Navigating Jigsaw's Challenges in JavaFX Development
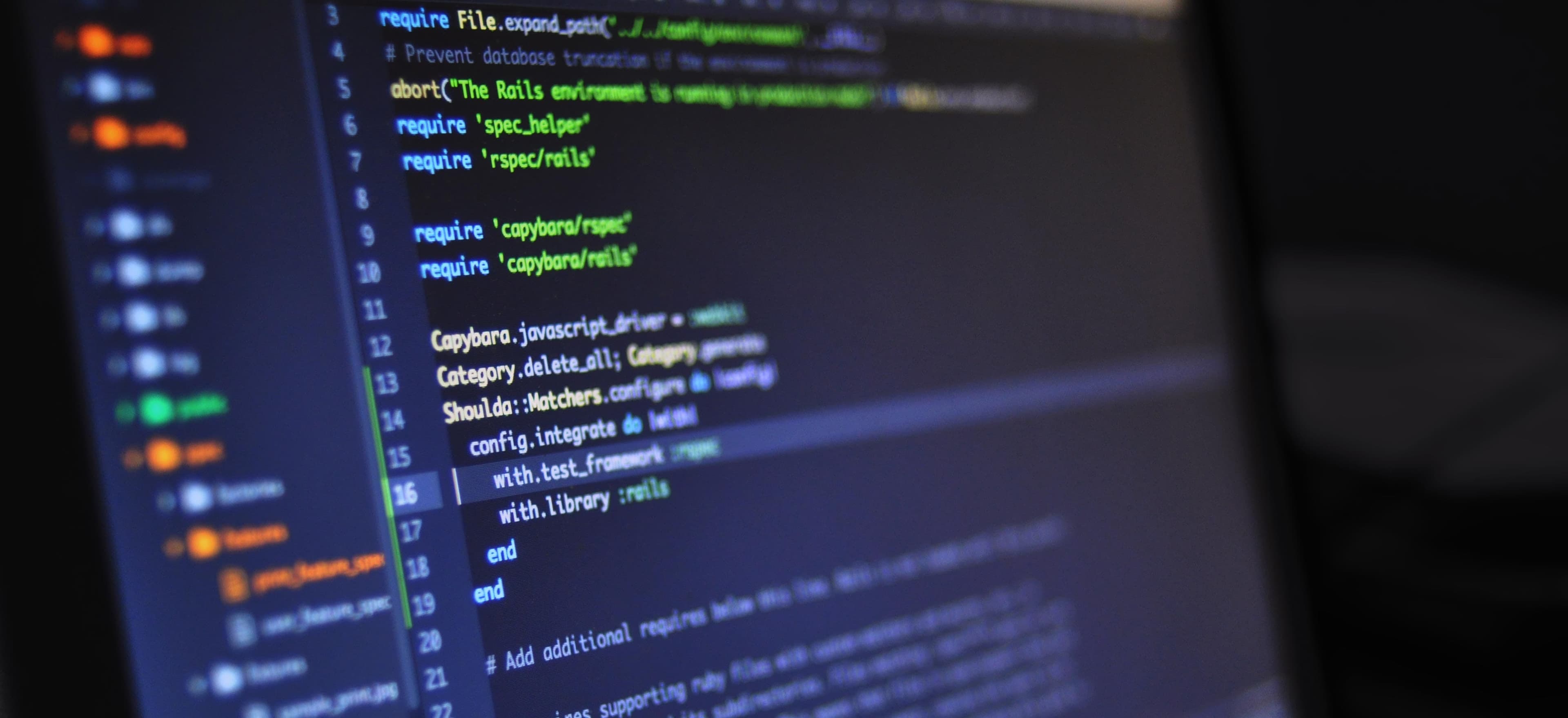
- Published on
Navigating Jigsaw's Challenges in JavaFX Development
JavaFX has become a go-to framework for building performant and aesthetic user interfaces in Java applications. However, with the introduction of the Java Platform Module System (JPMS), often referred to as Jigsaw, JavaFX developers have encountered new challenges. In this blog post, we will discuss these challenges, and how to effectively navigate them, while providing clear and practical examples.
