Mastering Data Consistency in Microservices with Spring Boot
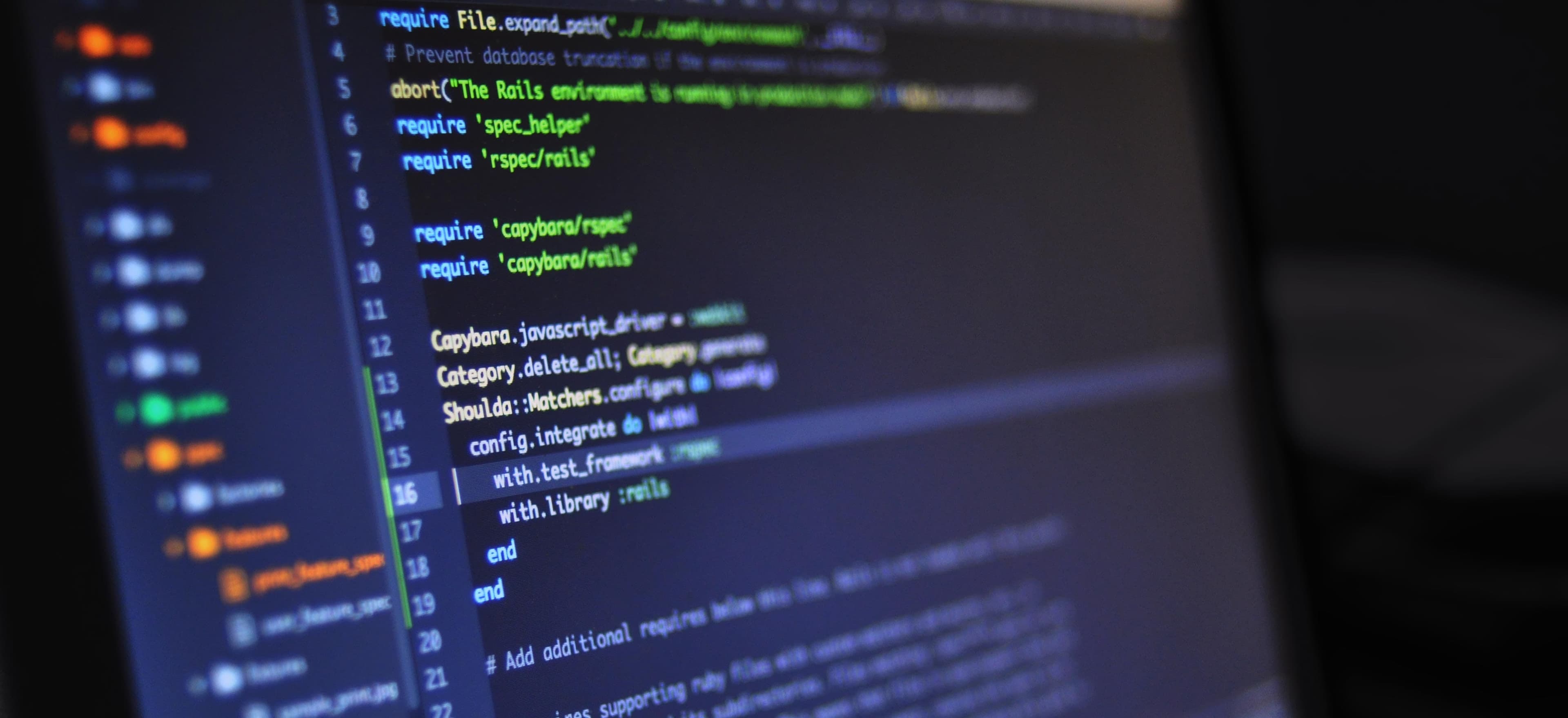
- Published on
Mastering Data Consistency in Microservices with Spring Boot
In the realm of modern software architecture, microservices have emerged as a boon for creating resilient and scalable applications. However, managing data consistency across these independently deployable services can be a formidable challenge. In this blog post, we will explore several strategies and best practices for achieving data consistency when building microservices with Spring Boot.
What is Data Consistency?
Data consistency refers to the state of data being reliable and uniform across multiple services. In a microservices architecture, where each service can have its own separate database, ensuring data consistency becomes a critical concern. Without effective strategies, you may end up with different states of the same data across services, leading to a fragmented application.
Types of Data Consistency
-
Strong Consistency: Guarantees that any read operation receives the most recent write operation. This is the strictest form of consistency, but it can introduce significant latency.
