Common Java Annotation Pitfalls and How to Avoid Them
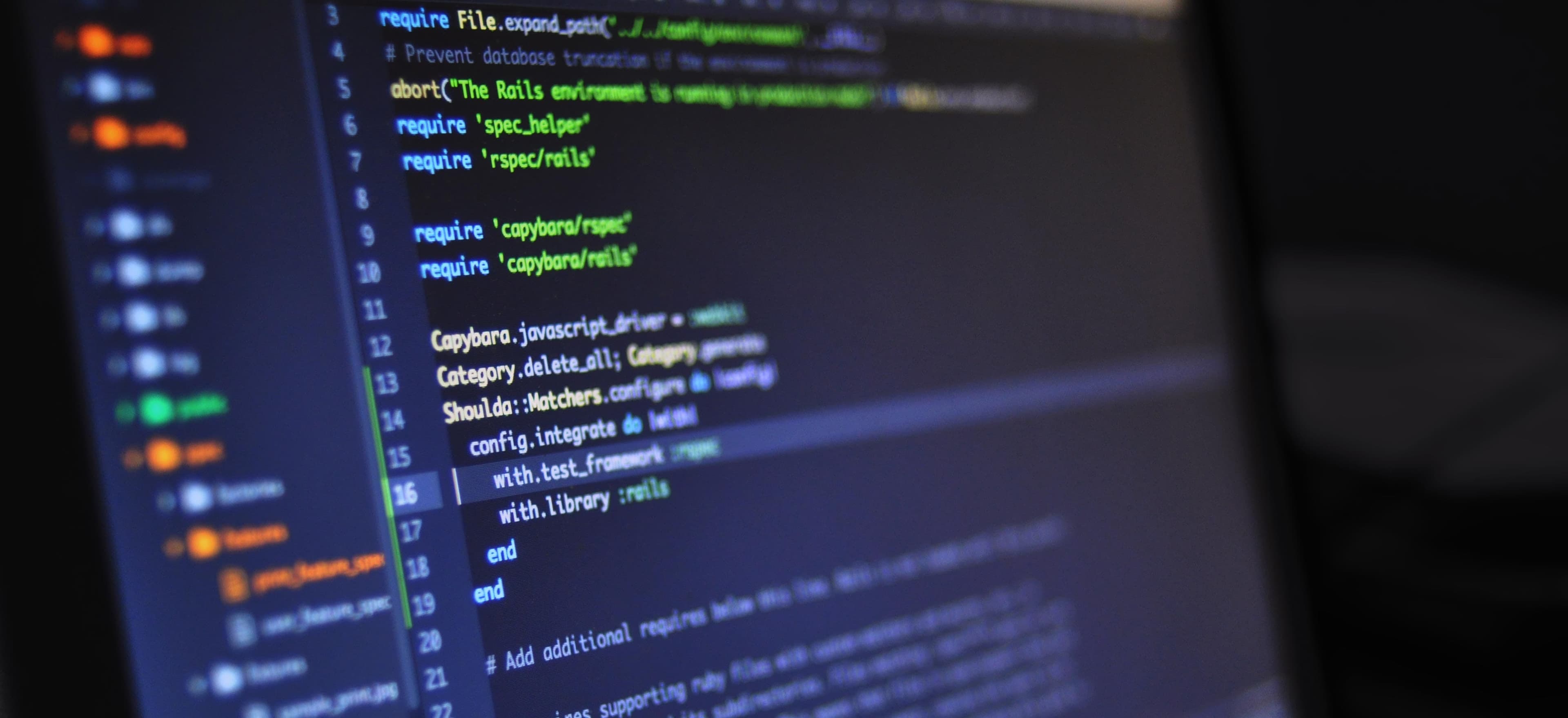
- Published on
Common Java Annotation Pitfalls and How to Avoid Them
Java annotations are a powerful feature that allows developers to add metadata to code elements such as classes, methods, and fields. They play a crucial role in frameworks like Spring and Hibernate, providing necessary information to libraries and tools. However, despite their advantages, annotations can lead to confusion and bugs when misused. In this blog post, we will explore common pitfalls associated with Java annotations and provide strategies to avoid them.
Understanding Java Annotations
Before diving into the pitfalls, let's briefly discuss what annotations are. Annotations in Java are a form of metadata, introduced in Java 5. They do not change the action of the annotated element but can provide information for the compiler or runtime processing. Here’s a simple example:
import java.lang.annotation.*;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface MyCustomAnnotation {
String value();
}
In this snippet:
@Retention(RetentionPolicy.RUNTIME)
indicates that the annotation will be available at runtime.@Target(ElementType.METHOD)
specifies that this annotation can only be applied to methods.value()
is an element for passing additional information.
