Mastering Dual-Pivot Quicksort for Better Performance
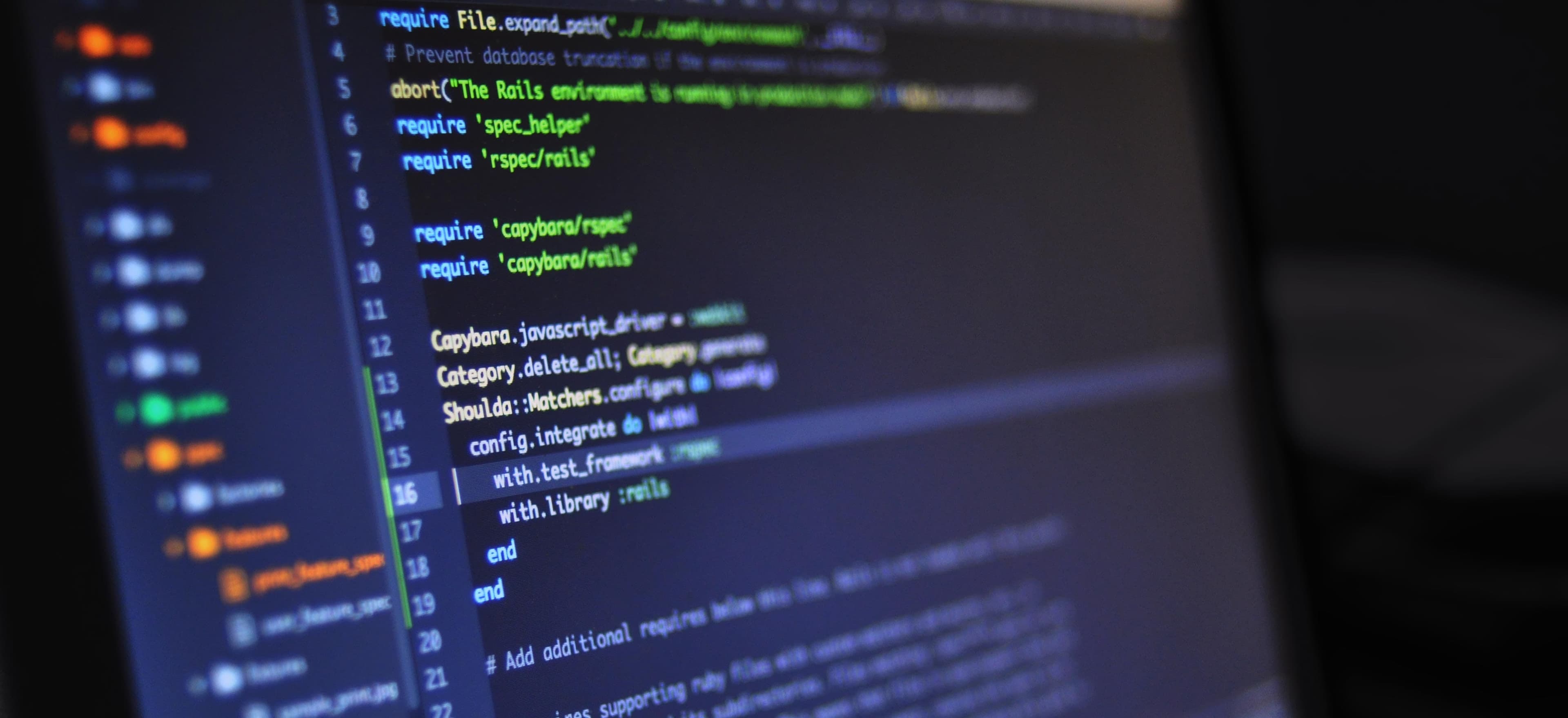
- Published on
Mastering Dual-Pivot Quicksort for Better Performance
In the realm of computer science, sorting algorithms play a crucial role in optimizing the performance of data processing tasks. Among various sorting methods, Quicksort often stands out due to its efficiency and speed. While the standard Quicksort algorithm is a powerful tool, an advancement known as Dual-Pivot Quicksort takes its performance to another level. In this blog post, we will explore Dual-Pivot Quicksort in depth—understanding its inner workings, its performance benefits, and how to implement it in your Java projects.
What is Quicksort?
Quicksort is a divide-and-conquer algorithm that sorts arrays by partitioning them into smaller subarrays. Below is a simplified explanation of the standard Quicksort algorithm:
- Choose a Pivot: Select a single element from the array as the pivot.
- Partitioning: Rearrange the array so that elements less than the pivot are on the left, and elements greater are on the right.
