Unlocking Spring AOP: Overcoming Annotation Confusion
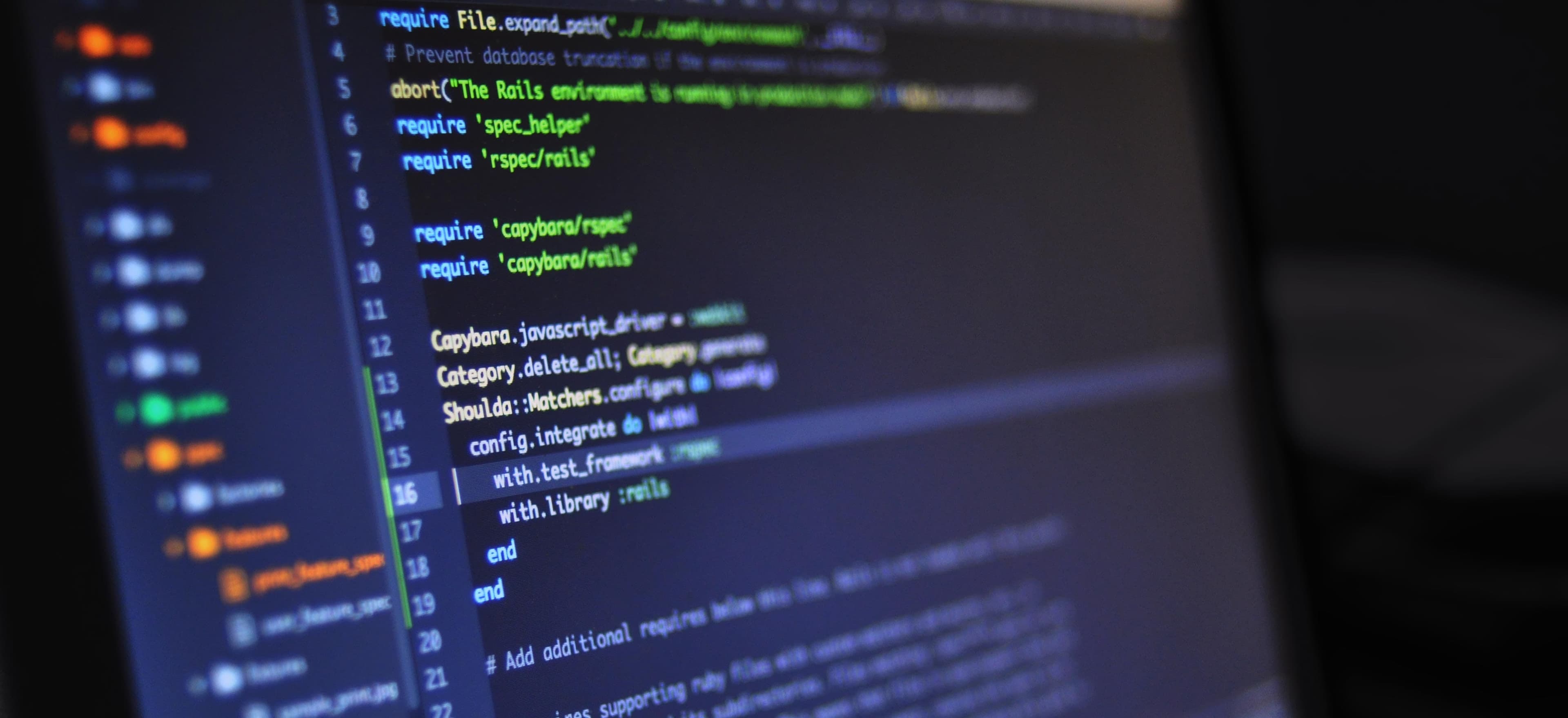
- Published on
Unlocking Spring AOP: Overcoming Annotation Confusion
Spring AOP (Aspect-Oriented Programming) is a powerful feature in the Spring Framework that enables developers to separate cross-cutting concerns from the business logic of an application. However, many developers experience confusion when dealing with the annotations involved in Spring AOP. In this blog post, we'll break down the key concepts and annotations associated with Spring AOP, provide some practical examples, and clear up any confusion you may have.
What is Spring AOP?
Understanding AOP
Aspect-Oriented Programming (AOP) is a programming paradigm that allows separation of cross-cutting concerns from the main business logic. Cross-cutting concerns include logging, transaction management, security, and performance monitoring, which can affect multiple parts of an application.
