Detecting Dirty Fields: Serialization Simplified
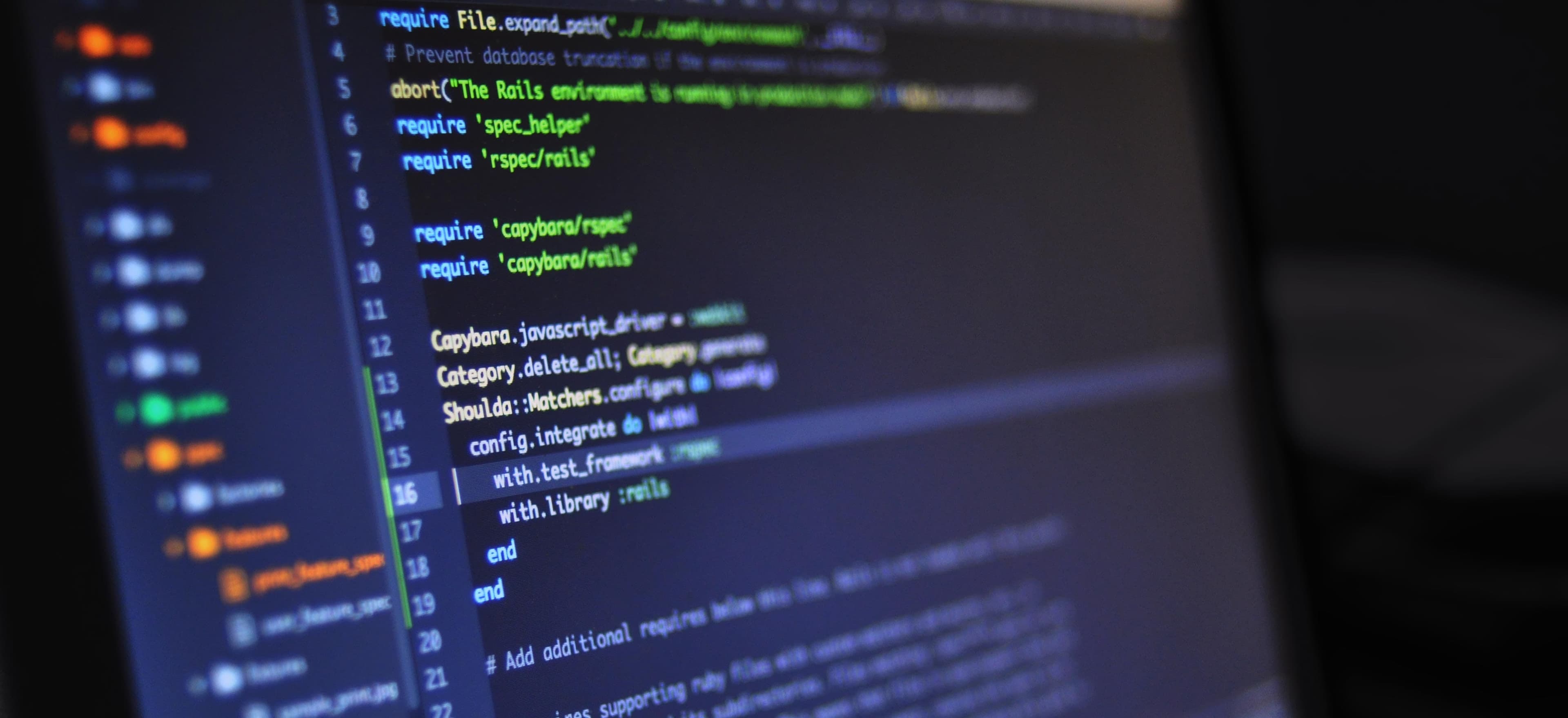
- Published on
Detecting Dirty Fields: Serialization Simplified
In the realm of Java development, effective data management is paramount. One of the more nuanced challenges that programmers face is determining which fields of an object have changed, particularly when it comes to serialization. This process, often referred to as "dirty checking," is an essential part of efficiently managing object state. In this blog post, we will explore how to detect dirty fields in Java, how this can simplify your serialization process, and discuss best practices to enhance your code.
Understanding Serialization
Serialization in Java refers to the conversion of an object into a byte stream, allowing for easy storage or transmission. When this object is later deserialized, it can be reconstructed in its original form. Java provides a built-in mechanism for serialization through the Serializable
interface. This is handy, but it can be cumbersome if you need to keep track of which fields have changed during the object's lifecycle.