- Recursive Sorting: Recursively apply the same process to the left and right subarrays.
With a typical time complexity of O(n log n), Quicksort can perform exceptionally well compared to other algorithms. However, its performance can degrade to O(n²) in the worst case, particularly with poor pivot selections.
What is Dual-Pivot Quicksort?
Dual-Pivot Quicksort, introduced by Vladimir Yaroslavskiy in 2009, enhances the traditional Quicksort by using two pivots instead of one. This allows partitioning the array into three subarrays, leading to fewer comparisons and a more refined sorting process. Consequently, it often results in improved performance, especially with large datasets.
How Dual-Pivot Quicksort Works
The Dual-Pivot Quicksort operates as follows:
- Select Two Pivots: Choose two distinct values as the pivot elements.
- Three-way Partitioning: Rearrange the array into three segments:
- Elements less than the first pivot
- Elements between the two pivots
- Elements greater than the second pivot
- Recursive Sorting: Recursively sort the three segments.
This method reduces the number of comparisons needed, especially beneficial when the dataset size grows.
Performance Benefits of Dual-Pivot Quicksort
The primary advantages of Dual-Pivot Quicksort include:
- Fewer Comparisons: Utilizing two pivots reduces the number of comparisons during partitioning.
- Improved Cache Efficiency: Better partitioning can help utilize the CPU cache efficiently, speeding up the sorting process.
- Better for Large Datasets: As dataset sizes increase, the benefits of fewer comparisons and better memory access patterns become more apparent.
According to various studies, Dual-Pivot Quicksort can outperform traditional Quicksort implementations, making it a preferred choice in many standard libraries, including Java's Arrays.sort() method.
Implementing Dual-Pivot Quicksort in Java
Let’s dive into the practical side and implement Dual-Pivot Quicksort in Java. This will give us a more concrete understanding of how it works in practice.
Dual-Pivot Quicksort Code Example
public class DualPivotQuicksort {
public static void dualPivotSort(int[] array, int low, int high) {
if (low < high) {
// Select two pivots
if (array[low] > array[high]) {
swap(array, low, high);
}
int pivot1 = array[low];
int pivot2 = array[high];
int less = low + 1;
int great = high - 1;
int i = low + 1;
// Partitioning process
while (i <= great) {
if (array[i] < pivot1) {
swap(array, i, less);
less++;
} else if (array[i] > pivot2) {
while (array[great] > pivot2 && i < great) {
great--;
}
swap(array, i, great);
great--;
if (array[i] < pivot1) {
swap(array, i, less);
less++;
}
}
i++;
}
less--;
great++;
// Swapping pivots into place
swap(array, low, less);
swap(array, high, great);
// Recursive sort on the three partitions
dualPivotSort(array, low, less - 1);
dualPivotSort(array, less + 1, great - 1);
dualPivotSort(array, great + 1, high);
}
}
private static void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
Code Commentary
- Pivot Selection: The two pivots are selected—ensuring they are in the correct order is crucial.
- Partitioning: The array is divided based on the pivot values. The
less
pointer tracks where elements less than the first pivot should go, while thegreat
pointer keeps track of elements more than the second pivot. - Recursive Calls: After a successful partitioning, the method recursively sorts the three resulting subarrays.
This implementation captures the essence of how Dual-Pivot Quicksort rearranges elements and performs efficiently.
Running the Code
To use the dualPivotSort
method, you can easily incorporate it into a driver function. Here's an example code snippet:
public static void main(String[] args) {
int[] array = {10, 7, 8, 9, 1, 5};
int n = array.length;
System.out.println("Original array:");
System.out.println(Arrays.toString(array));
dualPivotSort(array, 0, n - 1);
System.out.println("Sorted array:");
System.out.println(Arrays.toString(array));
}
Analyzing Performance
To benchmark the performance of your Dual-Pivot Quicksort implementation against other sorting algorithms, such as Merge Sort or the standard Quicksort, you can measure the time taken to sort large arrays.
Java provides excellent utilities to work with:
long startTime = System.nanoTime();
// Sorting logic
long endTime = System.nanoTime();
long duration = endTime - startTime; // Duration in nanoseconds
System.out.println("Sorting took " + duration + " nanoseconds");
The Bottom Line
Dual-Pivot Quicksort is a remarkable enhancement to the standard Quicksort algorithm, offering significant improvements in performance, especially for larger datasets. By understanding its mechanisms and implementation, you can optimize your applications significantly. The next time you're faced with sorting challenges, consider incorporating Dual-Pivot Quicksort into your toolkit.
For more in-depth discussions and advanced algorithms, you may want to check out GeeksforGeeks or Java Documentation.
Happy coding!