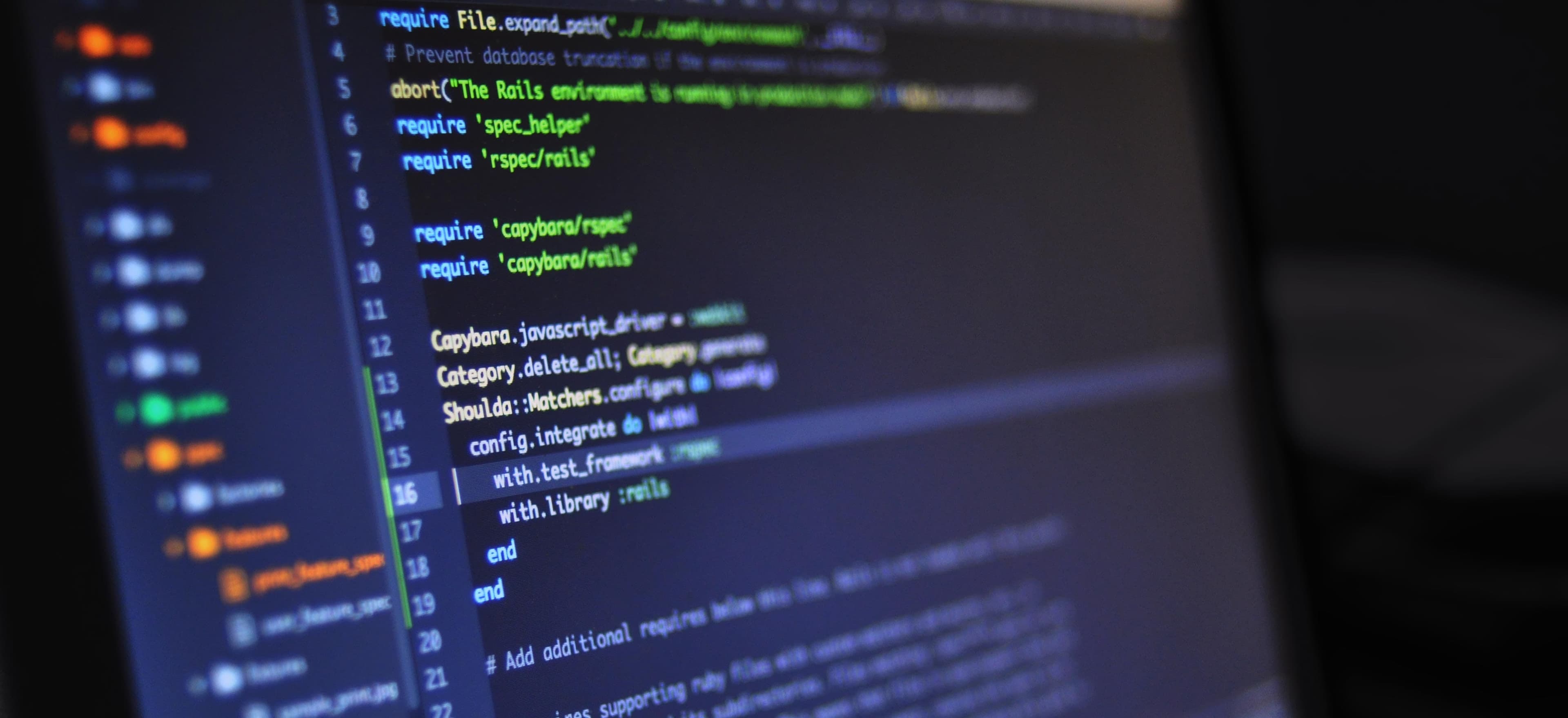
- Published on
Top Tips for Securing Your Database from Cyber Threats
In an era where data breaches are rampant, securing databases has never been more critical. Unauthorized access to sensitive data can severely impact an organization's reputation and finances. In this blog post, we will explore practical tips to help you bolster your database security against various cyber threats.
Understanding Threats to Your Database
Before diving into security tips, it's crucial to recognize the common types of threats your database may face:
- SQL Injection: Attackers can manipulate SQL queries to gain unauthorized access.
- Data Breaches: Sensitive data may be exposed due to poor security practices.
- Malicious Insiders: Employees with access can pose significant risks.
- Misconfigured Database Servers: Default settings can leave your database vulnerable.
- Backup Vulnerabilities: Insecure backups can be exploited, leading to data loss.
Awareness of these threats serves as a foundation for developing effective security strategies.
