Troubleshooting Common Spring Integration Setup Issues
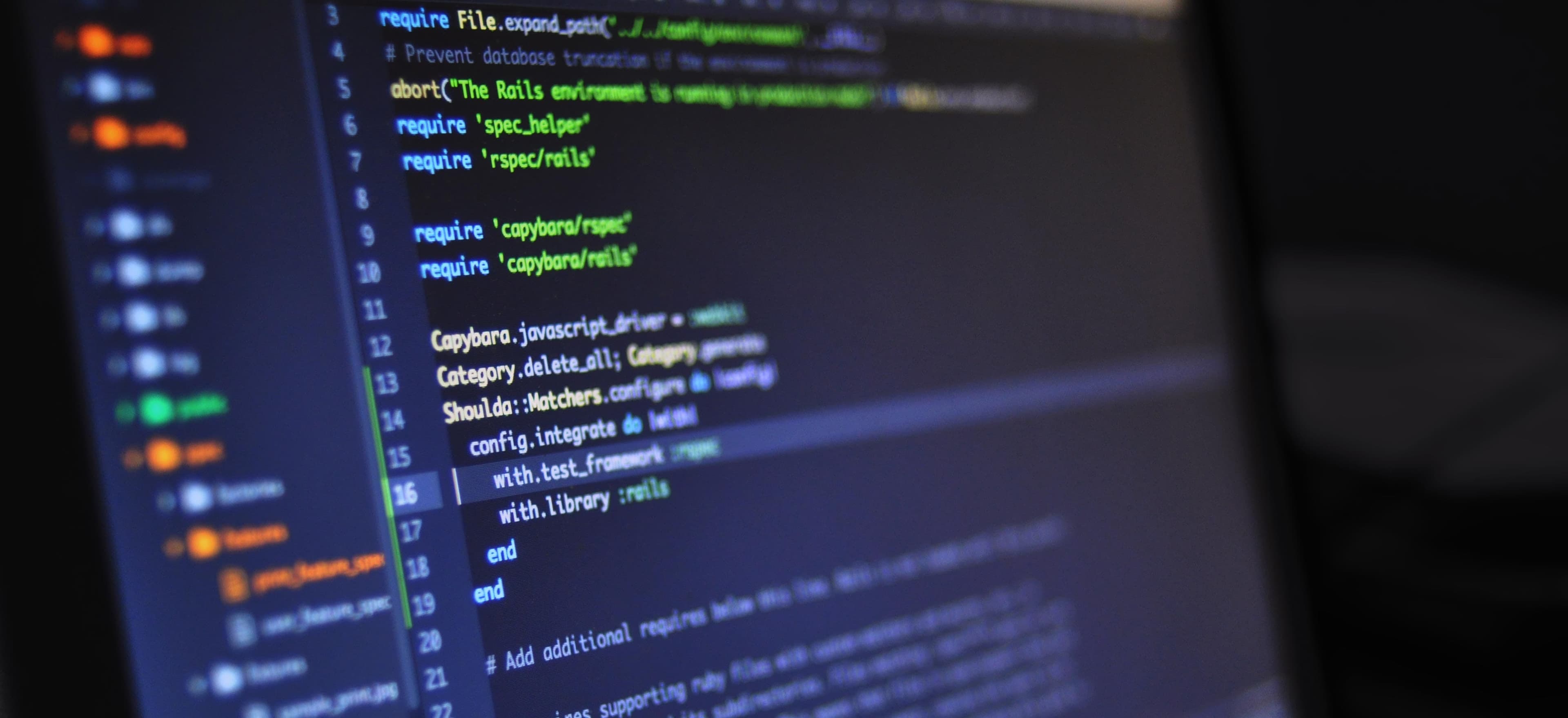
- Published on
Troubleshooting Common Spring Integration Setup Issues
Spring Integration is a powerful framework that makes it easy to build enterprise integration solutions using Spring. However, as with any technology, you may occasionally encounter issues during setup. This blog post will walk you through some of the most common troubleshooting problems and their solutions.
Understanding Spring Integration
Before we get into the troubleshooting tips, let's briefly discuss what Spring Integration is. It is an extension of the Spring framework that allows developers to design event-driven architectures as well as integrate various systems and applications in a decoupled manner. It utilizes various components such as channels, message endpoints, and service activators to facilitate communication.
