Avoiding Common Mistakes in Database Normalization
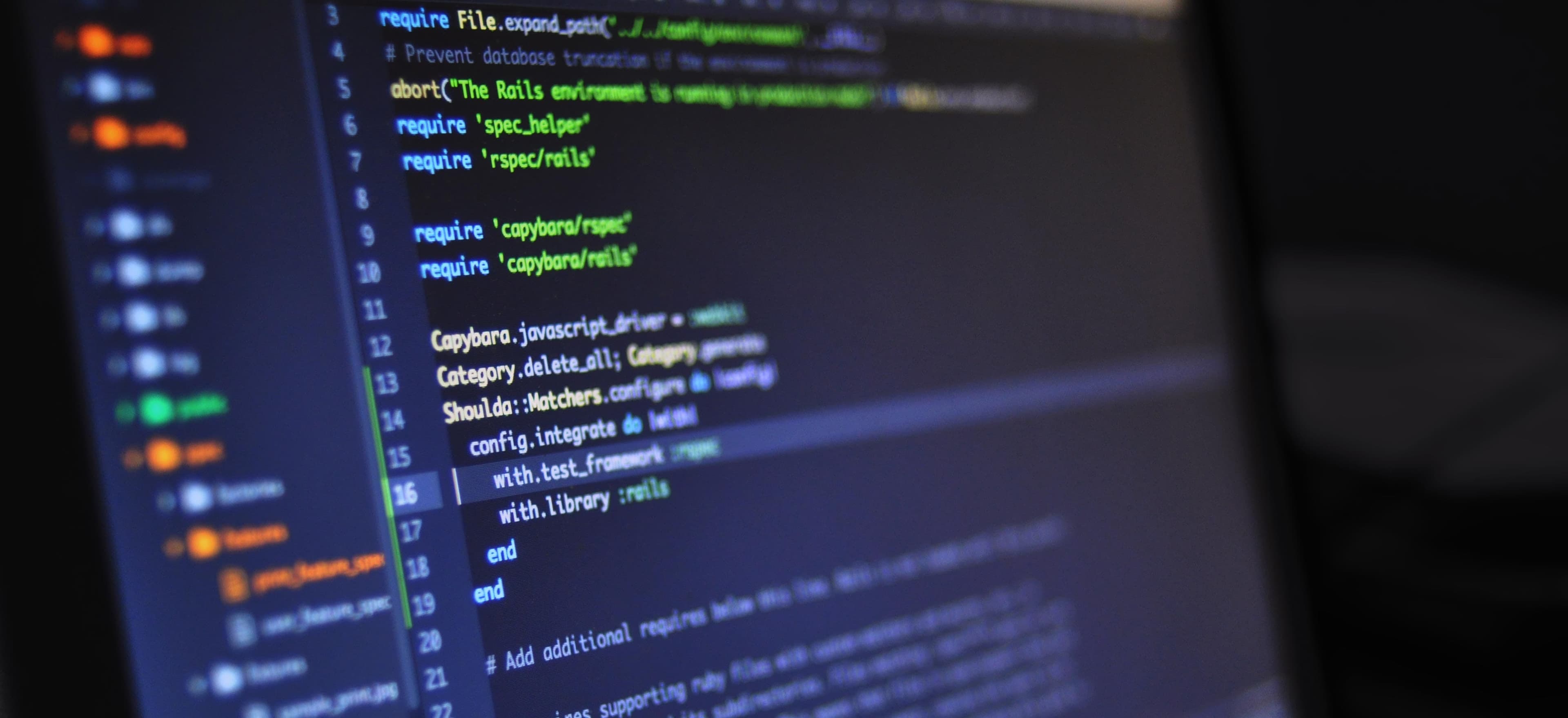
- Published on
Avoiding Common Mistakes in Database Normalization
Database normalization is an essential practice in database design that enhances data integrity and reduces redundancy. However, many developers stumble upon common pitfalls during this valuable process. In this blog, we'll delve into the various normalization forms, understand their significance, and highlight frequent mistakes, ensuring you can avoid them in your database designs.
What is Database Normalization?
Database normalization is a systematic approach to organizing data in a database. The main goals are to eliminate redundancy and ensure data dependencies make sense, contributing to data integrity. To accomplish this, normalization is divided into several "normal forms," each with specific rules.
Types of Normal Forms
- First Normal Form (1NF): Ensures that each column contains atomic values, and each record is unique.
- Second Normal Form (2NF): Ensures that all non-key attributes are fully functional dependent on the primary key.
- Third Normal Form (3NF): Eliminates transitive dependency, ensuring non-key attributes are dependent only on the primary key.
- Boyce-Codd Normal Form (BCNF): A stronger version of the 3NF that addresses some issues not covered by the third form.
