Overcoming the Challenges of Parallel Testing in Docker
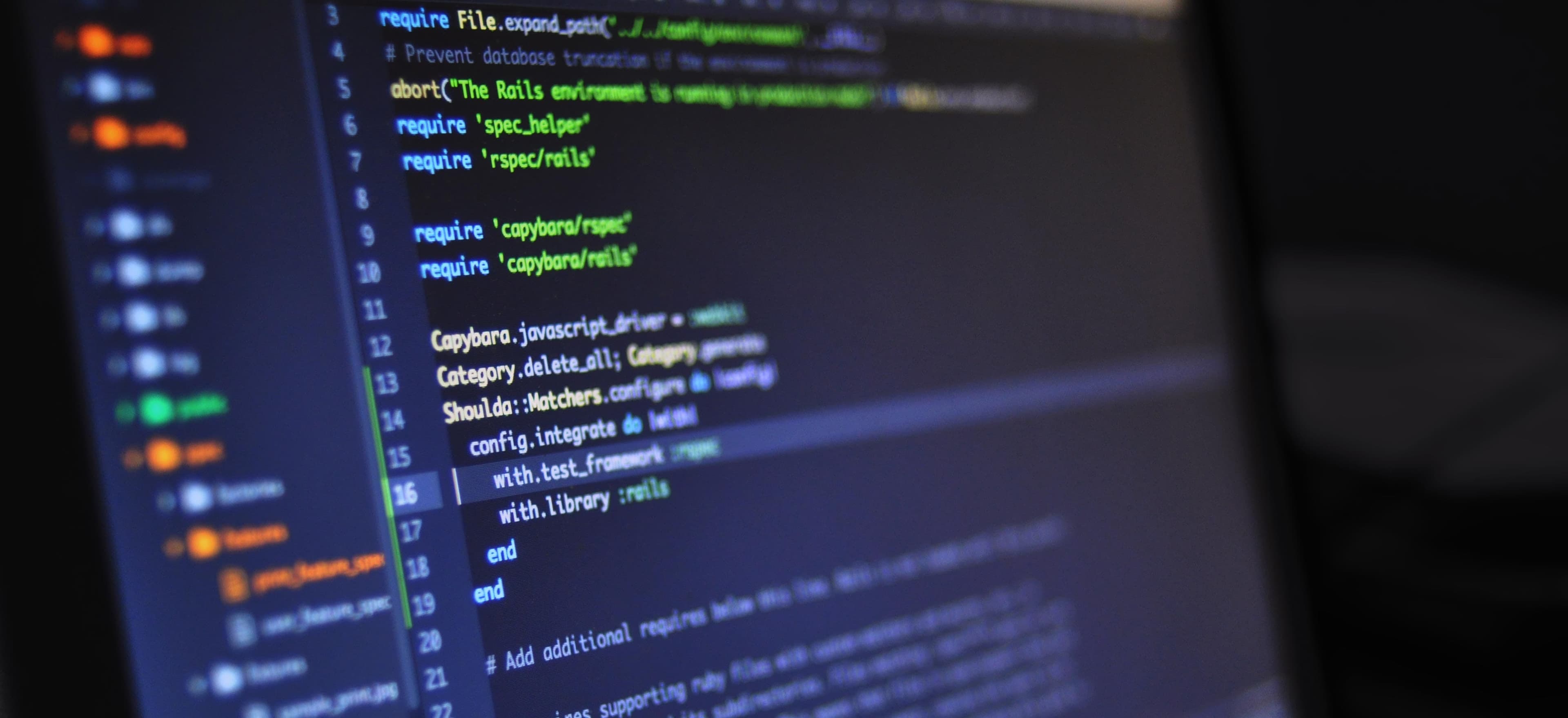
- Published on
Overcoming the Challenges of Parallel Testing in Docker
In the era of agile development, where rapid iterations and continuous integration/continuous delivery (CI/CD) are the norms, the ability to conduct parallel testing has become essential. With Docker gaining prominence for its ease of use and scalability, parallel testing within Docker containers has emerged as a powerful strategy for developers and QA teams alike. However, this approach is not without its challenges. In this blog post, we will delve into the intricacies of parallel testing in Docker, explore its common challenges, and offer practical solutions to overcome them.
What is Parallel Testing?
Parallel testing involves executing multiple tests simultaneously instead of sequentially. In a traditional testing setup, as tests run one after another, the entire process can become a bottleneck, slowing down the feedback loop. By leveraging parallelism, teams can significantly reduce their testing time. When combined with Docker’s containerization, parallel testing can lead to more efficient resource utilization and isolation of test environments.
