Mastering the Peek Method: Common Misuses in Java Streams
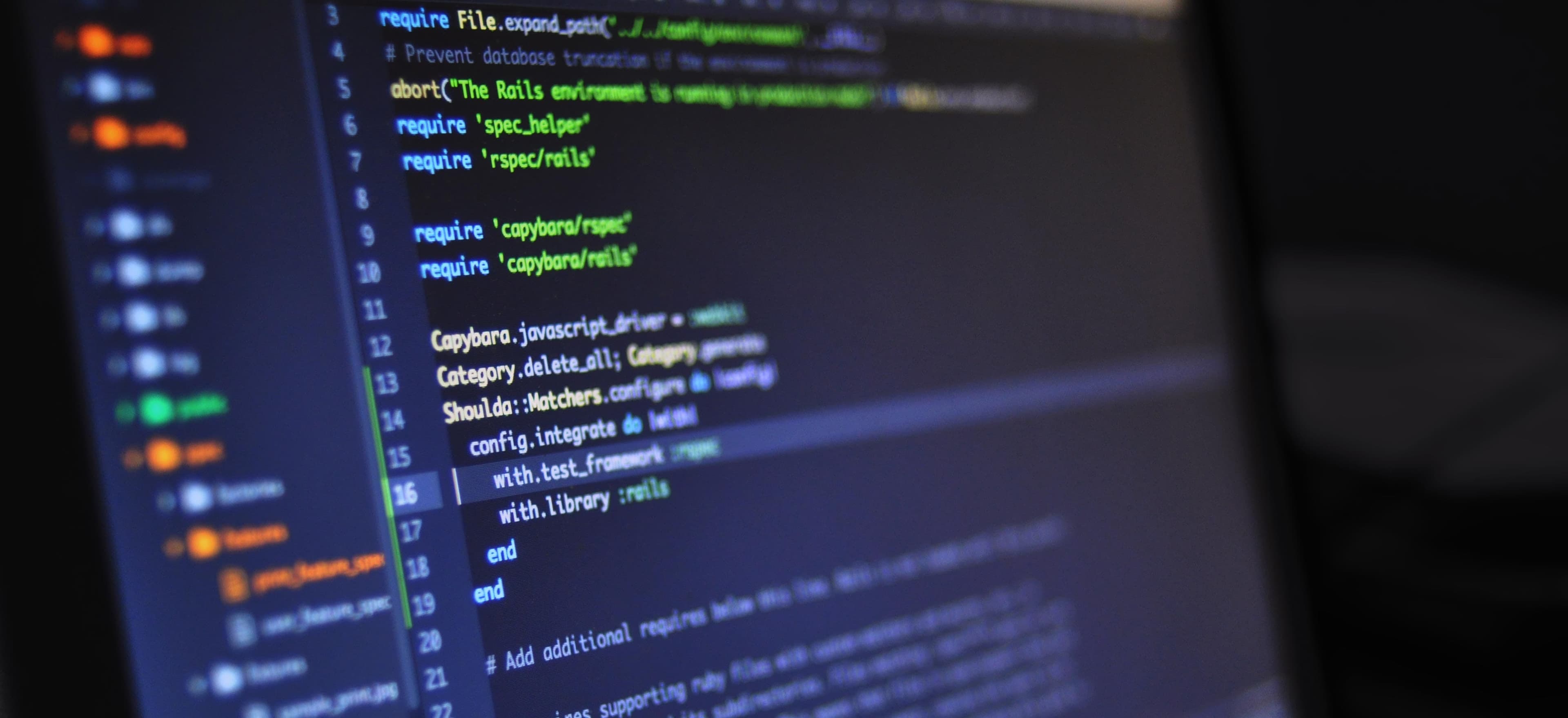
- Published on
Mastering the Peek Method: Common Misuses in Java Streams
Java Streams have transformed the way we manipulate collections of data. They provide a functional approach to processing data, making tasks easier and more intuitive. One of the methods available in the Stream API is the peek
method. While it can be incredibly useful, there are common pitfalls that developers encounter when using it. In this post, we'll explore the peek
method, the common misuses associated with it, and best practices to keep your code clean and efficient.