With that said, let's now explore common mistakes that developers make at each normalization stage and tips on avoiding them.
Common Mistakes in Database Normalization
1. Overlooking 1NF Requirements
Mistake Explanation
One of the most prevalent mistakes is failing to ensure that each table is in the first normal form. This means allowing multiple values per attribute in the same column—this violates the principle of atomicity.
Example Code Snippet
CREATE TABLE Students (
StudentID INT,
Name VARCHAR(100),
PhoneNumbers VARCHAR(255) -- Allows multiple phone numbers
);
In the example above, the PhoneNumbers
column violates 1NF because it attempts to store multiple phone numbers in a single field.
Solution
To address this, we should create a separate table for phone numbers:
CREATE TABLE Students (
StudentID INT PRIMARY KEY,
Name VARCHAR(100)
);
CREATE TABLE PhoneNumbers (
PhoneID INT PRIMARY KEY,
StudentID INT,
PhoneNumber VARCHAR(15),
FOREIGN KEY (StudentID) REFERENCES Students(StudentID)
);
By creating a one-to-many relationship, each student can now have multiple phone numbers while adhering to 1NF.
2. Ignoring Functional Dependency in 2NF
Mistake Explanation
In 2NF, a common oversight is neglecting the principle of functional dependency. A non-key attribute must depend on the entire primary key when it consists of multiple columns.
Example Code Snippet
CREATE TABLE CourseEnrollments (
EnrollmentID INT PRIMARY KEY,
StudentID INT,
CourseID INT,
StudentName VARCHAR(100) -- Non-key attribute depending on part of the composite key
);
Here, StudentName
is dependent only on StudentID
and not the composite key.
Solution
To achieve 2NF, we should split the CourseEnrollments
table:
CREATE TABLE Students (
StudentID INT PRIMARY KEY,
StudentName VARCHAR(100)
);
CREATE TABLE CourseEnrollments (
EnrollmentID INT PRIMARY KEY,
StudentID INT,
CourseID INT,
FOREIGN KEY (StudentID) REFERENCES Students(StudentID)
);
3. Falling Short on 3NF: Transitive Dependencies
Mistake Explanation
A critical mistake made during normalization is failing to eliminate transitive dependencies in 3NF. Transitive dependency occurs when one non-key attribute depends on another non-key attribute.
Example Code Snippet
CREATE TABLE Students (
StudentID INT PRIMARY KEY,
StudentName VARCHAR(100),
DepartmentID INT,
DepartmentName VARCHAR(100) -- Transitive dependency on DepartmentID
);
In this case, DepartmentName
is dependent on DepartmentID
, not directly on StudentID
.
Solution
We can resolve this by creating a separate Departments
table:
CREATE TABLE Departments (
DepartmentID INT PRIMARY KEY,
DepartmentName VARCHAR(100)
);
CREATE TABLE Students (
StudentID INT PRIMARY KEY,
StudentName VARCHAR(100),
DepartmentID INT,
FOREIGN KEY (DepartmentID) REFERENCES Departments(DepartmentID)
);
This way, we remove the transitive dependency and keep our design clean.
4. BCNF Issues: Overlooking Candidate Keys
Mistake Explanation
Many designers ignore the potential existence of multiple candidate keys. This can lead to violations of BCNF if dependencies exist that violate the principle of fully functional dependence on a candidate key.
Example Code Snippet
CREATE TABLE Lectures (
LectureID INT PRIMARY KEY,
CourseID INT,
InstructorID INT,
InstructorName VARCHAR(100) -- Potential BCNF violation
);
In this scenario, InstructorName
depends on InstructorID
, rather than LectureID
.
Solution
To correct this, separate the instructors into their own table:
CREATE TABLE Instructors (
InstructorID INT PRIMARY KEY,
InstructorName VARCHAR(100)
);
CREATE TABLE Lectures (
LectureID INT PRIMARY KEY,
CourseID INT,
InstructorID INT,
FOREIGN KEY (InstructorID) REFERENCES Instructors(InstructorID)
);
This update adheres to BCNF by making sure that non-key attributes depend solely on their respective key.
Final Considerations
By finalizing our discussions on the common mistakes made during database normalization, we can see how critical it is to follow the normalization process diligently. Proper normalization ensures data integrity, improves query performance, and saves space.
Remember, successful database design goes beyond implementing complex structures; it necessitates a deep understanding of how data relates and ensuring clarity amidst the architecture. You can always refer to this article on normalization for deeper insights and examples.
Ultimately, a well-structured database will pay dividends in the long run, fostering scalability and efficiency in your applications. So, embrace normalization principles to enhance your data architectures while steering clear of the common pitfalls discussed in this guide. Happy coding!