Improving Cardano Mempool Monitoring with Yaci Library
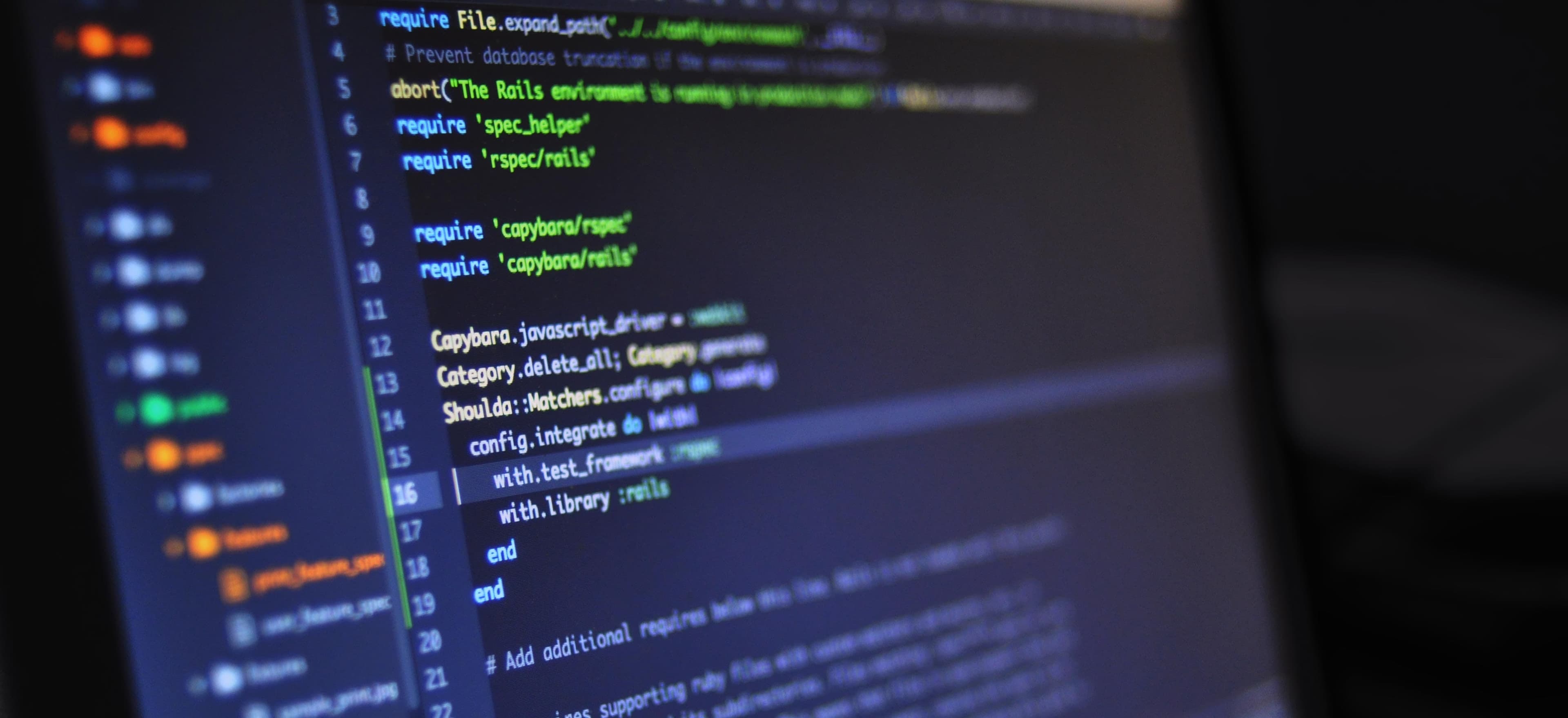
- Published on
Improving Cardano Mempool Monitoring with Yaci Library
The Cardano blockchain has gained remarkable traction as a decentralized platform that emphasizes security, scalability, and sustainability. Consequently, monitoring transactions is crucial for developers and stakeholders involved in the Cardano ecosystem. One effective way to enhance your application’s performance and integrity is to keep track of the mempool — the set of transactions that have not yet been confirmed.
This blog post will guide you through the concept of mempool monitoring in Cardano and how the Yaci library can simplify this process. Whether you're a beginner or an advanced developer, understanding and implementing effective monitoring can significantly elevate your project.
Understanding the Cardano Mempool
Before diving into specifics, let’s establish what the mempool is. The mempool acts as a waiting area for transactions that have been broadcasted to the Cardano network but have not yet been included in a block. Transactions remain in the mempool until they are confirmed or dropped due to network fees or other conditions.
