Common DAO and Service Layer Pitfalls in Spring Applications
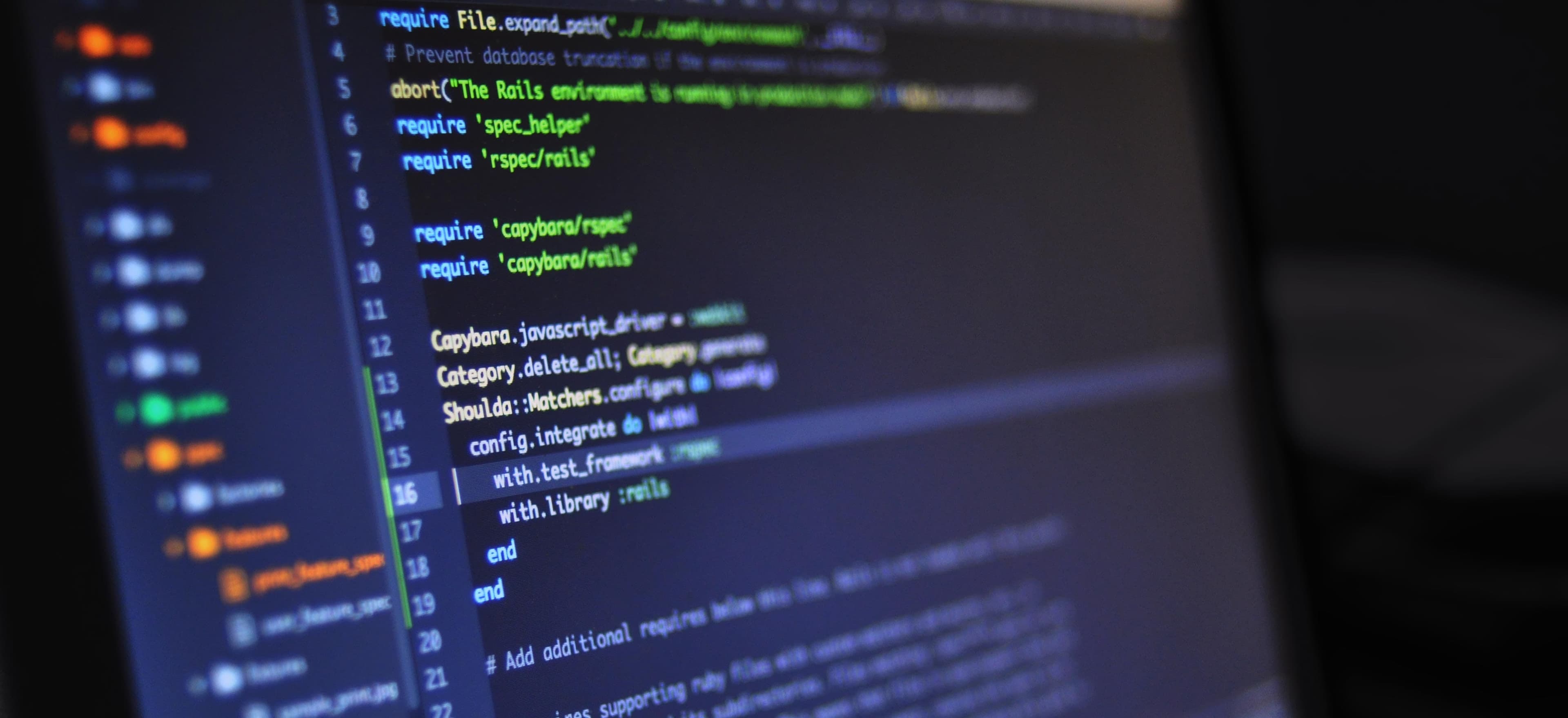
- Published on
Common DAO and Service Layer Pitfalls in Spring Applications
Spring Framework has revolutionized the way developers build enterprise applications. By separating concerns between data access and business logic, it allows for better organization and maintainability. However, even seasoned developers can fall victim to common pitfalls in the Data Access Object (DAO) and Service layers. This blog post will discuss these pitfalls, highlight their implications, and provide practical solutions to avoid them.
Understanding DAO and Service Layers
What is DAO?
The DAO pattern is a structural pattern that separates the data persistence logic from the rest of the application. DAOs are responsible for interacting with the database and returning domain objects to the Service layer. Here’s a simplistic example:
@Repository
public class UserDao {
@Autowired
private JdbcTemplate jdbcTemplate;
public User findById(Long id) {
String sql = "SELECT * FROM users WHERE id = ?";
return jdbcTemplate.queryForObject(sql, new Object[]{id}, new UserRowMapper());
}
}
In this example, the UserDao
class encapsulates all database operations related to the User
entity.
