Common Pitfalls When Using Java Iterators with Streams
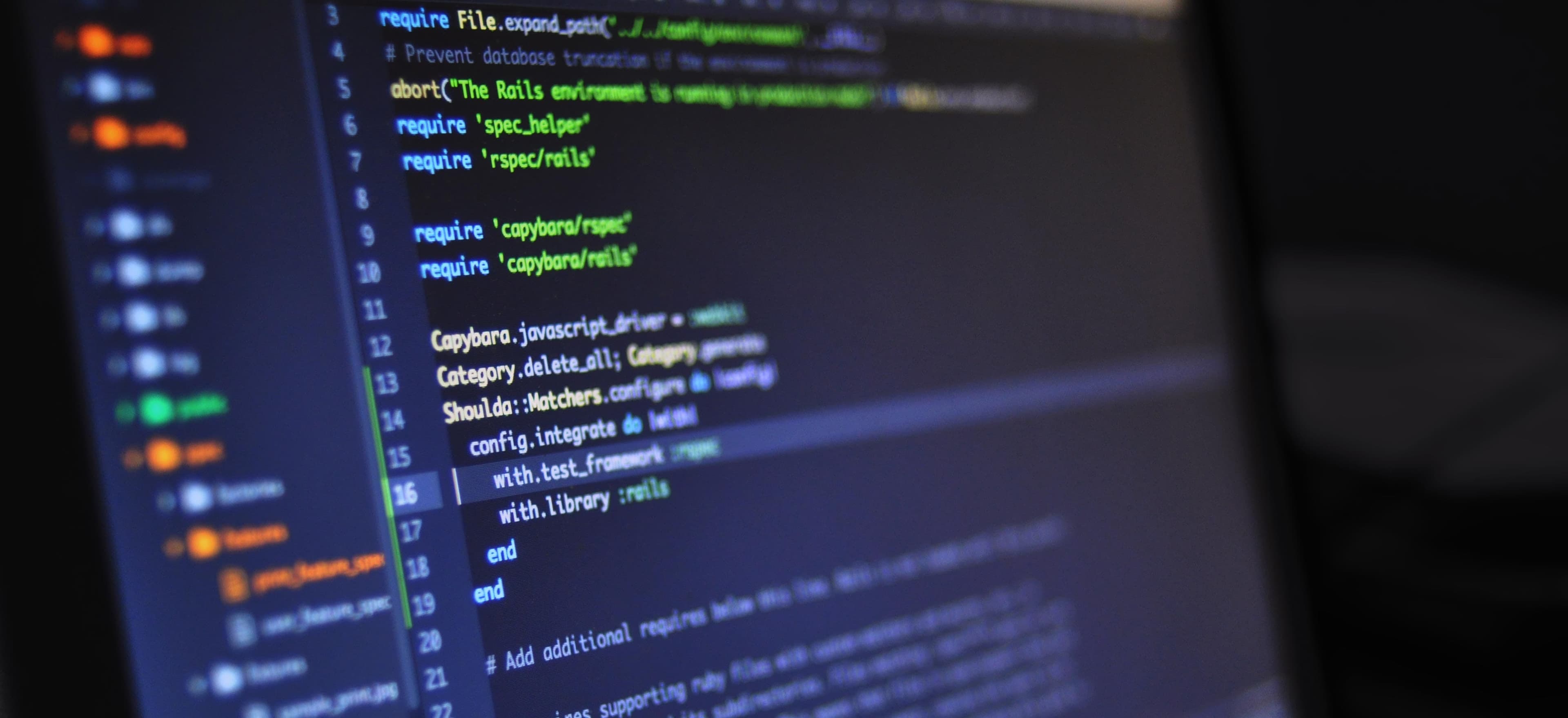
- Published on
Common Pitfalls When Using Java Iterators with Streams
Java's introduction of the Stream API has revolutionized how developers approach data processing collections. While the Stream API offers robust functionality and cleaner code, combining it with iterators can lead to some common pitfalls. In this blog post, we will explore these pitfalls, why they occur, and how to avoid them to ensure your Java applications remain efficient and bug-free.
Understanding Java Streams and Iterators
Before we delve into the pitfalls, it's crucial to understand the roles of Streams and Iterators.
-
Streams: Introduced in Java 8, Streams are a sequence of elements that support various operations to perform computations on these elements. They can be generated from collections, arrays, or even IO channels.
-
Iterators: An Iterator is an object that allows you to traverse through a collection, specifically lists, sets, or maps. While it provides methods to check if there are more elements and to retrieve the next element, the Iterator itself doesn’t provide bulk operations.
The Relationship Between Streams and Iterators
Streams are designed to work seamlessly with collections and iterators. However, they have different design principles—streams favor functional-style operations while iterators are more procedural. This difference can lead to some missteps when they are used interchangeably. Let's explore some of these pitfalls in detail.
