working with collections, null values can occasionally present challenges, particularly if not properly managed. It’s vital to ensure that your application logic accounts for cases where an actor may not have any awards.
Example: Safeguarding Against Nulls
Before attempting to access awards, you can implement a check:
MATCH (a:Actor)
RETURN a.name AS Actor,
CASE WHEN a.awards IS NULL THEN 'No awards' ELSE a.awards END AS Awards;
This CASE
statement allows for graceful handling of null values, ensuring that your data display remains clean and informative.
A Final Look
Mastering the nuances of Neo4j, particularly when it comes to managing multiple value collections, is a valuable skill for any data professional. From understanding how to store and retrieve collections to indexing and handling nulls, these techniques enhance the robustness of your databases.
For further exploration, consider diving deeper into the official Neo4j developer guides. With practice, you will unlock the true power of Neo4j, effectively managing complex relationships and vast data landscapes.
Key Takeaways
- Neo4j excels in representing and querying interconnected data.
- Use array properties to manage multiple value collections in nodes.
- Indexing can significantly enhance query performance.
- Implementing checks for null values ensures data integrity and robustness.
By applying the concepts discussed in this blog, you are set to tame your data collections within Neo4j, making your graph database efforts both efficient and fruitful. Happy graphing!