Understanding JavaFX and Jigsaw
JavaFX, a part of the Java ecosystem since Java 8, is designed to facilitate the creation of modern user interfaces. Jigsaw, introduced with Java 9, allows developers to modularize their applications, enhancing security and maintainability. However, the modular system also introduces complexities, particularly for frameworks like JavaFX that were originally developed without modularization in mind.
Why Jigsaw?
The primary aim of Jigsaw is to improve the structure of Java applications by allowing developers to:
- Encapsulate code
- Control dependencies
- Improve performance through better optimization
Yet, using Jigsaw in conjunction with JavaFX can create intricate hurdles, especially when dealing with module declarations, dependencies, and runtime issues.
Key Challenges in JavaFX with Jigsaw
1. Module Declarations
One of the most noticeable changes is the necessity to declare modules. If you are accustomed to using JavaFX in a non-modular setup, the first step of modularization may feel daunting. Each module must explicitly state its dependencies.
Example of Module Declaration
module my.application {
requires javafx.controls;
requires javafx.fxml;
opens my.application to javafx.fxml;
}
Commentary:
In the above code snippet, we declare a module named my.application
. The requires
keyword specifies the JavaFX modules our application depends on. The opens
directive allows for reflection, which is necessary when using FXML files to load UI components.
2. Packaging and Deployment
JavaFX applications packaged with Jigsaw can experience issues related to how Jigsaw packages modules dynamically. When creating an executable JAR file, you'll need to ensure all necessary JavaFX modules are included.
How to Package Your Application
- Create a modular JAR file for your application.
- Include necessary JavaFX modules:
You can include JavaFX modules in your build tool configuration (like Maven or Gradle) or use the
jlink
tool to package and create a custom runtime image.
Example using Maven
In your pom.xml
, you can define the JavaFX dependencies:
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>17.0.0</version>
</dependency>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-fxml</artifactId>
<version>17.0.0</version>
</dependency>
Commentary:
Using Maven simplifies the module dependency management and keeps your project clean. Each dependency corresponds to a JavaFX module, ensuring you're covered with what you need.
3. Run-time Configuration
Configuring your application at runtime in a modular environment can introduce complexities. This is especially true when referencing JavaFX components through FXML.
To launch your application, you might typically type:
java --module-path path-to-javafx-sdk --add-modules my.application
Commentary:
It's imperative to include both the JavaFX SDK's module path and your application's module path. Failing to do so can lead to ClassNotFoundException
for JavaFX components, as they won’t be resolved against the runtime module path.
Tips for Effective JavaFX Development with Jigsaw
1. Thoroughly Understand Module Declarations
Take the time to fully comprehend your module dependencies. Ensure every package that requires reflection is properly declared with opens
. This practice not only aids legibility but also helps prevent runtime exceptions.
2. Build System Integration
Utilize build systems like Maven or Gradle to manage dependencies. They manage transitive dependencies and help package your JavaFX application seamlessly.
3. Debugging
When issues arise, leverage debugging tools effectively. Java's built-in jcmd
and JVisualVM can provide insights into module loading issues.
4. Stay Updated
As Java evolves, so too does its ecosystem. Regularly review the OpenJFX documentation and other community resources to stay current with best practices.
5. Example Application
To illustrate these principles, let's create a simple JavaFX application structured modularly.
Java Class: Main.java
package my.application;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
primaryStage.setTitle("JavaFX and Jigsaw");
primaryStage.setScene(new Scene(root, 300, 275));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
FXML File: sample.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.layout.VBox?>
<VBox alignment="center" spacing="20" xmlns:fx="http://javafx.com/fxml">
<Button text="Click Me!" onAction="#handleButtonClick"/>
</VBox>
Controller Class: Controller.java
package my.application;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
public class Controller {
@FXML
private void handleButtonClick(ActionEvent event) {
System.out.println("Button clicked!");
}
}
Commentary:
In this simple JavaFX application, you can observe the modular structure. Each part of the application is clearly defined, showcasing how to navigate JavaFX’s functionalities while leveraging Jigsaw’s modular architecture.
The Last Word
Navigating the challenges posed by Jigsaw within the context of JavaFX development can be overwhelming. However, by understanding module declarations, properly packaging your applications, and utilizing the powerful features of build systems, you can build robust applications that are clean and maintainable.
For more insights on JavaFX development and modular programming, check out the JavaFX documentation and the Java Platform Module System Overview.
Happy coding!