Mitigating Apache Tomcat's Denial of Service Risks
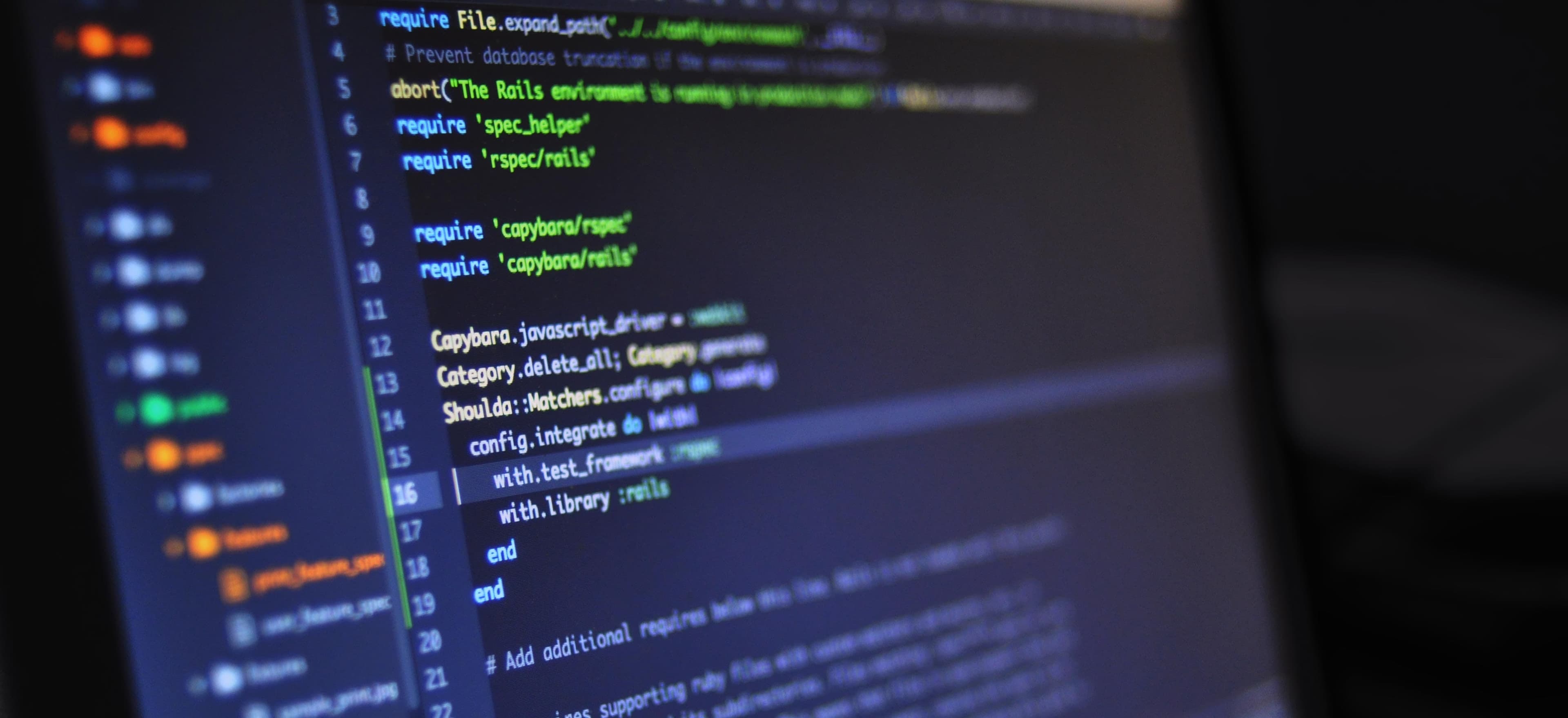
- Published on
Mitigating Apache Tomcat's Denial of Service Risks
Apache Tomcat is one of the most popular web servers and servlet containers. However, as with any technology, it comes with its own set of security risks—one of the most significant being Denial of Service (DoS). In this blog post, we will explore various techniques to mitigate DoS attacks effectively within an Apache Tomcat environment.
Understanding Denial of Service Attacks
Before diving into prevention strategies, let’s discuss what a DoS attack entails. In essence, a DoS attack aims to make a machine or network resource unavailable to its intended users by overwhelming it with a flood of illegitimate requests. For Apache Tomcat, this can manifest in multiple ways, such as HTTP request flooding or resource exhaustion.
