Mastering Unit Testing: Overcoming LocalTestServer Challenges
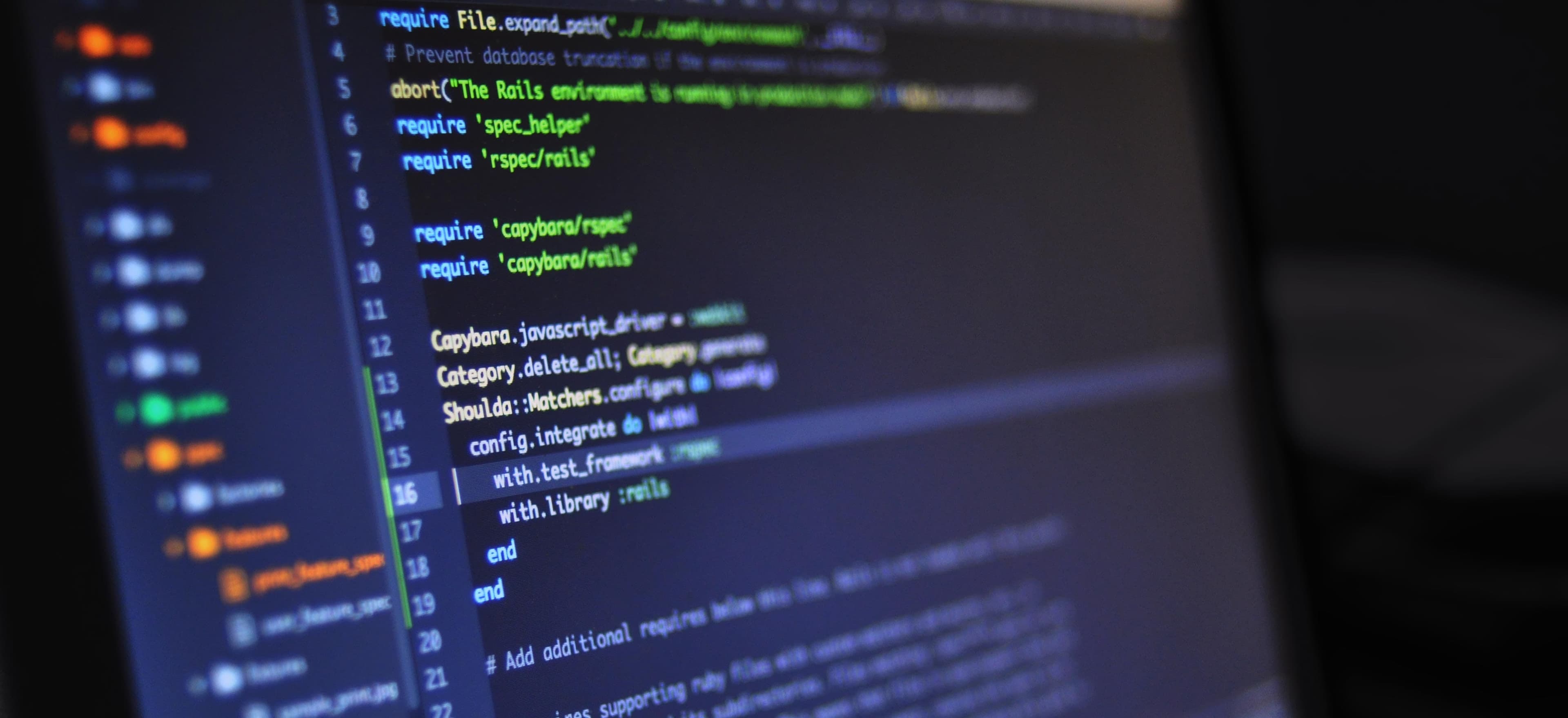
- Published on
Mastering Unit Testing: Overcoming LocalTestServer Challenges
Unit testing is a critical phase in software development that ensures each part of a program is working as intended. It can catch bugs early, enhance code quality, and streamline the development process. However, when it comes to unit testing applications that depend on local server environments, developers often face unique challenges. This post will guide you through overcoming these challenges, particularly while using Java, with a focus on LocalTestServer.
Understanding LocalTestServer
LocalTestServer is an abstraction layer for working with web servers during unit testing. It's widely used to mock HTTP requests and responses. This allows for testing without needing an actual server running, making unit tests faster and ensuring they can run in isolation.
