Struggling with Custom JAX-RS in JBoss 7? Here’s the Fix!
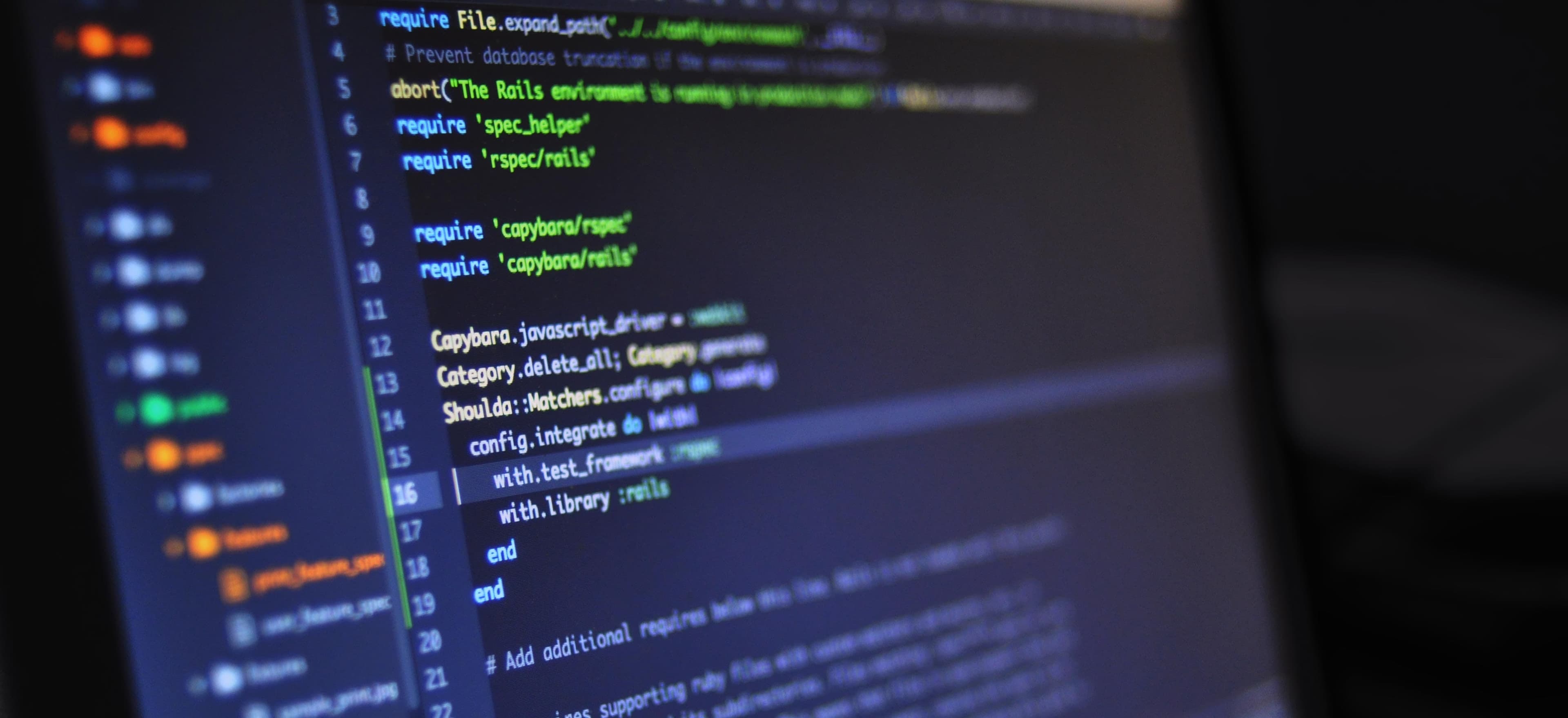
- Published on
Struggling with Custom JAX-RS in JBoss 7? Here’s the Fix!
Java developers often turn to JAX-RS for building RESTful web services. JBoss 7, with its robust support for JAX-RS, allows for straightforward application deployment. However, integrating custom JAX-RS components can be frustrating at times, especially for those new to the ecosystem. In this blog post, we'll delve into common problems faced when customizing JAX-RS on JBoss 7, and how to effectively solve them.
What is JAX-RS?
JAX-RS (Java API for RESTful Web Services) is a set of APIs to create web services that adhere to the REST architecture. It simplifies the development process, enabling Java developers to expose and consume RESTful services efficiently. It provides annotations that facilitate the mapping of HTTP requests to Java methods.
Why Use JBoss 7?
JBoss 7 provides a lightweight, powerful Java application server with advanced features like:
- Modular architecture: Reduces memory footprint.
- Management API: Simplifies server administration.
- Support for CDI: Enhances component-based development.
For more understanding of JBoss, you can check out the JBoss EAP documentation.