Why Detect Dirty Fields?
Understanding what constitutes a "dirty field" gives you more control over object persistence. Here are the primary benefits:
- Performance Optimization: By only serializing the fields that have changed, you reduce the amount of data that needs to be processed.
- Data Integrity: Detecting changes beforehand ensures that you are not sending stale data.
- Dynamic Methods: It leads to a more dynamic way to interact with data models, particularly when interfacing with databases or services.
Basic Implementation of Dirty Checking
To illustrate how you can implement dirty checking in Java, let’s create a simple class, Person
, that tracks whether its fields have changed since the last serialization.
import java.io.Serializable;
public class Person implements Serializable {
private String name;
private int age;
// Keep track of dirty state
private boolean dirty;
public Person(String name, int age) {
this.name = name;
this.age = age;
this.dirty = false;
}
public String getName() {
return name;
}
public void setName(String name) {
if (!this.name.equals(name)) {
this.name = name;
setDirty(true);
}
}
public int getAge() {
return age;
}
public void setAge(int age) {
if (this.age != age) {
this.age = age;
setDirty(true);
}
}
public boolean isDirty() {
return dirty;
}
private void setDirty(boolean dirty) {
this.dirty = dirty;
}
}
Explanation of the Code
-
Attributes: The
Person
class containsname
andage
as its primary attributes. Each user can set and get their values via appropriate methods. -
Dirty State: A private boolean flag
dirty
is added to keep track of whether the object has been modified. -
Setters: In the setter methods (
setName
andsetAge
), we compare the new value with the current value. If they differ, we update the object's state and mark it dirty. -
Checking Dirty State: The method
isDirty()
simply returns the state of thedirty
flag, allowing you to easily analyze if the object should be serialized or not.
Advanced Detection Methods
The above method works well for simple cases but can fall short when dealing with complex objects or collections. Let's look at an alternative approach using a more advanced technique, which utilizes a tracking mechanism for dirty fields.
Using a Map to Track Changes
For classes with multiple fields, we can utilize a Map
to keep track of which fields have changed. We can extend our Person
class to demonstrate this approach.
import java.io.*;
import java.util.HashMap;
import java.util.Map;
public class Person implements Serializable {
private String name;
private int age;
private transient Map<String, Boolean> dirtyFields = new HashMap<>();
public Person(String name, int age) {
this.name = name;
this.age = age;
markDirty("name", false);
markDirty("age", false);
}
public String getName() {
return name;
}
public void setName(String name) {
if (!this.name.equals(name)) {
this.name = name;
markDirty("name", true);
}
}
public int getAge() {
return age;
}
public void setAge(int age) {
if (this.age != age) {
this.age = age;
markDirty("age", true);
}
}
private void markDirty(String fieldName, boolean dirty) {
dirtyFields.put(fieldName, dirty);
}
public Map<String, Boolean> getDirtyFields() {
return dirtyFields;
}
}
Explanation of the Enhanced Code
-
Transient Field: The
dirtyFields
map is marked astransient
, which means it won't be serialized directly. -
Marking Fields as Dirty: The
markDirty
method allows us to specify which field is dirty. We update the map when any setter modifies a field. -
Getting Dirty Fields: Using the
getDirtyFields
method, you can quickly determine which fields are marked as dirty for further processing.
Example Usage
Here’s how you might use the Person
class with dirty field detection in practice:
public class Main {
public static void main(String[] args) {
Person person = new Person("John Doe", 30);
//Change some fields
person.setName("Jane Doe");
person.setAge(31);
// Check which fields are dirty
Map<String, Boolean> dirtyFields = person.getDirtyFields();
System.out.println("Dirty fields: " + dirtyFields); // Output: Dirty fields: {name=true, age=true}
// Serialization logic here based on dirty fields
serializePersonIfDirty(person);
}
private static void serializePersonIfDirty(Person person) {
if (!person.getDirtyFields().isEmpty()) {
// Serialize this person
System.out.println("Serializing the person object.");
} else {
System.out.println("No need to serialize; no dirty fields.");
}
}
}
Wrapping Up
Detecting dirty fields can significantly simplify the serialization process, leading to better performance and data integrity. By implementing a simple approach with flags or a more complex tracking mechanism, you can dynamically manage field changes in your Java applications.
For more in-depth discussions on object serialization, you might find these resources helpful:
Remember, the key is not only to make your code functional but also efficient and maintainable. Happy coding!
Checkout our other articles