Mastering Tutorial Unit Tests: Common Pitfalls to Avoid
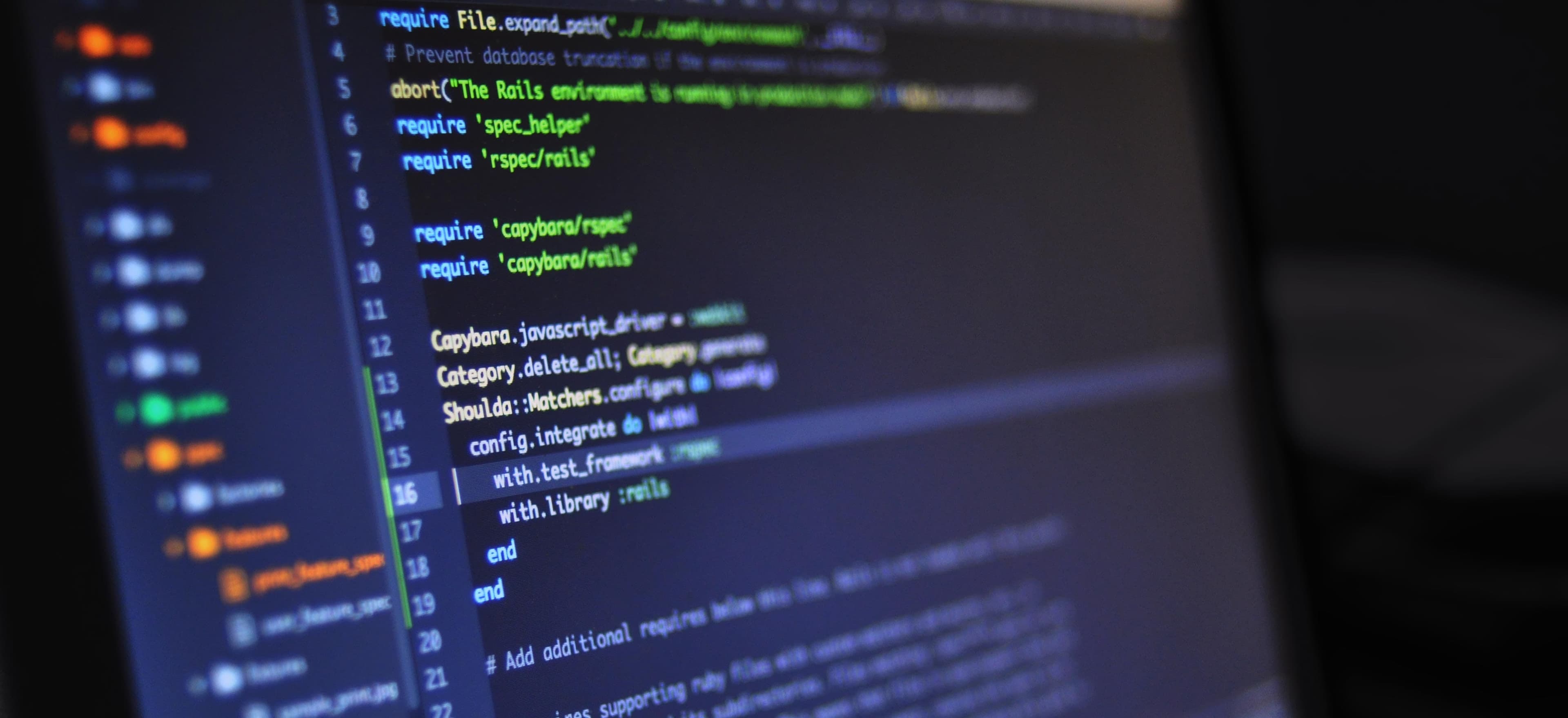
- Published on
Mastering Tutorial Unit Tests: Common Pitfalls to Avoid
Setting the Scene
Unit testing is a cornerstone of software development. It allows developers to verify that individual components of their code work as intended. However, as straightforward as it seems, writing effective unit tests isn't always smooth sailing. In this blog post, we will explore common pitfalls to avoid in unit testing, helping you to elevate both the quality of your code and your testing skills.
What are Unit Tests?