For anyone getting started, I recommend checking out the official Spring Integration Reference Documentation for more insights.
Common Setup Issues in Spring Integration
While setting up Spring Integration, you may face several issues. Here we will discuss troubleshooting strategies for the top common issues.
1. Missing Spring Integration Dependencies
One of the most common issues is forgetting to include the necessary Spring Integration dependencies in your project’s build configuration. Without these dependencies, you will face issues ranging from compilation errors to missing beans.
Solution:
Make sure you've added the correct dependencies in your pom.xml
for Maven or build.gradle
for Gradle.
Maven Example:
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-core</artifactId>
<version>5.5.3</version> <!--Use the latest stable version-->
</dependency>
Gradle Example:
implementation 'org.springframework.integration:spring-integration-core:5.5.3'
2. Configuration Errors
Spring Integration configurations can get messy, especially if you are mixing Java configuration with XML configuration. Configuration errors can lead to application context failures that are sometimes tricky to diagnose.
Using Java Configuration
When using Java configuration, ensure that your configuration class is annotated with @Configuration
and your beans are properly defined.
Example:
@Configuration
@EnableIntegration
public class IntegrationConfig {
@Bean
public MessageChannel myChannel() {
return new DirectChannel();
}
@Bean
@ServiceActivator(inputChannel = "myChannel")
public MyServiceActivator myServiceActivator() {
return new MyServiceActivator();
}
}
Why: Using @EnableIntegration
correctly initializes the context for Spring Integration, allowing the framework to register the required components.
Troubleshooting Tip:
- Check the application’s startup logs for exceptions related to bean creation or configuration issues.
3. Message Channel Mismatches
A very typical issue in Spring Integration is having message channels that don’t match between the producers and consumers. If a producer sends messages to a channel that no subscribers are listening to, the messages simply get dropped.
Solution: Double-check that the names of your channels match between the sender and receiver.
Example:
@Bean
public MessageChannel myChannel() {
return new DirectChannel();
}
// Producer
@Service
public class MyProducer {
@Autowired
private MessageChannel myChannel;
public void sendMessage(String messageContent) {
myChannel.send(MessageBuilder.withPayload(messageContent).build());
}
}
Why: Consistency in messaging ensures that producers are pushing messages to a channel that is actively handling those messages.
4. Endpoint Configuration Errors
The configuration of service activators, gateways, and other endpoints may sometimes lead to confusion. Misconfigured endpoints can lead to unexpected behavior or runtime exceptions.
Example
Ensure that all service activators are correctly set up and have valid request and reply channels.
Example:
@Bean
@ServiceActivator(inputChannel = "inputChannel", outputChannel = "outputChannel")
public MyService myService() {
return new MyService();
}
Why: The input and output channels directly influence how data is flowing through your application, and ensuring they are correctly aligned is key to achieving the desired outcome.
5. Testing with Integration Tests
Integration testing can be complex. But a good set of tests will help catch issues early. Sometimes, beans may not work in tests due to misconfiguration or missing context information.
Tip:
Make sure to set up your test classes correctly using @SpringBootTest
and to define the necessary beans in the context.
@SpringBootTest
public class MyIntegrationTest {
@Autowired
private MyService myService;
@Test
public void testService() {
// Perform your assertions here
}
}
Why: Properly running tests within the Spring context helps ensure that all configurations are coming together as expected.
6. Debugging and Logging
When facing issues in Spring Integration, enabling detailed logging can provide insights into the flow of messages and the state of the application's components.
Solution: Adjust your logging configuration.
# application.properties
logging.level.org.springframework.integration=DEBUG
Why: By changing the logging level, you will see detailed output of message flows, which can help identify bottlenecks or misconfigurations.
The Bottom Line
Setting up Spring Integration can be smooth sailing, but issues can arise that may seem daunting. By following the troubleshooting strategies outlined in this post — from ensuring you have the right dependencies to verifying correct channel configurations — you can handle these challenges effectively.
Spring Integration is rich and powerful, empowering developers to build robust integration solutions. Each problem you solve further hones your craft and deepens your understanding of the framework.
For more specialized topics on Spring Integration or further reading on building your integration solutions, visit Baeldung's Spring Integration Tutorials.
When you encounter issues, take a step back, revert to basics, review your configurations, and leverage the powerful features that Spring gives you for troubleshooting. Happy coding!