Choosing the Right Retry Strategy in Apache Camel
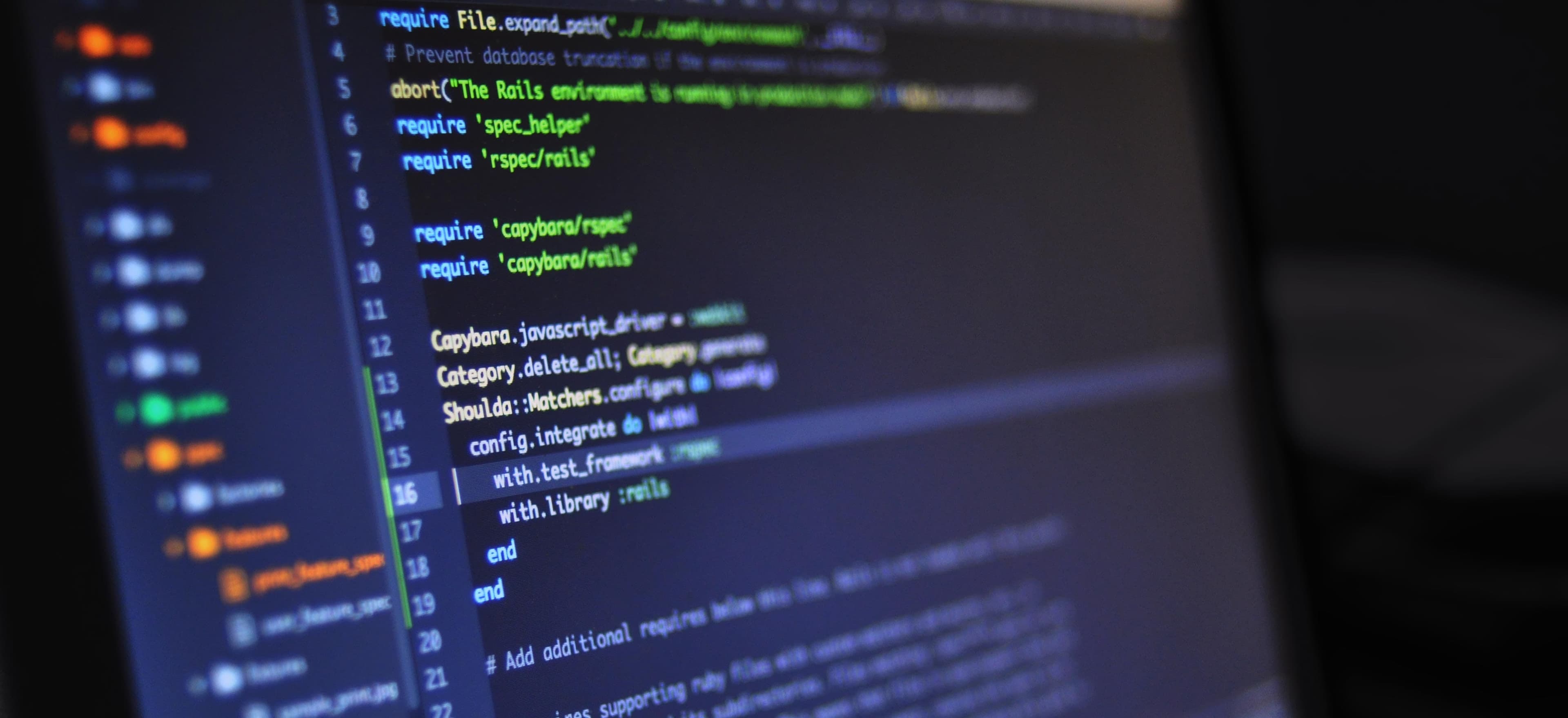
- Published on
Choosing the Right Retry Strategy in Apache Camel
When building integration solutions using Apache Camel, one critical aspect that developers often overlook is error handling, particularly setting up an effective retry strategy. Retry strategies are essential for ensuring that transient failures do not disrupt the entire message flow. In this blog post, we will explore the various retry strategies available in Apache Camel, their applications, and how to implement them effectively in your integration flows.
Understanding the Basics of Retry Strategies
Before diving into specific strategies, it’s important to understand what a retry strategy is. In essence, a retry strategy determines how an application responds when an operation fails. This includes how many times to attempt the operation, the delay between attempts, and the conditions under which to retry.
In Apache Camel, retry strategies can be applied at various levels, including the route level, processor level, or within specific components. This flexibility allows developers to customize error handling to suit their specific needs.
