Common Pitfalls with Spring Order Annotations Explained
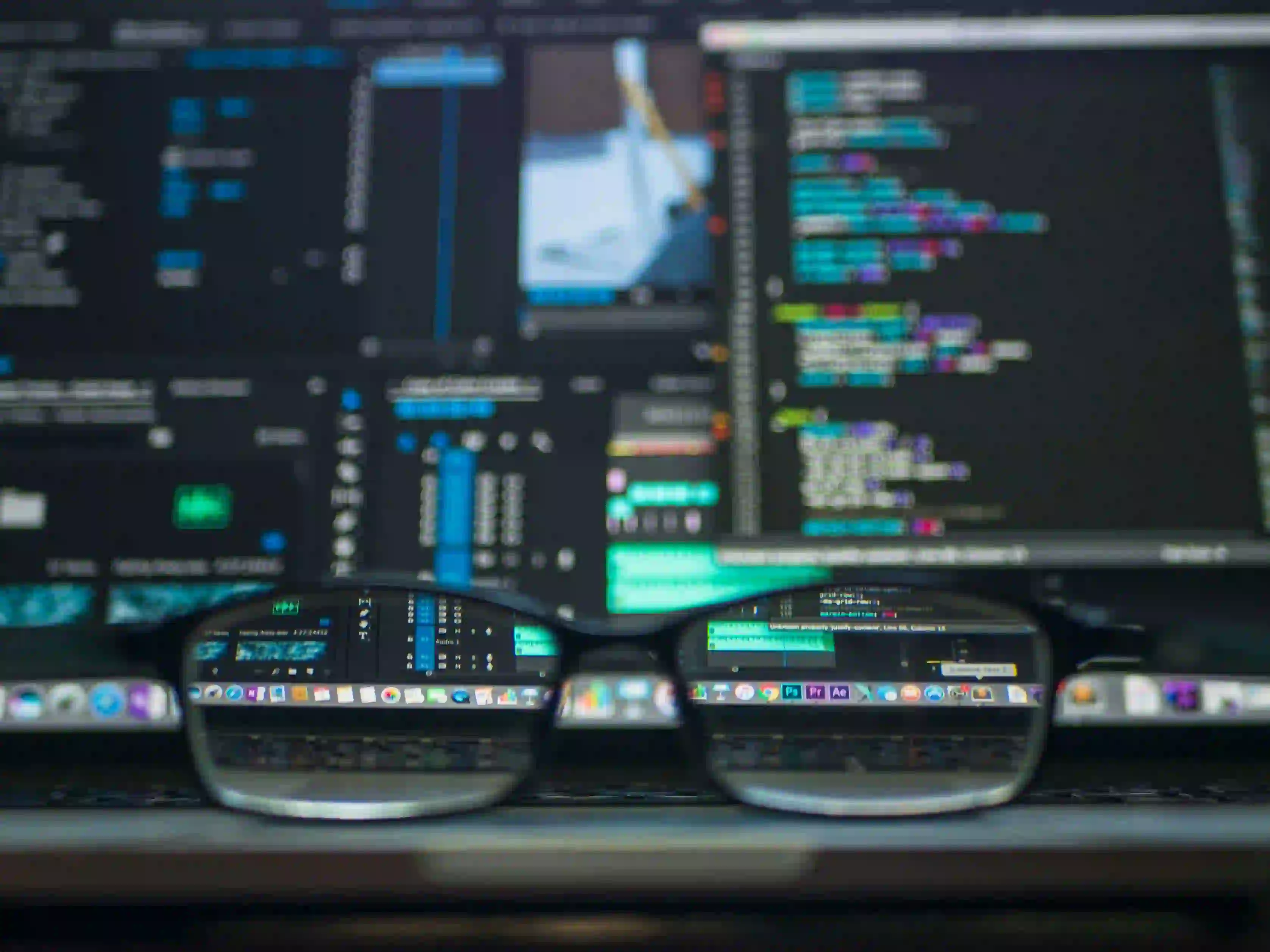
Common Pitfalls with Spring Order Annotations Explained
Spring is a powerful framework that simplifies Java application development. One of its often underappreciated features is the ability to control the order in which components are executed, particularly when dealing with beans, aspect-oriented programming (AOP), and filters.
In this blog post, we will discuss the concept of order annotations in Spring, including their benefits and common pitfalls developers may encounter. By the end of this article, you will have a clearer understanding of how to utilize these annotations effectively.
What are Spring Order Annotations?
