Unpacking ZIP Files in Java 8: Stream API Simplified
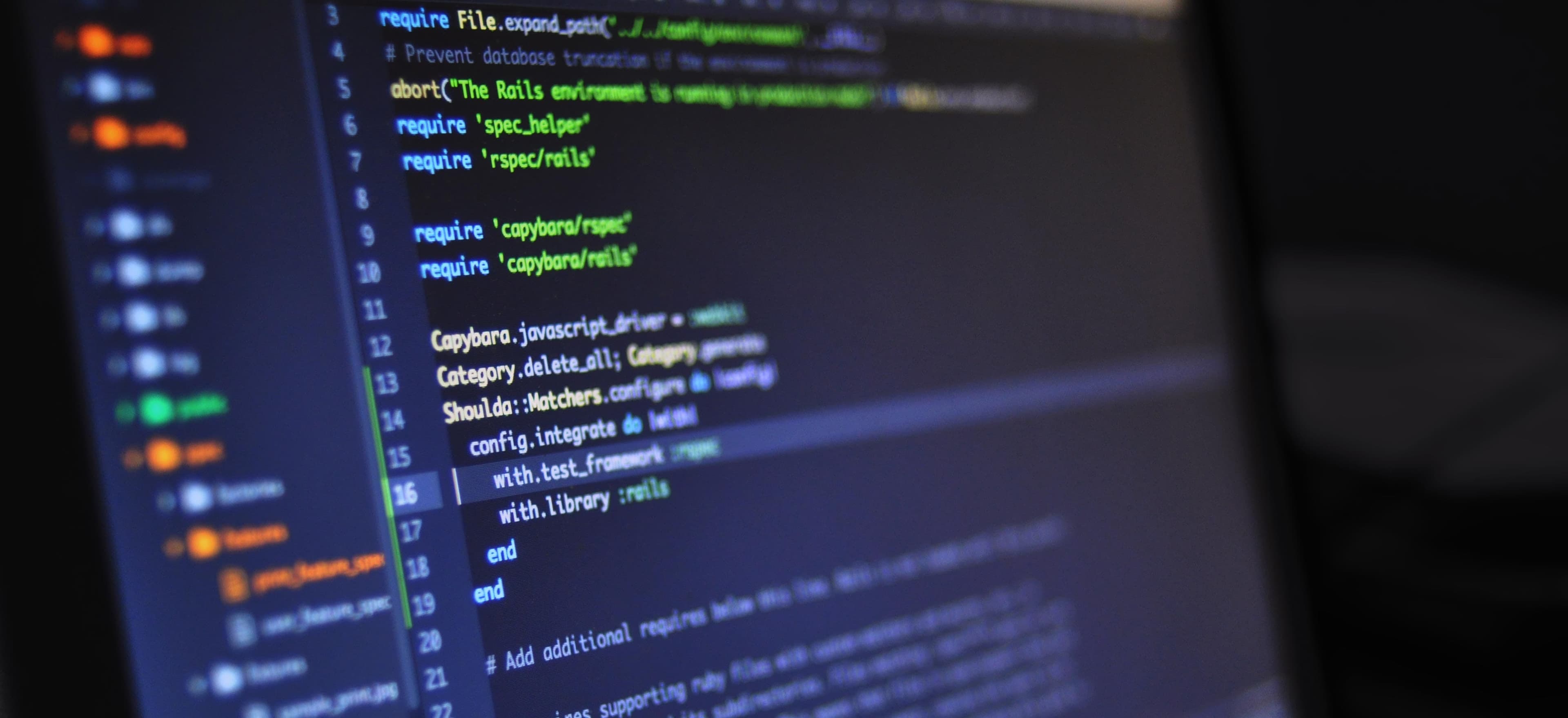
- Published on
Unpacking ZIP Files in Java 8: Stream API Simplified
Java has effortlessly adapted to the demands of software development, and with the advent of Java 8, we saw the introduction of the Stream API—a powerful tool for processing sequences of elements, including files. In this blog post, we will explore how to unpack ZIP files in Java 8 using the Stream API, allowing for an efficient, readable, and elegant solution to a common task.
Understanding ZIP Files
ZIP files are compressed archives that contain one or more files or folders. They save space and are convenient for distributing multiple files together. Unpacking or "unzipping" these files can be accomplished in Java using built-in libraries, but Java 8's Stream API offers a more modern approach.