Understanding the peek
Method
The peek
method is an intermediate operation that allows you to perform an action on each element as it passes through the stream. This is particularly useful for debugging purposes or when you want to perform side-effects without altering the stream itself.
Syntax
Stream<T> peek(Consumer<? super T> action);
Example Usage
Consider a simple example where we want to process a list of integers and print each integer as it is processed:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.stream()
.peek(n -> System.out.println("Processing: " + n))
.map(n -> n * 2)
.forEach(System.out::println);
In the code above, the peek
method is used to print each number before it’s doubled. The output will be:
Processing: 1
Processing: 2
Processing: 3
Processing: 4
Processing: 5
2
4
6
8
10
Common Misuses of the peek
Method
1. Using peek
for Mutation
One of the most common misuses of peek
is to perform mutations on the elements within the stream. The idea of using peek
to change the elements goes against the functional paradigm promoted by Stream API. Instead, consider using mapping operations.
Misuse Example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.stream()
.peek(name -> name.toUpperCase()) // Incorrect usage
.forEach(System.out::println);
This snippet does not change the original list due to the way strings are handled in Java (they are immutable), leading to confusion.
Correct Usage:
Instead of mutating within peek
, use map
to transform the data:
names.stream()
.map(String::toUpperCase) // Correct usage
.forEach(System.out::println);
2. Overusing peek
for Debugging
While peek
can be handy for debugging, relying on it too much can lead to messy code. You can lose track of the stream's flow if too many peek
calls are scattered throughout your operations.
Example:
List<String> items = Arrays.asList("Apple", "Banana", "Cherry");
items.stream()
.peek(item -> System.out.println("Initial: " + item))
.map(String::toLowerCase)
.peek(item -> System.out.println("Lowercased: " + item))
.filter(item -> item.startsWith("a"))
.peek(item -> System.out.println("Filtered: " + item))
.forEach(System.out::println);
Although this example shows the internal state at each step, it can clutter your output and make the stream's transformations harder to read.
Best Practice:
Instead of using multiple peek statements, it’s better to use logging tools or debugging sessions.
3. Ignoring the Lazy Nature of Streams
Streams are processed lazily, meaning that the operations are not executed until a terminal operation is invoked. This can lead to confusion if you think peek
will cause side effects immediately.
Example:
List<Integer> list = Arrays.asList(1, 2, 3);
list.stream()
.peek(i -> System.out.println("Before: " + i))
.filter(i -> i > 1);
In this case, you may assume that "Before: 1" will be printed, but it will not appear because no terminal operation is called.
Correct Approach:
Always ensure that an action that triggers the stream processing follows the intermediate operations.
list.stream()
.peek(i -> System.out.println("Before: " + i))
.filter(i -> i > 1)
.forEach(System.out::println);
When to Use peek
Effectively
- Debugging: Use
peek
within small scopes to understand what data is flowing through the stream. However, aim to limit this to a few calls or remove them once you finish debugging. - Logging: If you need simply to output information for logging purposes, using
peek
can be justified. Just remember it should not be used to mutate data.
Conclusion
The peek
method in Java Streams is a powerful tool, but it can easily be misused. By understanding its intended purpose and knowing the common pitfalls, you can use it effectively in your applications.
For more detailed exploration of Java Streams and their functionalities, check out the official Java Documentation and explore best practices for functional programming in Java.
By mastering the nuances of methods like peek
and applying best practices, you can create efficient, clean, and understandable code that leverages the full potential of Java Streams!
Happy coding! Remember that mastery of Java's Stream API comes with practice and continuous learning. If you have any questions or experiences to share, feel free to add a comment below!