-
Eventual Consistency: Guarantees that eventual reads will reflect the most recent writes. This model is often more forgiving and suitable for systems that can tolerate some time lag.
-
Causal Consistency: This model allows services to see updates in the order they occurred, which can be useful in collaborative environments.
Understanding these types of consistency is imperative in choosing a suitable strategy for your application.
Strategies for Managing Data Consistency
1. Sagas
Sagas are a design pattern for microservices that handle distributed transactions. Instead of a single database transaction spanning multiple services, sagas allow each service to perform its operations independently but maintain a consistent state overall.
Example of a Saga Implementation in Spring Boot
Here’s a brief look at how you might implement a saga using Spring Boot with the help of the Spring Cloud Data Flow
framework.
import org.springframework.web.bind.annotation.*;
import java.util.UUID;
@RestController
@RequestMapping("/saga")
public class OrderSagaController {
@PostMapping("/createOrder")
public String createOrder(@RequestBody Order order) {
// Step 1: Create an order and save it
String orderId = UUID.randomUUID().toString();
// orderService.save(orderId, order); // Simulated save operation
// Step 2: Reserve items
// itemService.reserveItems(orderId, order.getItems()); // Simulated operation
// Step 3: Initiate payment
// paymentService.processPayment(orderId, order.getTotal()); // Simulated operation
return "Order created successfully with ID: " + orderId;
}
}
In this pseudo-code, we simulate a saga for creating an order. Each of the components (order, item, payment) operates independently. If any step fails, we can roll back previous steps.
2. Event Sourcing
In event sourcing, state changes are stored as a sequence of events instead of the current state. Every service emits events, and other services can react to these events, ensuring that all services have the latest state reflected from the sequence of events.
Example of Event Source Implementation
Consider this pseudo-code implementation in a Spring Boot application using Spring Cloud Stream
.
import org.springframework.cloud.stream.annotation.EnableBinding;
import org.springframework.messaging.support.MessageBuilder;
@EnableBinding(OrderProcessor.class)
public class OrderService {
private final OrderProcessor orderProcessor;
public OrderService(OrderProcessor orderProcessor) {
this.orderProcessor = orderProcessor;
}
public void createOrder(Order order) {
// Save the order to the database
orderRepository.save(order);
// Emit event
OrderCreatedEvent event = new OrderCreatedEvent(order.getId(), order.getTotal());
orderProcessor.output().send(MessageBuilder.withPayload(event).build());
}
}
In this example, OrderService
saves an order in the database, then emits an OrderCreatedEvent
to notify other microservices. This ensures that any state change is consistently communicated across all services.
3. Two-Phase Commit (2PC)
Two-Phase Commit is a more traditional approach to distributed transactions. It involves a coordinator that ensures all participating services either commit or abort the transaction.
Example of 2PC Implementation
Typically, you wouldn't implement a 2PC at the application code level but rather use a framework that supports it, like JTA (Java Transaction API). Here is a high-level overview:
import javax.transaction.*;
public class OrderTransactionManager {
@Resource
private UserTransaction userTransaction;
public void processTransaction(Order order) {
try {
userTransaction.begin();
// Process order
// orderService.create(order);
// paymentService.process(order);
userTransaction.commit();
} catch (Exception e) {
userTransaction.rollback();
}
}
}
Note: While 2PC provides strong consistency, it can be costly in terms of performance and is rarely used in microservices due to its blocking nature.
Best Practices for Data Consistency
-
Use Idempotence: Ensure that repeated requests yield the same outcome, thus avoiding inconsistent states due to retries.
-
Versioning: Use an event versioning system to manage schema changes in your data. This ensures that services can handle events properly without breaking changes.
-
Fallback Mechanisms: Design your services to gracefully handle exceptions and timeouts to maintain a better user experience without compromising data consistency.
-
Leverage Distributed Traceability: Utilize tools like Sleuth and Zipkin to monitor requests going through various services, which ensures data integrity and offers insights into performance issues.
The Closing Argument
Achieving data consistency in a microservices architecture using Spring Boot can be daunting, but with the right strategies, it becomes manageable. Sagas, event sourcing, and two-phase commit offer various levels of consistency suited to different needs.
Understanding these mechanisms, along with best practices, allows you to create robust microservices that not only function efficiently but also maintain reliable and consistent data across the entire application.
For further reading, consider exploring these additional resources:
- Spring Cloud Data Flow
- Event Sourcing with Spring
By mastering data consistency in microservices, you set the foundation for a more reliable and maintainable application architecture. Happy coding!
Checkout our other articles