Overcoming Common Pitfalls in Spring XD Data Ingestion
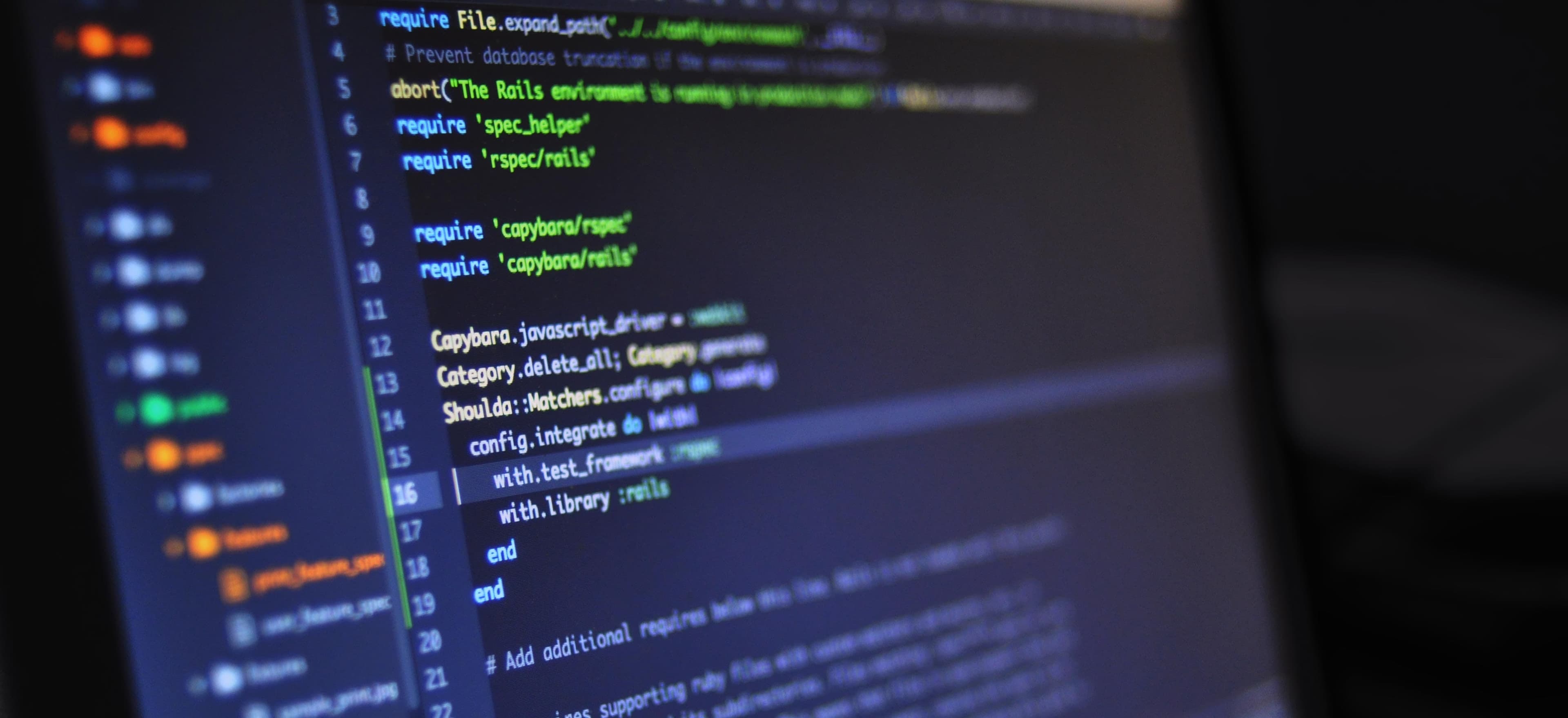
- Published on
Overcoming Common Pitfalls in Spring XD Data Ingestion
Spring XD, an open-source data ingestion and processing framework, has facilitated the development of real-time analytics by simplifying the creation of data ingestion pipelines. However, like any technology, it comes with its own set of challenges. In this blog post, we will explore common pitfalls associated with data ingestion using Spring XD and discuss best practices for overcoming them.
Understanding Spring XD
Before diving into the pitfalls, it's essential to grasp the core principles of Spring XD. It allows you to create a unified system for ingesting, processing, and analyzing streams of data. Users can configure streams and modules to specify how data flows through the system.
Basic Concepts
Streams: A stream is a pathway for data. It defines source modules, processor modules, and sink modules that manipulate data as it flows from input to output.
Modules: These are the individual components that handle the data at different stages. Common module types include:
- Source Modules: Capture data from various origins.
- Processor Modules: Transform the data in some way.
- Sink Modules: Output the processed data, such as to a database or message broker.