Why Mempool Monitoring?
-
Transaction Analysis: By observing the mempool, developers can analyze the frequency, size, and type of transactions being processed, aiding in strategic decision-making.
-
Performance Metrics: Monitoring the processing time of transactions can offer insights into network performance and the efficiency of user applications.
-
Predictions and Strategies: With historical data on transactions, one can formulate predictions on pending transaction rates and strategize fee settings accordingly.
Introducing Yaci Library
Yaci is a powerful library designed for monitoring the Cardano mempool. It offers a set of simple and efficient functions to interact with the Cardano network, making it easier for developers to manage transactions and monitor their state. Built with speed and usability in mind, Yaci allows for streamlined interactions, whether you are a solo developer or part of a larger team.
Features of Yaci
- Lightweight: Fast and easy to integrate into existing projects.
- Real-time Updates: Provides real-time updates, ensuring you get the data you need without delay.
- User-friendly API: Simple functions that require minimal setup.
Installation
Before you start using the Yaci library, you need to ensure that you have the required libraries installed. Here's how to install Yaci using Maven. Simply add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.cardano.yaci</groupId>
<artifactId>yaci-core</artifactId>
<version>latest_version</version>
</dependency>
Replace latest_version
with the most recent version available.
Code Example: Monitoring the Mempool
Let’s go through a basic example of monitoring the Cardano mempool using Yaci. This example will demonstrate how to fetch the mempool data, analyze it, and extract meaningful insights from it.
Step 1: Fetching Mempool Data
import org.cardano.yaci.core.Yaci;
import org.cardano.yaci.core.models.MempoolTransaction;
import java.util.List;
public class MempoolMonitor {
private Yaci yaciClient;
public MempoolMonitor() {
yaciClient = new Yaci("http://localhost:8080"); // ensure your Yaci node URL is correct
}
public List<MempoolTransaction> getMempoolTransactions() {
List<MempoolTransaction> mempoolTransactions = yaciClient.getMempoolTransactions();
return mempoolTransactions;
}
}
Why This Matters
- Yaci Client Initialization: At the outset, we create an instance of the Yaci client, ensuring it's connected to the appropriate Cardano node.
- Fetching Transactions: The
getMempoolTransactions
method fetches all transactions currently in the mempool, providing an essential insight into what's pending.
Step 2: Analyzing Transactions
You may want to analyze transactions to understand their nature. Let’s filter these transactions based on a simple metric: the transaction fee.
import java.util.stream.Collectors;
public List<MempoolTransaction> filterHighFeeTransactions(List<MempoolTransaction> transactions, long feeThreshold) {
return transactions.stream()
.filter(transaction -> transaction.getFee() > feeThreshold)
.collect(Collectors.toList());
}
This method filters transactions with fees exceeding a given threshold, allowing you to focus on potentially critical transactions.
Step 3: Displaying the Data
Finally, it would be beneficial to display the filtered data in a readable format:
public void displayTransactions(List<MempoolTransaction> transactions) {
for (MempoolTransaction transaction : transactions) {
System.out.println("Transaction ID: " + transaction.getId());
System.out.println("Fee: " + transaction.getFee());
System.out.println("Sender: " + transaction.getSender());
System.out.println("Timestamp: " + transaction.getTimestamp());
System.out.println("-------------------");
}
}
Why Show This Data?
Displaying the data allows developers to make informed decisions quickly. You can easily communicate transaction statuses and analyze user patterns.
Additional Context
For broader context related to Cardano and mempool monitoring, consider checking out the Cardano documentation and the Yaci GitHub repository. These resources provide comprehensive information that expands on the concepts discussed in this post.
Advanced Monitoring Techniques
While basic monitoring is essential, there are advanced techniques that can further improve your application's robustness.
Real-time Notifications
By integrating a notification system – like WebSockets – you can be alerted whenever new transactions are added to the mempool. This immediate feedback loop enhances responsiveness.
Historical Analysis
Storing mempool data over time can help analyze trends in transaction volumes, fees, and types, allowing for improved forecasting and decision-making.
Wrapping Up
Monitoring the Cardano mempool is an integral aspect of building robust applications in the blockchain space. With the Yaci library, you can easily retrieve and analyze mempool transactions, paving the way for improved performance and user satisfaction.
Implementing effective monitoring not only enhances your application's efficiency but also positions you to make well-informed decisions for future developments. Start utilizing Yaci today and elevate your integration with the Cardano network.
By following the structure and examples provided in this article, you should now have a clearer understanding of how to utilize the Yaci library for seamless Cardano mempool monitoring. Happy coding!
Checkout our other articles