1. Implement Strong Authentication Mechanisms
One of the first lines of defense against unauthorized access is authentication. Consider the following strategies:
Use Multi-Factor Authentication (MFA)
Enabling MFA significantly reduces the chances of unauthorized access. By requiring multiple forms of identity verification, you add a layer of security.
Example Code: Enforcing MFA in Java
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public void enforceMFA(HttpServletRequest request, HttpServletResponse response) throws ServletException {
boolean mfaEnabled = true; // check if MFA is required
if (mfaEnabled && !isMfaCompleted(request)) {
response.sendRedirect("mfa-challenge.jsp");
}
}
Why This Matters: By redirecting users who haven't completed MFA, you ensure that only valid users gain access to your database.
2. Use Encryption
Data encryption is paramount for protecting sensitive information. Encrypting your database can protect your data both at rest and in transit.
Transform Your Data with AES Encryption
The Advanced Encryption Standard (AES) is a robust standard for encrypting data. Here’s how you can encrypt user information:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
public class EncryptionUtil {
private static final String ALGORITHM = "AES";
public static byte[] encrypt(String data, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(data.getBytes());
}
public static SecretKey generateKey() throws Exception {
KeyGenerator keyGen = KeyGenerator.getInstance(ALGORITHM);
keyGen.init(128); // for example, using a 128-bit key
return keyGen.generateKey();
}
}
Why This Matters: Encrypting sensitive data ensures that even if an unauthorized party accesses the database, the information remains unreadable.
3. Regularly Update and Patch Database Software
Cyber threats evolve rapidly, and so must your database systems. Regularly updating your database software is essential in reducing vulnerabilities.
Automate Patching
Many database systems offer automated patching features. This capability can help you stay ahead of security updates without a manual process.
Why This Matters: Keeping your database software updated means you’re protected against known vulnerabilities identified by the software vendor.
4. Implement Role-Based Access Control (RBAC)
Restricting access based on user roles ensures that employees only have access to the data necessary for their jobs.
Example of Implementing RBAC
Below is a basic demonstration of how to implement RBAC in a Java application.
import java.util.HashMap;
import java.util.Map;
public class AccessControl {
private Map<String, String> userRoles = new HashMap<>();
public void assignRole(String username, String role) {
userRoles.put(username, role);
}
public boolean hasAccess(String username, String requiredRole) {
return userRoles.get(username).equals(requiredRole);
}
}
Why This Matters: By controlling access on a need-to-know basis, you significantly reduce the chances of data being compromised by insiders.
5. Regularly Back Up Your Data
Regular backups are essential for recovery in case of data loss or a breach. Implement a backup strategy that ensures your data is both encrypted and stored securely.
Best Practices for Database Backups
- Automate Backups: Set up automated backup schedules.
- Offsite Backups: Store backups in a secure, offsite location.
- Test Recovery Procedures: Regularly test your ability to recover from backups.
Why This Matters: Having a secure backup ensures data availability even after a cyber incident, thereby minimizing downtime.
6. Monitor Database Activity
Keeping an eye on what's happening in your database is fundamental for identifying suspicious activities.
Implement Logging
Utilizing logging can provide insights into database operations. A simple way to log activity is shown below:
import java.io.FileWriter;
import java.io.IOException;
public class ActivityLogger {
public static void logEvent(String message) {
try (FileWriter writer = new FileWriter("database_activity.log", true)) {
writer.write(message + "\n");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why This Matters: Logging provides transparency for operations and helps identify potential breaches before significant damage can occur.
7. Use Firewalls and Security Groups
Database firewalls and security groups act as a barrier against unauthorized access.
Configure a Database Firewall
Most database management systems (DBMS) allow you to implement rules for inbound and outbound traffic.
Why This Matters: A configured firewall can block unwanted access and improve overall security posture.
8. Conduct Regular Security Audits
Lastly, conducting regular security audits can help identify potential vulnerabilities and areas for improvement.
Manual and Automated Audits
Consider using both manual review processes and automated security tools to assess your database security.
Why This Matters: Regular audits keep your security measures aligned with the ever-evolving landscape of cybersecurity threats.
Closing the Chapter
Database security is a multifaceted challenge that requires continued attention. By implementing these top tips, you can significantly reduce the risk of cyber threats targeting your databases. Always remember that cybersecurity is a journey, not a destination; continuous education and proactive measures are essential to staying secure.
For more detailed guidance on database security, consider resources such as the OWASP Cheat Sheet or the National Institute of Standards and Technology (NIST).
If you found this post valuable, please share it with your network, and stay proactive about your database security!
Checkout our other articles