Debugging Application Context Hierarchy Issues in Spring Boot
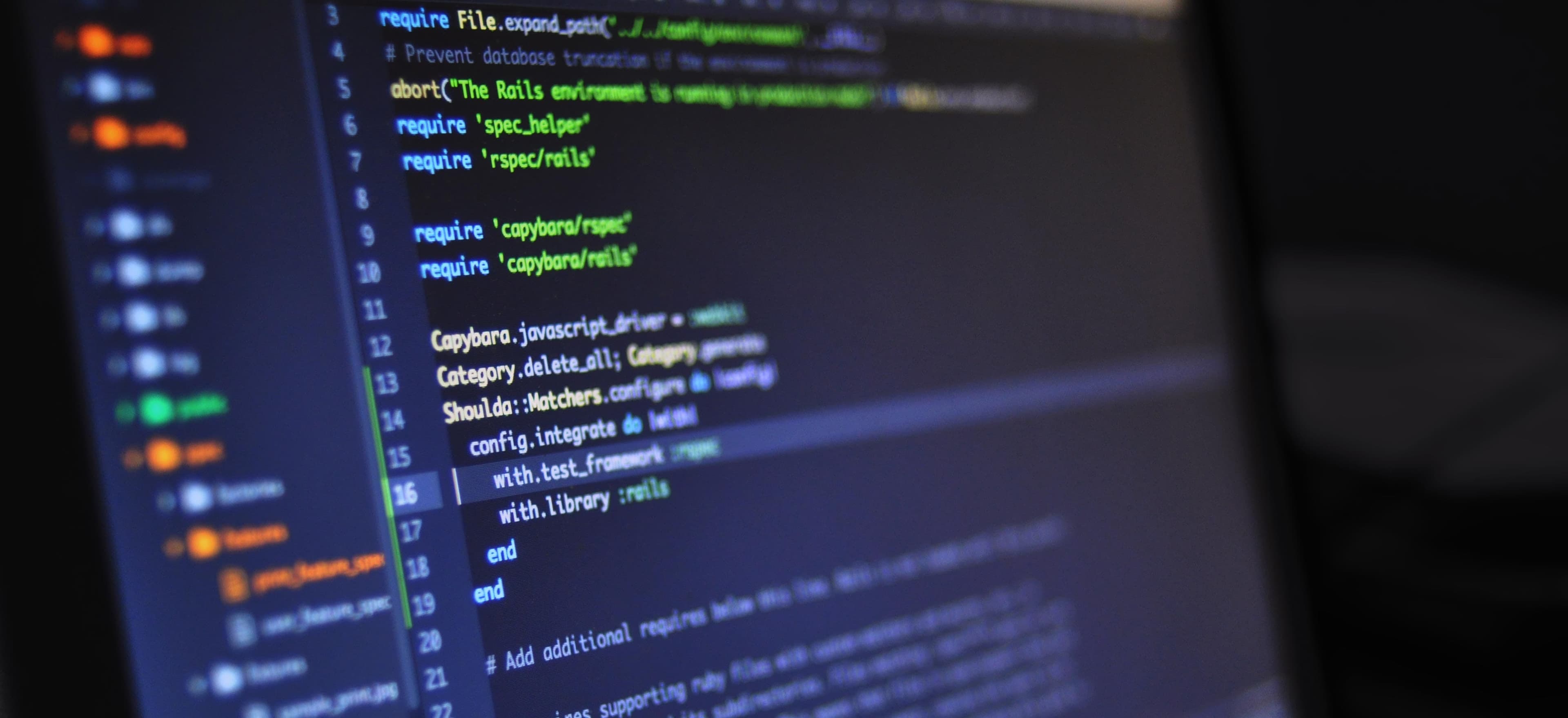
- Published on
Debugging Application Context Hierarchy Issues in Spring Boot
Stepping into the Topic
Spring Boot, with its powerful dependency injection and application context management capabilities, enables developers to create robust enterprise applications effortlessly. However, as applications grow in complexity and the number of application contexts increases, issues may arise—particularly in the context hierarchy. This blog post will explore common application context hierarchy issues in Spring Boot, offer debugging techniques, and provide practical examples to enhance understanding.
Understanding Spring's Application Context Hierarchy
What is an Application Context?
In the Spring framework, an Application Context is a central interface for providing configuration information to an application. It holds the beans that the application manages along with their dependencies. In simpler terms, it's the place where all your Spring beans live.