Common Retry Strategies
1. Simple Retry
The simplest form of retry strategy is the fixed delay. With this approach, if a failure occurs, the operation is retried after a defined period. This strategy is suitable for transient errors that are likely to resolve themselves after a short delay.
Example: Simple Retry with Fixed Delay
from("direct:start")
.to("http://example.com/api")
.retryAttemptedLogLevel(LoggingLevel.WARN)
.retryPolicy()
.fixedDelay(3000) // retry after 3 seconds
.maximumRetries(5) // maximum 5 attempts
.end();
Why Use It:
- Simple to implement and understand.
- Effective for operations where you know the situation might temporarily fail, like network issues.
2. Exponential Backoff
Exponential backoff augments the fixed delay approach by increasing the wait time after each failure. This strategy is beneficial in scenarios where repeated requests might overwhelm a service. For instance, after a failure, the first retry might occur after 1 second, the next after 2 seconds, then 4 seconds, and so on.
Example: Exponential Backoff
from("direct:start")
.to("http://example.com/api")
.retryAttemptedLogLevel(LoggingLevel.WARN)
.retryPolicy()
.exponentialBackoff() // set exponential backoff
.minimumDelay(1000) // start with 1 second
.maximumDelay(30000) // cap the delay at 30 seconds
.maximumRetries(5)
.end();
Why Use It:
- Reduces the load on the downstream service during failures.
- More graceful handling of failure scenarios, preventing potential cascading failures.
3. Circuit Breaker
The circuit breaker pattern is a more complex but powerful strategy. It prevents repeated requests from being sent to a service that is likely down or facing issues. After a certain number of failures, the circuit breaker “trips” and rejects requests for a specified timeout. This is useful in distributed systems where components can become unhealthy rather than just temporarily unavailable.
Example: Circuit Breaker Implementation
from("direct:start")
.to("http://example.com/api")
.retryPolicy()
.circuitBreaker() // enable circuit breaker
.failureThreshold(5) // 5 failures to trip the circuit
.successThreshold(2) // 2 successes to close the circuit
.timeout(20000) // 20 seconds timeout on failures
.end();
Why Use It:
- Protects your application from overloading failing services.
- Allows time for recovery in downstream services which can improve resilience.
Implementing Custom Retry Logic using Camel EIPs
Apache Camel provides several Enterprise Integration Patterns (EIPs) that can be utilized for implementing custom retry logic. You can enrich your retry handling scheme with these design patterns.
1. Dead Letter Channel
In a dead letter channel setup, after a certain number of retries, the message is sent to a specialized endpoint for further analysis. This approach allows developers to segregate messages that failed against the ones that were processed successfully.
Example: Dead Letter Channel Setup
from("direct:start")
.errorHandler(deadLetterChannel("direct:dead-letter"))
.retryAttemptedLogLevel(LoggingLevel.WARN)
.maximumRedeliveries(5) // attempt delivery five more times
.redeliveryDelay(1000) // delay of 1 second
.to("http://example.com/api");
Why Use It:
- Ensures that problematic messages don’t get lost.
- Helps in monitoring and analyzing recurring failures.
2. Custom Processor for Retry Logic
Sometimes, you may want to implement your specific retry logic based on business requirements. This can be achieved by creating a custom processor.
Example: Custom Processor Implementation
public class CustomRetryProcessor implements Processor {
@Override
public void process(Exchange exchange) throws Exception {
int attempts = 0;
boolean success = false;
while(attempts < 5 && !success) {
try {
// call external service
success = callExternalService(exchange.getIn().getBody(String.class));
} catch (Exception e) {
attempts++;
exchange.getIn().setHeader("retryAttempts", attempts);
Thread.sleep(1000 * attempts); // Increase delay for each attempt
}
}
if(!success) {
// Log or throw an exception as the final attempt failed
throw new Exception("Max retries exceeded");
}
}
private boolean callExternalService(String input) {
// Logic to call external service
return true; // Simulated successful call
}
}
Why Use It:
- Provides a fine-grained control over the retry logic.
- Developers can inject business-specific components into the retry mechanism.
The Closing Argument
Choosing the right retry strategy in Apache Camel significantly impacts the robustness and reliability of your integration applications. By understanding the different retry patterns—simple retries, exponential backoff, circuit breakers, dead letter channels, and custom processors—you can build resilient systems that gracefully handle transient failures.
Always remember, the goal of implementing a retry strategy is not just about retrying failed operations but also about designing a system that remains responsive and does not create further strain on already failing services.
For more information on Apache Camel’s error handling capabilities, check out the official documentation here.
Additionally, a deep dive into enterprise integration patterns can help further your understanding. Refer to Enterprise Integration Patterns for a more comprehensive study.
Happy coding!