Pitfall #1: Poorly Defined Data Schema
One of the most common issues developers encounter is not defining a clear data schema. Inconsistent data structures can lead to runtime errors and inefficiencies.
Solution
Define a structured data schema using formats like JSON or Avro. This ensures that all components interpret the data correctly.
Example: Defining a Data Schema in JSON
{
"type": "object",
"properties": {
"userId": { "type": "integer" },
"eventType": { "type": "string" },
"timestamp": { "type": "string", "format": "date-time" }
},
"required": ["userId", "eventType", "timestamp"]
}
Why this Works: By using a schema, all modules in your Spring XD pipeline can validate incoming data against predefined structures. This minimizes data quality issues and streamlines processing.
Pitfall #2: Inefficient Data Processing
Many developers underestimate the complexity of processing data in real-time. Poorly designed data processing can create bottlenecks, slowing down the entire ingestion pipeline.
Solution
Develop efficient processing strategies. Use parallel processing wherever possible and break down complex tasks into simpler, smaller steps.
Example: Using a Composite Processor
@Bean
public StreamProcessor compositeProcessor() {
return new StreamProcessor() {
@Override
public void process(Data event) {
// Step 1: Validate Input
if (!isValid(event)) {
throw new InvalidEventException("Invalid Event Data");
}
// Step 2: Transform Data
Data processedData = transform(event);
// Step 3: Send to next process or save.
sendToNext(processedData);
}
private boolean isValid(Data data) {
// Validation logic
}
private Data transform(Data data) {
// Transformation logic
}
private void sendToNext(Data data) {
// Logic to send data to the next stage
}
};
}
Why this Works: This modular approach breaks down processing into manageable parts. Each part has a single responsibility, reducing the chance of errors and enhancing readability. You can maintain and troubleshoot more easily.
Pitfall #3: Lack of Monitoring
In a production environment, improper monitoring can lead to undetected failures. If an ingestion pipeline breaks down, data loss can occur, and costs can skyrocket.
Solution
Implement robust monitoring and alerting mechanisms. Use Spring XD's built-in monitoring tools or integrate with established solutions like Prometheus and Grafana.
Example: Sample Monitoring Setup
spring:
xd:
stream:
monitoring:
enabled: true
server:
port: 8080
Why this Works: By enabling monitoring, you gain insights into the performance and status of your streams. Alerts can notify you when something goes wrong, allowing for rapid troubleshooting and minimizing downtime.
Pitfall #4: Ignoring Data Partitioning
As data volumes grow, inefficient data partitioning can lead to scalability issues. A single data sink can become overwhelmed with requests.
Solution
Implement data partitioning and sharding techniques. Place limits on the size of each data partition, ensuring that no single module becomes a bottleneck.
Example: Partitioning with Kafka
If your source is Kafka, you can use partitions effectively:
@Bean
public ConsumerFactory<String, String> consumerFactory() {
return new DefaultKafkaConsumerFactory<>(consumerConfigs());
}
@Bean
public ConcurrentKafkaListenerContainerFactory<String, String> kafkaListenerContainerFactory() {
ConcurrentKafkaListenerContainerFactory<String, String> factory = new ConcurrentKafkaListenerContainerFactory<>();
factory.setConsumerFactory(consumerFactory());
factory.setConcurrency(3); // Set desired partitions
return factory;
}
Why this Works: By configuring concurrency and partitioning, multiple consumer instances can process data simultaneously. This enhances throughput and resilience, allowing your application to scale with data input.
Pitfall #5: Inadequate Documentation
Finally, as with any software project, insufficient documentation can cause confusion among team members and make onboarding new developers difficult.
Solution
Maintain thorough documentation on your Spring XD setup. Document the structure of your streams, data schemas, and any specific configurations.
Example Documentation Outline:
- Overview of Streams: Describe each stream and its purpose.
- Data Schemas: Provide examples of expected data structures.
- Configurations: Include instructions on setting up modules.
- Monitoring Setup: Explain how to monitor streams effectively.
Why this Works: Good documentation fosters knowledge sharing and reduces reliance on specific individuals. New developers can get up to speed quickly, improving productivity and enhancing your project's sustainability.
Closing the Chapter
Spring XD offers remarkable capabilities for data ingestion and real-time processing, but avoiding common pitfalls is essential for optimal performance. By carefully defining data schemas, implementing efficient processing strategies, incorporating robust monitoring, applying data partitioning, and maintaining comprehensive documentation, you can build resilient data pipelines that meet your organization's needs.
For further reading and resources, you can explore:
By addressing these common pitfalls, you're not only enhancing your application's reliability but also setting the foundation for future scalability and maintainability. Happy coding!