Common JAX-RS Customization Challenges in JBoss 7
-
Configuration Issues: Misconfigured deployment descriptors can lead to application failures or unintended behaviors. Understanding how to set up
web.xml
properly is crucial. -
Dependency Problems: Missing or conflicting library dependencies often cause runtime issues.
-
Exception Handling: Sometimes, the default exception handling in JAX-RS isn't sufficient. You may need a custom solution that returns user-friendly error messages.
Setting Up a Simple JAX-RS Application in JBoss 7
Let's create a basic RESTful API for managing books as an example. Below you'll find step-by-step instructions with code snippets.
Step 1: Create a Maven Project
Start by creating a Maven project with the following structure.
src
└── main
├── java
│ └── com
│ └── example
│ └── bookstore
│ ├── Book.java
│ ├── BookResource.java
│ └── AppConfig.java
└── resources
└── META-INF
└── persistence.xml
Step 2: Add Dependencies
In your pom.xml
, include the necessary dependencies for JAX-RS. Here's an example:
<dependencies>
<dependency>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
<version>2.1</version>
</dependency>
<dependency>
<groupId>org.jboss.resteasy</groupId>
<artifactId>resteasy-jaxrs</artifactId>
<version>3.6.3.Final</version>
</dependency>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
Commentary: The above dependencies include JAX-RS API, RESTEasy (JAX-RS implementation), and Java EE API which is required for JBoss 7.
Step 3: Create a Model Class (Book.java
)
The model represents your data. Here's a simple book class.
package com.example.bookstore;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class Book {
private String title;
private String author;
public Book() {}
public Book(String title, String author) {
this.title = title;
this.author = author;
}
// Getters and Setters
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
}
Commentary: The
@XmlRootElement
annotation is crucial. It allows the class to be converted to XML or JSON when sent as a response.
Step 4: Create the Resource Class (BookResource.java
)
The resource class handles incoming HTTP requests.
package com.example.bookstore;
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
import java.util.ArrayList;
import java.util.List;
@Path("/books")
public class BookResource {
private static List<Book> bookList = new ArrayList<>();
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<Book> getBooks() {
return bookList;
}
@POST
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public Book addBook(Book book) {
bookList.add(book);
return book;
}
}
Commentary: Here, we define two endpoints—
GET
retrieves the list of books, whilePOST
allows for adding a new book. Content type for responses and requests is managed using the@Produces
and@Consumes
annotations.
Step 5: Application Configuration (AppConfig.java
)
Define the application configuration.
package com.example.bookstore;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/api")
public class AppConfig extends Application {
}
Commentary: The
@ApplicationPath
annotation sets the base URI for all resource URIs provided by the application. This means all endpoints will start with/api
.
Step 6: Deployment Descriptor (web.xml
)
While JBoss 7 allows for auto-discovery, it's good to have a web.xml
.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<servlet>
<servlet-name>resteasy-servlet</servlet-name>
<servlet-class>org.jboss.resteasy.plugins.server.servlet.HttpServletDispatcher</servlet-class>
<init-param>
<param-name>javax.ws.rs.Application</param-name>
<param-value>com.example.bookstore.AppConfig</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>resteasy-servlet</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
</web-app>
Commentary: Here we define a servlet for JAX-RS and set the servlet mapping for
api/*
. This setup ensures that all REST calls are routed appropriately.
Step 7: Running the Application
- Package your application with Maven using
mvn clean package
. - Deploy the generated WAR file to your JBoss 7 instance.
Troubleshooting Common Issues
-
HTTP 404 Errors: Check if your package and path mappings are correctly defined. Ensure that the
@ApplicationPath
is accurately set. -
ClassNotFound Exception: If you encounter this error, verify your dependencies in the
pom.xml
. Sometimes, incompatible versions can cause issues. -
Dependency Conflicts: Clean the Maven cache by running
mvn clean install
to ensure all dependencies are correctly resolved.
Custom Exception Handling Example
To enhance user experience, you might want to add custom exception handling.
import javax.ws.rs.*;
import javax.ws.rs.core.Response;
@Provider
public class CustomExceptionMapper implements ExceptionMapper<Exception> {
@Override
public Response toResponse(Exception exception) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity(exception.getMessage())
.type(MediaType.TEXT_PLAIN)
.build();
}
}
Commentary: The above custom exception mapper can capture general exceptions emitted by the resource classes, making your API more robust.
Bringing It All Together
Customizing JAX-RS in JBoss 7 may seem daunting initially, but with a clear understanding of its components and relevant configurations, the process can be smooth and efficient. By following the structured approach in this article, you can design your RESTful APIs and handle exceptions gracefully.
For further reading on the JAX-RS specification, visit the JAX-RS documentation.
Final Thoughts
Always remember to test thoroughly. Unit tests enhance stability and performance, confirming that your JAX-RS application responds correctly. With these solutions at your disposal, you're equipped to tackle any hurdles you might face in your journey of creating custom RESTful services.
Feel free to reach out if you have any questions or need further assistance!
Checkout our other articles