Unit tests are automated tests that confirm that a specific section of code (usually a function or method) behaves as expected. In Java, JUnit is the most widely used framework for writing unit tests. Here's a quick example:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
In this example, a simple Calculator
class is tested to ensure the add
method returns the correct result. This is a basic yet effective structure to demonstrate unit testing.
Common Pitfalls in Unit Testing
1. Over-Testing or Under-Testing
One of the primary pitfalls when writing unit tests is the imbalance of coverage. Over-testing can lead to bloated test suites that are hard to maintain, while under-testing can leave your code vulnerable to bugs.
Balancing the Coverage
To find the sweet spot, focus on the most critical components of your application. Test for:
- Business logic
- Edge cases
- Integration points with other systems
2. Ignoring Setup and Teardown Methods
JUnit offers @BeforeEach
and @AfterEach
annotations for setup and teardown methods which can save you from repetitive code. Ignoring these can lead to duplicated code and harder-to-maintain tests.
Example
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.AfterEach;
public class CalculatorTest {
private Calculator calculator;
@BeforeEach
public void setUp() {
calculator = new Calculator();
}
@AfterEach
public void tearDown() {
calculator = null; // Helps with memory management
}
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3));
}
}
3. Test Independence
Each unit test should be independent. Tests can easily influence each other, causing flakiness. If one test fails, it can skew results from others if they don’t reset their state.
How to Ensure Independence
- Utilize setup and teardown methods.
- Avoid shared static states.
- Use mocks for external dependencies.
4. Misusing Assertions
Assertions should clearly express the conditions being validated. Overly complex assertions can cause confusion and obscure the intent of the test.
Best Practices for Assertions
- Use descriptive messages on failures.
- Keep assertions simple and focused.
- Aim for a single responsibility per test method.
Example of Effective Assertion
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class UserTest {
@Test
public void testUserCreation() {
User user = new User("Alice", "alice@example.com");
assertAll("User should be created with correct properties",
() -> assertEquals("Alice", user.getName()),
() -> assertEquals("alice@example.com", user.getEmail())
);
}
}
5. Not Isolating External Dependencies
Unit tests should be isolated from external systems like databases or web services. Using mocks or stubs will allow you to simulate these dependencies without requiring an actual connection.
Mocking Example
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testGetUser() {
UserRepository mockRepo = mock(UserRepository.class);
UserService userService = new UserService(mockRepo);
User dummyUser = new User("Alice", "alice@example.com");
when(mockRepo.findById(1)).thenReturn(dummyUser);
User user = userService.getUser(1);
assertEquals("Alice", user.getName());
}
}
6. Not Running Tests Regularly
Unit tests are wasted if they are only run once at the end of development. Incorporating testing regularly in your development cycle using Continuous Integration (CI) tools can save headaches down the road.
Why Continuous Testing Matters
- Catches issues early when they are easier to fix.
- Encourages developers to write better tests.
- Provides immediate feedback on code quality.
7. Poor Test Readability
Code quality applies to tests as well. A unit test should read similarly to a well-written piece of literature. Obscure or verbose tests can lead to misinterpretation of the intent or function being tested.
Enhancing Readability
- Keep test names descriptive.
- Use the
@DisplayName
annotation to provide context. - Divide larger tests into smaller auxiliary methods.
Example of a Readable Test
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class RegistrationServiceTest {
@Test
@DisplayName("Should throw exception for duplicate email registration")
public void testDuplicateEmail() {
RegistrationService service = new RegistrationService();
service.register("alice@example.com");
assertThrows(EmailAlreadyExistsException.class, () ->
service.register("alice@example.com"),
"Exception should be thrown for duplicate email"
);
}
}
A Final Look
Mastering unit tests is an ongoing journey fraught with challenges. By avoiding the common pitfalls outlined in this blog post, you will not only write more effective unit tests but also improve the overall design and reliability of your code.
Unit testing is not just about achieving coverage; it’s about enhancing the maintainability and quality of your software. Dive into the world of TDD (Test-Driven Development), explore frameworks, and make unit testing an integral part of your development process.
For further reading, you may consider checking these resources:
- JUnit 5 User Guide
- Effective Unit Testing
By learning from the common pitfalls, your experience with unit tests will be smoother and your applications, more resilient. Happy testing!
Checkout our other articles