Common Pitfalls When Using Java Iterators with Streams
1. Modifying the Source Collection
One prevalent mistake is modifying the underlying collection while streaming through an iterator. Consider the following example:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class ModifyWhileIterating {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
// This loop modifies the list while iterating
Iterator<String> iterator = names.iterator();
while (iterator.hasNext()) {
String name = iterator.next();
if (name.equals("Bob")) {
names.remove(name); // Pitfall: Modifying the collection
}
}
System.out.println(names); // Unexpected result!
}
}
Why This Happens
Modifying a collection while iterating over it can lead to ConcurrentModificationException
. The iterator's internal state becomes inconsistent with the collection's state, resulting in potentially undefined behavior.
Solution
Instead of modifying the collection directly while iterating, consider collecting the items to be removed in a separate list and using removeAll()
afterward. For example:
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class SafeModification {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
// Using Streams to filter without modifying the original list
List<String> filteredNames = names.stream()
.filter(name -> !name.equals("Bob")) // Filter out the unwanted name
.collect(Collectors.toList()); // Collect results
System.out.println(filteredNames); // Correct result
}
}
2. Using Iterators with Statefulness
Another common pitfall is using stateful lambda expressions when operating with streams. Stateful operations can lead to unpredictable behavior, especially in concurrent environments.
Consider this example:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.stream.Stream;
public class StatefulLambdaExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
// Using a stateful lambda
Stream<String> stream = names.stream();
Iterator<String> iterator = stream.iterator();
while (iterator.hasNext()) {
String name = iterator.next();
if (name.startsWith("A")) {
// Stateful operation; should be avoided
System.out.println(name);
}
}
}
}
Why This Happens
When using stateful lambdas, the behavior can change unpredictably in repetitive calls, especially in parallel streams. This can lead to performance degradation and logical errors.
Solution
Use stateless lambdas where possible. Here’s an example of improving the above approach:
import java.util.List;
import java.util.stream.Collectors;
public class StatelessLambdaExample {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", "Charlie");
// Using stateless lambda expression
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A")) // Purely function
.collect(Collectors.toList()); // Collect results
filteredNames.forEach(System.out::println); // Outputs Alice
}
}
3. Mixing Stream and Iterator Operations
Mixing Stream and Iterator operations can lead to confusion and runtime exceptions. For instance, attempting to iterate a Stream after it has already been operated on will result in an IllegalStateException
.
import java.util.List;
public class MixedOperationExample {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", "Charlie");
// Creating a Stream
var stream = names.stream()
.filter(name -> name.startsWith("A"));
// Consuming the stream
stream.forEach(System.out::println); // Valid!
// Now trying to modify the stream: IllegalStateException
stream.forEach(System.out::println); // Error!
}
}
Why This Happens
A stream can only be consumed once. After the terminal operation is performed, it becomes unusable. This behavior differs markedly from iterators, which can continuously be reused unless modified.
Solution
If you need to traverse the data multiple times, consider creating a new stream each time. As shown below:
import java.util.List;
public class SafeStreamUsage {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", "Charlie");
// Generating a new stream each time
processNames(names);
processNames(names); // Okay to process again
}
private static void processNames(List<String> names) {
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
}
}
4. Not Handling Nulls Properly
When using streams, ignoring null pointers can cause NullPointerException
at runtime. For instance:
import java.util.List;
public class NullHandlingExample {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", null, "Charlie");
// This can throw a NullPointerException
names.stream().filter(name -> name.equals("Alice")).forEach(System.out::println);
}
}
Why This Happens
Streams do not inherently handle null values, which means your filters and operations must account for existing nulls to avoid runtime exceptions.
Solution
You can handle null values gracefully using the Optional
class. Below is an improved version of the above code:
import java.util.List;
import java.util.Optional;
public class SafeNullHandlingExample {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", null, "Charlie");
// Using Optional to handle nulls
names.stream()
.filter(name -> Optional.ofNullable(name)
.map(String::equals).orElse(false))
.forEach(System.out::println); // Safely handle nulls
}
}
Closing the Chapter
Java's Stream API is a powerful feature that, when used with caution, can greatly enhance the efficiency and readability of your code. However, combining streams with iterators introduces certain pitfalls that can lead to exceptions and unpredictable behavior. By understanding these issues, using stateless lambdas, properly handling modifications to collections, and avoiding null pointers, you can write cleaner, more effective Java code.
For further reading on Java Streams and best design practices, consider checking out Java 8 in Action and the official Java documentation.
Would you like to share your experiences with using streams and iterators in Java, or have questions about specific scenarios? Feel free to comment below!
Checkout our other articles