Common Vulnerabilities in Apache Tomcat
- Resource Exhaustion: Tomcat can be overwhelmed if too many requests are processed simultaneously.
- Session Fixation: Attackers might manipulate session IDs to hijack user sessions.
- Misconfigured settings: This can lead to a weaker defense against attacks.
Basic Steps for Risk Mitigation
-
Keep Tomcat Updated: Always use the latest stable version of Tomcat. Updates often include critical security patches. You can find the latest version on the official Tomcat website.
-
Tune Connector Parameters: You can configure the server to limit the number of concurrent connections. Add attributes like
maxConnections
,maxThreads
, andacceptCount
in yourserver.xml
as shown below:
<Connector port="8080"
protocol="HTTP/1.1"
connectionTimeout="20000"
redirectPort="8443"
maxConnections="1000"
maxThreads="200"
acceptCount="50"/>
Why?
Setting these parameters limits the number of simultaneous connections, thereby protecting against traffic floods.
Implementing Rate Limiting
Rate limiting can serve as a formidable barrier against DoS attacks. By restricting the number of requests a user can make in a specific timeframe, you deflect flood attempts.
Using a Filter for Rate Limiting
Implement a simple Java filter in your Tomcat web application to control request rates.
import javax.servlet.*;
import javax.servlet.http.HttpServletRequest;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
public class RateLimitFilter implements Filter {
private static final long MAX_TIME = TimeUnit.SECONDS.toMillis(1);
private long lastRequestTime;
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletRequest httpRequest = (HttpServletRequest) request;
long currentTime = System.currentTimeMillis();
if (currentTime - lastRequestTime < MAX_TIME) {
response.getWriter().write("Too Many Requests");
return; // Block further processing
}
lastRequestTime = currentTime;
chain.doFilter(request, response);
}
public void init(FilterConfig filterConfig) {}
public void destroy() {}
}
Why?
This filter prevents two requests from the same user within a 1-second interval, showcasing a straightforward yet effective deterrent.
Session Management Best Practices
To mitigate session fixation risks, ensure that your sessions are securely managed.
Secure Session Attributes
Add secure flags to your session configurations:
<Context path="" docBase="yourApp" reloadable="true">
<Manager sessionCookiePath="/secure"
sessionCookieHttpOnly="true"
sessionCookieSecure="true"/>
</Context>
Why?
These configurations ensure that session cookies are only sent over HTTPS and are inaccessible to JavaScript.
Firewall Configurations
Consider setting up a firewall to filter incoming traffic. Use tools like iptables on Linux servers or services such as AWS Security Groups or Cloudflare to shield your Tomcat server from unwanted requests.
Basic iptables Rule:
sudo iptables -A INPUT -p tcp --dport 8080 -m conntrack --ctstate NEW,ESTABLISHED -m limit --limit 1/minute -j ACCEPT
Why?
This rule limits new incoming connections, helping to preempt potential DoS scenarios.
Application Performance Monitoring
To detect anomalies indicative of a DoS attack, monitor your application consistently.
Using Tools like JavaMelody
JavaMelody is a monitoring tool specifically designed for Java applications. You can integrate it easily with your Tomcat application:
- Add JavaMelody as a dependency.
- Configure it in web.xml to watch your application.
- Access JavaMelody’s dashboard to see metrics.
Why?
By keeping an eye on your environment’s metrics, you can identify unusual spikes in traffic early.
Load Balancing Solutions
In high-traffic applications, implementing a load balancer can distribute incoming requests across multiple Tomcat instances, reducing the likelihood of a single point of failure.
Example Configuration Using NGINX:
http {
upstream app_servers {
server app1.example.com;
server app2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://app_servers;
}
}
}
Why?
Balancing requests among several servers increases fault tolerance and minimalizes risk exposure during large traffic spikes.
Bringing It All Together
Securing your Apache Tomcat server against Denial of Service attacks is vital in today's digital landscape. Each of the strategies discussed contributes substantially to establishing a robust defense. From keeping your software updated to implementing rate limiting and effective session management, all constitute best practices every developer should prioritize.
For further reading, you may want to check out the Apache Tomcat Security Information page, which provides ongoing updates and insights.
By following the outlined strategies, you can mitigate the risk of DoS attacks and uphold the availability of your applications. Staying proactive in your server management will prevent you from becoming another statistic in the ever-evolving world of cyber threats.
Checkout our other articles