However, successfully implementing it can be fraught with difficulties such as configuration issues, managing server states, and dealing with dependencies. Let's take a closer look at these challenges and how you can overcome them.
Common Challenges with LocalTestServer
-
Configuration Issues: Setting up a LocalTestServer can be tricky. If not configured correctly, tests may fail inconsistently or require significant time to set up.
-
Server State Management: Keeping track of the server state, especially between tests, can lead to problems. If one test modifies the server state, it may inadvertently affect others.
-
Dependencies: Mocking external services or databases can sometimes be challenging and lead to brittle tests.
Best Practices for Using LocalTestServer
Here are several best practices to help you overcome challenges while using LocalTestServer in your unit tests.
1. Proper Configuration
When using LocalTestServer, proper configuration is paramount for success. You can use JUnit to set up your tests. Below is an example of how to configure a LocalTestServer.
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
public class MyServerTest {
private LocalTestServer server;
@Before
public void setUp() {
// Start the LocalTestServer with a specific port
server = new LocalTestServer();
server.start(8080);
}
@After
public void tearDown() {
// Ensure the server is stopped after tests
server.stop();
}
@Test
public void testEndpoint() throws Exception {
// Example of how to test an endpoint
HttpGet request = new HttpGet(server.getUrl() + "/some-endpoint");
HttpResponse response = server.execute(request);
assertEquals(200, response.getStatusLine().getStatusCode());
// Additional assertions based on expected response
}
}
Why this matters: The @Before
and @After
annotations ensure that your server starts and stops appropriately for every test. This encapsulation is essential for maintaining a consistent test state, avoiding pitfalls associated with server state management.
2. Isolate Tests with Unique States
To ensure that tests do not interfere with one another, make sure each one starts from a clean state. You can achieve this by using a fresh instance of LocalTestServer for each test case.
@Test
public void testAnotherEndpoint() throws Exception {
// Create another unique server instance
LocalTestServer anotherServer = new LocalTestServer();
anotherServer.start(8081);
try {
HttpGet request = new HttpGet(anotherServer.getUrl() + "/another-endpoint");
HttpResponse response = anotherServer.execute(request);
assertEquals(200, response.getStatusLine().getStatusCode());
} finally {
anotherServer.stop(); // Always stop server instance
}
}
Why this matters: By creating separate instances for each test, you diminish the risks of shared states and ensure that each test can run independently, which is a core principle of unit testing.
3. Mocking Dependencies
When unit testing, don’t forget about mocking external dependencies. This includes databases, message queues, or other web services. Libraries like Mockito can significantly ease this process. Here’s an example:
import static org.mockito.Mockito.*;
// Mocking a DatabaseService
DatabaseService mockDbService = mock(DatabaseService.class);
// Setting up the behavior of the mock
when(mockDbService.getData()).thenReturn(someData);
// Use mockDbService in your test
MyService service = new MyService(mockDbService);
assertEquals(someData, service.fetchData());
Why this matters: Mocking reduces external dependencies that could lead to unstable tests. You maintain greater control over your test environment and can replicate various scenarios without much complexity.
Leveraging Libraries and Tools
Using tools such as JUnit for testing and Mockito for mocking can streamline your unit testing process significantly.
-
JUnit: Simplifies the test process through annotations and a straightforward structure.
-
Mockito: Provides a powerful way to create mock objects and stub behavior, allowing you to isolate the class under test from its dependencies.
The Bottom Lines
Mastering unit testing with LocalTestServer can significantly improve the reliability and speed of your tests in Java. While challenges such as configuration issues, server state management, and dependency mocking may arise, employing best practices can help you overcome them effectively.
Investing time in setting up a robust unit testing environment can lead to greater confidence in your code's quality and functionality in the long term. As you refine your practices, remember that unit tests are not just a box to check—they are a crucial part of building maintainable and scalable software solutions.
Further Reading & Resources
- Effective Unit Testing by Elisha Sacks
- JUnit 5 User Guide
- Mockito: Simulate Python Unit Tests
Unit testing is an art, and mastering it might take some time. But remember that every line of code you write belongs to an ongoing conversation with the surrounding code base. Happy testing!