Context Hierarchy Explained
Spring allows for a parent-child relationship between application contexts. This can be particularly useful in large applications and enables modularization. However, this hierarchy can also be a source of confusion and potential issues, such as bean overriding, classpath issues, and scope problems.
Benefits of Using Context Hierarchies
-
Modularity: Different modules can have their contexts. For example, a web application can have a parent context that serves as a common configuration and separate child contexts for each module.
-
Resource Sharing: Common beans can reside in the parent context and be shared across child contexts, reducing redundancy.
-
Isolation: Each module can have its configuration, allowing for easy management and reduced coupling.
Common Issues in Application Context Hierarchies
Despite the benefits, developers often encounter issues related to application context hierarchies. Here are some common ones:
1. Bean Overriding
When multiple contexts define a bean with the same name, Spring may throw a BeanDefinitionStoreException
. Spring Boot has a default configuration to allow bean overriding, but it's not always desirable.
2. Classpath Scanning Conflicts
If multiple contexts scan the same packages without proper exclusions, it can lead to loading the same bean multiple times.
3. Scope Conflicts
Scopes define the lifecycle of beans. When a prototype-scoped bean is defined in a singleton context, it can lead to unexpected behaviors, particularly if the prototype bean is accessed through the singleton bean.
Debugging Techniques
Step 1: Enable Debug Logging
The first step in debugging any issue is to enable debug logging. In Spring Boot, you can do this by adding the following line to your application.properties
file:
logging.level.org.springframework=DEBUG
This setting allows you to see detailed logs related to bean creation and context loading.
Step 2: Analyze the Context Configuration
Inspect your application context configuration by printing out the beans. You can retrieve and display the names of all beans in your application context, helping you identify duplicates and conflicts.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class ApplicationContextAwareRunner implements CommandLineRunner {
@Autowired
private ApplicationContext applicationContext;
@Override
public void run(String... args) throws Exception {
String[] beanNames = applicationContext.getBeanDefinitionNames();
System.out.println("Beans in the context:");
for (String beanName : beanNames) {
System.out.println(beanName);
}
}
}
Why This Code?
This snippet retrieves all the beans in the application context and outputs their names. Analyzing this output can pinpoint bean name conflicts or misconfigurations.
Step 3: Check for @Primary and @Qualifier Annotations
If you have multiple beans of the same type, ensure you use the @Primary
annotation on the preferred bean, or use @Qualifier
to specify which bean to inject. For instance:
@Service
public class ServiceA {
// service implementation
}
@Service
@Primary
public class ServiceB {
// service implementation
}
@Controller
public class MyController {
private final ServiceA serviceA;
private final ServiceB serviceB;
@Autowired
public MyController(ServiceA serviceA, @Qualifier("serviceB") ServiceB serviceB) {
this.serviceA = serviceA;
this.serviceB = serviceB;
}
}
Why This Code?
The @Primary
annotation designates ServiceB
as the primary candidate for autowiring when multiple beans of type ServiceA
exist. Using @Qualifier
in the constructor helps specify which bean to inject where ambiguity arises.
Step 4: Investigate Parent-Child Context Interactions
When you set up parent-child contexts, make sure child contexts do not hide the beans from the parent. Always remember that child contexts can override parent beans with the same name. Here's an example:
@Configuration
public class ParentConfig {
@Bean
public MyService myService() {
return new MyService("from parent context");
}
}
@Configuration
public class ChildConfig {
@Bean
public MyService myService() {
return new MyService("from child context");
}
}
Why This Code?
This comparison demonstrates how a bean defined in the child context can overshadow the parent's equivalent bean. Understanding this behavior is crucial in avoiding unintended overrides.
Step 5: Test with Profiles
Using different Spring profiles can help isolate and test different contexts. You can enable specific profiles in your application.properties
file:
spring.profiles.active=dev
Why This Code?
Profiles allow you to simulate different environments, providing a mechanism to configure beans relevant to those environments. This is particularly useful when debugging context hierarchies.
To Wrap Things Up
Debugging application context hierarchy issues in Spring Boot can be challenging, but by following systematic techniques and employing the tips outlined in this post, you can resolve most issues that arise. Ensure you understand your context structure and utilize logging and configuration analysis to identify and fix problems effectively.
For an in-depth exploration of Spring Boot configurations, check out the official Spring Boot documentation.
As your Spring Boot application grows, keeping track of context hierarchy will become crucial. Proactive management and debugging can lead to smoother development and deployment processes, ultimately contributing to your application's success. Happy coding!
Checkout our other articles