Understanding how these components work is essential to avoid common pitfalls.
Pitfall 1: Overusing Annotations
Why It’s a Problem
Java annotations should be used to provide clarity and essential information, not to clutter your code. Overusing annotations can lead to a situation where the added complexity obscures the code's intent, making it harder for others (or yourself) to understand what's going on later.
How to Avoid
-
Annotate for a Purpose: Use annotations only when they provide valuable insights or functionality. For instance, don’t annotate every method simply to indicate that it is part of your API.
-
Document Annotations: Ensure that every annotation used has a clear purpose documented in your project’s guidelines. This practice will help your team understand the conventions and avoid unnecessary annotations.
Here’s a quick example:
public class UserService {
@Deprecated
public void oldMethod() {
// This method is deprecated and should no longer be used.
}
public void newMethod() {
// Use this method instead.
}
}
In this case, the @Deprecated
annotation makes it clear that oldMethod()
should not be used, ensuring the developers are directed to newMethod()
.
Pitfall 2: Misunderstanding Retention Policies
Why It’s a Problem
Java provides three retention policies for annotations: SOURCE
, CLASS
, and RUNTIME
. Misunderstanding these can lead to annotations being ignored or misapplied.
How to Avoid
-
Choose the Right Policy: Ensure you're using the correct retention policy for your annotation’s intended use. If the annotation is meant to influence runtime behavior, it should be retained at runtime.
-
Testing: Decide your annotations' retention policies during the design phase and test them thoroughly to ensure they're processed as expected.
Example of a runtime annotation:
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface Transactional {
}
With this setup, the @Transactional
annotation is available at runtime, which is crucial for frameworks like Spring, which use reflection to process annotations.
Pitfall 3: Confusing Annotation Targets
Why It’s a Problem
Annotations can be applied to various program elements like classes, fields, methods, and parameters. Misapplying these can create unexpected behavior or runtime errors.
How to Avoid
-
Use @Target Wisely: When creating custom annotations, always specify an appropriate target using the
@Target
annotation. -
Check the JavaDocs: Consult the official documentation or the JavaDocs for existing annotations before applying them to ensure you are using them in the right context.
Here’s an example:
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
public @interface Service {
}
The above custom annotation can only be applied to classes due to the ElementType.TYPE
specification.
Pitfall 4: Ignoring Annotation Processing
Why It’s a Problem
Annotations can be processed using tools such as Reflection or Annotation Processors. Ignoring this can lead to missed validations or configurations.
How to Avoid
-
Learn About Processing: Familiarize yourself with how annotations are processed at both compile-time and runtime.
-
Utilize Reflection Cautiously: When using reflection, remember that it can make your code less efficient. Use it only when necessary.
Here’s an example of using reflection to execute methods annotated with a custom annotation:
import java.lang.reflect.Method;
public class AnnotationProcessor {
public void processAnnotations(Object obj) {
for (Method method : obj.getClass().getDeclaredMethods()) {
if (method.isAnnotationPresent(MyCustomAnnotation.class)) {
MyCustomAnnotation annotation = method.getAnnotation(MyCustomAnnotation.class);
System.out.println("Processing method: " + method.getName() + " with value: " + annotation.value());
}
}
}
}
This example shows how to dynamically find and process annotated methods in a class at runtime. It demonstrates the power of annotations when used correctly.
Pitfall 5: Failing to Leverage Existing Frameworks
Why It’s a Problem
Many popular frameworks provide their annotations that are well-tested and designed for specific functions. Creating custom annotations for functionality already provided can lead to unnecessary complexity and maintenance overhead.
How to Avoid
- Explore Available Libraries: Before creating a custom annotation, investigate if there's an existing one that meets your needs—like using Spring’s annotations for dependency injection.
For example, instead of creating your own annotation for service components, you can leverage Spring's:
import org.springframework.stereotype.Service;
@Service
public class UserService {
// Your business logic
}
This usage streamlines your code and relies on a robust, widely-used framework.
Closing Remarks
Java annotations are powerful tools when applied correctly, but common pitfalls can arise from misunderstandings and misuse. Awareness of how to use annotations effectively can greatly enhance your Java code's clarity and maintainability.
To summarize:
- Use annotations selectively and purposefully.
- Understand retention policies and targets.
- Process annotations carefully for desired outcomes.
- Leverage existing frameworks to simplify your codebase.
By adhering to these guidelines, you can avoid the typical pitfalls of Java annotations while harnessing their potential to create robust, clean, and maintainable code.
For more about Java annotations and their application, check out the official Java documentation or explore Spring's annotation capabilities.
By embracing the rationale behind proper annotation usage, you will not only improve your coding skills but also contribute positively to your team's productivity and the overall quality of your projects. Happy coding!