Benefits of Parallel Testing with Docker
- Speed: Running tests in parallel drastically reduces the overall testing time.
- Isolation: Each test case runs in its container, leading to less interference and more reliable results.
- Scalability: Docker's architecture allows you to scale horizontally, adding more containers as needed.
- Consistent Environments: Docker ensures that each test runs in a standardized environment, minimizing "it works on my machine" problems.
Challenges of Parallel Testing in Docker
Despite its benefits, parallel testing in Docker presents unique challenges that teams need to navigate carefully:
1. Resource Management
Running multiple Docker containers can lead to resource exhaustion. Each container consumes CPU, memory, and other system resources. If not managed properly, this can lead to degraded performance or even failed tests.
Solution: Optimize resource allocation for each container. Use Docker’s resource limitation flags to control CPU and memory usage.
docker run --memory="256m" --cpus="1.0" your-image
This command restricts the container to 256 MB of memory and 1 CPU core, allowing for a more balanced distribution of resources across multiple containers.
2. Networking Issues
When running tests in parallel, containers may need to interact with each other or external services. Managing network configurations and dependencies becomes crucial.
Solution: Use Docker’s built-in network features. Create a custom network for your containers to simplify communication among them.
docker network create my-network
docker run --network=my-network your-image
This command establishes a dedicated network, allowing your containers to communicate seamlessly, mitigating the chances of connection failures.
3. Data State Management
Parallel tests might interact with shared data resources (like databases). This can lead to conflicts, inconsistent states, or even data corruption.
Solution: Utilize isolated databases for each test run. You can achieve this by spinning up a new containerized instance of the database for each test.
services:
db:
image: postgres
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
Using Docker Compose, each test can connect to a fresh database, maintaining clean and consistent states.
4. Dependency Conflicts
In complex applications, tests may depend on specific versions of libraries or components. Running them in parallel can lead to versioning conflicts.
Solution: Use Dockerfiles to specify exact dependencies for each test case.
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
CMD ["npm", "test"]
This Dockerfile ensures that your test runs with Node.js version 14, eliminating conflicts arising from mismatched library versions.
5. Debugging Challenges
Debugging parallel tests can be more complex than normal tests due to simultaneous execution.
Solution: Implement structured logging and reporting. Use tools like Allure to generate detailed reports including execution duration and failure reasons. Collect logs from each container for better insight.
docker logs <container_id>
By capturing logs from each test execution, you can trace back errors to their root cause more effectively.
Best Practices for Implementing Parallel Testing in Docker
Adopting best practices can simplify parallel testing in Docker and enhance your testing framework's reliability and efficiency.
-
Use Docker Compose: This allows you to define and manage complex multi-container Docker applications with ease.
-
Maintain a Small Container Size: Smaller containers result in faster startup times and less overhead. Strip down your base images to only include necessary dependencies.
-
Utilize CI Tools: Tools like Jenkins or GitHub Actions can manage Docker builds and orchestrate parallel test runs effectively.
-
Implement Full Isolation for Tests: Always choose to run tests in isolation. Use separate containers for different tests to eliminate state issues and ensure independence.
-
Monitor Resource Usage: Continuously track the resource consumption of your containers. Tools like Docker stats provide real-time insights.
-
Run Tests on a Scheduled Basis: While real-time feedback is crucial, consider running resource-intensive tests during off-hours or as part of nightly builds.
Example: Parallel Testing with Docker Compose
Here's a brief example of how to configure parallel testing using Docker Compose:
version: '3'
services:
test-service-1:
build:
context: .
dockerfile: Dockerfile1
networks:
- test-network
test-service-2:
build:
context: .
dockerfile: Dockerfile2
networks:
- test-network
networks:
test-network:
In this setup, multiple test services can run concurrently, each with its isolated configurations.
The Bottom Line
While parallel testing in Docker presents several challenges, being mindful of resource management, networking, data state, dependency conflicts, and debugging can lead to a highly efficient testing process. By following the outlined solutions and best practices, development teams can take full advantage of parallel testing, ensuring faster releases and higher quality software.
By investing in the right strategies and tools, such as Docker and CI testing pipelines, teams can embrace parallel testing with confidence. This not only optimizes the testing phase but ultimately enhances the entire software development lifecycle, thereby leading to a more agile and resilient development process.
For further reading on optimizing your Docker usage, consider visiting Docker Documentation or check out our previous posts on CI/CD Best Practices and Microservices Architecture.
Embrace these strategies and unleash the full potential of parallel testing in Docker. Happy testing!