In Spring AOP, we define aspects, which consist of pointcuts and advice.
- Pointcuts are expressions that define where the advice should be applied.
- Advice is the action taken by the aspect at a specific join point (e.g., method execution).
Key Annotations in Spring AOP
When implementing Spring AOP, several key annotations come into play. Let's take a look:
1. @Aspect
The @Aspect
annotation indicates that the class is an aspect. This is the starting point for implementing AOP in Spring.
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
@Aspect
public class LoggingAspect {
@Pointcut("execution(* com.example.service.*.*(..))")
public void serviceLayer() {
// Pointcut expression for service layer
}
}
Why this matters: In this example, the LoggingAspect
class is marked as an aspect, allowing us to define pointcuts and advice that can be applied to methods within the com.example.service
package.
2. @Pointcut
The @Pointcut
annotation is used to define the conditions under which advice should be applied.
@Pointcut("execution(* com.example.controller.*.*(..))")
public void controllerLayer() {
// Pointcut expression for controller layer
}
Why this matters: Here, we defined a pointcut that targets all methods within the com.example.controller
package. This modularizes our logging logic and keeps it separated from our business logic.
3. @Before
The @Before
annotation indicates that advice should be executed before a join point.
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.JoinPoint;
@Before("serviceLayer()")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Executing: " + joinPoint.getSignature().getName());
}
Why this matters: This advice logs the method signature as it is being executed. It provides visibility into application behavior for debugging and auditing.
4. @After
In contrast, the @After
annotation is used for advice that runs after the join point.
import org.aspectj.lang.annotation.After;
@After("serviceLayer()")
public void logAfter(JoinPoint joinPoint) {
System.out.println("Executed: " + joinPoint.getSignature().getName());
}
Why this matters: This allows you to perform any necessary clean-up or logging after a method has executed.
5. @Around
The @Around
annotation is more powerful. It allows you to define advice that runs both before and after the join point, and it has the ability to control whether the advised method executes.
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
@Around("serviceLayer()")
public Object aroundAdvice(ProceedingJoinPoint proceedingJoinPoint) throws Throwable {
System.out.println("Before executing: " + proceedingJoinPoint.getSignature().getName());
Object result = proceedingJoinPoint.proceed(); // Proceeding with the method execution
System.out.println("After executing: " + proceedingJoinPoint.getSignature().getName());
return result;
}
Why this matters: The aroundAdvice
method lets you control method execution. It’s particularly useful for aspects that require extensive management like transaction management, where you might want to begin or commit transactions based on success or failure.
Real-World Example
Let’s create a more complete example. Assume you have a simple Spring application with a service that processes transactions.
Service Layer
package com.example.service;
import org.springframework.stereotype.Service;
@Service
public class TransactionService {
public void processTransaction(String transactionId) {
System.out.println("Processing transaction: " + transactionId);
}
}
Aspect Implementation
package com.example.aspect;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class TransactionAspect {
@After("execution(* com.example.service.TransactionService.processTransaction(..))")
public void logAfter(JoinPoint joinPoint) {
System.out.println("Transaction processed: " + joinPoint.getArgs()[0]);
}
}
Configuring Aspect in Spring
To enable AspectJ support in your Spring application, you need to add the @EnableAspectJAutoProxy
annotation in your configuration class.
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
@Configuration
@EnableAspectJAutoProxy
public class AppConfig {
// Configuration details
}
Summary of Execution Flow
- The method
processTransaction
inTransactionService
is called. - The advice defined in
logAfter
withinTransactionAspect
is triggered. - After the main logic is completed, the aspect logs the transaction.
The Last Word
Spring AOP is a powerful tool to manage cross-cutting concerns in your application. Understanding the annotations such as @Aspect
, @Pointcut
, @Before
, @After
, and @Around
is crucial for effectively leveraging this feature.
By properly segregating your business logic from cross-cutting concerns, you create a more maintainable and scalable codebase.
If you're interested in diving deeper, consider reading the official Spring documentation which provides comprehensive insights into the inner workings of AOP in Spring.
For more practical applications of AOP and discussion on related patterns, explore frameworks or libraries such as AspectJ, which can also be used with Spring for advanced AOP capabilities.
Now that you have a clearer understanding of Spring AOP and its annotations, you'll be better equipped to implement it in your projects. Happy coding!
Checkout our other articles