What is Service Layer?
The Service layer contains business logic and orchestrates operations involving multiple DAOs. It serves as a bridge between the presentation layer and the data access layer.
@Service
public class UserService {
@Autowired
private UserDao userDao;
public User getUser(Long id) {
return userDao.findById(id);
}
}
Here, the UserService
class retrieves user information while keeping business rules intact.
Common Pitfalls
1. Tight Coupling Between DAO and Service Layers
The Problem: When DAO and Service layers are tightly coupled, changes in one layer might impact the other. This makes the application hard to maintain and reduces testability.
Solution: Use interfaces to abstract the DAO implementation. This promotes loose coupling and improves flexibility.
public interface UserDao {
User findById(Long id);
}
Now, you can create multiple implementations of UserDao
without affecting the UserService
class.
2. Ignoring Transaction Management
The Problem: Transactions are crucial for ensuring data integrity. Neglecting them might lead to partial updates or inconsistent data.
Solution: Use Spring's declarative transaction management. Annotate your service methods with @Transactional
.
@Service
public class UserService {
@Autowired
private UserDao userDao;
@Transactional
public void updateUser(User user) {
userDao.update(user);
}
}
By marking the method with @Transactional
, Spring handles the transaction automatically, rolling back in case of an exception.
3. Overloading the Service Layer with Logic
The Problem: If you lean too heavily on the Service layer for complex business logic, it can become bloated and difficult to manage.
Solution: Keep the Service layer focused on orchestrating calls between DAOs and perform simple business validations. Extract complex logic into separate utility classes or components.
@Service
public class UserService {
@Autowired
private UserDao userDao;
public User getUser(Long id) {
// Simple validation logic
if (id == null) {
throw new IllegalArgumentException("ID must not be null");
}
return userDao.findById(id);
}
}
4. Not Handling Exceptions Properly
The Problem: Catching exceptions at the DAO layer and failing to propagate meaningful messages can lead to confusion and difficulty in debugging.
Solution: Wrap checked exceptions into runtime exceptions and use custom exception classes. This provides clearer error information.
public class UserNotFoundException extends RuntimeException {
public UserNotFoundException(Long id) {
super("User not found with ID: " + id);
}
}
public class UserDao {
public User findById(Long id) {
// Fetch user...
if (user == null) {
throw new UserNotFoundException(id);
}
return user;
}
}
5. Excessive Use of @Autowired
and Implicit Dependencies
The Problem: Relying too heavily on @Autowired
can lead to a lack of clarity around class dependencies, making it hard to track what a class requires.
Solution: Use constructor injection instead. This not only clarifies dependencies but makes unit testing easier.
@Service
public class UserService {
private final UserDao userDao;
@Autowired
public UserService(UserDao userDao) {
this.userDao = userDao;
}
public User getUser(Long id) {
return userDao.findById(id);
}
}
6. Neglecting Caching
The Problem: For repeated queries, constantly accessing the database can lead to performance bottlenecks.
Solution: Implement caching in your application. Spring provides a comprehensive caching abstraction that makes it relatively straightforward.
@Service
public class UserService {
@Autowired
private UserDao userDao;
@Cacheable("users")
public User getUser(Long id) {
return userDao.findById(id);
}
}
7. Deficient Unit Tests
The Problem: Failing to test the DAO and Service layers undermines the robustness of your application. When issues arise, the source may be obscured.
Solution: Write unit tests using frameworks like JUnit and Mockito.
@RunWith(MockitoJUnitRunner.class)
public class UserServiceTest {
@InjectMocks
private UserService userService;
@Mock
private UserDao userDao;
@Test
public void testGetUser() {
User mockUser = new User(1L, "testUser");
when(userDao.findById(1L)).thenReturn(mockUser);
User user = userService.getUser(1L);
assertEquals("testUser", user.getUsername());
verify(userDao).findById(1L);
}
}
Final Thoughts
Incorporating well-structured DAO and Service layers is essential for building scalable, maintainable Spring applications. By being aware of these common pitfalls and addressing them proactively, you can enhance the quality of your codebase and seamlessly manage complexities as your application grows.
Further Reading
- Spring Framework Documentation
- Best Practices for DAO Layer in Spring
- Understanding Spring Transaction Management
By taking the time to absorb these insights and implementing them into your development practices, you will not only improve your own work but also contribute to the health and longevity of your applications. Happy coding!
Checkout our other articles