In Spring, several annotations can dictate the ordering of components. The most common ones include:
- @Order
- @Priority
- @DependsOn
These annotations help manage the execution sequence of beans, aspects, or filters, which can influence application stability, performance, and correctness.
Why is Order Important?
In many scenarios, the sequence in which beans are initialized or aspects are applied can have significant implications. For example:
- Initializers might depend on other beans being available.
- AOP aspects (e.g., transaction management) might need to be applied in a specific order.
- Filters in a web application must be executed in a defined sequence for correct request handling.
Understanding the order of execution can save developers from subtle bugs that could lead to runtime errors or unexpected behavior.
Using @Order Annotation
The @Order
annotation is primarily used for specifying the order of beans of the same type or in the same context. The lower the value, the higher the precedence.
Example Usage of @Order
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.annotation.Order;
@Configuration
public class AppConfig {
@Bean
@Order(1)
public MyService firstService() {
return new MyService("First Service");
}
@Bean
@Order(2)
public MyService secondService() {
return new MyService("Second Service");
}
}
Commentary on the Example
In the example, we define a configuration class that contains two beans of the same type, MyService
. By annotating these beans with the @Order
annotation, we specify that firstService
should be initialized before secondService
.
When these beans are injected, Spring will respect this order, which can be particularly crucial when these services depend on each other or need to interact in a specific way.
Common Pitfall: Ignoring Context
One common mistake is to forget that @Order
works within the context of bean types.
If you have multiple bean types (e.g., beans of different interfaces), the order of injection may not work as intended. In such cases, be sure to group related beans together using the same interface.
Understanding @Priority Annotation
The @Priority
annotation can also be employed, particularly in cases involving AOP aspects or filters. It defines the order in which these components should be executed.
Example Usage of @Priority
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.core.annotation.Order;
import org.springframework.stereotype.Component;
@Aspect
@Component
@Order(1)
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore() {
System.out.println("Logging before method execution");
}
}
Commentary on the Example
In this example, we have an AOP aspect that logs method calls within the service layer. By using @Order(1)
, we ensure that the logging aspect is executed first before any service method is called, allowing for accurate logging.
Common Pitfall: Confusion with Bean Types
Developers sometimes confuse @Priority
with @Order
. It’s essential to recognize that @Priority
is often used in an AOP context while @Order
is generally applicable to bean initialization order. Misapplying these annotations can lead to ineffective execution order.
Dependency Injection with @DependsOn
While @Order
and @Priority
dictate the order of execution, @DependsOn
defines explicit dependencies between beans, ensuring that a bean is only created after the specified dependencies.
Example Usage of @DependsOn
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.DependsOn;
@Configuration
public class ServiceConfig {
@Bean
public DataSource dataSource() {
return new DataSource();
}
@Bean
@DependsOn("dataSource")
public MyService myService() {
return new MyService(dataSource());
}
}
Commentary on the Example
In this configuration class, we have a MyService
bean that explicitly depends on the DataSource
bean. The @DependsOn
annotation guarantees that the dataSource()
method gets called and the DataSource
bean is created before myService()
.
Common Pitfall: Overusing @DependsOn
While @DependsOn
is valuable, overusing it can introduce unnecessary rigidity into your application. Often, Spring's dependency injection can manage bean creation order based on field injection without explicit dependencies. Aim for simplicity and consider the aspects of loose coupling when structuring your beans.
Tips for Avoiding Pitfalls
-
Be Clear on Dependencies: Understand which components depend heavily on each other. Over-specifying order might lead to tightly coupled components.
-
Keep It Simple: Avoid unnecessary complexity by using the simplest ordering mechanism available.
-
Use Profiles Wisely: Configure different order requirements if you have multiple profiles; keeping different contexts organized can prevent confusion.
-
Document Your Decisions: If you find that a particular order is crucial, include comments in your code to explain the reasoning behind the decisions made.
-
Test Extensively: Write integration tests to ensure that the desired ordering works as expected, especially in complex scenarios.
Key Takeaways
Using Spring Order Annotations can be a powerful way to manage component execution and dependencies. By understanding how @Order
, @Priority
, and @DependsOn
function, you can structure your application more effectively.
However, as with any feature, it's essential to be aware of potential pitfalls. By following best practices and thoroughly comprehending your application's architecture, you can mitigate these issues.
For further reading on Spring annotations, refer to the Spring Framework Documentation. With the knowledge acquired here, you're now equipped to handle order annotations like a pro! Happy coding!