By using the Stream API, we can handle file input and output in a functional manner, making our code cleaner and reducing boilerplate code.
Setting Up Your Java Environment
Before we dive into coding, ensure you have the following:
- Java Development Kit (JDK) 8 or higher.
- A suitable Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or Visual Studio Code.
Unpacking a ZIP File: The Basics
The key Java classes we will be using include:
java.util.zip.ZipInputStream
: To read the ZIP file.java.nio.file.Files
: To handle files and directories.java.nio.file.Paths
: To represent file paths.
Reading a ZIP File in Java
Let's start with a simple method to unpack a ZIP file. Here is a straightforward implementation:
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class ZipUnpacker {
public void unpack(String zipFilePath, String destDir) throws IOException {
Path destDirPath = Paths.get(destDir);
try (ZipInputStream zipInputStream = new ZipInputStream(Files.newInputStream(Paths.get(zipFilePath)))) {
ZipEntry entry;
while ((entry = zipInputStream.getNextEntry()) != null) {
Path filePath = destDirPath.resolve(entry.getName());
if (entry.isDirectory()) {
Files.createDirectories(filePath);
} else {
// Ensure the parent directory exists
Files.createDirectories(filePath.getParent());
// Copy the file
try (InputStream inputStream = zipInputStream) {
Files.copy(inputStream, filePath, StandardCopyOption.REPLACE_EXISTING);
}
}
zipInputStream.closeEntry();
}
}
}
}
Code Explanation
- ZipInputStream: We create a
ZipInputStream
to read the ZIP file. - ZipEntry: Each entry in the ZIP file (be it a file or directory) is represented by this class.
- Resolving Paths: We resolve the destination path where files will be unzipped.
- Creating Directories: Before copying files, we check if any directories need to be created.
- Copying Files: Finally, we copy each file from the ZIP to the desired location.
This code effectively unpacks a ZIP file, handling both files and directories. It also gracefully closes entries to avoid memory leaks.
Using Stream API for Unpacking
Java 8 provides a concise way to process collections with its Stream API, enabling a more functional style of programming. Although unpacking isn't a typical use case for streams, we can streamline our code’s readability and maintainability.
Here’s how we can modify our earlier code using Files.list
combined with streams for handling the input and creating directories:
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.*;
import java.util.stream.Stream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class ZipUnpackerWithStreams {
public void unpack(String zipFilePath, String destDir) throws IOException {
Path destDirPath = Paths.get(destDir);
// Process the ZIP file
try (ZipInputStream zipInputStream = new ZipInputStream(Files.newInputStream(Paths.get(zipFilePath)))) {
Stream<ZipEntry> zipEntryStream = Stream.generate(() -> {
try {
return zipInputStream.getNextEntry();
} catch (IOException e) {
return null;
}
}).takeWhile(entry -> entry != null);
zipEntryStream.forEach(entry -> {
try {
Path filePath = destDirPath.resolve(entry.getName());
if (entry.isDirectory()) {
Files.createDirectories(filePath);
} else {
// Ensure the parent directory exists
Files.createDirectories(filePath.getParent());
// Copy the file
try (InputStream inputStream = zipInputStream) {
Files.copy(inputStream, filePath, StandardCopyOption.REPLACE_EXISTING);
}
}
} catch (IOException e) {
e.printStackTrace();
}
});
}
}
}
What Changed?
In this code:
- Stream Generation: We generate a stream of
ZipEntry
objects. TheStream.generate
method allows us to produce entries on-the-fly. This approach can enhance performance in certain scenarios. - takeWhile: This method keeps generating entries until it encounters a null (indicating the end of the stream).
- forEach Loop: Rather than a traditional for loop, we leverage the
forEach
method to iterate through the entries, promoting a functional style.
Handling Exceptions
Proper error handling is critical to improving user experience. In our examples, we catch exceptions where appropriate, allowing the application to handle problems gracefully. You might want to throw exceptions customarily or log them based on your application needs.
Final Thoughts
Unpacking ZIP files in Java 8 can be efficiently achieved using the Stream API. This transformation from imperative to functional programming is not just about writing less code; it's about clarity and maintainability. The elegance of the Stream API allows developers to express their intentions more clearly and handle files with ease.
Next Steps
Ready to dive deeper? Explore how to compress files into ZIP archives, handle various file formats, or even integrate ZIP file handling into larger applications! If you're looking for comprehensive documentation, visit the official Java Documentation.
Additional Resources
Happy coding!
Checkout our other articles